Hướng dẫn và ví dụ Java List
1. List
List là một interface nằm trong nền tảng tập hợp của Java (Java Collection Framework), nó là một interface con của Collection vì vậy nó có đầy đủ các tính năng của một Collection, ngoài ra nó cung cấp cơ sở để duy trì một Collection có thứ tự (ordered Collection). Nó bao gồm các phương thức cho phép chèn, cập nhập, xoá và tìm kiếm một phần tử theo chỉ số. List cho phép các phần tử trùng lặp, và các phần tử null.
public interface List<E> extends Collection<E>
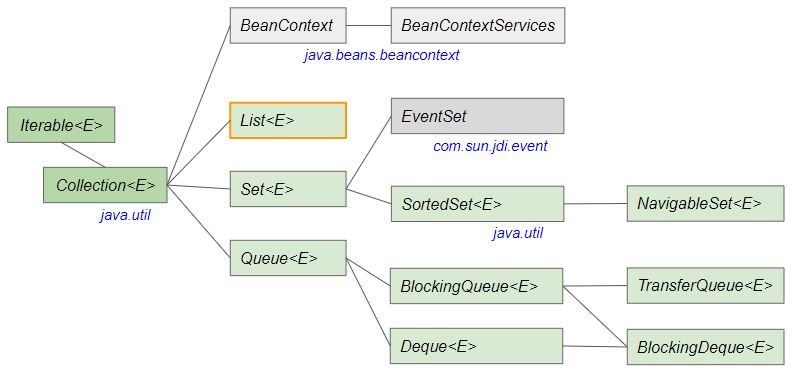
- Collection
- Queue
- Set
- SortedSet
- NavigableSet
- BlockingQueue
- BlockingDeque
- Deque
- TransferQueue
Hệ thống phân cấp các lớp thi hành (implement) interface List:
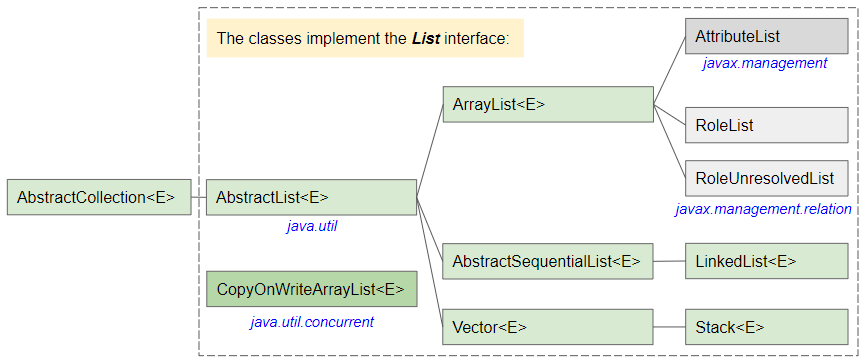
- LinkedList
- ArrayList
- CopyOnWriteArrayList
- Vector
- Stack
LinkedList là một lớp đặc biệt, nó vừa thuộc nhóm List vừa thuộc nhóm Queue:
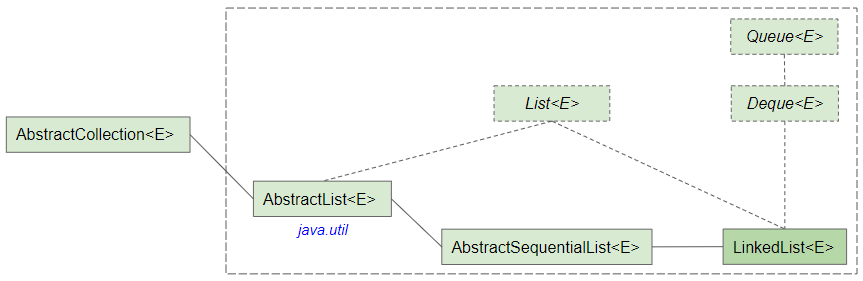
java.util.List
List cung cấp phương thức listIterator() trả về một đối tượng ListIterator cho phép lặp (iterate) trên các phần từ theo hướng tiến hoặc lùi. Các lớp thi hành interface List có thể kể tới là ArrayList, LinkedList, Stack, Vector. Trong đó ArrayList và LinkedList được sử dụng rộng rãi hơn cả.
Các phương thức được định nghĩa trong List:
List interface
public interface List<E> extends Collection<E> {
boolean isEmpty();
boolean contains(Object o);
Object[] toArray();
<T> T[] toArray(T[] a);
boolean containsAll(Collection<?> c);
boolean addAll(Collection<? extends E> c);
boolean addAll(int index, Collection<? extends E> c);
boolean removeAll(Collection<?> c);
boolean retainAll(Collection<?> c);
int size();
boolean equals(Object o);
int hashCode();
E get(int index);
E set(int index, E element);
boolean add(E e);
void add(int index, E element);
E remove(int index);
boolean remove(Object o);
void clear();
int indexOf(Object o);
int lastIndexOf(Object o);
Iterator<E> iterator();
ListIterator<E> listIterator();
ListIterator<E> listIterator(int index);
List<E> subList(int fromIndex, int toIndex);
default Spliterator<E> spliterator()
default void replaceAll(UnaryOperator<E> operator)
default void sort(Comparator<? super E> c)
static <E> List<E> of()
static <E> List<E> of(E e1)
static <E> List<E> of(E e1, E e2)
static <E> List<E> of(E e1, E e2, E e3)
static <E> List<E> of(E e1, E e2, E e3, E e4)
static <E> List<E> of(E e1, E e2, E e3, E e4, E e5)
static <E> List<E> of(E e1, E e2, E e3, E e4, E e5, E e6)
static <E> List<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7)
static <E> List<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8)
static <E> List<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8, E e9)
static <E> List<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8, E e9, E e10)
static <E> List<E> of(E... elements)
static <E> List<E> copyOf(Collection<? extends E> coll)
}
2. Examples
List là một interface, vì vậy để tạo một đối tượng List bạn cần tạo thông qua một lớp thi hành nó, chẳng hạn ArrayList, LinkedList,..
ListEx1.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class ListEx1 {
public static void main(String[] args) {
// Create a List
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Three");
list.add("Four");
// Insert an element at index 1
list.add(1, "Two");
for(String s: list) {
System.out.println(s);
}
System.out.println(" ----- ");
// Remove an element at index 2
list.remove(2);
for(String s: list) {
System.out.println(s);
}
}
}
Output:
One
Two
Three
Four
-----
One
Two
Four
Book.java
package org.o7planning.list.ex;
public class Book {
private String title;
private float price;
public Book(String title, float price) {
this.title = title;
this.price = price;
}
public String getTitle() {
return title;
}
public float getPrice() {
return price;
}
}
Ví dụ: Một đối tượng List chứa các kiểu dữ liệu Book.
ListEx2.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class ListEx2 {
public static void main(String[] args) {
Book b1 = new Book("Python Tutorial", 100f);
Book b2 = new Book("HTML Tutorial", 120f);
// Create an Unmodifiable List
List<Book> bookList1 = List.of(b1, b2);
// Create a List of Books using ArrayList
List<Book> bookList = new ArrayList<Book>();
bookList.add(new Book("Java Tutorial", 300f));
bookList.add(new Book("C# Tutorial", 200f));
// Append all elements to the end of list.
bookList.addAll(bookList1);
for(Book book: bookList) {
System.out.println(book.getTitle() + " / " + book.getPrice());
}
}
}
Output:
Java Tutorial / 300.0
C# Tutorial / 200.0
Python Tutorial / 100.0
HTML Tutorial / 120.0
Array -> List
Phương thức tĩnh Arrays.asList(..) cho phép chuyển đổi một mảng thành một đối tượng List, tuy nhiên đối tượng này có kích thước cố định, không thể thêm hoặc loại bỏ các phần tử sẵn có, vì nó không hỗ trợ các phương thức tuỳ chọn: add, remove, set và clear.
ListEx3.java
package org.o7planning.list.ex;
import java.util.Arrays;
import java.util.List;
public class ListEx3 {
public static void main(String[] args) {
Book b1 = new Book("Python Tutorial", 100f);
Book b2 = new Book("HTML Tutorial", 120f);
Book b3 = new Book("Java Tutorial", 300f);
Book[] bookArray = new Book[] {b1, b2, b3};
// Create a Fixed-size List.
List<Book> bookList = Arrays.asList(bookArray);
for(Book b: bookList) {
System.out.println("Book: " + b.getTitle());
}
}
}
Output:
Book: Python Tutorial
Book: HTML Tutorial
Book: Java Tutorial
3. listInterator()
Phương thức listIterator() trả về một đối tượng ListIterator cho phép bạn lặp (iterate) trên các phần tử của danh sách theo chiều tiến hoặc lùi.
ListIterator<E> listIterator();
ListIterator<E> listIterator(int index);
Ví dụ:
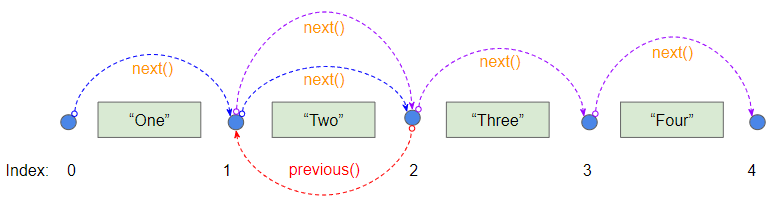
List_listIterator.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class List_listIterator {
public static void main(String[] args) {
// Create a List
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Get a ListIterator.
ListIterator<String> listIterator = list.listIterator();
String first = listIterator.next();
System.out.println("First:" + first);// -->"One"
String second = listIterator.next();
System.out.println("Second:" + second);// -->"Two"
if (listIterator.hasPrevious()) {
System.out.println("Jump back...");
String value = listIterator.previous();
System.out.println("Value:" + value);// -->"Two"
}
System.out.println(" ----- ");
while (listIterator.hasNext()) {
String value = listIterator.next();
System.out.println("Value:" + value);
}
}
}
Output:
First:One
Second:Two
Jump back...
Value:Two
-----
Value:Two
Value:Three
Value:Four
4. stream()
List là một interface con của Collection, vì vậy nó được thừa kế phương thức stream(). Truy cập vào các phần tử của một Collection thông qua Stream sẽ giúp code của bạn ngắn gọn và dễ hiểu hơn:
- Collection
- Stream
List_stream.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class List_stream {
public static void main(String[] args) {
Book b1 = new Book("Python Tutorial", 100f);
Book b2 = new Book("HTML Tutorial", 120f);
// Create an Unmodifiable List
List<Book> bookList1 = List.of(b1, b2);
// Create a List of Books using ArrayList
List<Book> bookList = new ArrayList<Book>();
bookList.add(new Book("Java Tutorial", 300f));
bookList.add(new Book("C# Tutorial", 200f));
// Append all elements to the end of list.
bookList.addAll(bookList1);
// Using Stream
bookList.stream() //
.filter(b -> b.getPrice() > 100) // Filter Books with price > 100
.forEach(b -> System.out.println(b.getTitle() +" / " + b.getPrice()));
}
}
Output:
Java Tutorial / 300.0
C# Tutorial / 200.0
HTML Tutorial / 120.0
5. subList(int,int)
Phương thức subList(int,int) trả về một chế độ xem một phần của đối tượng List hiện tại, bao gồm các phần tử từ chỉ số fromIndex đến toIndex.
Đối tượng "subList" và đối tượng List hiện tại có liên thông với nhau, chẳng hạn nếu bạn xoá một phần tử trên "subList" thì phần tử đó cũng bị xoá khỏi đối tượng List hiện tại.
List<E> subList(int fromIndex, int toIndex);
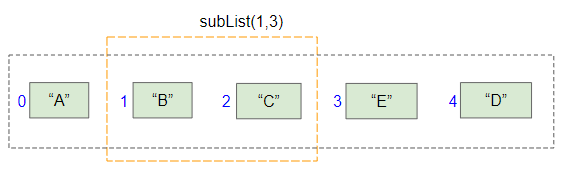
List_subList.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class List_subList {
public static void main(String[] args) {
// Create a List
List<String> originList = new ArrayList<String>();
originList.add("A"); // 0
originList.add("B"); // 1
originList.add("C"); // 2
originList.add("D"); // 3
originList.add("E"); // 4
List<String> subList = originList.subList(1, 3); // fromIndex .. toIndex
System.out.println("Elements in subList: ");
for(String s: subList) {
System.out.println(s); // B C
}
subList.clear(); // Remove all elements from subList.
System.out.println("Elements in original List after removing all elements from subList: ");
for(String s: originList) {
System.out.println(s); // B C
}
}
}
Output:
Elements in subList:
B
C
Elements in original List after removing all elements from subList:
A
D
E
6. spliterator()
Tạo một đối tượng Spliterator cho việc duyệt và phân vùng (traversing and partitioning) các phần tử của List.
default Spliterator<E> spliterator() // Java 8
Spliterator được sử dụng rộng rãi cho việc duyệt và phân vùng (traversing and partitioning) nhiều nguồn dữ liệu khác nhau như Collection (List, Set, Queue), BaseStream, array.
- Hướng dẫn và ví dụ Java Spliterator
7. toArray(..)
Phương thức toArray(..) trả về một mảng chứa tất cả các phần tử của List.
Object[] toArray();
<T> T[] toArray(T[] a);
// Java 11, The default method, inherited from Collection.
default <T> T[] toArray(IntFunction<T[]> generator)
Ví dụ:
List_toArray.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class List_toArray {
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("A"); // 0
list.add("B"); // 1
list.add("C"); // 2
list.add("D"); // 3
list.add("E"); // 4
String[] array = new String[list.size()];
list.toArray(array);
for(String s: array) {
System.out.println(s);
}
}
}
Output:
A
B
C
D
E
8. sort(Comparator)
Phương thức mặc định sort(Comparator) dựa vào đối tượng Comparator được cung cấp để so sánh các phần tử trong List, và xắp xếp lại thứ tự của chúng.
default void sort(Comparator<? super E> c)
Ví dụ: Một đối tượng List chứa các phần tử kiểu Book, chúng ta sẽ xắp xếp chúng tăng dần theo giá và tiêu đề.
List_sort_ex1.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class List_sort_ex1 {
public static void main(String[] args) {
Book b1 = new Book("Python Tutorial", 100f);
Book b2 = new Book("HTML Tutorial", 120f);
Book b3 = new Book("Java Tutorial", 300f);
Book b4 = new Book("Javafx Tutorial", 100f);
Book b5 = new Book("CSS Tutorial", 300f);
List<Book> bookList = new ArrayList<Book>();
bookList.add(b1);
bookList.add(b2);
bookList.add(b3);
bookList.add(b4);
bookList.add(b5);
// Sort by ascending price.
// And Sort by ascending title.
bookList.sort((book1, book2) -> {
float a = book1.getPrice() - book2.getPrice();
if(a > 0) {
return 1;
} else if(a < 0) {
return -1;
}
int b = book1.getTitle().compareTo(book2.getTitle());
return b;
});
for(Book b: bookList) {
System.out.println(b.getPrice() +" : " + b.getTitle());
}
}
}
Output:
100.0 : Javafx Tutorial
100.0 : Python Tutorial
120.0 : HTML Tutorial
300.0 : CSS Tutorial
300.0 : Java Tutorial
Ví dụ trên sử dụng một số khái niệm mới được giới thiệu từ Java 8 như Functional interface, biểu thức Lambda, bạn có thể đọc thêm các bài viết dưới đây để nhận được giải thích chi tiết hơn:
- Functional Interface
- Comparator
9. replaceAll(UnaryOperator)
Phương thức replaceAll(UnaryOperator) sử dụng tham số UnaryOperator được cung cấp để thay thế mỗi phần tử trong List bởi một phần tử mới.
default void replaceAll(UnaryOperator<E> operator)
Ví dụ: Một List chứa các phần tử kiểu Book, thay thế mỗi phần tử Book của nó bởi một Book mới với giá tăng 50%.
List_replaceAll.java
package org.o7planning.list.ex;
import java.util.ArrayList;
import java.util.List;
public class List_replaceAll {
public static void main(String[] args) {
Book b1 = new Book("Python Tutorial", 100f);
Book b2 = new Book("HTML Tutorial", 120f);
Book b3 = new Book("Java Tutorial", 300f);
Book b4 = new Book("Javafx Tutorial", 100f);
Book b5 = new Book("CSS Tutorial", 300f);
List<Book> bookList = new ArrayList<Book>();
bookList.add(b1);
bookList.add(b2);
bookList.add(b3);
bookList.add(b4);
bookList.add(b5);
// Replace Book with new Book with the price increased by 50%.
bookList.replaceAll(b -> new Book(b.getTitle(), b.getPrice() * 150f/100));
for(Book b: bookList) {
System.out.println(b.getPrice() + " : " + b.getTitle());
}
}
}
Output:
150.0 : Python Tutorial
180.0 : HTML Tutorial
450.0 : Java Tutorial
150.0 : Javafx Tutorial
450.0 : CSS Tutorial
- Hướng dẫn và ví dụ Java UnaryOperator
Các hướng dẫn Java Collections Framework
- Hướng dẫn và ví dụ Java PriorityBlockingQueue
- Hướng dẫn sử dụng nền tảng tập hợp trong Java (Java Collection Framework)
- Hướng dẫn và ví dụ Java SortedSet
- Hướng dẫn và ví dụ Java List
- Hướng dẫn và ví dụ Java Iterator
- Hướng dẫn và ví dụ Java NavigableSet
- Hướng dẫn và ví dụ Java ListIterator
- Hướng dẫn và ví dụ Java ArrayList
- Hướng dẫn và ví dụ Java CopyOnWriteArrayList
- Hướng dẫn và ví dụ Java LinkedList
- Hướng dẫn và ví dụ Java Set
- Hướng dẫn và ví dụ Java TreeSet
- Hướng dẫn và ví dụ Java CopyOnWriteArraySet
- Hướng dẫn và ví dụ Java Queue
- Hướng dẫn và ví dụ Java Deque
- Hướng dẫn và ví dụ Java IdentityHashMap
- Hướng dẫn và ví dụ Java WeakHashMap
- Hướng dẫn và ví dụ Java Map
- Hướng dẫn và ví dụ Java SortedMap
- Hướng dẫn và ví dụ Java NavigableMap
- Hướng dẫn và ví dụ Java HashMap
- Hướng dẫn và ví dụ Java TreeMap
- Hướng dẫn và ví dụ Java PriorityQueue
- Hướng dẫn và ví dụ Java BlockingQueue
- Hướng dẫn và ví dụ Java ArrayBlockingQueue
- Hướng dẫn và ví dụ Java TransferQueue
Show More