Bài thực hành Flutter với gói http và dart:convert (2)
Trong bài thực hành Flutter này chúng ta sẽ sử dụng gói thư viện http để lấy dữ liệu từ máy chủ dưới dạng văn bản JSON, sau đó sử dụng gói thư viện dart:convert chuyển đổi nó thành một đối tượng Dart và hiển thị trên Flutter.
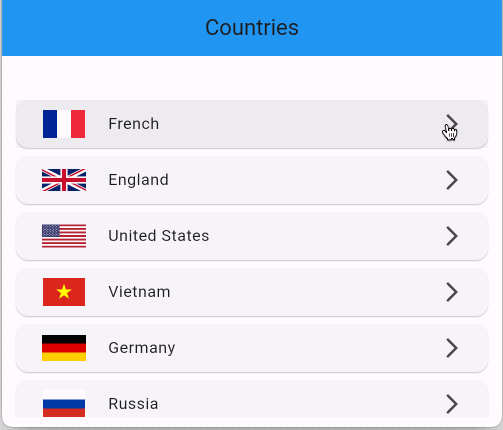
Một URL của máy chủ trả về một văn bản JSON chứa danh sách các quốc gia:
[
{
"countryCode": "fr",
"countryName": "French"
},
{
"countryCode": "gb",
"countryName": "England"
},
...
]
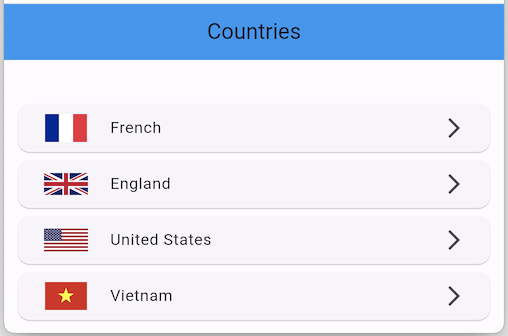
Một URL của máy chủ trả về một văn bản JSON bao gồm thông tin chi tiết của một quốc gia.
{
"countryCode": "vn",
"countryName": "Vietnam",
"nationalDay": "September 2",
"population": 100.00,
"gdp": 408
}
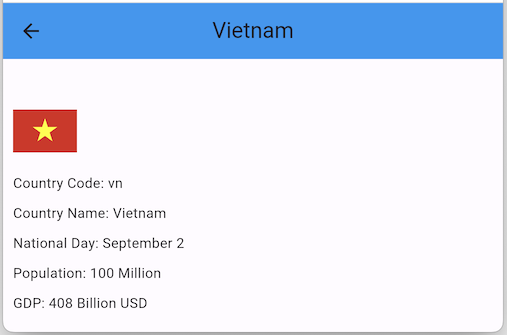
Hình ảnh quốc kỳ cho bởi mã quốc gia:
1. Thư viện
Trong bài học này chúng ta sẽ sử dụng thư viện http để gửi yêu cầu lấy dữ liệu từ máy chủ. Vì vậy bạn cần khai báo thư viện này trong file "pubspec.yaml":
pubspec.yaml
dependencies:
http:
Xem thêm bài viết về gói thư viện http và dart:convert:
2. Data Model
CountryBriefData | Lớp này đại diện cho thông tin ngắn gọn của một quốc gia, nó bao gồm mã quốc gia và tên quốc gia.
|
CountryData | Lớp này đại diện cho một thông tin chi tiết của một quốc gia.
|
_model.dart
import 'dart:convert';
// {
// "countryCode": "vn",
// "countryName": "Vietnam"
// }
class CountryBriefData {
String countryCode;
String countryName;
CountryBriefData(this.countryCode, this.countryName);
// Example: https://flagcdn.com/w320/vn.png
String get imageUrl {
return 'https://flagcdn.com/w320/$countryCode.png';
}
static CountryBriefData fromMap(Map<String, dynamic> map) {
String countryCode = map['countryCode'];
String countryName = map['countryName'];
return CountryBriefData(countryCode, countryName);
}
// https://raw.githubusercontent.com/o7planning/rs/master/rest/countries
static List<CountryBriefData> getListFromJson(String json) {
List<dynamic> list = jsonDecode(json);
return list.map((m) => fromMap(m)).toList();
}
}
// {
// "countryCode": "vn",
// "countryName": "Vietnam",
// "nationalDay": "September 2",
// "population": 100.00,
// "gdp": 408
// }
class CountryData {
String countryCode;
String countryName;
String nationalDay;
double population;
double gdp;
CountryData(
this.countryCode,
this.countryName,
this.nationalDay,
this.population,
this.gdp,
);
CountryData.empty()
: countryCode = '',
countryName = '',
nationalDay = '',
population = 0,
gdp = 0;
// Example: https://flagcdn.com/w320/vn.png
String? get imageUrl {
if (countryCode.isEmpty) {
return null;
}
return 'https://flagcdn.com/w320/$countryCode.png';
}
// https://raw.githubusercontent.com/o7planning/rs/master/rest/country/vn
static CountryData fromMap(Map<String, dynamic> map) {
String countryCode = map['countryCode'];
String countryName = map['countryName'];
String nationalDay = map['nationalDay'];
double population = map['population'];
double gdp = map['gdp'];
return CountryData(countryCode, countryName, nationalDay, population, gdp);
}
static CountryData fromJsonString(String jsonString) {
Map<String, dynamic> map = jsonDecode(jsonString);
return fromMap(map);
}
}
3. Rest Utils
Chúng ta viết một hàm tiện ích để lấy dữ liệu từ một URL và trả về một đối tượng Result<D>.
class Result<D> {
D? data;
String? errorMessage;
Result(this.data, this.errorMessage);
}
_rest_utils.dart
import 'dart:convert';
import 'package:http/http.dart' as http;
/// A Converter to convert responseText to Dart Object.
typedef Converter<D> = D Function(String responseText);
/// responseText = '{"errorMsg": "No Internet"}';
/// Return ==> "No Internet"
typedef ErrorConverter = String Function(String responseText);
/// responseText = '{"errorMsg": "No Internet"}';
/// Return ==> "No Internet"
String exampleErrorConverter(String responseText) {
Map<String, dynamic> map = jsonDecode(responseText);
return map['errorMsg'] ?? responseText;
}
class Result<D> {
D? data;
String? errorMessage;
Result(this.data, this.errorMessage);
}
Future<Result<D>> fetchData<D>({
required String urlString,
Map<String, String>? headers,
required Converter<D> converter,
ErrorConverter? errorConverter,
}) async {
Uri url = Uri.parse(urlString); // Uri object.
D? data; // Dart Object.
String? errorMessage;
try {
final response = await http.get(url, headers: headers);
String responseText = response.body;
if (response.statusCode == 200) {
data = converter(responseText);
} else {
if (errorConverter != null) {
errorMessage = errorConverter!(responseText);
} else {
errorMessage = responseText;
}
}
} catch (e) {
errorMessage = e.toString();
}
return Result(data, errorMessage);
}
Các hàm tiện ích để lấy dữ liệu các quốc gia từ máy chủ:
country_rest_utils.dart
import '_model.dart';
import '_rest_utils.dart';
Future<Result<List<CountryBriefData>>> fetchCountries() {
return fetchData(
urlString:
'https://raw.githubusercontent.com/o7planning/rs/master/rest/countries',
converter: CountryBriefData.getListFromJson,
);
}
Future<Result<CountryData>> fetchCountry(String countryCode) {
return fetchData(
urlString:
'https://raw.githubusercontent.com/o7planning/rs/master/rest/country/$countryCode',
converter: CountryData.fromJsonString,
);
}
4. CountriesScreen
Khi người dùng nhấn vào một quốc gia trên danh sách, ứng dụng sẽ chuyển tới CountryScreen cùng với tham số "countryCode":
countries_screen.dart. (**)
onTap: () {
Navigator.of(context)
.pushNamed("/country", arguments: country.countryCode);
}
main.dart (**)
routes: {
'/countries': (context) => CountriesScreen(),
'/country': (context) {
String? countryCode =
ModalRoute.of(context)?.settings.arguments as String?;
return CountryScreen(countryCode);
},
},
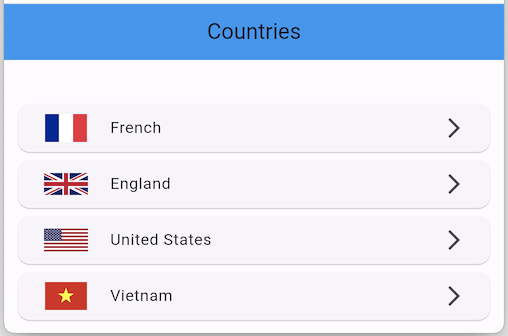
countries_screen.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import '_model.dart';
import '_rest_utils.dart';
import 'country_rest_utils.dart';
class CountriesScreen extends StatefulWidget {
@override
State<StatefulWidget> createState() {
return _CountriesScreenState();
}
}
class _CountriesScreenState extends State<CountriesScreen> {
List<CountryBriefData>? _countries;
String? _errorMessage;
@override
void initState() {
super.initState();
//
loadCountries();
}
Future<void> loadCountries() async {
Result<List<CountryBriefData>> result = await fetchCountries();
if (result.errorMessage != null) {
_errorMessage = result.errorMessage;
} else {
_countries = result.data;
}
setState(() {});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Countries'),
backgroundColor: Colors.blue,
),
body: Padding(
padding: const EdgeInsets.all(10),
child: Column(
children: [
const SizedBox(height: 30),
if (_errorMessage != null) _buildErrorMessage(),
if (_errorMessage != null) const SizedBox(height: 20),
Expanded(
child: _buildListView(),
),
],
),
),
);
}
Widget _buildErrorMessage() {
return Text(
'Error: $_errorMessage',
style: TextStyle(color: Colors.red),
);
}
Widget _buildListView() {
var countries = _countries ?? [];
List<Widget> children = countries
.map(
(country) => CountryBriefWidget(country),
)
.toList();
//
return ListView(
shrinkWrap: true,
children: children,
);
}
}
class CountryBriefWidget extends StatelessWidget {
final CountryBriefData country;
const CountryBriefWidget(this.country, {super.key});
@override
Widget build(BuildContext context) {
return Card(
color: Colors.white,
child: ListTile(
title: Text(country.countryName),
leading: SizedBox(
width: 64,
height: 64,
child: Padding(
padding: const EdgeInsets.all(10),
child: Image.network(country.imageUrl),
),
),
trailing: const Icon(Icons.arrow_forward_ios),
onTap: () {
Navigator.of(context)
.pushNamed("/country", arguments: country.countryCode);
},
),
);
}
}
5. CountryScreen
Màn hình hiển thị thông tin chi tiết của một quốc gia:
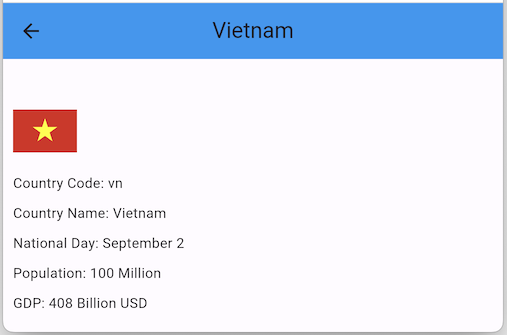
country_screen.dart
import 'package:flutter/material.dart';
import '_model.dart';
import '_rest_utils.dart';
import 'country_rest_utils.dart';
class CountryScreen extends StatefulWidget {
final String? countryCode;
const CountryScreen(this.countryCode, {super.key});
@override
State<StatefulWidget> createState() {
return _CountryScreenState();
}
}
class _CountryScreenState extends State<CountryScreen> {
CountryData _country = CountryData.empty();
String? _errorMessage;
@override
void initState() {
super.initState();
//
loadCountry();
}
Future<void> loadCountry() async {
if (widget.countryCode == null) {
_errorMessage = 'No Country Code';
} else {
Result<CountryData> result = await fetchCountry(widget.countryCode!);
if (result.errorMessage != null) {
_errorMessage = result.errorMessage;
} else {
_country = result.data!;
}
}
setState(() {});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(
_country.countryName.isEmpty ? 'Country' : _country.countryName,
),
backgroundColor: Colors.blue,
),
body: Padding(
padding: const EdgeInsets.all(10),
child: Column(
children: [
const SizedBox(height: 30),
if (_errorMessage != null) _buildErrorMessage(),
if (_errorMessage != null) const SizedBox(height: 20),
_buildCountry(),
],
),
),
);
}
Widget _buildErrorMessage() {
return Text(
'Error: $_errorMessage',
style: const TextStyle(color: Colors.red),
);
}
Widget _buildCountry() {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
SizedBox(
height: 64,
width: 64,
child: _country.imageUrl == null
? const SizedBox()
: Image.network(_country.imageUrl!),
),
const SizedBox(height: 10),
Text('Country Code: ${_country.countryCode}'),
const SizedBox(height: 10),
Text('Country Name: ${_country.countryName}'),
const SizedBox(height: 10),
Text('National Day: ${_country.nationalDay}'),
const SizedBox(height: 10),
Text('Population: ${_country.population} Million'),
const SizedBox(height: 10),
Text('GDP: ${_country.gdp} Billion USD'),
],
);
}
}
6. MainApp
main.dart
import 'package:flutter/material.dart';
import 'countries_screen.dart';
import 'country_screen.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
initialRoute: "/countries",
routes: {
'/countries': (context) => CountriesScreen(),
'/country': (context) {
String? countryCode =
ModalRoute.of(context)?.settings.arguments as String?;
return CountryScreen(countryCode);
},
},
);
}
}
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More