Ví dụ Thymeleaf Form Select option
1. Ví dụ 1 (Form Select + Java List)
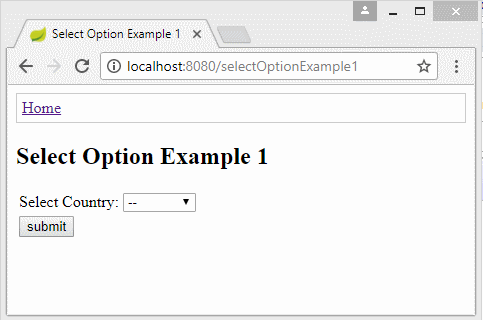
** Spring Controller **
@RequestMapping(value = { "/selectOptionExample1" }, method = RequestMethod.GET)
public String selectOptionExample1Page(Model model) {
PersonForm form = new PersonForm();
model.addAttribute("personForm", form);
List<Country> list = countryDAO.getCountries();
model.addAttribute("countries", list);
return "selectOptionExample1";
}
selectOptionExample1.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Select Option Example 1</title>
</head>
<body>
<!-- Include _menu.html -->
<th:block th:include="/_menu"></th:block>
<h2>Select Option Example 1</h2>
<form name='f' th:object="${personForm}" method='POST'>
<table>
<tr>
<td>Select Country:</td>
<td>
<select th:field="*{countryId}">
<option value=""> -- </option>
<option th:each="country : ${countries}"
th:value="${country.countryId}"
th:utext="${country.countryName}"/>
</select>
</td>
</tr>
<tr>
<td><input name="submit" type="submit" value="submit" /></td>
</tr>
</table>
</form>
</body>
</html>
2. Ví du 2 (Form Select + Java Map)
** Spring Controller **
@RequestMapping(value = { "/selectOptionExample2" }, method = RequestMethod.GET)
public String selectOptionExample2Page(Model model) {
PersonForm form = new PersonForm();
model.addAttribute("personForm", form);
// Long: countryId
// String: countryName
Map<Long, String> mapCountries = countryDAO.getMapCountries();
model.addAttribute("mapCountries", mapCountries);
return "selectOptionExample2";
}
selectOptionExample2.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Select Option Example 2</title>
</head>
<body>
<!-- Include _menu.html -->
<th:block th:include="/_menu"></th:block>
<h2>Select Option Example 2</h2>
<form name='f' th:object="${personForm}" method='POST'>
<table>
<tr>
<td>Select Country:</td>
<td>
<select th:field="*{countryId}">
<option value=""> -- </option>
<option th:each="entry : ${mapCountries.entrySet()}"
th:value="${entry.key}"
th:utext="${entry.value}">
</option>
</select>
</td>
</tr>
<tr>
<td><input name="submit" type="submit" value="submit" /></td>
</tr>
</table>
</form>
</body>
</html>
3. Ví dụ 3 (Form Select + Java Map)
** Spring Controller **
@RequestMapping(value = { "/selectOptionExample3" }, method = RequestMethod.GET)
public String selectOptionExample3Page(Model model) {
PersonForm form = new PersonForm();
model.addAttribute("personForm", form);
// Long: countryId
// String: countryName
Map<Long, String> mapCountries = countryDAO.getMapCountries();
model.addAttribute("mapCountries", mapCountries);
return "selectOptionExample3";
}
selectOptionExample3.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Select Option Example 3</title>
</head>
<body>
<!-- Include _menu.html -->
<th:block th:include="/_menu"></th:block>
<h2>Select Option Example 3</h2>
<form name='f' th:object="${personForm}" method='POST'>
<table>
<tr>
<td>Select Country:</td>
<td>
<select th:field="*{countryId}">
<option value=""> -- </option>
<option th:each="key : ${mapCountries.keySet()}"
th:value="${key}"
th:utext="${mapCountries.get(key)}">
</option>
</select>
</td>
</tr>
<tr>
<td><input name="submit" type="submit" value="submit" /></td>
</tr>
</table>
</form>
</body>
</html>
4. Java Classes
Country.java
package org.o7planning.sbthymeleaf.model;
public class Country {
private Long countryId;
private String countryCode;
private String countryName;
public Country() {
}
public Country(Long countryId, String countryCode, String countryName) {
super();
this.countryId = countryId;
this.countryCode = countryCode;
this.countryName = countryName;
}
public Long getCountryId() {
return countryId;
}
public void setCountryId(Long countryId) {
this.countryId = countryId;
}
public String getCountryCode() {
return countryCode;
}
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
public String getCountryName() {
return countryName;
}
public void setCountryName(String countryName) {
this.countryName = countryName;
}
}
PersonForm.java
package org.o7planning.sbthymeleaf.form;
public class PersonForm {
private String fullName;
private Long countryId;
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public Long getCountryId() {
return countryId;
}
public void setCountryId(Long countryId) {
this.countryId = countryId;
}
}
CountryDAO.java
package org.o7planning.sbthymeleaf.dao;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.o7planning.sbthymeleaf.model.Country;
import org.springframework.stereotype.Repository;
@Repository
public class CountryDAO {
private static final List<Country> COUNTRIES = new ArrayList<Country>();
static {
initData();
}
private static void initData() {
Country vn = new Country(1L, "VN", "Vietnam");
Country en = new Country(2L, "EN", "England");
Country ru = new Country(3L, "VN", "Russia");
COUNTRIES.add(en);
COUNTRIES.add(vn);
COUNTRIES.add(ru);
}
public List<Country> getCountries() {
return COUNTRIES;
}
public Map<Long, String> getMapCountries() {
Map<Long, String> map = new HashMap<Long, String>();
for (Country c : COUNTRIES) {
map.put(c.getCountryId(), c.getCountryName());
}
return map;
}
}
Các hướng dẫn Thymeleaf
- Toán tử Elvis trong Thymeleaf
- Vòng lặp trong Thymeleaf
- Câu lệnh điều kiện if, unless, switch trong Thymeleaf
- Các đối tượng định nghĩa sẵn trong Thymeleaf
- Sử dụng Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Giới thiệu về Thymeleaf
- Biến (Variable) trong Thymeleaf
- Sử dụng Fragment trong Thymeleaf
- Sử dụng Layout trong Thymeleaf
- Sử dụng Thymeleaf th:object và cú pháp asterisk *{ }
- Ví dụ Thymeleaf Form Select option
Show More