Hướng dẫn và ví dụ Flutter multi_dropdown
Flutter multi_dropdown là một thư viện cung cấp widget MultiDropdown cho phép người dùng lựa chọn nhiều tuỳ chọn từ một danh sách thả xuống.
2. Ví dụ cơ bản
Chúng ta hãy bắt đầu với một ví dụ cơ bản:
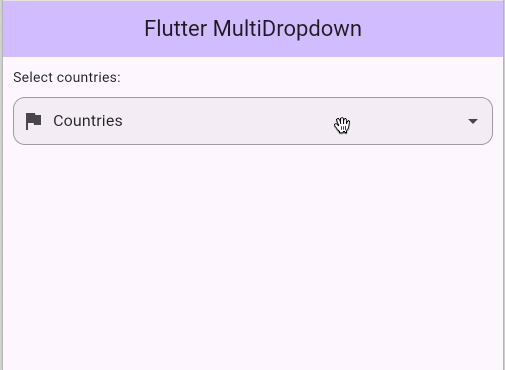
main_ex1.dart
import 'package:flutter/material.dart';
import 'package:multi_dropdown/multi_dropdown.dart';
import 'model.dart';
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key});
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final controller = MultiSelectController<Country>();
@override
Widget build(BuildContext context) {
var items = countryList
.map(
(country) => DropdownItem(
label: country.name,
value: country,
disabled: country.code == "cn",
selected: false,
),
)
.toList();
//
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text("Flutter MultiDropdown"),
),
body: Padding(
padding: const EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text("Select countries:"),
const SizedBox(height: 10),
MultiDropdown<Country>(
items: items,
controller: controller,
enabled: true,
searchEnabled: true,
maxSelections: 3,
chipDecoration: ChipDecoration(
backgroundColor: Colors.indigo.withAlpha(30),
wrap: true,
runSpacing: 2,
spacing: 10,
),
fieldDecoration: FieldDecoration(
hintText: 'Countries',
hintStyle: const TextStyle(color: Colors.black87),
prefixIcon: const Icon(Icons.flag),
showClearIcon: true,
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(12),
borderSide: const BorderSide(color: Colors.grey),
),
focusedBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(12),
borderSide: const BorderSide(
color: Colors.black87,
),
),
),
dropdownDecoration: const DropdownDecoration(
marginTop: 2,
maxHeight: 300,
header: Padding(
padding: EdgeInsets.all(8),
child: Text(
'Select countries from the list',
textAlign: TextAlign.start,
style: TextStyle(
fontSize: 14,
fontWeight: FontWeight.bold,
),
),
),
),
dropdownItemDecoration: DropdownItemDecoration(
selectedIcon: const Icon(Icons.check_box, color: Colors.green),
disabledIcon: Icon(Icons.lock, color: Colors.grey.shade600),
),
validator: (value) {
if (value == null || value.isEmpty) {
return 'Please select a country';
}
return null;
},
onSelectionChange: (selectedItems) {
debugPrint("OnSelectionChange: $selectedItems");
},
),
],
),
),
);
}
}
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
home: MyHomePage(),
);
}
}
Mô hình dữ liệu được sử dụng trong các ví dụ:
model.dart
class Country {
final String name;
final String code;
Country({required this.name, required this.code});
@override
String toString() {
return 'Country(name: $name, code: $code)';
}
}
final List<Country> countryList = [
Country(name: 'Nepal', code: "np"),
Country(name: 'India', code: "in"),
Country(name: 'China', code: "cn"),
Country(name: 'USA', code: "us"),
Country(name: 'UK', code: "gb"),
Country(name: 'Australia', code: "au"),
Country(name: 'Germany', code: "de"),
Country(name: 'France', code: "fr")
];
3. Tuỳ biến DropdownItem
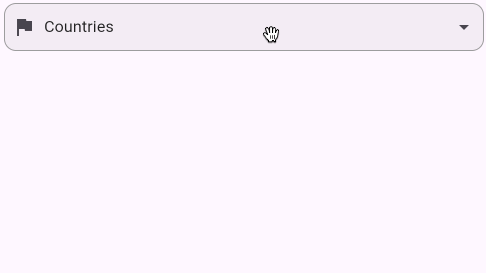
main_custom_item_ex1.dart (*)
MultiDropdown<Country>(
items: items,
controller: controller,
enabled: true,
searchEnabled: true,
maxSelections: 3,
itemBuilder: (
DropdownItem<Country> item,
int index,
VoidCallback onTap,
) {
var imgUrl =
"https://raw.githubusercontent.com/o7planning/rs/master/flutter/flags/${item.value.code}-64.png";
return CheckboxListTile(
secondary: Image.network(imgUrl, width: 24, height: 24),
title: Text(item.label),
enabled: !item.disabled,
value: item.selected,
onChanged: (bool? value) {
onTap();
},
);
},
...
),
- Hướng dẫn và ví dụ Flutter CheckboxListTile
4. MultiSelectController
Sử dụng MultiSelectController cho phép bạn điều khiển trạng thái của MultiDropdown bằng code. Chẳng hạn, lựa chọn hoặc bỏ lựa chọn các Item, vô hiệu hoá các item, ẩn hoặc hiển thị danh sách thả xuống,..
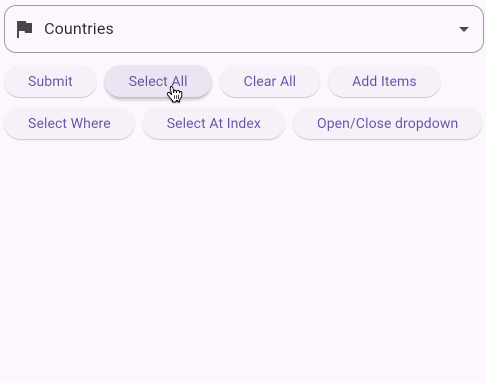
// Validate form
if (_formKey.currentState?.validate() ?? false) {
final selectedItems = controller.selectedItems;
debugPrint(selectedItems.toString());
}
// Select All
controller.selectAll();
// Deselect All
controller.clearAll();
// Add new item
Country vn = Country(name: 'Vietnam', code: "vn");
controller.addItems([
DropdownItem(
label: vn.name,
value: vn,
),
]);
//
controller.selectWhere(
(element) =>
element.value.code == "np"
|| element.value.code == "in"
|| element.value.code == "cn",
);
controller.selectAtIndex(0);
controller.openDropdown();
5. MultiDropdown
MultiDropdown Constructor (*)
MultiDropdown({
required List<DropdownItem<T>> items,
FieldDecoration fieldDecoration = const FieldDecoration(),
ChipDecoration chipDecoration = const ChipDecoration(),
DropdownDecoration dropdownDecoration = const DropdownDecoration(),
SearchFieldDecoration searchDecoration = const SearchFieldDecoration(),
DropdownItemDecoration dropdownItemDecoration = const DropdownItemDecoration(),
Widget Function(DropdownItem<T>, int, void Function())? itemBuilder,
Widget Function(DropdownItem<T>)? selectedItemBuilder,
AutovalidateMode autovalidateMode = AutovalidateMode.disabled,
bool singleSelect = false,
Widget? itemSeparator,
MultiSelectController<T>? controller,
String? Function(List<DropdownItem<T>>?)? validator,
bool enabled = true,
bool searchEnabled = false,
int maxSelections = 0,
FocusNode? focusNode,
void Function(List<T>)? onSelectionChange,
bool closeOnBackButton = false,
Key? key,
});
Hình ảnh dưới đây minh hoạ các thuật ngữ liên quan tới MultiDropdown.
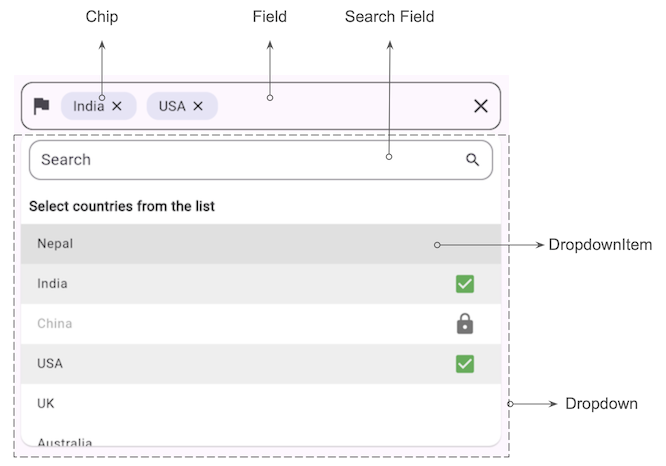
6. FieldDecoration
FieldDecoration Constructor (*)
FieldDecoration({
String? labelText,
String? hintText = 'Select',
InputBorder? border,
InputBorder? focusedBorder,
InputBorder? disabledBorder,
InputBorder? errorBorder,
Widget? suffixIcon = const Icon(Icons.arrow_drop_down),
Widget? prefixIcon,
TextStyle? labelStyle,
double borderRadius = 12,
TextStyle? hintStyle,
bool animateSuffixIcon = true,
EdgeInsets? padding =
const EdgeInsets.symmetric(horizontal: 12, vertical: 8),
Color? backgroundColor,
bool showClearIcon = true,
});
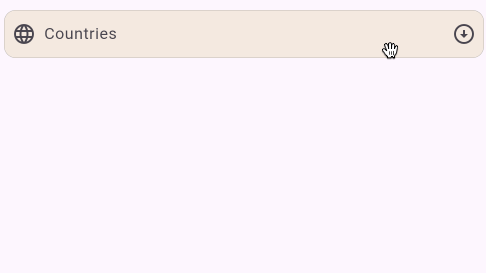
field_decoration_ex1.dart (*)
FieldDecoration(
hintText: 'Countries',
labelText: 'Countries',
hintStyle: const TextStyle(color: Colors.black87),
prefixIcon: const Icon(Icons.language),
suffixIcon: const Icon(Icons.arrow_circle_down_outlined),
showClearIcon: true,
backgroundColor: Colors.amberAccent.withAlpha(30),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(12),
borderSide: const BorderSide(
color: Colors.grey,
width: 0.3,
),
),
focusedBorder: OutlineInputBorder(
borderRadius: BorderRadius.circular(12),
borderSide: const BorderSide(
color: Colors.red,
width: 0.5,
),
),
),
7. DropdownDecoration
DropdownDecoration Constructor (*)
DropdownDecoration({
Color backgroundColor = Colors.white,
double elevation = 1,
double maxHeight = 400,
double marginTop = 0,
BorderRadius borderRadius = const BorderRadius.all(Radius.circular(12)),
Widget? footer,
Widget? header,
});
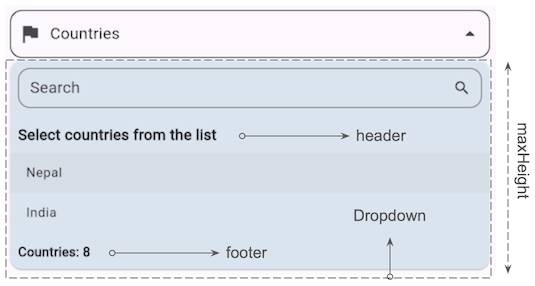
DropdownDecoration(
marginTop: 2,
maxHeight: 300,
backgroundColor: Colors.blue.withAlpha(80),
header: const Padding(
padding: EdgeInsets.all(8),
child: Text(
'Select countries from the list',
textAlign: TextAlign.start,
style: TextStyle(
fontSize: 15,
fontWeight: FontWeight.bold,
),
),
),
footer: Padding(
padding: const EdgeInsets.all(8),
child: Text(
'Countries: ${controller.items.length}',
textAlign: TextAlign.start,
style: const TextStyle(
fontSize: 13,
fontWeight: FontWeight.bold,
),
),
),
),
8. SearchFieldDecoration
SearchFieldDecoration Constructor (*)
SearchFieldDecoration({
String hintText = 'Search',
InputBorder? border = const OutlineInputBorder(
borderSide: BorderSide(color: Color(0xFFE0E0E0)),
borderRadius: BorderRadius.all(Radius.circular(12)),
),
InputBorder? focusedBorder = const OutlineInputBorder(
borderSide: BorderSide(color: Colors.grey),
borderRadius: BorderRadius.all(Radius.circular(12)),
),
Icon searchIcon = const Icon(Icons.search),
});
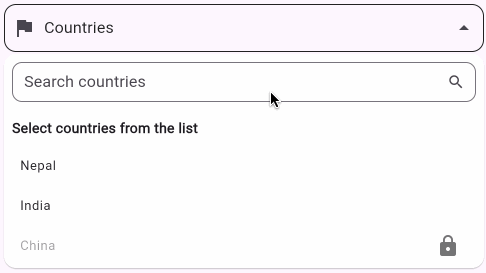
SearchFieldDecoration(
hintText: "Search countries",
border: OutlineInputBorder(
gapPadding: 10,
borderRadius: const BorderRadius.all(Radius.circular(10.0)),
borderSide:
const BorderSide(color: Colors.indigo, width: 0.3),
),
focusedBorder: OutlineInputBorder(
gapPadding: 10,
borderRadius: const BorderRadius.all(Radius.circular(10.0)),
borderSide: const BorderSide(
color: Colors.red,
width: 0.5,
),
),
),
9. DropdownItemDecoration
DropdownItemDecoration Constructor (*)
DropdownItemDecoration({
Color? backgroundColor,
Color? disabledBackgroundColor,
Color? selectedBackgroundColor,
Color? selectedTextColor,
Color? textColor,
Color? disabledTextColor,
Icon? selectedIcon = const Icon(Icons.check),
Icon? disabledIcon,
});
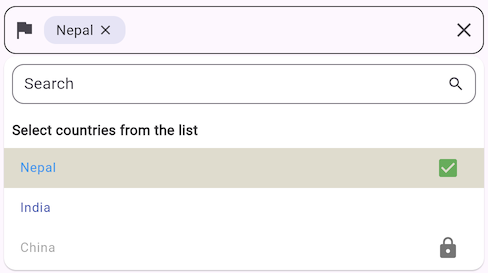
dropdownItemDecoration: DropdownItemDecoration(
selectedIcon: const Icon(Icons.check_box, color: Colors.green),
disabledIcon: Icon(Icons.lock, color: Colors.grey.shade600),
selectedTextColor: Colors.blue,
selectedBackgroundColor: Colors.amberAccent.withAlpha(30),
textColor: Colors.indigo,
),
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More