Câu lệnh điều kiện if, unless, switch trong Thymeleaf
1. th:if, th:unless
Trong một vài tình huống bạn muốn một đoạn nào đó của Thymeleaf Template xuất hiện trong kết quả nếu một điều kiện nhất định nào đó được đánh giá là true (Đúng). Để làm được điều này bạn có thể sử dụng thuộc tính (attribute) th:if.
Chú ý: Trong Thymeleaf, một biến hoặc một biểu thức nào đó được đánh giá là false (Sai) nếu giá trị của nó là null, false, 0, "false", "off", "no". Các trường hợp khác được đánh giá là true (Đúng).
Cú pháp:
<someHtmlTag th:if="condition">
<!-- Other code -->
</someHtmlTag>
<!-- OR: -->
<th:block th:if="condition">
<!-- Other code -->
</th:block>
Một thuộc tính (attribute) khác bạn cũng có thể sử dụng đó là th:unless, nó là phủ nhận của th:if.
<someTag th:unless = "condition"> ... </someTag>
<!-- Same as -->
<someTag th:if = "!condition"> ... </someTag>
Example th:if
Ví dụ với th:if:
if-example1.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>If/Unless</title>
<style>
.product-container {
padding: 5px;
border: 1px solid #ccc;
margin-top: 10px;
height: 80px;
}
.img-container {
float: left;
margin-right: 5px;
}
</style>
</head>
<body>
<h1>th:if</h1>
<div class="product-container" th:each="product : ${products}">
<!--/* If the product has image, this code will be rendered. */-->
<div class="img-container" th:if="${product.image}">
<img th:src="@{|/${product.image}|}" height="70" />
</div>
<div>
<b>Code:</b> <span th:utext="${product.code}"></span>
</div>
<div>
<b>Name:</b> <span th:utext="${product.name}"></span>
</div>
</div>
</body>
</html>
(Java Spring)
@RequestMapping("/if-example1")
public String ifExample1(Model model) {
Product prod1 = new Product(1L, "SS-S9", "Sam Sung Galaxy S9", "samsung-s9.png");
Product prod2 = new Product(2L, "NK-5P", "Nokia 5.1 Plus", null);
Product prod3 = new Product(3L, "IP-7", "iPhone 7", "iphone-7.jpg");
List<Product> list = new ArrayList<Product>();
list.add(prod1);
list.add(prod2);
list.add(prod3);
model.addAttribute("products", list);
return "if-example1";
}
Product.java
package org.o7planning.thymeleaf.model;
public class Product {
private Long id;
private String code;
private String name;
private String image;
public Product() {
}
public Product(Long id, String code, String name, String image) {
this.id = id;
this.code = code;
this.name = name;
this.image = image;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
}
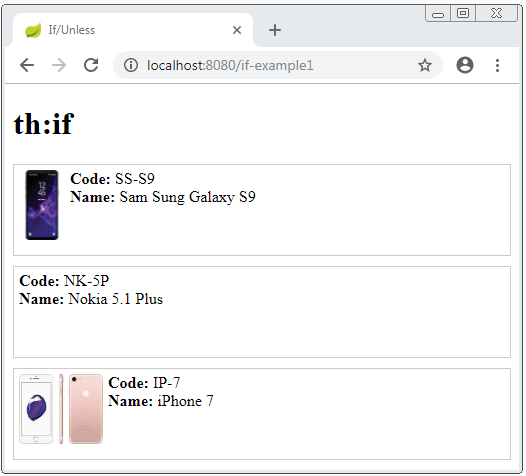
Example th:if, th:unless
Ví dụ với th:if và th:unless:
if-unless-example1.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>If/Unless</title>
<style>
.product-container {
padding: 5px;
border: 1px solid #ccc;
margin-top: 10px;
height: 80px;
}
.img-container {
float: left;
margin-right: 5px;
}
</style>
</head>
<body>
<h1>th:if, th:unless</h1>
<div class="product-container" th:each="product : ${products}">
<!--/* If the product has image, this code will be rendered. */-->
<div class="img-container" th:if="${product.image}">
<img th:src="@{|/${product.image}|}" height="70" />
</div>
<!--/* If the product has no image, display default Image. */-->
<div class="img-container" th:unless="${product.image}">
<img th:src="@{/no-image.png}" height="70" />
</div>
<div>
<b>Code:</b> <span th:utext="${product.code}"></span>
</div>
<div>
<b>Name:</b> <span th:utext="${product.name}"></span>
</div>
</div>
</body>
</html>
(Java Spring)
@RequestMapping("/if-unless-example1")
public String ifUnlessExample1(Model model) {
Product prod1 = new Product(1L, "SS-S9", "Sam Sung Galaxy S9", "samsung-s9.png");
Product prod2 = new Product(2L, "NK-5P", "Nokia 5.1 Plus", null);
Product prod3 = new Product(3L, "IP-7", "iPhone 7", "iphone-7.jpg");
List<Product> list = new ArrayList<Product>();
list.add(prod1);
list.add(prod2);
list.add(prod3);
model.addAttribute("products", list);
return "if-unless-example1";
}
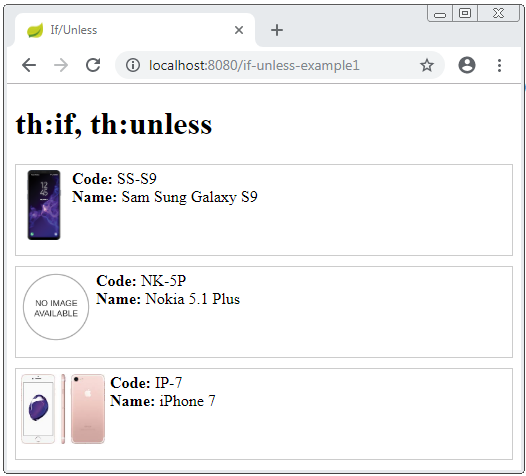
Xem thêm về toán tử Elvis:<!--/* Elvis Operator */--> <p>Age: <span th:utext="${person.age}?: '(no age specified)'">27</span>.</p>
2. th:switch, th:case
Trong Java bạn quen thuộc với cấu trúc switch/case, Thymeleaf cũng có một cấu trúc tương tự đó là th:swith/th:case.
<div th:switch="${user.role}">
<p th:case="'admin'">User is an administrator</p>
<p th:case="${roles.manager}">User is a manager</p>
<p th:case="'staff'">User is a staff</p>
</div>
<!-- th:switch/th:case with default case: -->
<div th:switch="${user.role}">
<p th:case="'admin'">User is an administrator</p>
<p th:case="${roles.manager}">User is a manager</p>
<p th:case="'staff'">User is a staff</p>
<p th:case="*">User is some other thing</p>
</div>
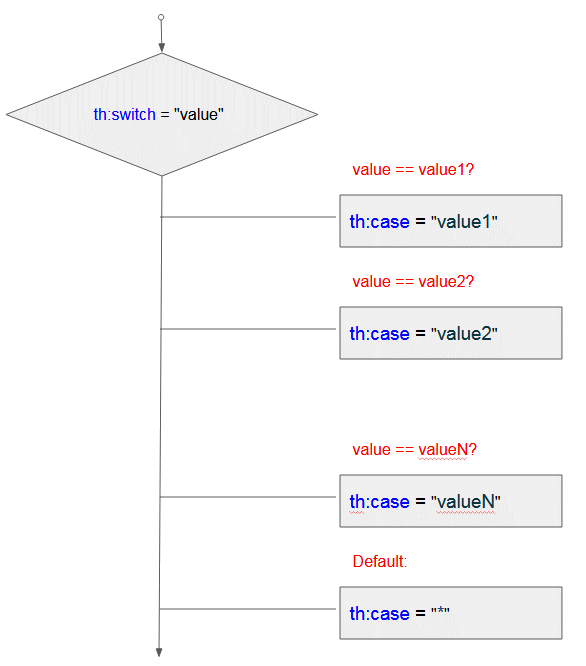
Chương trình sẽ đánh giá lần lượt các case (trường hợp) từ trên xuống dưới, nếu tìm thấy 1 case được đánh giá là true (đúng) nó sẽ "render" mã trong case này, tất các case khác sẽ bị bỏ qua.
th:case = "*" là case mặc định của cấu trúc th:swith/th:case. Nếu tất cả các case ở phía trên bị đánh giá là false thì mã của case mặc định sẽ được "render".
Example:
switch-example.html (Template)
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>th:witch/th:case</title>
</head>
<body>
<h1>tth:witch/th:case</h1>
<h4 th:utext="${user.userName}"></h4>
<div th:switch="${user.role}">
<p th:case="'admin'">User is an administrator</p>
<p th:case="'manager'">User is a manager</p>
<p th:case="'staff'">User is a staff</p>
<p th:case="*">User is some other thing</p>
</div>
</body>
</html>
(Java Spring)
@RequestMapping("/switch-example")
public String ifTestFalse(Model model) {
User user = new User("Administrator", "admin");
model.addAttribute("user", user);
return "switch-example";
}
User.java
package org.o7planning.thymeleaf.model;
public class User {
private String userName;
private String role;
public User(String userName, String role) {
this.userName = userName;
this.role = role;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
}
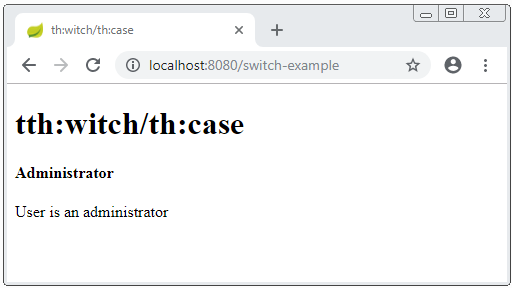
Các hướng dẫn Thymeleaf
- Toán tử Elvis trong Thymeleaf
- Vòng lặp trong Thymeleaf
- Câu lệnh điều kiện if, unless, switch trong Thymeleaf
- Các đối tượng định nghĩa sẵn trong Thymeleaf
- Sử dụng Thymeleaf th:class, th:classappend, th:style, th:styleappend
- Giới thiệu về Thymeleaf
- Biến (Variable) trong Thymeleaf
- Sử dụng Fragment trong Thymeleaf
- Sử dụng Layout trong Thymeleaf
- Sử dụng Thymeleaf th:object và cú pháp asterisk *{ }
- Ví dụ Thymeleaf Form Select option
Show More