Bắt đầu với Google Search Console Java API
API Search Console cung cấp quyền truy cập theo chương trình vào nhiều chức năng của Google Search Console. Sử dụng API để xem, thêm hoặc xóa tài sản (property) và sơ đồ trang web, chạy truy vấn nâng cao về dữ liệu kết quả của Google Search cho các thuộc tính mà bạn quản lý trong Search Console và kiểm tra từng trang riêng lẻ.
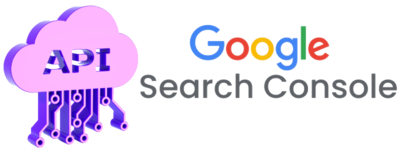
API này được phơi bầy dưới dạng HTTP Rest Service, bạn có thể gọi các chức năng của nó thông qua các thư viện REST.
Trong bài viết này tôi sẽ hướng dẫn bạn thiết lập một dự án Java sử dụng Google Search Console API.
1. Các yêu cầu đòi hỏi
Trước hết bạn cần đăng ký và tạo một dự án trên Google Cloud Console.
Tiếp theo, bật dịch vụ "Google Search Console" cho tài khoản của bạn.
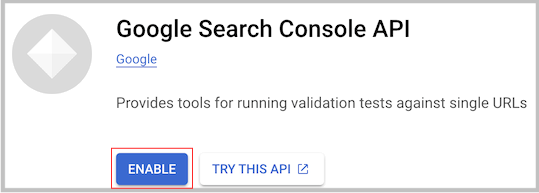
Tạo một "Google Service Account" (Tài khoản dịch vụ Google) và download thông tin xác thực (Credentials) dưới dạng một file JSON.
Sau bước trên, bạn được cung cấp một email có định dạng "xxx@yyy.iam.gserviceaccount.com".
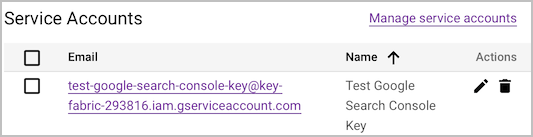
Trên Google Search Console, thêm Google Service Account email mà bạn có ở bước trên như một chủ sở hữu cho website của bạn.
Chọn một property (website), chẳng hạn:
- https://mydomain.com/
Settings > Users and permissions:
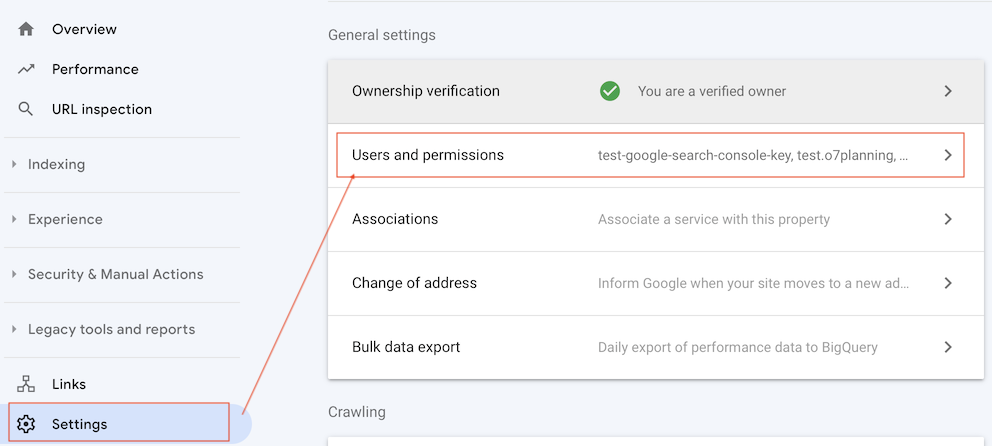
Thêm Google Service Account email mà bạn có ở bước trên như một chủ sở hữu cho property (tài sản) của bạn.
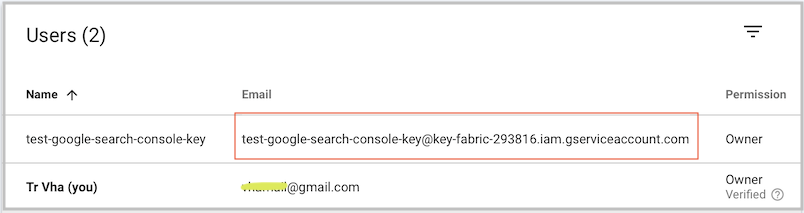
2. Thư viện
Thêm các thư viện Java vào dự án của bạn:
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client</artifactId>
<version>2.2.0</version>
</dependency>
<!-- Github -->
<!-- https://github.com/googleapis/google-api-java-client-services/tree/main/clients/google-api-services-searchconsole/v1 -->
<!-- SEE ALL VERSION -->
<!-- https://libraries.io/maven/com.google.apis:google-api-services-searchconsole/versions -->
<dependency>
<groupId>com.google.apis</groupId>
<artifactId>google-api-services-searchconsole</artifactId>
<version>v1-rev20230920-2.0.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.auth/google-auth-library-oauth2-http -->
<dependency>
<groupId>com.google.auth</groupId>
<artifactId>google-auth-library-oauth2-http</artifactId>
<version>1.20.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client-gson -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client-gson</artifactId>
<version>2.2.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.15.1</version>
</dependency>
3. Các lớp tiện ích
Sau khi download khoá của "Google Service Account" ở bước trên chúng ta có một file JSON. Tiếp theo, chúng ta viết một lớp tiện ích để tạo đối tượng HttpRequestInitializer, đối tượng này cần thiết để khởi tạo các yêu cầu trước khi chúng được gửi tới Google.
MyUtils.java
package org.o7planning.googleapis.utils;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import org.apache.commons.io.FileUtils;
import com.google.api.client.http.HttpRequest;
import com.google.api.client.http.HttpRequestInitializer;
import com.google.api.client.json.JsonObjectParser;
import com.google.api.client.json.gson.GsonFactory;
import com.google.api.client.util.ObjectParser;
import com.google.auth.http.HttpCredentialsAdapter;
import com.google.auth.oauth2.GoogleCredentials;
import com.google.auth.oauth2.ServiceAccountCredentials;
public class MyUtils {
public static final String SERVICE_ACCOUNT_FILE_PATH = "/Volumes/New/_gsc/test-google-search-console-key.json";
private static byte[] serviceAccountBytes;
public static HttpRequestInitializer createHttpRequestInitializer(String... scopes) throws IOException {
InputStream serviceAccountInputStream = getServiceAccountInputStream();
GoogleCredentials credentials = ServiceAccountCredentials //
.fromStream(serviceAccountInputStream) //
.createScoped(scopes);
HttpRequestInitializer requestInitializer = new HttpRequestInitializer() {
@Override
public void initialize(HttpRequest request) throws IOException {
HttpCredentialsAdapter adapter = new HttpCredentialsAdapter(credentials);
adapter.initialize(request);
//
if (request.getParser() == null) {
ObjectParser parser = new JsonObjectParser(GsonFactory.getDefaultInstance());
request.setParser(parser);
}
//
request.setConnectTimeout(60000); // 1 minute connect timeout
request.setReadTimeout(60000); // 1 minute read timeout
}
};
return requestInitializer;
}
public static synchronized InputStream getServiceAccountInputStream() throws IOException {
if (serviceAccountBytes == null) {
serviceAccountBytes = FileUtils.readFileToByteArray(new File(SERVICE_ACCOUNT_FILE_PATH));
}
return new ByteArrayInputStream(serviceAccountBytes);
}
}
- Hướng dẫn sử dụng Google reCAPTCHA trong ứng dụng Web Java
- Thao tác với tập tin và thư mục trên Google Drive sử dụng Java
- Bắt đầu với Google Search Console Java API
- Đánh chỉ mục trang với Java Google Indexing API
- Tạo một Google Service Account
- Liệt kê, thêm và xoá các Sitemap với Google Search Java API
- Liệt kê, thêm và xoá các Sites với Google Search Java API
- Đăng ký Google Map API Key
- Tạo Google API Console Project và OAuth2 Client ID
- Kiếm tiền chuyên nghiệp với Ezoic - đối tác của Google
Show More