Hướng dẫn và ví dụ NodeJS Module
1. NodeJS Module là gì?
Nói một cách đơn giản, NodeJS Module là một thư viện Javascript, nó là một tập hợp các hàm (function) đối tượng và các biến, mà bạn có thể đưa vào ứng dụng của bạn để sử dụng. Sử dụng Module giúp đơn giản việc viết code, và quản lý code trong ứng dụng của bạn. Thông thường mỗi module sẽ được viết trong một tập tin riêng rẽ.
NodeJS đã xây dựng sẵn khá nhiều Module, nó các các thư viện chuẩn để bạn phát triển ứng dụng. Dưới đây là danh sách:
Module | Description |
assert | Cung cấp tập hợp các assertion tests (Kiểm tra khẳng định) |
buffer | Để xử lý các dữ liệu nhị phân (binary data). |
child_process | Để chạy một tiến trình con (child process) |
cluster | Để tách một tiến trình (process) thành nhiều tiến trình. |
crypto | Chứa các hàm mã hóa OpenSSL (OpenSSL cryptographic functions) |
dgram | Cung cấp các thực hiện (implementation) cho UDP sockets |
dns | Cung cấp các chức năng để tìm kiếm (lookups) và phân giải (resolution) DNS. |
events | Để xử lý các sự kiện (events) |
fs | Để xử lý file system |
http | Để làm cho Node.js hoạt động như một HTTP server. |
https | Để làm cho Node.js hoạt động như một HTTPS server. |
net | Để tạo các server và các client. |
os | Cung cấp các thông tin về hệ điều hành. |
path | Để xử lý các đường dẫn file (file paths). |
querystring | Để xử lý URL query strings |
readline | Để xử lý các luồng dữ liệu (data streams) mà có thể đọc từng dòng (line) dữ liệu. |
stream | Xử lý các luồng dữ liệu (streaming data) |
string_decoder | Để giải mã (decode) đối tượng bộ đệm (buffer objects) thành string |
timers | Hẹn giờ để thực thi một hàm Javascript. |
tls | Để thực hiện các giao thức TLS & SSL. |
tty | Cung cấp các lớp được sử dụng bởi text terminal. |
url | Giúp phân tích (parse) các chuỗi URL (URL strings) |
util | Cung cấp các hàm tiện ích (Utility functions). |
v8 | Truy cập vào các thông tin của V8 JavaScript engine. |
vm | Biên dịch (compile) mã JavaScript trong máy ảo (Virtual machine) |
zlib | Xử lý nén và giải nén các tập tin. |
Để sử dụng một module nào đó, sử dụng hàm require(moduleName). Chẳng hạn http là một module nó làm cho NodeJS hoạt động như một HTTP Server.
Đảm bảo rằng bạn đã tạo thành công một dự án NodeJS. Nếu không hãy tham khảo bài học dưới đây:
Trên project NodeJS của bạn, tạo một thư mục module-examples để chứa các tập tin Javascript mà bạn thực hành trong bài học này.
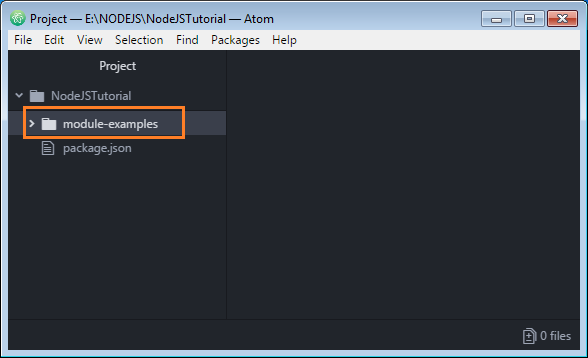
Tạo một tập tin có tên http-example.js trong thư mục module-examples.
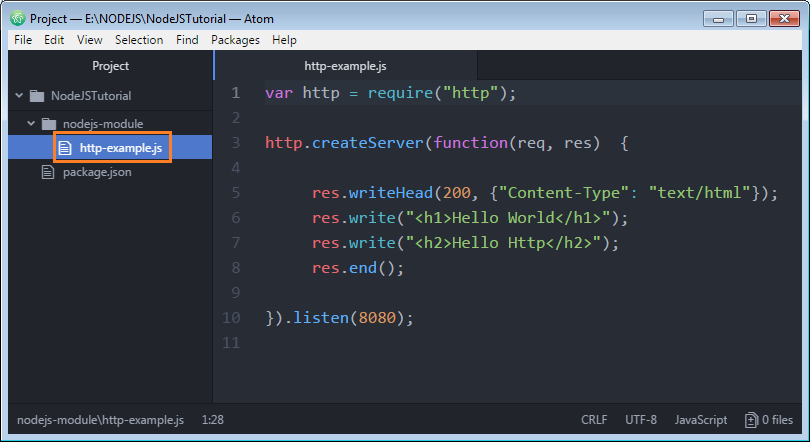
http-example.js
var http = require("http");
http.createServer(function(req, res) {
res.writeHead(200, {"Content-Type": "text/html"});
res.write("<h1>Hello World</h1>");
res.write("<h2>Hello Http</h2>");
res.end();
}).listen(8080);
Mở cửa sổ CMD và CD tới thư mục project của bạn, sử dụng NodeJS để chạy tập tin http-example.js:
node ./module-examples/http-example.js
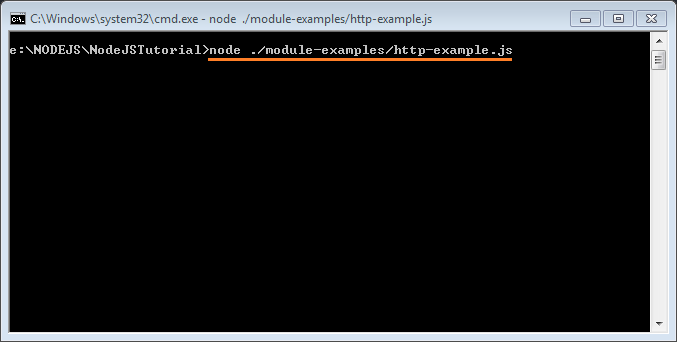
Mở trình duyệt và truy cập vào đường dẫn:
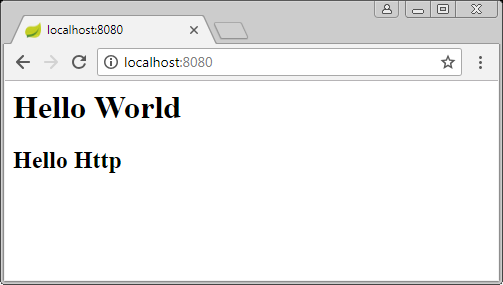
2. Tạo Module của bạn
Trong NodeJS bạn có thể tạo một Module tùy biến. Module là một file Javascript, hãy chú ý tới vị trí đặt file này vì nó ảnh hưởng tới cách để bạn sử dụng nó. Bạn có 2 cách chọn vị trí đặt file này:
- Tập tin Module được đặt trong thư mục có tên node_modules (Thư mục con của project). Đây là vị trí được khuyến khích.
- Tập tin Module được đặt trong một thư mục nào đó của bạn, không phải node_modules.
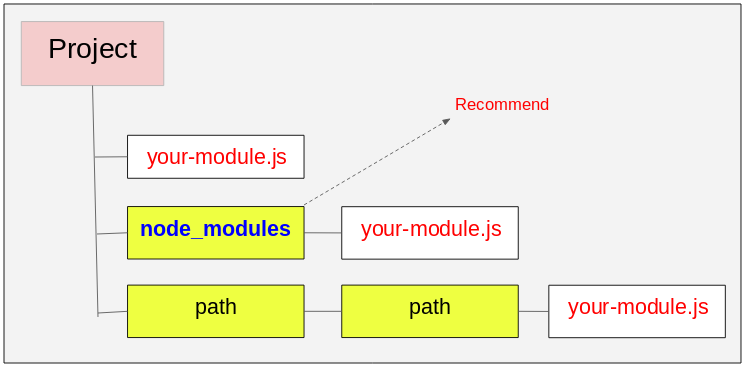
NodeJS khuyên bạn nên đặt các tập tin Module của bạn vào thư mục node_modules của project. Chờ một chút, tôi sẽ giải thích lý do tại sao nên đặt module của bạn trong thư mục này.
Cú pháp để export (Xuất khẩu) cái gì đó trong tập tin Javascript của bạn ra bên ngoài.
exports = something;
// or:
module.exports = something;
my-first-module.js
Trước hết, tạo một tập tin my-first-module.js trong thư mục node_modules, và tập tin test-my-first-module.js trong một thư mục khác.
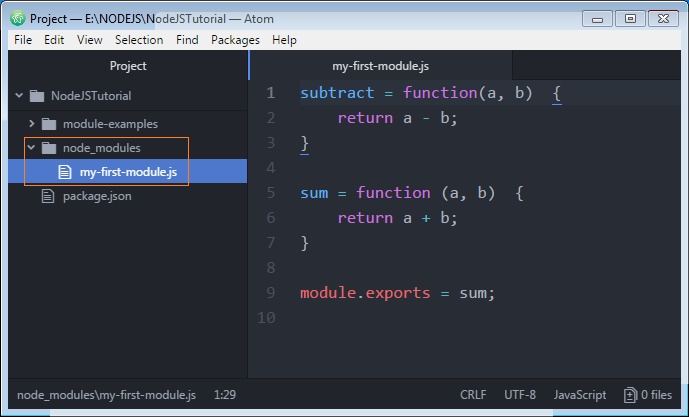
Trong ví dụ này, tập tin my-first-module.js có nhiều hàm (function), nhưng tôi chỉ export (xuất khẩu) hàm "sum".
node_modules/my-first-module.js
subtract = function(a, b) {
return a - b;
}
sum = function (a, b) {
return a + b;
}
module.exports = sum;
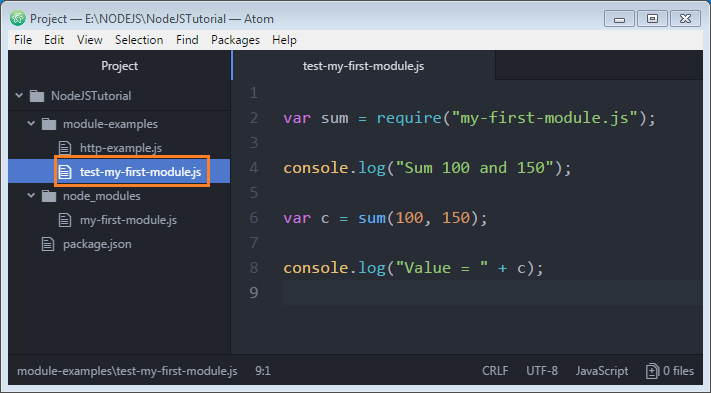
module-examples/test-my-first-module.js
var sum = require("my-first-module.js");
console.log("Sum 100 and 150");
var c = sum(100, 150);
console.log("Value = " + c);
Chạy ví dụ trên:
node ./module-examples/test-my-first-module.js
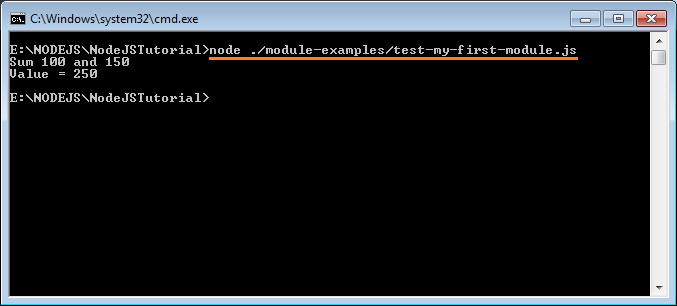
Với các tập tin module được đặt trong thư mục node_modules cách sử dụng nó rất đơn giản. Bạn không cần quan tâm tới đường dẫn tương đối hay tuyệt đối.var myVar = require("module-file-name.js"); // or: var myVar = require("module-file-name");
my-second-module.js
Tiếp theo, chúng ta tạo một tập tin my-second-module.js nằm trong một thư mục nào đó chẳng hạn: node_modules_2.
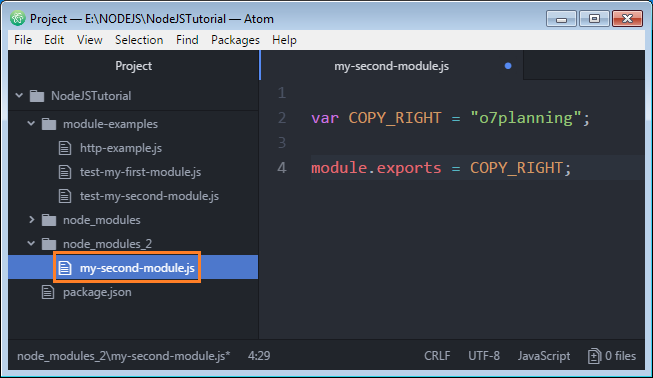
node_modules_2/my-second-module.js
var COPY_RIGHT = "o7planning";
module.exports = COPY_RIGHT;
Để sử dụng các module nằm trong thư mục khác với node_modules bạn phải sử dụng đường dẫn tương đối.
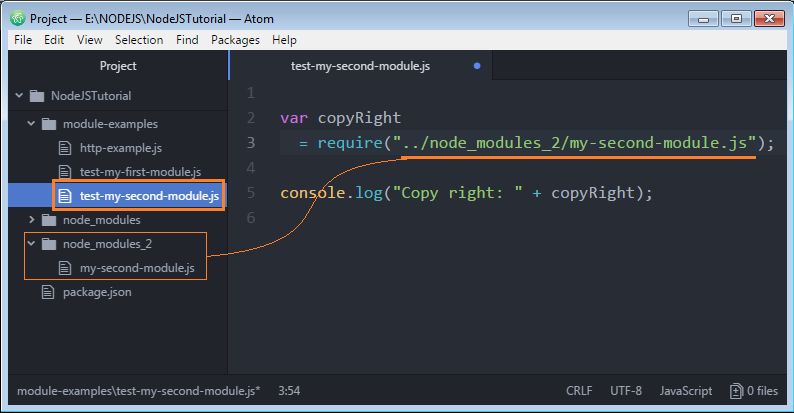
module-examples/test-my-second-module.js
var copyRight
= require("../node_modules_2/my-second-module.js");
console.log("Copy right: " + copyRight);
node ./module-examples/test-my-second-module.js
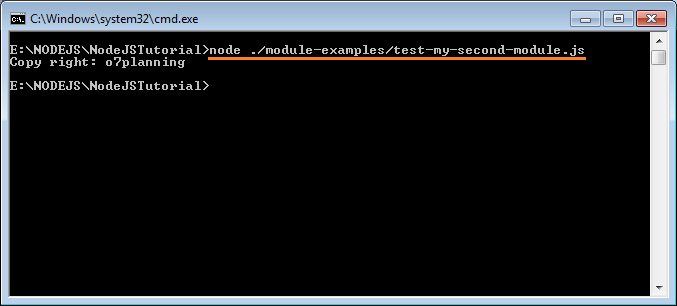
3. Export nhiều thứ
Trong một file module có thể bao gồm nhiều hàm (function), nhiều biến (variable), và nhiều lớp (class). Ví dụ này chỉ cho bạn cách export (xuất khẩu) nhiều thứ trong file này.
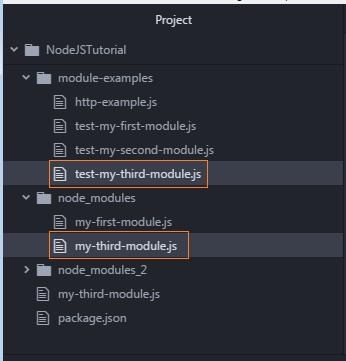
node_modules/my-third-module.js
// A variable!!
var COPY_RIGHT = "o7planning";
// A function!!
var multiple = function(a, b) {
return a * b;
};
// A class!!
var Person = class {
constructor(name, age) {
this.name = name;
this.age = age;
}
showInfo() {
console.log("name="+ this.name+", age="+ this.age);
}
};
// Something to Export!!
var somethingToExport = {
Person: Person,
multiple : multiple,
COPY_RIGHT : COPY_RIGHT
};
// Exprort it!!
module.exports = somethingToExport;
module-examples/test-my-third-module.js
var module3 = require("my-third-module.js");
console.log("Using Person class of module:");
var p = new module3.Person("Smith", 20);
p.showInfo();
console.log(" -- ");
console.log("Using multiple Function of module:");
var c = module3.multiple(20, 5);
console.log("Value= "+ c);
console.log(" -- ");
console.log("Using Variable of module:");
var copyRight = module3.COPY_RIGHT;
console.log("Copy right: " + copyRight);
node ./module-examples/test-my-third-module.js
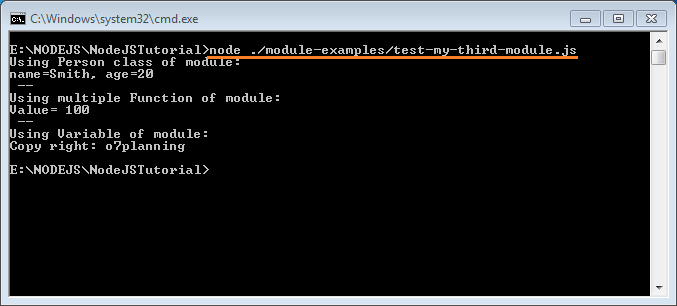
Các hướng dẫn NodeJS
- Giới thiệu về NodeJs
- NPM là gì?
- Hướng dẫn NodeJS cho người mới bắt đầu
- Cài đặt trình soạn thảo Atom
- Cài đặt NodeJS trên Windows
- Hướng dẫn và ví dụ NodeJS Module
- Khái niệm Callback trong NodeJS
- Tạo một HTTP Server đơn giản với NodeJS
- Tìm hiểu về Event Loop trong NodeJS
- Hướng dẫn và ví dụ NodeJS EventEmitter
- Hướng dẫn và ví dụ NodeJS Buffer
- Kết nối cơ sở dữ liệu MySQL trong NodeJS
Show More