Toán tử instanceof trong TypeScript
1. Toán tử instanceof
Toán tử instanceof được sử dụng để kiểm tra một đối tượng nào đó có phải là một đối tượng của một lớp được chỉ định hay không. Toán tử này trả về giá trị true hoặc false.
Cú pháp:
anObject instanceof AClass;
let result = anObject instanceof AClass;
Đặc điểm của toán tử instanceof:
- Phía bên trái của biểu thức instanceof không thể là kiểu dữ liệu nguyên thuỷ, nó phải là một đối tượng.
- Phía bên phải của biểu thức instanceof phải là một lớp.
Chúng ta sẽ làm rõ các đặc điểm nói trên của toán tử instanceof thông qua các ví dụ:
Ví dụ dưới đây cho thấy trình biên dịch sẽ thông báo lỗi nếu phía bên trái của biểu thức instanceof là một giá trị nguyên thuỷ.
- null, string, number, boolean, NaN, undefined, Symbol
instanceof_ex1a.ts
class Abc { }
let aNaN = NaN;
aNaN instanceof Abc; // Compile Error!!!
let aNumber = 123;
aNumber instanceof Abc; // Compile Error!!!
let anUndefined = undefined;
anUndefined instanceof Abc; // Compile Error!!!
let aSymbol = Symbol("Something");
aSymbol instanceof Abc; // Compile Error!!!
let aNull = null;
aNull instanceof Abc; // Compile Error!!!
let aBoolean = true;
aBoolean instanceof Abc; // Compile Error!!!
let aBooleanObject = new Boolean(true); // Boolean Object
aBooleanObject instanceof Abc; // Compile OK!
Ví dụ: Phía bên trái của biểu thức instanceof phải là một đối tượng:
instanceof_ex1b.ts
interface IEmployee {
empId: number,
empName: string
}
class Mouse {}
class Cat {}
var anObject1 = {name: 'Tom', gender: 'Male'};
anObject1 instanceof Mouse; // Compile OK!
var anObject2:IEmployee = {empId: 1, empName: 'Donald'};
anObject2 instanceof Cat; // Compile OK!
var anObject3 = new Mouse();
anObject3 instanceof Cat; // Compile OK!
Ví dụ: Trình biên dịch sẽ thông báo lỗi nếu phía bên phải của biểu thức instanceof không phải là một lớp.
instanceof_ex2a.ts
interface IStaff {
staffId: number,
staffName: string
}
class Person {}
let aAnyObject = {}; // Any Objet
aAnyObject instanceof IStaff; // Compile Error !!!! (IStaff is not a class).
aAnyObject instanceof Person; // Compile OK!
- Interface trong TypeScript
- Classes
2. Các ví dụ
Trong ví dụ này chúng ta có các lớp Animal, Duck và Horse, và interface IMove. Chúng ta tạo một vài đối tượng và xem cách hoạt động của toán tử instanceof.
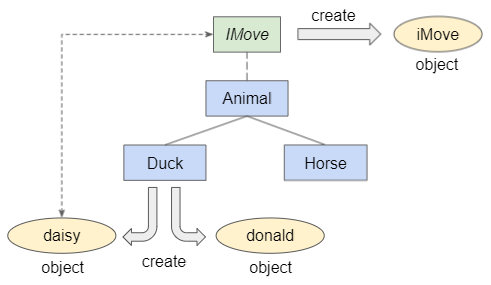
instanceof_ex3.ts
interface IMove {
move(): void;
}
class Animal implements IMove {
move() {
console.log("Animal move!");
}
}
class Duck extends Animal {}
class Horse extends Animal {}
let donald = new Duck();
console.log("donald instancef Duck? " + (donald instanceof Duck)); // true
console.log("donald instancef Animal? " + (donald instanceof Animal)); // true
console.log("donald instancef Horse? " + (donald instanceof Horse)); // false
let daisy: IMove = new Duck();
console.log("daisy instancef Duck? " + (daisy instanceof Duck)); // true
console.log("daisy instancef Animal? " + (daisy instanceof Animal)); // true
console.log("daisy instancef Horse? " + (daisy instanceof Horse)); // false
let iMove: IMove = {
move : function() {
console.log('IMove move!');
}
};
console.log("iMove instancef Duck? " + (iMove instanceof Duck)); // false
console.log("iMove instancef Animal? " + (iMove instanceof Animal)); // false
console.log("iMove instancef Horse? " + (iMove instanceof Horse)); // false
Output:
donald instancef Duck? true
donald instancef Animal? true
donald instancef Horse? false
daisy instancef Duck? true
daisy instancef Animal? true
daisy instancef Horse? false
iMove instancef Duck? false
iMove instancef Animal? false
iMove instancef Horse? false
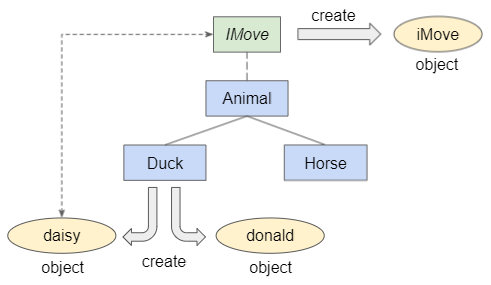
Ví dụ: Sử dụng toán tử instanceof để phân biệt kiểu tham số đã truyền vào hàm.
instanceof_cls_ex1.ts
class Food {
foodName: string;
constructor(foodName: string) {
this.foodName = foodName;
}
}
class Drink {
drinkName: string;
constructor(drinkName: string) {
this.drinkName = drinkName;
}
}
function getName(arg: Food | Drink) {
if(arg instanceof Food) {
let food = arg as Food;
return food.foodName;
} else {
let drink = arg as Drink;
return drink.drinkName;
}
}
let coca = new Drink("Cocacola");
console.log(getName(coca)); // Cocacola
let pho = new Food("Vietnamese Pho");
console.log(getName(pho)); // Vietnamese Pho
Output:
Cocacola
Vietnamese Pho
Duck Typing
Toán tử instanceof được sử dụng để kiểm tra xem một đối tượng có phải là đối tượng của một lớp hay không. Nó không thể sử dụng cho interface, và bạn cần một kỹ thuật khác, hãy xem ví dụ:
duck_typing_ex1.ts
interface IWorker {
workerId: number,
workerName: string
}
interface IStudent {
studentId: number,
studentName: string
}
function getNameOf(arg: IWorker | IStudent) {
let test1 = arg as IWorker;
if(test1.workerId && test1.workerName) {
return test1.workerName;
}
let test2 = arg as IStudent;
return test2.studentName;
}
let tom = {workerId: 1, workerName: 'Tom'};
let jerry = {studentId: 1, studentName: 'Jerry'};
console.log(getNameOf(tom)); // Tom
console.log(getNameOf(jerry)); // Jerry
Output:
Tom
Jerry
Các hướng dẫn TypeScript
- Phân tích JSON trong TypeScript
- Phân tích JSON trong TypeScript với thư viện json2typescript
- Trình chuyển đổi mã nguồn (Transpiler) là gì?
- Cài đặt TypeScript trên Windows
- Chạy ví dụ TypeScript đầu tiên của bạn trong Visual Studio Code
- Biến (Variable) trong TypeScript
- Toán tử typeof trong TypeScript
- Toán tử instanceof trong TypeScript
- Vòng lặp trong TypeScript
- Hướng dẫn và ví dụ hàm trong TypeScript
- Tuples trong TypeScript
- Phương thức trong TypeScript
- Hướng dẫn và ví dụ TypeScript Closures
- Property trong TypeScript
- Constructor trong TypeScript
- Interface trong TypeScript
- Mảng (Array) trong TypeScript
- Không gian tên (Namespace) trong TypeScript
- Module trong TypeScript
Show More