Phân tích JSON trong TypeScript
1. TypeScript JSON
Như đã biết, TypeScript có thể coi là một phiên bản nâng cao của JavaScript. Cuối cùng thì TypeScript cũng được chuyển đổi thành mã JavaScript để cho thể thực thi trên trình duyệt hoặc các môi trường JavaScript khác như NodeJS. TypeScript hỗ trợ tất cả các thư viện JavaScript và các tài liệu API.
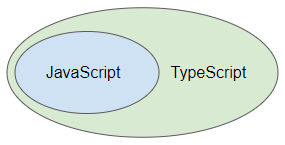
Cách đơn giản nhất để phân tích (parse) các dữ liệu JSON là sử dụng phương thức JSON.parse(..) và JSON.stringify(..). Không có khác biệt gì về cách sử dụng chúng trong JavaScript và TypeScript.
JSON
// Static methods:
parse(text: string, reviver?: (this: any, key: string, value: any) => any): any;
stringify(value: any, replacer?: (this: any, key: string, value: any) => any, space?: string | number): string;
stringify(value: any, replacer?: (number | string)[] | null, space?: string | number): string;
Hãy cân nhắc sử dụng một trong các thư viện JSON dưới đây nếu bạn đang phát triển một dự án TypeScript lớn.
2. JSON.parse(..)
parse(text: string, reviver?: (this: any, key: string, value: any) => any): any;
Chuyển đổi một văn bản JSON thành một đối tượng TypeScript.
- text: Một văn bản JSON.
- reviver: Một hàm tuỳ chọn được gọi cho mỗi property của JSON này. Tham số [key=''] tương ứng với đối tượng JSON toàn cục. Các property ở mức lá sẽ được thực thi đầu tiên, property ở mức gốc ở được thực thi cuối cùng.
Ví dụ: Sử dụng phương thức JSON.parse(..) để chuyển đổi một văn bản JSON thành một đối tượng.
json_parse_ex1.ts
function json_parse_ex1_test() {
let jsonString = ` {
"name": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
}
`;
// Return an object.
let empObj = JSON.parse(jsonString);
console.log(empObj);
console.log(`Address: ${empObj.contact.address}`);
}
json_parse_ex1_test(); // Call the function.
Output:
{
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
}
Address: Address 1
Ví dụ: Sử dụng phương thức JSON.parse(..) với sự tham gia của hàm reviver, để chuyển đổi một văn bản JSON thành một đối tượng của một lớp.
json_parse_ex2.ts
class Employee {
employeeName: string;
employeeEmail: string;
contact: Contact;
constructor(employeeName: string, employeeEmail: string, contact: Contact) {
this.employeeName = employeeName;
this.employeeEmail = employeeEmail;
this.contact = contact;
}
}
class Contact {
address: string;
phone: string;
constructor(address: string, phone: string) {
this.address = address;
this.phone = phone;
}
}
function json_parse_ex2_test() {
let jsonString = ` {
"name": "John Smith",
"email": "john@example.com",
"contact": {
"address": "Address 1",
"phone": "12345"
}
}
`;
// Return an object.
let empObj = JSON.parse(jsonString, function (key: string, value: any) {
if(key == 'name') {
return (value as string).toUpperCase(); // String.
} else if (key == 'contact') {
return new Contact(value.address, value.phone);
} else if (key == '') { // Entire JSON
return new Employee(value.name, value.email, value.contact);
}
return value;
});
console.log(empObj);
console.log(`Employee Name: ${empObj.employeeName}`);
console.log(`Employee Email: ${empObj.employeeEmail}`);
console.log(`Phone: ${empObj.contact.phone}`);
}
json_parse_ex2_test(); // Call the function.
Output:
Employee {
employeeName: 'JOHN SMITH',
employeeEmail: 'john@example.com',
contact: Contact { address: 'Address 1', phone: '12345' }
}
Employee Name: JOHN SMITH
Employee Email: john@example.com
Phone: 12345
3. JSON.stringify(..) *
stringify(value: any, replacer?: (this: any, key: string, value: any) => any, space?: string | number): string;
Chuyển đổi một giá trị hoặc đối tượng thành một văn bản JSON.
- value: Một giá trị, thường là một đối tượng hoặc một mảng, để chuyển đổi thành văn bản JSON.
- replacer: Một hàm tuỳ chọn, được sử dụng để tuỳ biến kết quả.
- space: Thêm các ký tự thụt lề, khoảng trắng hoặc ký tự ngắt dòng vào văn bản JSON để nó dễ đọc hơn.
Ví dụ: Chuyển đổi các giá trị thành văn bản JSON:
json_stringify_ex1a.ts
console.dir(JSON.stringify(1));
console.dir(JSON.stringify(5.9));
console.dir(JSON.stringify(true));
console.dir(JSON.stringify(false));
console.dir(JSON.stringify('falcon'));
console.dir(JSON.stringify("sky"));
console.dir(JSON.stringify(null));
Output:
'1'
'5.9'
'true'
'false'
'"falcon"'
'"sky"'
'null'
Ví dụ: Chuyển đổi một đối tượng thành một văn bản JSON:
json_stringify_ex1b.ts
function json_stringify_ex1b_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let jsonString = JSON.stringify(emp);
console.log(jsonString);
}
json_stringify_ex1b_test(); // Call the function.
Output:
{"name":"John Smith","email":"john@example.com","contact":{"address":"Address 1","phone":"12345"}}
Ví dụ: Sử dụng phương thức JSON.stringify(..) với tham số replacer.
json_stringify_replacer_ex1a.ts
function json_stringify_replacer_ex1a_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let jsonString = JSON.stringify(emp, function (key: string, value: any) {
if (key == 'contact') {
return undefined;
} else if (key == 'name') {
return (value as string).toUpperCase();
}
return value;
});
console.log(jsonString);
}
json_stringify_replacer_ex1a_test(); // Call the function.
Output:
{"name":"JOHN SMITH","email":"john@example.com"}
4. JSON.stringify(..) **
stringify(value: any, replacer?: (number | string)[] | null, space?: string | number): string;
Chuyển đổi một giá trị hoặc đối tượng thành một văn bản JSON.
- value: Một giá trị, thường là một đối tượng hoặc một mảng, để chuyển đổi thành văn bản JSON.
- replacer: Một mảng tuỳ chọn của các string hoặc các số. Là danh sách các property được phê duyệt, chúng sẽ xuất hiện trong văn bản JSON trả về.
- space: Thêm các ký tự thụt lề, khoảng trắng hoặc ký tự ngắt dòng vào văn bản JSON để nó dễ đọc hơn.
json_stringify_replacer_ex2a.ts
function json_stringify_replacer_ex2a_test() {
// An Object:
let emp = {
name: 'John Smith',
email: 'john@example.com',
contact: { address: 'Address 1', phone: '12345' }
};
let replacer = ['email', 'contact', 'address'];
let jsonString = JSON.stringify(emp, replacer, ' ');
console.log(jsonString);
}
json_stringify_replacer_ex2a_test(); // Call the function.
Output:
{
"email": "john@example.com",
"contact": {
"address": "Address 1"
}
}
Các hướng dẫn TypeScript
- Chạy ví dụ TypeScript đầu tiên của bạn trong Visual Studio Code
- Không gian tên (Namespace) trong TypeScript
- Module trong TypeScript
- Toán tử typeof trong TypeScript
- Biến (Variable) trong TypeScript
- Vòng lặp trong TypeScript
- Cài đặt TypeScript trên Windows
- Hướng dẫn và ví dụ hàm trong TypeScript
- Tuples trong TypeScript
- Interface trong TypeScript
- Mảng (Array) trong TypeScript
- Toán tử instanceof trong TypeScript
- Phương thức trong TypeScript
- Hướng dẫn và ví dụ TypeScript Closures
- Constructor trong TypeScript
- Property trong TypeScript
- Phân tích JSON trong TypeScript
- Phân tích JSON trong TypeScript với thư viện json2typescript
- Trình chuyển đổi mã nguồn (Transpiler) là gì?
Show More