Thao tác với tập tin và thư mục trong C#
1. Hệ thống phân cấp các lớp
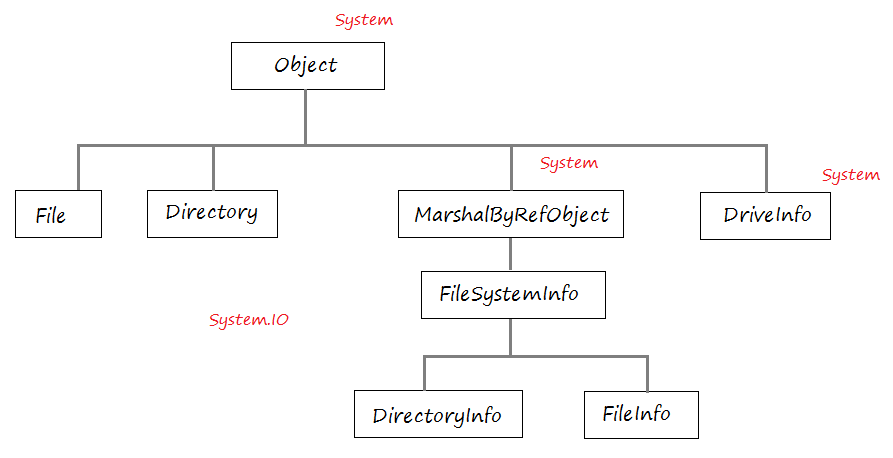
Class | Mô tả |
File | File là một class tiện ích. Nó cung cấp các phương thức tĩnh cho việc tạo, copy, xóa, di chuyển và mở một file, và hỗ trợ tạo đối tượng FileStream. |
Directory | Directory là một class tiện ích. Nó cung cấp các phương thức tĩnh để tạo, di chuyển, và liệt kê các thư mục và các thư mục con. Class này không cho phép có class con. |
FileInfo | FileInfo là một class đại diện cho một file, nó cung cấp các thuộc tính, phương thức cho việc tạo, copy, xóa, di chuyển và mở file. Nó hỗ trợ tạo đối tượng FileStream. Class này không cho phép có class con. |
DirectoryInfo | DirectoryInfo là một class đại diện cho một thư mục, nó cung cấp phương thức cho việc tạo, di chuyển, liệt kê các thư mục và các thư mục con. Class này không cho phép có class con. |
DriveInfo | DirveInfo là một class, nó cung cấp các phương thức truy cập thông tin ổ cứng. |
2. File
File là một class tiện ích. Nó cung cấp các phương thức tĩnh cho việc tạo, copy, xóa, di chuyển và mở một file, và hỗ trợ tạo đối tượng FileStream.
Ví dụ dưới đây kiểm tra xem một đường dẫn file có tồn tại hay không, nếu tồn tại xóa file này.
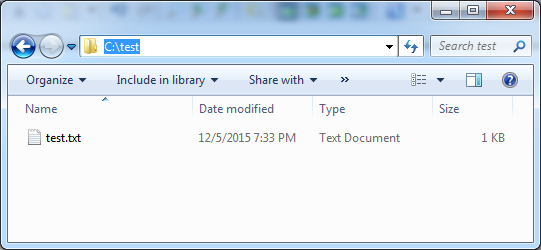
DeleteFileDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DeleteFileDemo
{
public static void Main(string[] args)
{
string filePath = "C:/test/test.txt";
// Kiểm tra đường dẫn này có tồn tại hay không?
if (File.Exists(filePath))
{
// Xóa file
File.Delete(filePath);
// Kiểm tra lại xem file còn tồn tại không.
if (!File.Exists(filePath))
{
Console.WriteLine("File deleted...");
}
}
else
{
Console.WriteLine("File test.txt does not yet exist!");
}
Console.ReadKey();
}
}
}
Chạy ví dụ:
File deleted...
Đổi tên file là một hành động có thể bao gồm di chuyển file tới một thư mục khác và đổi tên file. Trong trường hợp file bị di chuyển tới một thư mục khác phải đảm bảo rằng thư mục mới đã tồn tại.
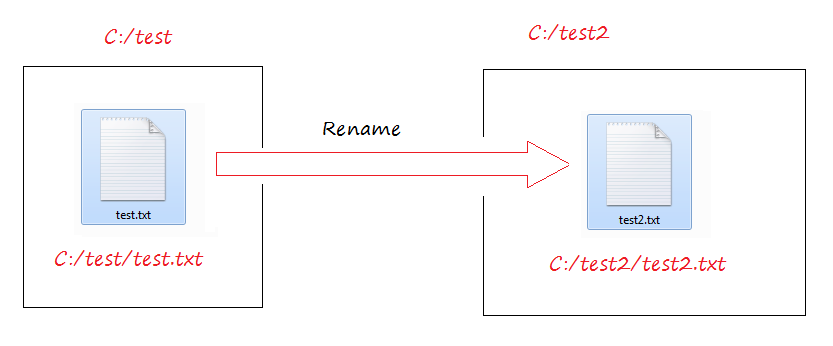
RenameFileDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class RenameFileDemo
{
public static void Main(string[] args)
{
String filePath = "C:/test/test.txt";
if (File.Exists(filePath))
{
Console.WriteLine(filePath + " exist");
Console.WriteLine("Please enter a new name for this file:");
// Một String mà người dùng nhập vào.
// Ví dụ: C:/test/test2.txt
string newFilename = Console.ReadLine();
if (newFilename != String.Empty)
{
// Đổi tên file:
// Có thể bao gồm, chuyển file tới một thư mục cha khác, và đổi tên file.
// Phải đảm bảo rằng thư mục cha mới tồn tại.
// (nếu không ngoại lệ DirectoryNotFoundException sẽ được ném ra).
File.Move(filePath, newFilename);
if (File.Exists(newFilename))
{
Console.WriteLine("The file was renamed to " + newFilename);
}
}
}
else
{
Console.WriteLine("Path " + filePath + " does not exist.");
}
Console.ReadLine();
}
}
}
Chạy ví dụ:
C:/test/test.txt exist
Please enterr a new name for this file:
C:/test/test2.txt
The file was renamed to C:/test/test2.txt
3. Directory
Directory là một class tiện ích. Nó cung cấp các phương thức tĩnh để tạo, di chuyển, và liệt kê các thư mục và các thư mục con. Class này không cho phép có class con.
Ví dụ kiểm tra một đường dẫn thư mục có tồn tại hay không, nếu không tồn tại tạo thư mục đó, ghi ra thông tin thời gian tạo, lần ghi dữ liệu cuối vào thư mục, ....
DirectoryInformationDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DirectoryInformationDemo
{
public static void Main(string[] args)
{
String dirPath = "C:/test/CSharp";
// Kiểm tra xem đường dẫn thư mục tồn tại không.
bool exist = Directory.Exists(dirPath);
// Nếu không tồn tại, tạo thư mục này.
if (!exist)
{
Console.WriteLine(dirPath + " does not exist.");
Console.WriteLine("Create directory: " + dirPath);
// Tạo thư mục.
Directory.CreateDirectory(dirPath);
}
Console.WriteLine("Directory Information " + dirPath);
// In ra các thông tin thư mục trên.
// Thời điểm tạo thư mục.
Console.WriteLine("Creation time: "+ Directory.GetCreationTime(dirPath));
// Thời điểm cuối cùng thư mục có sự thay đổi.
Console.WriteLine("Last Write Time: " + Directory.GetLastWriteTime(dirPath));
// Thư mục cha.
DirectoryInfo parentInfo = Directory.GetParent(dirPath);
Console.WriteLine("Parent directory: " + parentInfo.FullName);
Console.Read();
}
}
}
Chạy ví dụ:
Directory Information C:/test/CSharp
Creation time: 12/5/2015 9:46:10 PM
Last Write Time: 12/5/2015 9:46:10 PM
Parent directory: C:\test
Đổi tên thư mục:
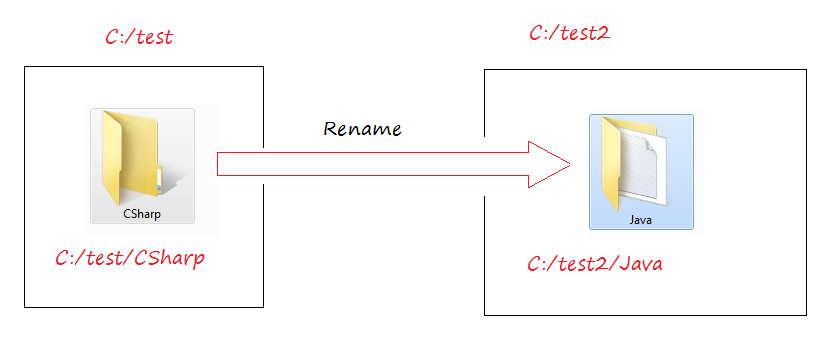
Bạn có thể thay đổi tên một thư mục. Nó có thể làm thư mục đó chuyển ra khỏi thư mục cha hiện tại. Nhưng bạn phải đảm bảo rằng thư mục cha mới đã tồn tại. Ví dụ dưới đây minh họa đổi tên một thư mục:
RenameDirectoryDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class RenameDirectoryDemo
{
public static void Main(string[] args)
{
// Một đường dẫn thư mục.
String dirPath = "C:/test/CSharp";
// Nếu đường dẫn này tồn tại.
if (!Directory.Exists(dirPath))
{
Console.WriteLine(dirPath + " does not exist.");
Console.Read();
return;
}
Console.WriteLine(dirPath + " exist");
Console.WriteLine("Please enter a new name for this directory:");
// String mà người dùng nhập vào.
// Ví dụ: C:/test2/Java
string newDirname = Console.ReadLine();
if (newDirname == String.Empty)
{
Console.WriteLine("You not enter new directory name. Cancel rename.");
Console.Read();
return;
}
// Nếu đường dẫn mà người dùng nhập vào là tồn tại.
if (Directory.Exists(newDirname))
{
Console.WriteLine("Cannot rename directory. New directory already exist.");
Console.Read();
return;
}
// Thư mục cha.
DirectoryInfo parentInfo = Directory.GetParent(newDirname);
// Tạo thư mục cha của thư mục mà người dùng nhập vào.
Directory.CreateDirectory(parentInfo.FullName);
// Bạn có thể đổi đường dẫn (path) của một thư mục.
// nhưng phải đảm bảo đường dẫn cha của đường dẫn mới phải tồn tại.
// (Nếu không ngoại lệ DirectoryNotFoundException sẽ được ném ra).
Directory.Move(dirPath, newDirname);
if (Directory.Exists(newDirname))
{
Console.WriteLine("The directory was renamed to " + newDirname);
}
Console.ReadLine();
}
}
}
Chạy ví dụ:
C:/test/CSharp exist
Please enter a new name for this directory:
C:/test2/Java
The directory was renamed to C:/test2/Java
Ví dụ dưới đây đệ quy và in ra tất cả các thư mục hậu đuệ (con, cháu,...) của một thư mục.
EnumeratingDirectoryDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class EnumeratingDirectoryDemo
{
public static void Main(string[] args)
{
string dirPath = "C:/Windows/System32";
PrintDirectory(dirPath);
Console.Read();
}
// Phương thức đệ quy (Recursive) liệt kê ra các thư mục con của một thư mục.
public static void PrintDirectory(string dirPath)
{
try
{
// Nếu bạn không có quyền truy cập thư mục 'dirPath'
// một ngoại lệ UnauthorizedAccessException sẽ được ném ra.
IEnumerable<string> enums = Directory.EnumerateDirectories(dirPath);
List<string> dirs = new List<string>(enums);
foreach (var dir in dirs)
{
Console.WriteLine(dir);
PrintDirectory(dir);
}
}
// Lỗi bảo mật khi truy cập vào thư mục mà bạn không có quyền.
catch (UnauthorizedAccessException e)
{
Console.WriteLine("Can not access directory: " + dirPath);
Console.WriteLine(e.Message);
}
}
}
}
Chạy ví dụ:
...
C:/Windows/System32\wbem\en-US
C:/Windows/System32\wbem\Logs
C:/Windows/System32\wbem\Repository
C:/Windows/System32\wbem\tmf
C:/Windows/System32\wbem\eml
C:/Windows/System32\WCN
C:/Windows/System32\WCN\en-US
...
4. FileInfo
FileInfo là một class đại diện cho một file, nó cung cấp các thuộc tính, phương thức cho việc tạo, copy, xóa, di chuyển và mở file. Nó hỗ trợ tạo đối tượng FileStream. Class này không cho phép có class con.
Sự khác biệt giữa 2 class File và FileInfo đó là File là một class tiện ích tất các phương thức của nó là tĩnh, còn FileInfo đại diện cho một file cụ thể.
FileInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class FileInfoDemo
{
static void Main(string[] args)
{
// Một đối tượng đại diện cho một file.
FileInfo testFile = new FileInfo("C:/test/test.txt");
if (testFile.Exists)
{
Console.WriteLine(testFile.FullName + " exist.");
// Thông tin thời điểm tạo file.
Console.WriteLine("Creation time: " + testFile.CreationTime);
// Thông tin thời điểm file được sửa đổi lần cuối.
Console.WriteLine("Last Write Time " + testFile.LastWriteTime);
// Tên thư mục chứa file này.
Console.WriteLine("Directory Name: " + testFile.DirectoryName);
}
else
{
Console.WriteLine(testFile.FullName + " does not exist.");
}
Console.Read();
}
}
}
Chạy ví dụ:
C:\test\test.txt exist.
Creation time: 12/5/2015 7:47:40 PM
Last Write Time 12/5/2015 10:17:29 PM
Directory Name: C:\test
Đổi tên file là một hành động có thể bao gồm di chuyển file tới một thư mục khác và đổi tên file. Trong trường hợp file bị di chuyển tới một thư mục khác phải đảm bảo rằng thư mục mới tồn tại.
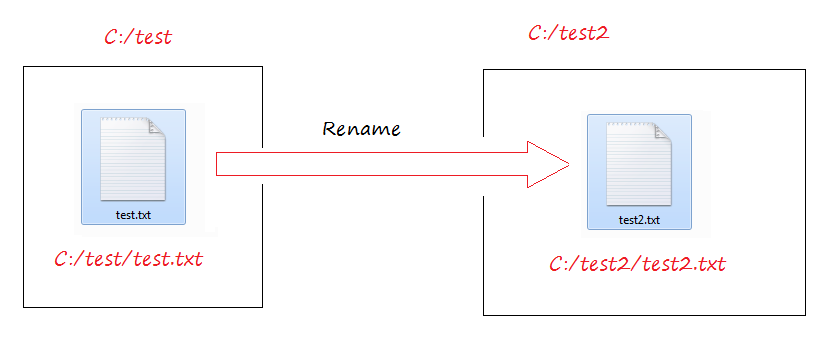
RenameFileInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class RenameFileInfoDemo
{
public static void Main(string[] args)
{
FileInfo fileInfo = new FileInfo("C:/test/test.txt");
if (!fileInfo.Exists)
{
Console.WriteLine(fileInfo.FullName + " does not exist.");
Console.Read();
return;
}
Console.WriteLine(fileInfo.FullName + " exist");
Console.WriteLine("Please enter a new name for this file:");
// Một String mà người dùng nhập vào.
// Ví dụ: C:/test/test2.txt
string newFilename = Console.ReadLine();
if (newFilename == String.Empty)
{
Console.WriteLine("You not enter new file name. Cancel rename");
Console.Read();
return;
}
FileInfo newFileInfo = new FileInfo(newFilename);
// Nếu 'newFileInfo' tồn tại (Không thể đổi tên).
if (newFileInfo.Exists)
{
Console.WriteLine("Can not rename file to " + newFileInfo.FullName + ". File already exist.");
Console.Read();
return;
}
// Tạo thư mục cha của 'newFileInfo'.
newFileInfo.Directory.Create();
// Đổi tên file.
fileInfo.MoveTo(newFileInfo.FullName);
// Refresh.
newFileInfo.Refresh();
if (newFileInfo.Exists)
{
Console.WriteLine("The file was renamed to " + newFileInfo.FullName);
}
Console.ReadLine();
}
}
}
Chạy ví dụ:
C:\test\test.txt exist
Please enter a new name for this file:
C:/test2/test2.txt
The file was renamed to C:\test2\test2.txt
5. DirectoryInfo
DirectoryInfo là một class đại diện cho một thư mục, nó cung cấp phương thức cho việc tạo, di chuyển, liệt kê các thư mục và các thư mục con. Class này không cho phép có class con.
Sự khác biệt giữa 2 class Directory và DirectoryInfo đó là Directory là một class tiện ích, tất cả các phương thức của nó là tĩnh, còn DirectoryInfo đại diện cho một thư mục cụ thể.
DirectoryInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DirectoryInfoDemo
{
static void Main(string[] args)
{
// Một đối tượng đại diện cho một thư mục.
DirectoryInfo dirInfo = new DirectoryInfo("C:/Windows/System32/drivers");
// Ghi ra các thông tin.
// Thông tin ngày tạo file.
Console.WriteLine("Creation time: " + dirInfo.CreationTime);
// Thông tin thời điểm file được sửa đổi lần cuối.
Console.WriteLine("Last Write Time " + dirInfo.LastWriteTime);
// Tên thư mục.
Console.WriteLine("Directory Name: " + dirInfo.FullName);
// Một mảng các thư mục con.
DirectoryInfo[] childDirs = dirInfo.GetDirectories();
// Mảng các file nằm trong thư mục.
FileInfo[] childFiles = dirInfo.GetFiles();
foreach(DirectoryInfo childDir in childDirs ){
Console.WriteLine(" - Directory: " + childDir.FullName);
}
foreach (FileInfo childFile in childFiles)
{
Console.WriteLine(" - File: " + childFile.FullName);
}
Console.Read();
}
}
}
Chạy ví dụ:
Creation time: 7/14/2009 10:20:14 AM
Last Write Time 11/21/2010 2:06:51 PM
Directory Name: C:\Windows\System32\drivers
- Directory: C:\Windowws\System32\drivers\en-US
- File: C:\Windowws\System32\gm.dls
- File: C:\Windowws\System32\gmreadme.txt
- File: C:\Windowws\System32\winmount.sys
6. DriveInfo
DirveInfo là một class, nó cung cấp các phương thức truy cập thông tin ổ cứng.
DriveInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DriveInfoDemo
{
static void Main(string[] args)
{
DriveInfo[] drives = DriveInfo.GetDrives();
foreach (DriveInfo drive in drives)
{
Console.WriteLine(" ============================== ");
// Tên của ổ đĩa (C, D, ..)
Console.WriteLine("Drive {0}", drive.Name);
// Loại ổ đĩa (Removable,..)
Console.WriteLine(" Drive type: {0}", drive.DriveType);
// Nếu ổ đĩa sẵn sàng.
if (drive.IsReady)
{
Console.WriteLine(" Volume label: {0}", drive.VolumeLabel);
Console.WriteLine(" File system: {0}", drive.DriveFormat);
Console.WriteLine(
" Available space to current user:{0, 15} bytes",
drive.AvailableFreeSpace);
Console.WriteLine(
" Total available space: {0, 15} bytes",
drive.TotalFreeSpace);
Console.WriteLine(
" Total size of drive: {0, 15} bytes ",
drive.TotalSize);
}
}
Console.Read();
}
}
}
Chạy ví dụ:
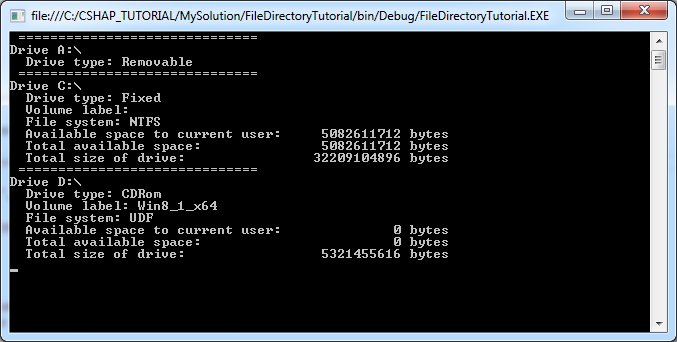
Các hướng dẫn lập trình C#
- Thừa kế và đa hình trong C#
- Bắt đầu với C# cần những gì?
- Học nhanh C# cho người mới bắt đầu
- Cài đặt Visual Studio 2013 trên Windows
- Abstract class và Interface trong C#
- Cài đặt Visual Studio 2015 trên Windows
- Nén và giải nén trong C#
- Hướng dẫn lập trình đa luồng trong C#
- Hướng dẫn và ví dụ C# Delegate và Event
- Cài đặt AnkhSVN trên Windows
- Lập trình C# theo nhóm sử dụng Visual Studio và SVN
- Cài đặt .Net Framework
- Access Modifier trong C#
- Hướng dẫn và ví dụ C# String và StringBuilder
- Hướng dẫn và ví dụ C# Property
- Hướng dẫn và ví dụ C# Enum
- Hướng dẫn và ví dụ C# Structure
- Hướng dẫn và ví dụ C# Generics
- Hướng dẫn xử lý ngoại lệ trong C#
- Hướng dẫn và ví dụ Date Time trong C#
- Thao tác với tập tin và thư mục trong C#
- Hướng dẫn sử dụng Stream - luồng vào ra nhị phân trong C#
- Hướng dẫn sử dụng biểu thức chính quy trong C#
- Kết nối cơ sở dữ liệu SQL Server trong C#
- Làm việc với cơ sở dữ liệu SQL Server trong C#
- Kết nối cơ sở dữ liệu MySQL trong C#
- Làm việc với cơ sở dữ liệu MySQL trong C#
- Kết nối cơ sở dữ liệu Oracle trong C# không cần Oracle Client
- Làm việc với cơ sở dữ liệu Oracle trong C#
Show More