Kết nối cơ sở dữ liệu MySQL trong C#
1. Download MySQL Connector cho Dotnet
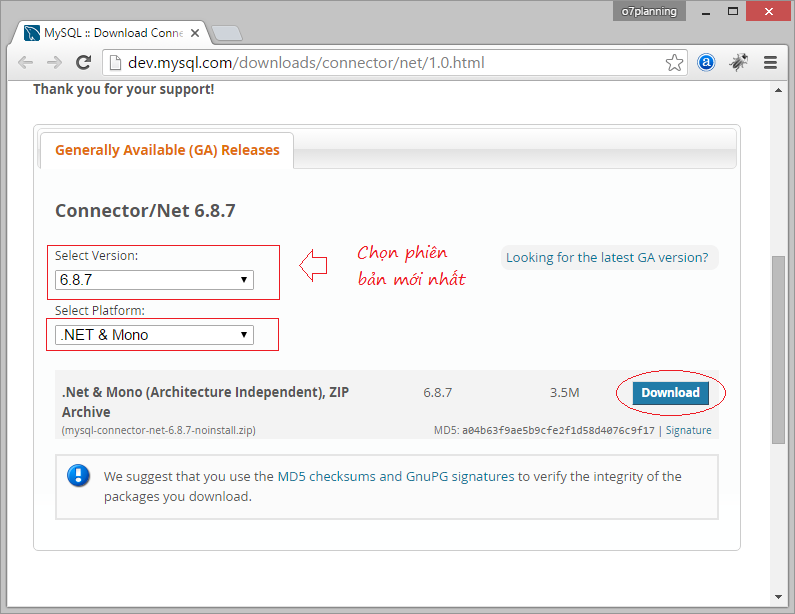
Việc download yêu cầu bạn phải đăng nhập vào. Bạn có thể đăng ký miễn phí một tài khoản. Kết quả bạn download được:
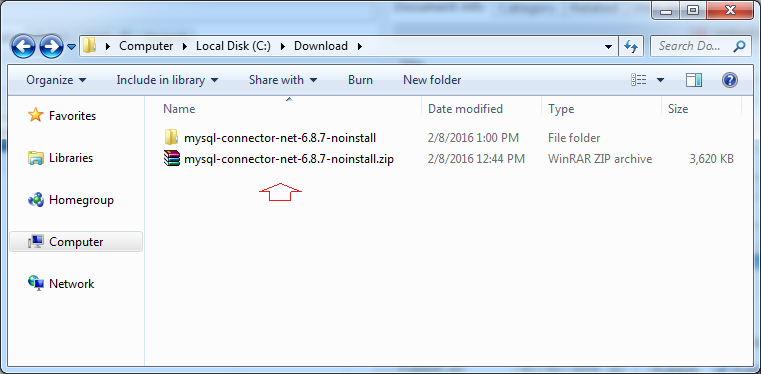
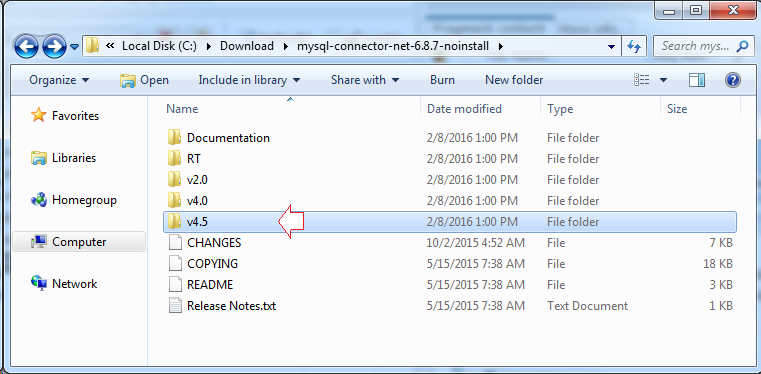
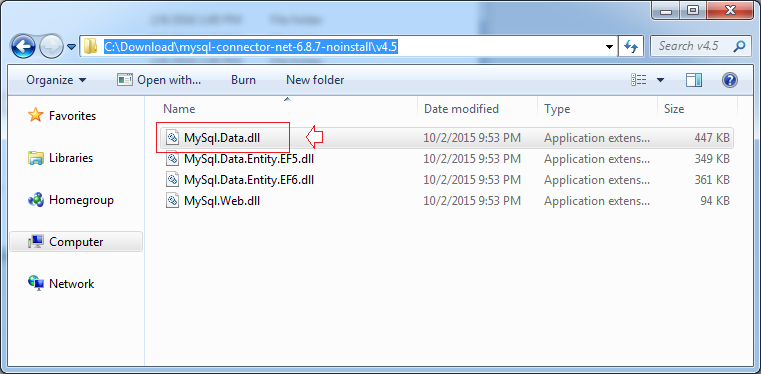
2. Kết nối C# vào MySQL
Tạo một Project có tên ConnectMySQL:
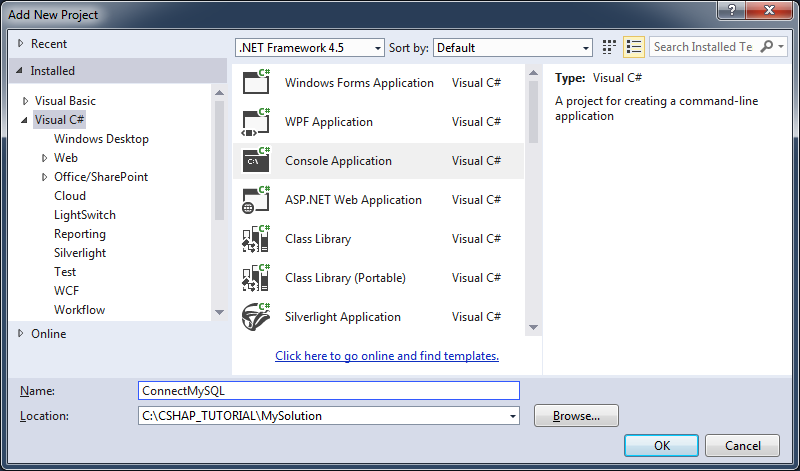
Project đã được tạo ra, bạn cần khai báo tham chiếu (Reference) tới thư viện MySql.Data.dll.
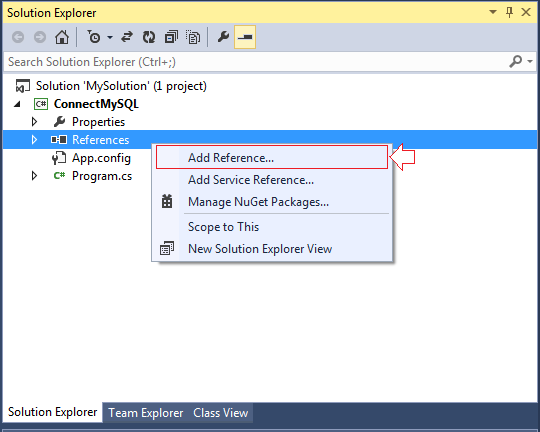
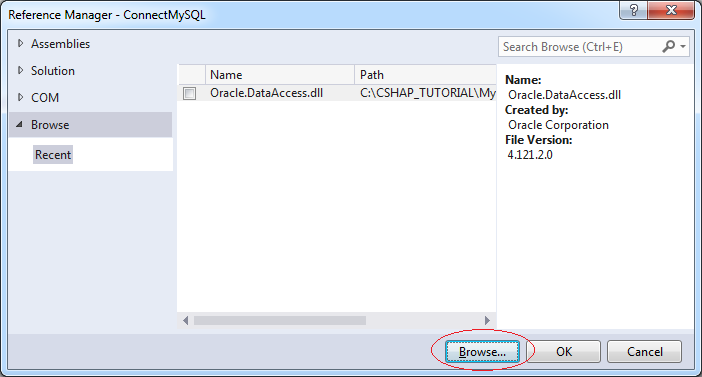
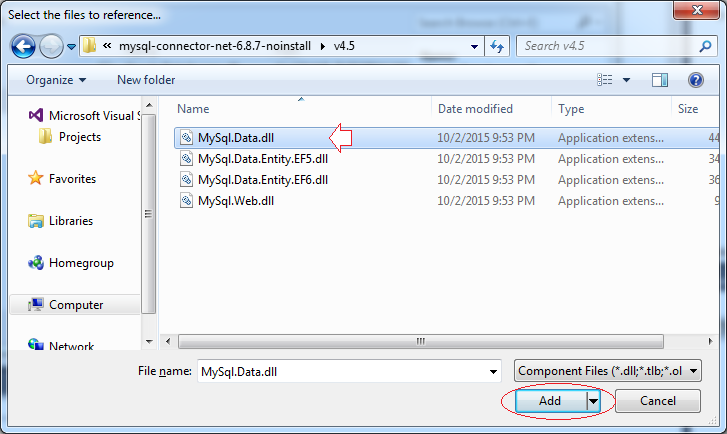
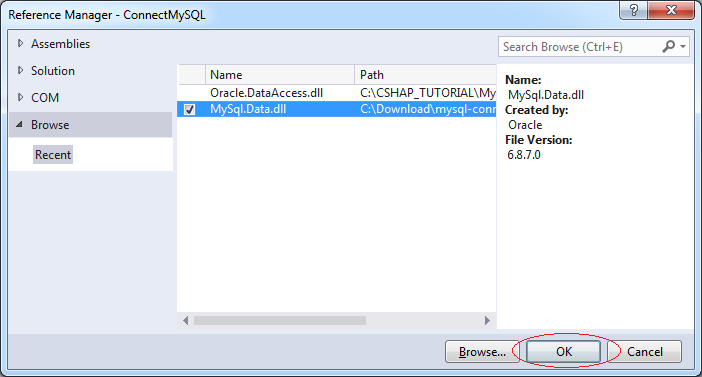
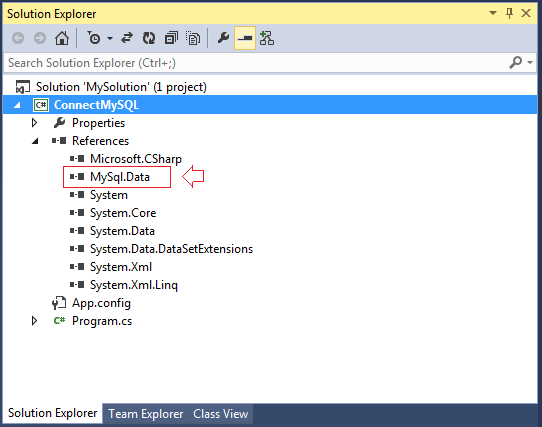
Tạo một vài class tiện ích giúp kết nối vào database MySQL:
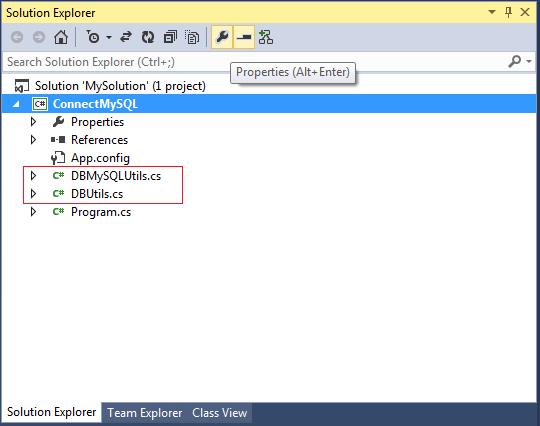
DBMySQLUtils.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
namespace Tutorial.SqlConn
{
class DBMySQLUtils
{
public static MySqlConnection
GetDBConnection(string host, int port, string database, string username, string password)
{
// Connection String.
String connString = "Server=" + host + ";Database=" + database
+ ";port=" + port + ";User Id=" + username + ";password=" + password;
MySqlConnection conn = new MySqlConnection(connString);
return conn;
}
}
}
DBUtils.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
namespace Tutorial.SqlConn
{
class DBUtils
{
public static MySqlConnection GetDBConnection( )
{
string host = "192.168.205.130";
int port = 3306;
string database = "simplehr";
string username = "root";
string password = "1234";
return DBMySQLUtils.GetDBConnection(host, port, database, username, password);
}
}
}
Test kết nối:
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Tutorial.SqlConn;
using MySql.Data.MySqlClient;
namespace ConnectMySQL
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Getting Connection ...");
MySqlConnection conn = DBUtils.GetDBConnection();
try
{
Console.WriteLine("Openning Connection ...");
conn.Open();
Console.WriteLine("Connection successful!");
}
catch(Exception e)
{
Console.WriteLine("Error: " + e.Message);
}
Console.Read();
}
}
}
Chạy class Program để test kết nối:
Getting Connection ...
Openning Connection ...
Connection successful!
3. Làm việc với MySQL sử dụng C#
Bạn có thể xem tiếp tài liệu làm việc với MySQL sử dụng C#:
Nội dung bao gồm:
- Insert
- Update
- Delete
- Gọi Hàm (Function) và thủ tục (Procedure)
4. Phụ lục: Các lỗi kết nối và cách khắc phục
Trong trường hợp bạn kết nối với database MySQL nằm trên một máy tính khác bạn có thể nhận một lỗi như minh họa dưới đây, nguyên nhân là do MySQL đang vô hiệu hóa các kết nối từ máy tính khác, bạn cần phải "cấu hình MySQL" cho phép điều này. Bạn có thể xem hướng dẫn tại:
Error: Host '192.168.205.134' is not allowed to connect to this MySQL server
Nếu MySQL của bạn cài đặt trên máy tính khác (Với hệ điều hành Windows), bạn cũng cần phải mở firewall cho cổng 3306.
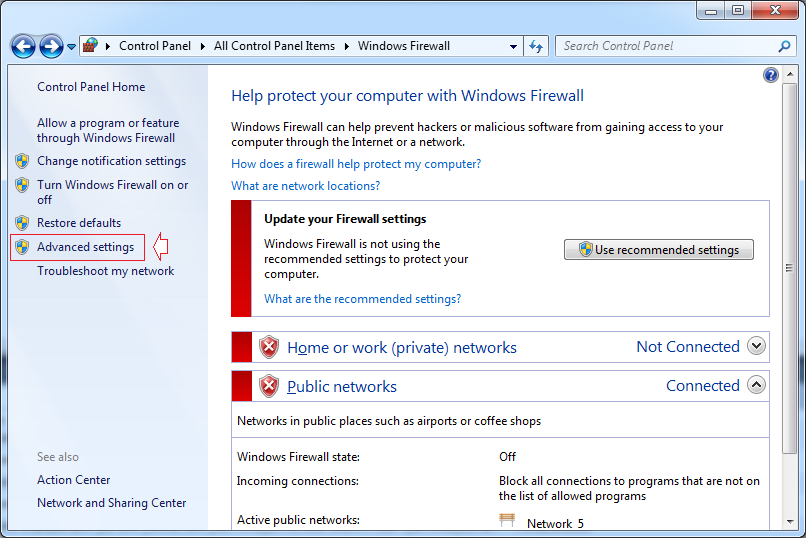
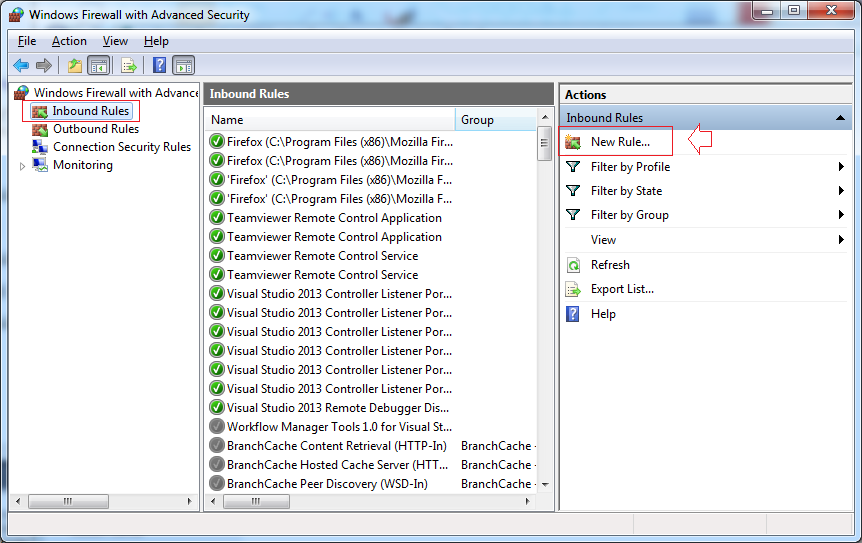
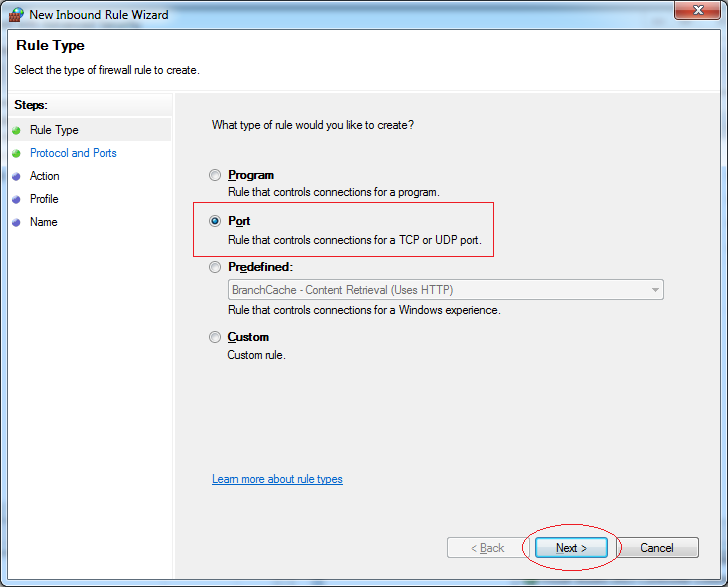
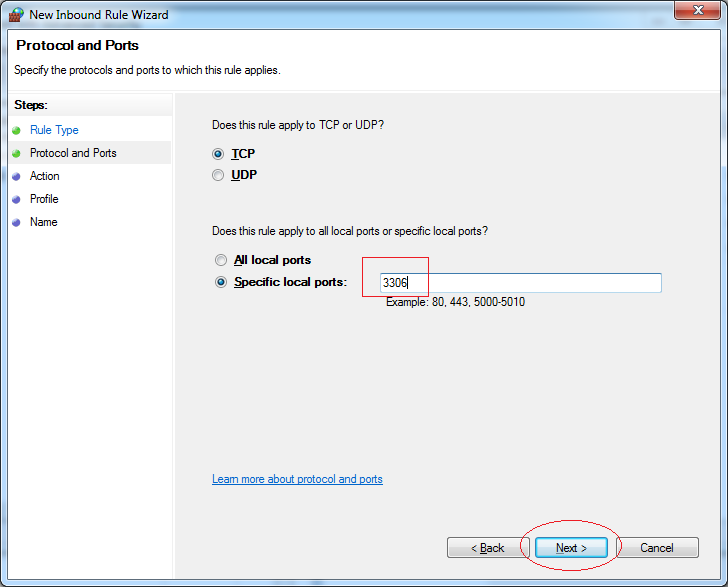
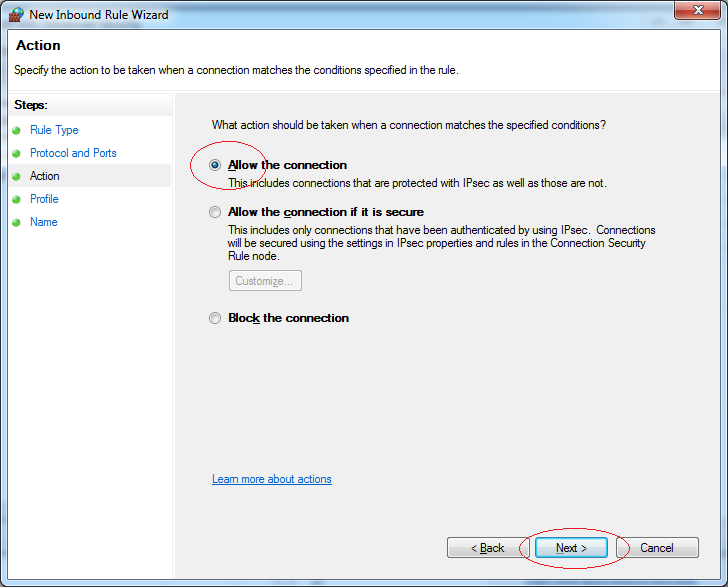
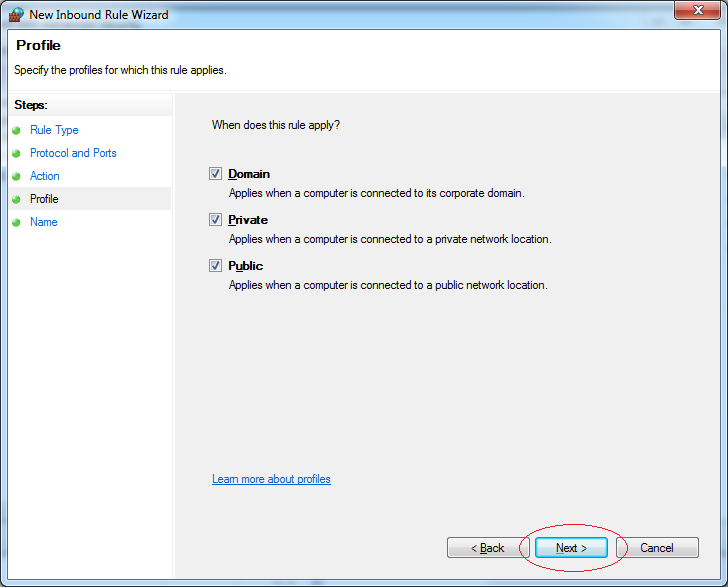
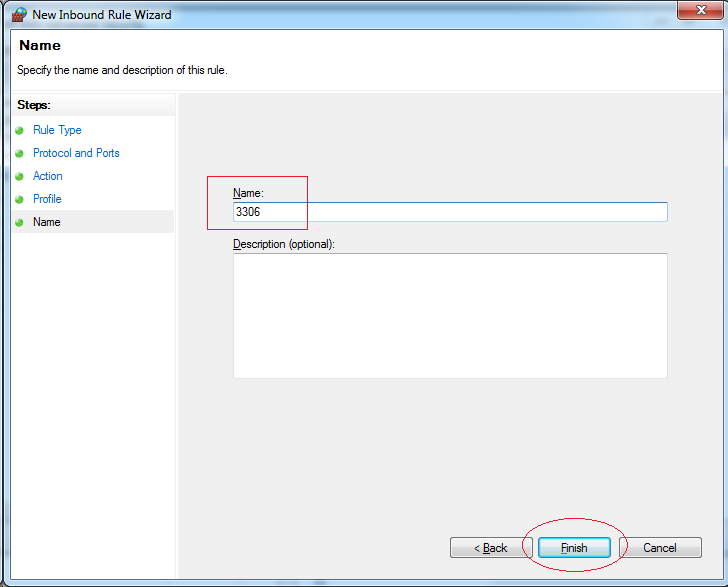
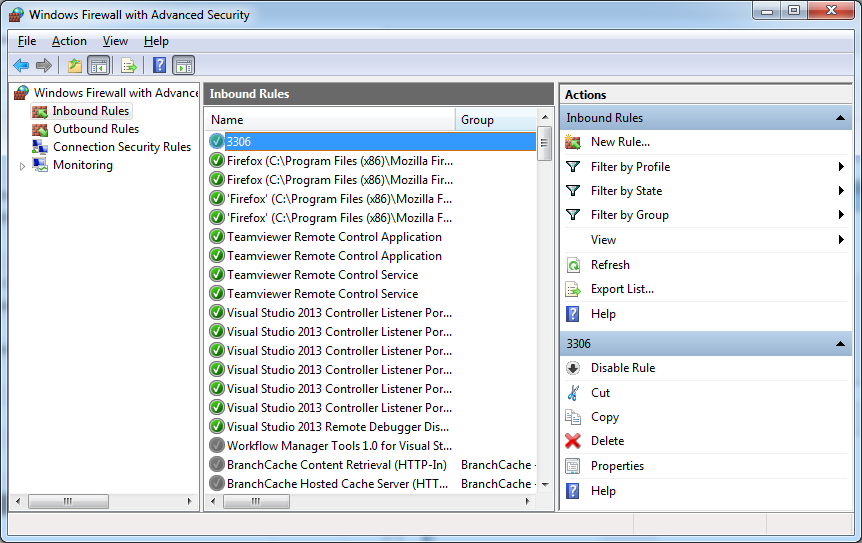
Các hướng dẫn lập trình C#
- Thừa kế và đa hình trong C#
- Bắt đầu với C# cần những gì?
- Học nhanh C# cho người mới bắt đầu
- Cài đặt Visual Studio 2013 trên Windows
- Abstract class và Interface trong C#
- Cài đặt Visual Studio 2015 trên Windows
- Nén và giải nén trong C#
- Hướng dẫn lập trình đa luồng trong C#
- Hướng dẫn và ví dụ C# Delegate và Event
- Cài đặt AnkhSVN trên Windows
- Lập trình C# theo nhóm sử dụng Visual Studio và SVN
- Cài đặt .Net Framework
- Access Modifier trong C#
- Hướng dẫn và ví dụ C# String và StringBuilder
- Hướng dẫn và ví dụ C# Property
- Hướng dẫn và ví dụ C# Enum
- Hướng dẫn và ví dụ C# Structure
- Hướng dẫn và ví dụ C# Generics
- Hướng dẫn xử lý ngoại lệ trong C#
- Hướng dẫn và ví dụ Date Time trong C#
- Thao tác với tập tin và thư mục trong C#
- Hướng dẫn sử dụng Stream - luồng vào ra nhị phân trong C#
- Hướng dẫn sử dụng biểu thức chính quy trong C#
- Kết nối cơ sở dữ liệu SQL Server trong C#
- Làm việc với cơ sở dữ liệu SQL Server trong C#
- Kết nối cơ sở dữ liệu MySQL trong C#
- Làm việc với cơ sở dữ liệu MySQL trong C#
- Kết nối cơ sở dữ liệu Oracle trong C# không cần Oracle Client
- Làm việc với cơ sở dữ liệu Oracle trong C#
Show More