Hướng dẫn và ví dụ Date Time trong C#
1. Các class liên quan Date, Time trong C#
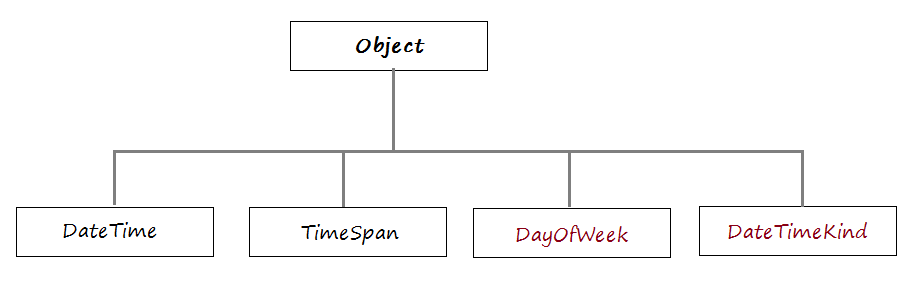
public DateTime(int year, int month, int day)
public DateTime(int year, int month, int day, Calendar calendar)
public DateTime(int year, int month, int day, int hour, int minute, int second)
public DateTime(int year, int month, int day, int hour, int minute, int second, Calendar calendar)
public DateTime(int year, int month, int day, int hour, int minute, int second, DateTimeKind kind)
public DateTime(int year, int month, int day, int hour, int minute, int second, int millisecond)
public DateTime(int year, int month, int day, int hour,
int minute, int second, int millisecond, Calendar calendar)
public DateTime(int year, int month, int day, int hour,
int minute, int second, int millisecond, Calendar calendar, DateTimeKind kind)
public DateTime(int year, int month, int day, int hour,
int minute, int second, int millisecond, DateTimeKind kind)
public DateTime(long ticks)
public DateTime(long ticks, DateTimeKind kind)
// Đối tượng mô tả thời điểm hiện tại.
DateTime now = DateTime.Now;
Console.WriteLine("Now is "+ now);
2. Các thuộc tính DateTime
Thuộc tính | Kiểu dữ liệu | Mô tả |
Date | DateTime | Lấy ra thành phần date (Chỉ chứa thông tin ngày tháng năm) của đối tượng này. |
Day | int | Lấy ra ngày trong tháng được mô tả bởi đối tượng này. |
DayOfWeek | DayOfWeek | Lấy ra ngày trong tuần được đại diện bởi đối tượng này. |
DayOfYear | int | Lấy ra ngày của năm được đại diện bởi đối tượng này. |
Hour | int | Lấy ra thành phần giờ được đại diện bởi đối tượng này. |
Kind | DateTimeKind | Lấy giá trị cho biết liệu thời gian được đại diện bởi đối tượng này dựa trên thời gian địa phương, Coordinated Universal Time (UTC), hoặc không. |
Millisecond | int | Lấy ra thành phần mili giây được đại diện bởi đối tượng này. |
Minute | int | Lấy ra thành phần phút được đại diện bởi đối tượng này. |
Month | int | Lấy ra thành phần tháng được đại diện bởi đối tượng này. |
Now | DateTime | Lấy ra đối tượng DateTime được sét thông tin ngày tháng thời gian hiện tại theo máy tính địa phương. |
Second | int | Lấy ra thành phần giây được đại diện bởi đối tượng này. |
Ticks | long | Lấy ra số lượng "tick" được đại diện bởi đối tượng này. |
TimeOfDay | TimeSpan | Trả về thời gian của ngày được đại diện bởi đối tượng này. |
Today | DateTime | Trả về ngày hiện tại. |
UtcNow | DateTime | Trả về đối tượng DateTime được sét thời gian hiện tại của máy tính, được thể hiện dưới dạng Coordinated Universal Time (UTC). |
Year | int | Lấy ra thành phần năm được đại diện bởi đối tượng này. |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class DateTimePropertiesExample
{
public static void Main(string[] args)
{
// Tạo một đối tượng DateTime (năm, tháng, ngày, giờ, phút, giây).
DateTime aDateTime = new DateTime(2005, 11, 20, 12, 1, 10);
// In ra các thông tin:
Console.WriteLine("Day:{0}", aDateTime.Day);
Console.WriteLine("Month:{0}", aDateTime.Month);
Console.WriteLine("Year:{0}", aDateTime.Year);
Console.WriteLine("Hour:{0}", aDateTime.Hour);
Console.WriteLine("Minute:{0}", aDateTime.Minute);
Console.WriteLine("Second:{0}", aDateTime.Second);
Console.WriteLine("Millisecond:{0}", aDateTime.Millisecond);
// Enum {Monday, Tuesday,... Sunday}
DayOfWeek dayOfWeek = aDateTime.DayOfWeek;
Console.WriteLine("Day of Week:{0}", dayOfWeek );
Console.WriteLine("Day of Year: {0}", aDateTime.DayOfYear);
// Một đối tượng chỉ mô tả thời gian (giờ phút giây,..)
TimeSpan timeOfDay = aDateTime.TimeOfDay;
Console.WriteLine("Time of Day:{0}", timeOfDay);
// Quy đổi ra Ticks (1 giây = 10.000.000 Ticks)
Console.WriteLine("Tick:{0}", aDateTime.Ticks);
// {Local, Itc, Unspecified}
DateTimeKind kind = aDateTime.Kind;
Console.WriteLine("Kind:{0}", kind);
Console.Read();
}
}
}
Day:20
Month:11
Year:2005
Hour:12
Minute:1
Second:10
Millisecond:0
Day of Week:Sunday
Day of Year: 325
Time of Day:12:01:10
Tick:632680848700000000
3. Thêm và bớt thời gian
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class AddSubtractExample
{
public static void Main(string[] args)
{
// Thời điểm hiện tại.
DateTime aDateTime = DateTime.Now;
Console.WriteLine("Now is " + aDateTime);
// Một khoảng thời gian.
// 1 giờ + 1 phút
TimeSpan aInterval = new System.TimeSpan(0, 1, 1, 0);
// Thêm một khoảng thời gian.
DateTime newTime = aDateTime.Add(aInterval);
Console.WriteLine("After add 1 hour, 1 minute: " + newTime);
// Trừ khoảng thời gian.
newTime = aDateTime.Subtract(aInterval);
Console.WriteLine("After subtract 1 hour, 1 minute: " + newTime);
Console.Read();
}
}
}
Now is 12/8/2015 10:52:03 PM
After add 1 hour, 1 minute: 12/8/2015 11:53:03 PM
After subtract 1 hour, 1 minute: 12/8/2015 9:51:03 PM
- AddYears
- AddDays
- AddMinutes
- ...
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class AddSubtractExample2
{
public static void Main(string[] args)
{
// Thời điểm hiện tại.
DateTime aDateTime = DateTime.Now;
Console.WriteLine("Now is " + aDateTime);
// Thêm 1 năm
DateTime newTime = aDateTime.AddYears(1);
Console.WriteLine("After add 1 year: " + newTime);
// Trừ đi 1 giờ
newTime = aDateTime.AddHours(-1);
Console.WriteLine("After add -1 hour: " + newTime);
Console.Read();
}
}
}
Now is 12/8/2015 11:28:34 PM
After add 1 year: 12/8/2016 11:28:34 PM
After add -1 hour: 12/8/2015 10:28:34 PM
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class FirstLastDayDemo
{
public static void Main(string[] args)
{
Console.WriteLine("Today is " + DateTime.Today);
DateTime yesterday = GetYesterday();
Console.WriteLine("Yesterday is " + yesterday);
// Ngày đầu tiên của tháng 2 năm 2015
DateTime aDateTime = GetFistDayInMonth(2015, 2);
Console.WriteLine("First day of 2-2015: " + aDateTime);
// Ngày cuối cùng của tháng 2 năm 2015
aDateTime = GetLastDayInMonth(2015, 2);
Console.WriteLine("Last day of 2-2015: " + aDateTime);
// Ngày đầu tiên của năm 2015
aDateTime = GetFirstDayInYear(2015);
Console.WriteLine("First day year 2015: " + aDateTime);
// Ngày cuối cùng của năm 2015
aDateTime = GetLastDayInYear(2015);
Console.WriteLine("Last day year 2015: " + aDateTime);
Console.Read();
}
// Trả về ngày hôm qua.
public static DateTime GetYesterday()
{
// Ngày hôm nay.
DateTime today = DateTime.Today;
// Trừ đi một ngày.
return today.AddDays(-1);
}
// Trả về ngày đầu tiên của năm
public static DateTime GetFirstDayInYear(int year)
{
DateTime aDateTime = new DateTime(year, 1, 1);
return aDateTime;
}
// Trả về ngày cuối cùng của năm.
public static DateTime GetLastDayInYear(int year)
{
DateTime aDateTime = new DateTime(year +1, 1, 1);
// Trừ đi một ngày.
DateTime retDateTime = aDateTime.AddDays(-1);
return retDateTime;
}
// Trả về ngày đầu tiên của tháng
public static DateTime GetFistDayInMonth(int year, int month)
{
DateTime aDateTime = new DateTime(year, month, 1);
return aDateTime;
}
// Trả về ngày cuối cùng của tháng.
public static DateTime GetLastDayInMonth(int year, int month)
{
DateTime aDateTime = new DateTime(year, month, 1);
// Cộng thêm 1 tháng và trừ đi một ngày.
DateTime retDateTime = aDateTime.AddMonths(1).AddDays(-1);
return retDateTime;
}
}
}
Today is 12/9/2015 12:00:00 AM
Yesterday is 12/8/2015 12:00:00 AM
First day of 2-2015: 2/1/2015 12:00:00 AM
Last day of 2-2015: 2/28/2015 12:00:00 AM
First day year 2015: 2/1/2015 12:00:00 AM
Last day year 2015: 12/31/2015 12:00:00 AM
4. Đo khoảng thời gian
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class IntervalDemo
{
public static void Main(string[] args)
{
// Thời điểm hiện tại.
DateTime aDateTime = DateTime.Now;
// Thời điểm năm 2000
DateTime y2K = new DateTime(2000,1,1);
// Khoảng thời gian từ năm 2000 tới nay.
TimeSpan interval = aDateTime.Subtract(y2K);
Console.WriteLine("Interval from Y2K to Now: " + interval);
Console.WriteLine("Days: " + interval.Days);
Console.WriteLine("Hours: " + interval.Hours);
Console.WriteLine("Minutes: " + interval.Minutes);
Console.WriteLine("Seconds: " + interval.Seconds);
Console.Read();
}
}
}
Interval from Y2K to Now: 5820.23:51:08.1194036
Days: 5820
Hours: 23
Minutes: 51
Seconds: 8
5. So sánh hai đối tượng DateTime
// Nếu giá trị < 0 nghĩa là firstDateTime sớm hơn (đứng trước)
// Nếu giá trị > 0 nghĩa là secondDateTime sớm hơn (đứng trước).
// Nếu giá trị = 0 nghĩa là 2 đối tượng này giống nhau về mặt thời gian.
public static int Compare(DateTime firstDateTime, DateTime secondDateTime);

using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class CompareDateTimeExample
{
public static void Main(string[] args)
{
// Thời điểm hiện tại.
DateTime firstDateTime = new DateTime(2000, 9, 2);
DateTime secondDateTime = new DateTime(2011, 1, 20);
int compare = DateTime.Compare(firstDateTime, secondDateTime);
Console.WriteLine("First DateTime: " + firstDateTime);
Console.WriteLine("Second DateTime: " + secondDateTime);
Console.WriteLine("Compare value: " + compare);// -1
if (compare < 0)
{
// firstDateTime sớm hơn secondDateTime.
Console.WriteLine("firstDateTime is earlier than secondDateTime");
}
else
{
// firstDateTime muộn hơn secondDateTime
Console.WriteLine("firstDateTime is laster than secondDateTime");
}
Console.Read();
}
}
}
First DateTime: 9/2/2000 12:00:00 AM
Second DateTime: 1/20/2011 12:00:00 AM
Compare value: -1
firstDateTime is earlier than secondDateTime
6. Định dạng tiêu chuẩn DateTime
- Chuyển đổi giá trị của đối tượng này (DateTime) thành một mảng các string đã định dạng theo các chuẩn được hỗ trợ.
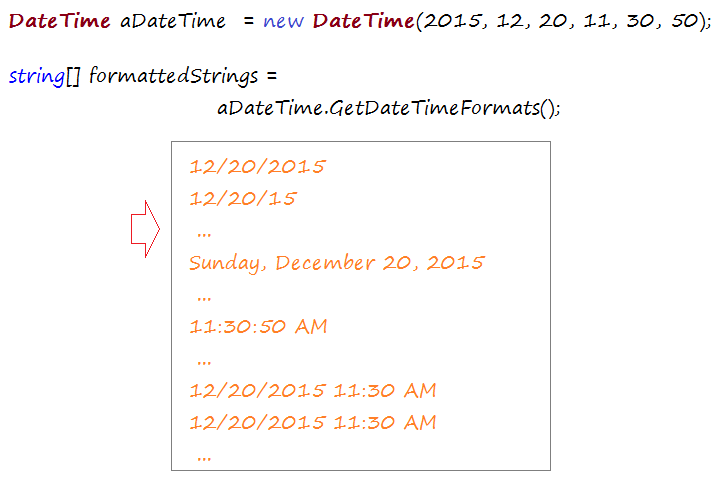
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class AllStandardFormatsDemo
{
public static void Main(string[] args)
{
DateTime aDateTime = new DateTime(2015, 12, 20, 11, 30, 50);
// Các định dạng date-time được hỗ trợ.
string[] formattedStrings = aDateTime.GetDateTimeFormats();
foreach (string format in formattedStrings)
{
Console.WriteLine(format);
}
Console.Read();
}
}
}
12/20/2015
12/20/15
12/20/15
12/20/2015
15/12/20
2015-12-20
20-Dec-15
Sunday, December 20, 2015
December 20, 2015
Sunday, 20 December, 2015
20 December, 2015
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30
Sunday, December 20, 2015 11:30
December 20, 2015 11:30 AM
December 20, 2015 11:30 AM
December 20, 2015 11:30
December 20, 2015 11:30
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30
Sunday, 20 December, 2015 11:30
20 December, 2015 11:30 AM
20 December, 2015 11:30 AM
...
Phương thức | Mô tả |
ToString(String, IFormatProvider) | Chuyển đổi các giá trị của đối tượng DateTime hiện tại thành string đại diện tương đương của nó bằng cách sử dụng định dạng cho bởi tham số String, và các thông tin định dạng văn hóa (Culture) cho bởi tham số IFormatProvider. |
ToString(IFormatProvider) | Chuyển đổi giá trị của đối tượng DateTime hiện tại thành một string tương ứng với thông tin định dạng văn hóa (Culture) cho bởi tham số IFormatProvider. |
ToString(String) | Chuyển đổi các giá trị của đối tượng DateTime hiện tại thành một string tương đương của nó bằng cách sử dụng định dạng cho bởi tham số String và các quy ước định dạng của nền văn hóa hiện tại. |
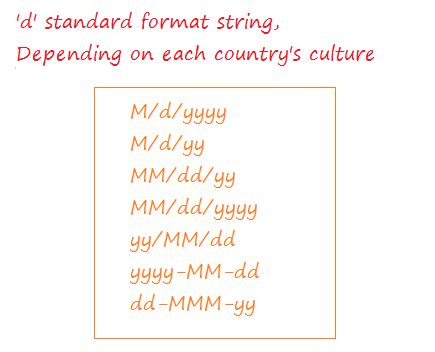
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Globalization;
namespace DateTimeTutorial
{
class SimpleDateTimeFormat
{
public static void Main(string[] args)
{
DateTime aDateTime = new DateTime(2015, 12, 20, 11, 30, 50);
Console.WriteLine("DateTime: " + aDateTime);
String d_formatString = aDateTime.ToString("d");
Console.WriteLine("Format 'd' : " + d_formatString);
// Một đối tượng mô tả văn hóa của Mỹ (en-US Culture)
CultureInfo enUs = new CultureInfo("en-US");
// ==> 12/20/2015 (MM/dd/yyyy)
Console.WriteLine("Format 'd' & en-US: " + aDateTime.ToString("d", enUs));
// Theo văn hóa Việt Nam.
CultureInfo viVn = new CultureInfo("vi-VN");
// ==> 12/20/2015 (dd/MM/yyyy)
Console.WriteLine("Format 'd' & vi-VN: " + aDateTime.ToString("d", viVn));
Console.Read();
}
}
}
DateTime: 12/20/2015 11:30:50 AM
Format 'd' : 12/20/2015
Format 'd' & en-US: 12/20/2015
Format 'd' & vi-VN: 20/12/2015
Code | Pattern |
"d" | Ngày tháng năm ngắn |
"D" | Ngày tháng năm dài |
"f" | Ngày tháng năm dài, thời gian ngắn |
"F" | Ngày tháng năm dài, thời gian dài. |
"g" | Ngày tháng thời gian nói chung. Thời gian ngắn. |
"G" | Ngày tháng thời gian nói chung. Thời gian dài. |
"M", 'm" | Tháng/ngày. |
"O", "o" | Round-trip date/time. |
"R", "r" | RFC1123 |
"s" | Ngày tháng thời gian có thể sắp xếp |
"t" | Thời gian ngắn |
"T" | Thời gian dài |
"u" | Ngày tháng năm có thể sắp xếp, phổ biến (Universal sortable date time). |
"U" | Ngày tháng năm thời gian dài, phổ biến (Universal full date time). |
"Y", "y" | Năm tháng |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class SimpleDateTimeFormatAll
{
public static void Main(string[] args)
{
char[] formats = {'d', 'D','f','F','g','G','M', 'm','O', 'o','R', 'r','s','t','T','u','U','Y', 'y'};
DateTime aDateTime = new DateTime(2015, 12, 20, 11, 30, 50);
foreach (char ch in formats)
{
Console.WriteLine("\n======" + ch + " ========\n");
// Các định dạng date-time được hỗ trợ.
string[] formattedStrings = aDateTime.GetDateTimeFormats(ch);
foreach (string format in formattedStrings)
{
Console.WriteLine(format);
}
}
Console.ReadLine();
}
}
}
======d ========
12/20/2015
12/20/15
12/20/15
12/20/2015
15/12/20
2015-12-20
20-Dec-15
======D ========
Sunday, December 20, 2015
December 20, 2015
Sunday, 20 December, 2015
20 December, 2015
======f ========
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30 AM
Sunday, December 20, 2015 11:30
Sunday, December 20, 2015 11:30
December 20, 2015 11:30 AM
December 20, 2015 11:30 AM
December 20, 2015 11:30
December 20, 2015 11:30
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30 AM
Sunday, 20 December, 2015 11:30
Sunday, 20 December, 2015 11:30
20 December, 2015 11:30 AM
20 December, 2015 11:30 AM
20 December, 2015 11:30
20 December, 2015 11:30
======F ========
Sunday, December 20, 2015 11:30:50 AM
Sunday, December 20, 2015 11:30:50 AM
Sunday, December 20, 2015 11:30:50
Sunday, December 20, 2015 11:30:50
December 20, 2015 11:30:50 AM
December 20, 2015 11:30:50 AM
December 20, 2015 11:30:50
December 20, 2015 11:30:50
Sunday, 20 December, 2015 11:30:50 AM
Sunday, 20 December, 2015 11:30:50 AM
Sunday, 20 December, 2015 11:30:50
Sunday, 20 December, 2015 11:30:50
20 December, 2015 11:30:50 AM
20 December, 2015 11:30:50 AM
20 December, 2015 11:30:50
20 December, 2015 11:30:50
======g ========
12/20/2015 11:30 AM
12/20/2015 11:30 AM
12/20/2015 11:30
12/20/2015 11:30
12/20/15 11:30 AM
12/20/15 11:30 AM
12/20/15 11:30
12/20/15 11:30
12/20/15 11:30 AM
12/20/15 11:30 AM
12/20/15 11:30
12/20/15 11:30
12/20/2015 11:30 AM
12/20/2015 11:30 AM
12/20/2015 11:30
12/20/2015 11:30
15/12/20 11:30 AM
15/12/20 11:30 AM
15/12/20 11:30
15/12/20 11:30
2015-12-20 11:30 AM
2015-12-20 11:30 AM
2015-12-20 11:30
2015-12-20 11:30
20-Dec-15 11:30 AM
20-Dec-15 11:30 AM
20-Dec-15 11:30
20-Dec-15 11:30
======G ========
12/20/2015 11:30:50 AM
12/20/2015 11:30:50 AM
12/20/2015 11:30:50
12/20/2015 11:30:50
12/20/15 11:30:50 AM
12/20/15 11:30:50 AM
12/20/15 11:30:50
12/20/15 11:30:50
12/20/15 11:30:50 AM
12/20/15 11:30:50 AM
12/20/15 11:30:50
12/20/15 11:30:50
12/20/2015 11:30:50 AM
12/20/2015 11:30:50 AM
12/20/2015 11:30:50
12/20/2015 11:30:50
15/12/20 11:30:50 AM
15/12/20 11:30:50 AM
15/12/20 11:30:50
15/12/20 11:30:50
2015-12-20 11:30:50 AM
2015-12-20 11:30:50 AM
2015-12-20 11:30:50
2015-12-20 11:30:50
20-Dec-15 11:30:50 AM
20-Dec-15 11:30:50 AM
20-Dec-15 11:30:50
20-Dec-15 11:30:50
======M ========
December 20
======m ========
December 20
======O ========
2015-12-20T11:30:50.0000000
======o ========
2015-12-20T11:30:50.0000000
======R ========
Sun, 20 Dec 2015 11:30:50 GMT
======r ========
Sun, 20 Dec 2015 11:30:50 GMT
======s ========
2015-12-20T11:30:50
======t ========
11:30 AM
11:30 AM
11:30
11:30
======T ========
11:30:50 AM
11:30:50 AM
11:30:50
11:30:50
======u ========
2015-12-20 11:30:50Z
======U ========
Sunday, December 20, 2015 4:30:50 AM
Sunday, December 20, 2015 04:30:50 AM
Sunday, December 20, 2015 4:30:50
Sunday, December 20, 2015 04:30:50
December 20, 2015 4:30:50 AM
December 20, 2015 04:30:50 AM
December 20, 2015 4:30:50
December 20, 2015 04:30:50
Sunday, 20 December, 2015 4:30:50 AM
Sunday, 20 December, 2015 04:30:50 AM
Sunday, 20 December, 2015 4:30:50
Sunday, 20 December, 2015 04:30:50
20 December, 2015 4:30:50 AM
20 December, 2015 04:30:50 AM
20 December, 2015 4:30:50
20 December, 2015 04:30:50
======Y ========
December, 2015
======y ========
December, 2015
// Chuỗi thời gian bạn thấy trên Http Header
string httpTime = "Fri, 21 Feb 2011 03:11:31 GMT";
// Chuỗi thời gian thấy trên w3.org
string w3Time = "2016/05/26 14:37:11";
// Chuỗi thời gian thấy trên nytimes.com
string nyTime = "Thursday, February 26, 2012";
// Chuỗi thời gian tiêu chuẩn ISO 8601.
string isoTime = "2016-02-10";
// Chuỗi thời gian khi xem ngày giờ tạo/sửa của một file trên Windows.
string windowsTime = "11/21/2015 11:35 PM";
// Chuỗi thời gian trên "Windows Date and Time panel"
string windowsPanelTime = "11:07:03 PM";
7. Tùy biến định dạng DateTime
string format = "dd/MM/yyyy HH:mm:ss";
DateTime now = DateTime.Now;
// ==> 18/03/2016 23:49:39
string s = now.ToString(format);
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class CustomDateTimeFormatExample
{
public static void Main(string[] args)
{
// Một định dạng ngày tháng và thời gian.
string format = "dd/MM/yyyy HH:mm:ss";
DateTime now = DateTime.Now;
string s = now.ToString(format);
Console.WriteLine("Now: " + s);
Console.Read();
}
}
}
public static DateTime Parse(string s)
public static DateTime Parse(string s, IFormatProvider provider)
public static DateTime Parse(string s, IFormatProvider provider, DateTimeStyles styles)
public static bool TryParseExact(string s, string format,
IFormatProvider provider, DateTimeStyles style,
out DateTime result)
public static bool TryParseExact(string s, string[] formats,
IFormatProvider provider, DateTimeStyles style,
out DateTime result)
Phương thức | Ví dụ |
static DateTime Parse(string) | // Xem thêm phần định dạng chuẩn DateTime. string httpHeaderTime = "Fri, 21 Feb 2011 03:11:31 GMT"; DateTime.Parse(httpHeaderTime); |
static DateTime ParseExact(
string s, string format, IFormatProvider provider ) | string dateString = "20160319 09:57";
DateTime.ParseExact(dateString ,"yyyyMMdd HH:mm",null); |
static bool TryParseExact(
string s, string format, IFormatProvider provider, DateTimeStyles style, out DateTime result ) | Phương thức này rất giống với ParseExact, tuy nhiên nó trả về kiểu boolean, true nếu chuỗi thời gian là phân tích được, ngược lại trả về false.
(Xem thêm ví dụ dưới đây). |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DateTimeTutorial
{
class ParseDateStringExample
{
public static void Main(string[] args)
{
string dateString = "20160319 09:57";
// Sử dụng ParseExact để phân tích một String thành DateTime.
DateTime dateTime = DateTime.ParseExact(dateString, "yyyyMMdd HH:mm", null);
Console.WriteLine("Input dateString: " + dateString);
Console.WriteLine("Parse Results: " + dateTime.ToString("dd-MM-yyyy HH:mm:ss"));
// Một chuỗi datetime có khoảng trắng phía trước.
dateString = " 20110319 11:57";
// Sử dụng phương thức TryParseExact.
// Phương thức này trả về true nếu chuỗi 'dateString' có thể phân tích được.
// Và trả về giá trị cho tham số 'out dateTime'.
bool successful = DateTime.TryParseExact(dateString, "yyyyMMdd HH:mm", null,
System.Globalization.DateTimeStyles.AllowLeadingWhite,
out dateTime);
Console.WriteLine("\n------------------------\n");
Console.WriteLine("Input dateString: " + dateString);
Console.WriteLine("Can Parse? :" + successful);
if (successful)
{
Console.WriteLine("Parse Results: " + dateTime.ToString("dd-MM-yyyy HH:mm:ss"));
}
Console.Read();
}
}
}
Input dateString: 20160319 09:57
Parse Results: 19-0302016 09:57:00
------------------------
Input dateString: 20110319 11:57
Can Parse? :True
Parse Results: 19-03-2011 11:57:00
Các hướng dẫn lập trình C#
- Thừa kế và đa hình trong C#
- Bắt đầu với C# cần những gì?
- Học nhanh C# cho người mới bắt đầu
- Cài đặt Visual Studio 2013 trên Windows
- Abstract class và Interface trong C#
- Cài đặt Visual Studio 2015 trên Windows
- Nén và giải nén trong C#
- Hướng dẫn lập trình đa luồng trong C#
- Hướng dẫn và ví dụ C# Delegate và Event
- Cài đặt AnkhSVN trên Windows
- Lập trình C# theo nhóm sử dụng Visual Studio và SVN
- Cài đặt .Net Framework
- Access Modifier trong C#
- Hướng dẫn và ví dụ C# String và StringBuilder
- Hướng dẫn và ví dụ C# Property
- Hướng dẫn và ví dụ C# Enum
- Hướng dẫn và ví dụ C# Structure
- Hướng dẫn và ví dụ C# Generics
- Hướng dẫn xử lý ngoại lệ trong C#
- Hướng dẫn và ví dụ Date Time trong C#
- Thao tác với tập tin và thư mục trong C#
- Hướng dẫn sử dụng Stream - luồng vào ra nhị phân trong C#
- Hướng dẫn sử dụng biểu thức chính quy trong C#
- Kết nối cơ sở dữ liệu SQL Server trong C#
- Làm việc với cơ sở dữ liệu SQL Server trong C#
- Kết nối cơ sở dữ liệu MySQL trong C#
- Làm việc với cơ sở dữ liệu MySQL trong C#
- Kết nối cơ sở dữ liệu Oracle trong C# không cần Oracle Client
- Làm việc với cơ sở dữ liệu Oracle trong C#