Hướng dẫn và ví dụ Java SWT Label
1. SWT Label
SWT Label là một thành phần giao diện (UI Component), nó có thể hiện thị text, Image. Nhưng không thể hiển thị cả hai. Để hiển thị đồng thời cả Text và Image bạn có thể sử dụng CLabel, một class con của Label.
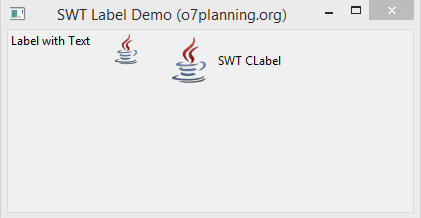
2. Ví dụ Label
Đây là ví dụ đơn giản với Label hiển thị nội dung text, đôi khi class Label cũng sử dụng để tạo ra các đường ngăn cách (Separator) nằm ngang hoặc thẳng đứng.
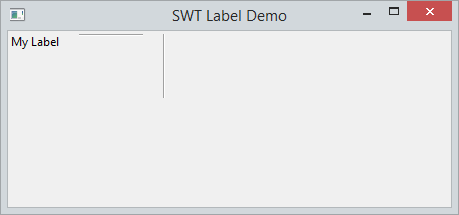
LabelDemo.java
package org.o7planning.swt.label;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class LabelDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
RowLayout layout = new RowLayout();
layout.spacing = 20;
shell.setLayout(layout);
// Tạo một Label với Text
Label label1 = new Label(shell, SWT.NONE);
label1.setText("My Label");
// Tạo một Label là một đường nằm ngang (Horizontal Separator)
Label hSeparator = new Label(shell, SWT.SEPARATOR | SWT.HORIZONTAL);
// Tạo một Label là một đường nằm ngang (Vertical Separator)
Label vSeparator = new Label(shell, SWT.SEPARATOR | SWT.VERTICAL);
shell.setText("SWT Label Demo");
shell.setSize(450, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
3. Label với Icon
Chú ý rằng class Label có thể hiển thị Text hoặc Icon, nếu bạn muốn hiển thị cả hai Text & Icon, bạn nên sử dụng class CLabel.
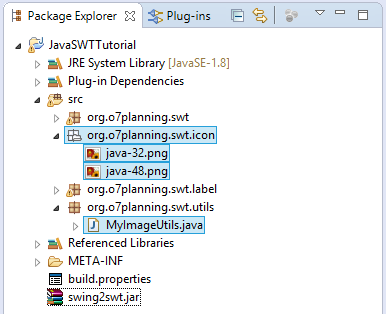
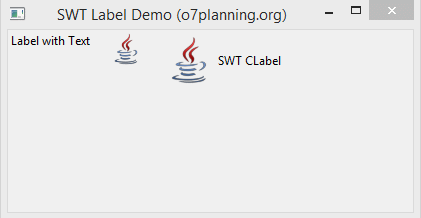
MyImageUtils.java
package org.o7planning.swt.utils;
import java.io.IOException;
import java.io.InputStream;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.widgets.Display;
public class MyImageUtils {
// resourcePath: "/org/o7planning/swt/icon/java-32.png"
public static Image getImage(Display display, String resourcePath) {
InputStream input = null;
try {
// /org/o7planning/swt/icon/java-32.png
input = MyImageUtils.class.getResourceAsStream(resourcePath);
Image image = new Image(display, input);
return image;
} finally {
closeQuietly(input);
}
}
private static void closeQuietly(InputStream is) {
try {
if (is != null) {
is.close();
}
} catch (IOException e) {
}
}
}
Xem ví dụ đầy đủ:
LabelIconDemo.java
package org.o7planning.swt.label;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.CLabel;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.o7planning.swt.utils.MyImageUtils;
public class LabelIconDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
RowLayout layout = new RowLayout();
layout.spacing = 20;
shell.setLayout(layout);
Image image = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/java-48.png");
Image image2 = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/java-32.png");
// Tạo một Label với Text
Label label1 = new Label(shell, SWT.NONE);
label1.setText("Label with Text");
// Tạo một Label với Icon
Label label2 = new Label(shell, SWT.NONE);
label2.setImage(image2);
// Tạo một CLabel với Text & Icon
CLabel cLabel = new CLabel(shell, SWT.NONE);
cLabel.setImage(image);
cLabel.setText("SWT CLabel");
shell.setText("SWT Label Demo (o7planning.org)");
shell.setSize(450, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
4. Label với Font và Color
Bạn có thể xem thêm tài liệu SWT Font & Color tại:
- swt font and color
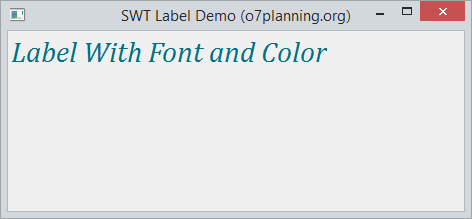
Font
Bạn có thể sét đặt Font (Bao gồm tên Font và kích thước) cho Label thông qua phương thức setFont.
// Tạo một đối tượng Font mới
Font font = new Font(display, "Cambria", 22, SWT.ITALIC);
label.setFont(font);
Color
Sử dụng phương thức setForeground để sét đặt màu chữ cho Label.
Color color = new Color(display, 0, 114, 135);
// Set màu chữ.
label.setForeground(color);
Xem ví dụ đầy đủ:
LabelFullDemo.java
package org.o7planning.swt.label;
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class LabelFontColorDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
RowLayout layout = new RowLayout();
layout.spacing = 20;
shell.setLayout(layout);
// ------ Label -----
Label label = new Label(shell, SWT.NONE);
label.setText("Label With Font and Color");
label.setFont(new Font(display, "Cambria", 22, SWT.ITALIC));
Color color = new Color(display, 0, 114, 135);
// Set màu chữ.
label.setForeground(color);
shell.setText("SWT Label Demo (o7planning.org)");
shell.setSize(450, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Các hướng dẫn lập trình Java SWT
- Hướng dẫn và ví dụ Java SWT FillLayout
- Hướng dẫn và ví dụ Java SWT RowLayout
- Hướng dẫn và ví dụ Java SWT SashForm
- Hướng dẫn và ví dụ Java SWT Label
- Hướng dẫn và ví dụ Java SWT Button
- Hướng dẫn và ví dụ Java SWT Toggle Button
- Hướng dẫn và ví dụ Java SWT Radio Button
- Hướng dẫn và ví dụ Java SWT Text
- Hướng dẫn và ví dụ Java SWT Password Field
- Hướng dẫn và ví dụ Java SWT Link
- Lập trình ứng dụng Java Desktop sử dụng SWT
- Hướng dẫn và ví dụ Java SWT Combo
- Hướng dẫn và ví dụ Java SWT Spinner
- Hướng dẫn và ví dụ Java SWT Slider
- Hướng dẫn và ví dụ Java SWT Scale
- Hướng dẫn và ví dụ Java SWT ProgressBar
- Hướng dẫn và ví dụ Java SWT TabFolder và CTabFolder
- Hướng dẫn và ví dụ Java SWT List
Show More