Hướng dẫn và ví dụ Java SWT Text
1. SWT Text
Class Text là một control chấp nhận và hiển thị đầu vào văn bản. Nó cung cấp khả năng tiếp nhận đầu vào văn bản từ một người sử dụng.
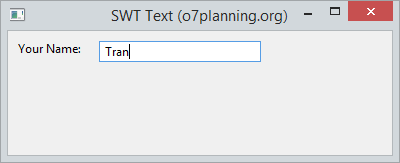
Class Text có thể sử dụng để tạo ra một đầu vào Text, cho phép người dùng nhập trên một dòng. Nó cũng có thể tạo ra một đầu vào text cho phép nhập trên nhiều dòng, hoặc tạo ra một trường mật khẩu (Password field).
Xem thêm trường mật khẩu (Password Field) trong SWT:
// Style ....
int style = SWT.BORDER;
int style = SWT.BORDER | SWT.PASSWORD;
Text text = new Text(parent, style);
Các kiểu dáng (Style) có thể được áp dụng cho Text:
Style | Mô tả |
SWT.BORDER | Hiển thị viền (border). |
SWT.MULTI | Cho phép nhập nội dung trên nhiều dòng. |
SWT.PASSWORD | Cho phép người dùng nhập mật khẩu. |
SWT.V_SCROLL | Hiển thị thanh cuộn thẳng đứng, thường sử dụng cùng với SWT.MULTI. |
SWT.H_SCROLL | Hiển thị thanh cuộn nằm ngang, thường sử dụng với SWT.MULTI. |
SWT.WRAP | Tự động cuộn (wrap) xuống dòng nếu đoạn văn bản quá dài. |
SWT.READ_ONLY | Không cho phép hiệu chỉnh, nó tương đương với gọi phương thức text.setEditable(false); |
SWT.LEFT | Căn lề trái cho text |
SWT.RIGHT | Căn lề phải cho text |
SWT.CENTER | Căn lề giữa cho text |
Xem một vài phương thức hữu ích bạn có thể sử dụng với Text.
text.setEnabled(enabled);
text.setFont(font);
text.setForeground(color);
text.setEditable(editable);
text.setTextLimit(limit);
text.setToolTipText(string);
text.setTextDirection(textDirection);
text.addSegmentListener(listener);
2. Ví dụ với SWT Text
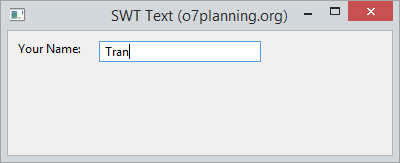
TextDemo.java
package org.o7planning.swt.text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class TextDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Text (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Label
Label label = new Label(shell, SWT.NONE);
label.setText("Your Name: ");
// Text
Text text = new Text(shell, SWT.BORDER);
RowData layoutData = new RowData();
layoutData.width = 150;
text.setLayoutData(layoutData);
text.setText("Tran");
shell.setSize(400, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
3. SWT Text và Styles
Ví dụ dưới đây tạo các SWT Text khác nhau với các style SWT.BORDER, SWT.PASSWORD, SWT.MULTI, SWT.WRAP, SWT.READ_ONLY, ...
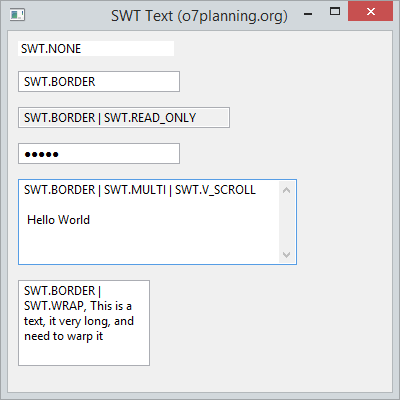
TextStylesDemo.java
package org.o7planning.swt.text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class TextStylesDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Text (o7planning.org)");
RowLayout rowLayout = new RowLayout(SWT.VERTICAL);
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Text không có viền (border)
Text text1 = new Text(shell, SWT.NONE);
text1.setText("SWT.NONE");
text1.setLayoutData(new RowData(150, SWT.DEFAULT));
// Text có viền (border)
Text text2 = new Text(shell, SWT.BORDER);
text2.setText("SWT.BORDER");
text2.setLayoutData(new RowData(150, SWT.DEFAULT));
// Text có viền và không cho phép sửa.
Text text3 = new Text(shell, SWT.BORDER | SWT.READ_ONLY);
text3.setText("SWT.BORDER | SWT.READ_ONLY");
text3.setLayoutData(new RowData(200, SWT.DEFAULT));
// Password field.
Text text4 = new Text(shell, SWT.BORDER | SWT.PASSWORD);
text4.setText("12345");
text4.setLayoutData(new RowData(150, SWT.DEFAULT));
// Text cho phép nhiều dòng và thanh cuộn thẳng đứng.
Text text5 = new Text(shell, SWT.BORDER | SWT.MULTI | SWT.V_SCROLL);
text5.setText("SWT.BORDER | SWT.MULTI | SWT.V_SCROLL \n\n Hello World");
text5.setLayoutData(new RowData(250, 80));
// Wrap
Text text6 = new Text(shell, SWT.BORDER | SWT.WRAP);
text6.setLayoutData(new RowData(120, 80));
text6.setText("SWT.BORDER | SWT.WRAP, This is a text, it very long, and need to warp it");
shell.setSize(400, 400);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
4. Các phương thức hữu ích
Ví dụ dưới đây minh họa sử dụng các phương thức clear(),copy(), paste(), cut(), chúng là các phương thức hữu ích của TextField.
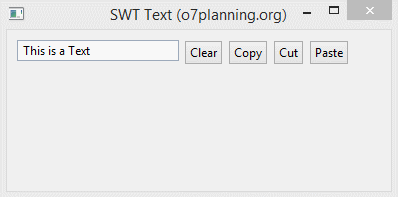
TextDemo2.java
package org.o7planning.swt.text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class TextDemo2 {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Text (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 5;
shell.setLayout(rowLayout);
// Text
Text text = new Text(shell, SWT.BORDER);
text.setLayoutData(new RowData(150, SWT.DEFAULT));
text.setText("This is a Text");
// Clear Button
Button clearButton = new Button(shell, SWT.PUSH);
clearButton.setText("Clear");
// Copy Button
Button copyButton = new Button(shell, SWT.PUSH);
copyButton.setText("Copy");
// Cut Button
Button cutButton = new Button(shell, SWT.PUSH);
cutButton.setText("Cut");
// Paste Button
Button pasteButton = new Button(shell, SWT.PUSH);
pasteButton.setText("Paste");
clearButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.setText("");
text.forceFocus();
}
});
copyButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.copy();
text.forceFocus();
}
});
cutButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.cut();
text.forceFocus();
}
});
pasteButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text.paste();
text.forceFocus();
}
});
shell.setSize(400, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Các hướng dẫn lập trình Java SWT
- Hướng dẫn và ví dụ Java SWT FillLayout
- Hướng dẫn và ví dụ Java SWT RowLayout
- Hướng dẫn và ví dụ Java SWT SashForm
- Hướng dẫn và ví dụ Java SWT Label
- Hướng dẫn và ví dụ Java SWT Button
- Hướng dẫn và ví dụ Java SWT Toggle Button
- Hướng dẫn và ví dụ Java SWT Radio Button
- Hướng dẫn và ví dụ Java SWT Text
- Hướng dẫn và ví dụ Java SWT Password Field
- Hướng dẫn và ví dụ Java SWT Link
- Lập trình ứng dụng Java Desktop sử dụng SWT
- Hướng dẫn và ví dụ Java SWT Combo
- Hướng dẫn và ví dụ Java SWT Spinner
- Hướng dẫn và ví dụ Java SWT Slider
- Hướng dẫn và ví dụ Java SWT Scale
- Hướng dẫn và ví dụ Java SWT ProgressBar
- Hướng dẫn và ví dụ Java SWT TabFolder và CTabFolder
- Hướng dẫn và ví dụ Java SWT List
Show More