Hướng dẫn và ví dụ Java OutputStream
1. OutputStream
OutputStream là một lớp nằm trong package java.io, nó là một lớp cơ sở đại diện cho một dòng chảy của các bytes (stream of bytes) để ghi các bytes vào một mục tiêu nào đó, chẳng hạn file.
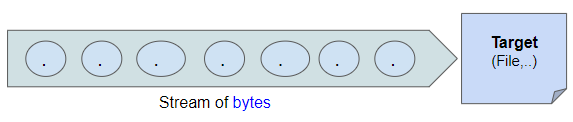
public abstract class OutputStream implements Closeable, Flushable
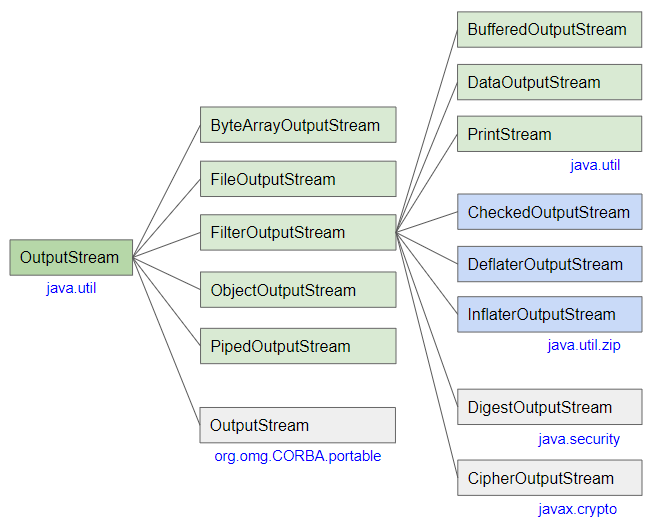
- ByteArrayOutputStream
- FileOutputStream
- FilterOutputStream
- ObjectOutputStream
- PipedOutputStream
- BufferedOutputStream
- DataOutputStream
- PrintStream
- CheckedOutputStream
- CipherOutputStream
- DeflaterOutputStream
- DigestOutputStream
- InflaterOutputStream
Về cơ bản bạn không thể sử dụng lớp OutputStream một cách trực tiếp vì nó là một lớp trừu tượng, tuy nhiên trong trường hợp cụ thể bạn có thể sử dụng một trong các lớp con của nó.
OutputStream methods
public static OutputStream nullOutputStream()
public abstract void write(int b) throws IOException
public void write(byte b[]) throws IOException
public void write(byte b[], int off, int len) throws IOException
public void flush() throws IOException
public void close() throws IOException
2. write(int)
public abstract void write(int b) throws IOException
Ghi một byte vào OutputStream. Tham số b là mã không dấu (unsigned code) của byte, nó là một số nguyên nằm trong khoảng 0 đến 255. Nếu giá trị của b nằm ngoài phạm vi nói trên nó sẽ bị bỏ qua.
OutputStream_write_ex1.java
package org.o7planning.outputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_write_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileOutputStream
OutputStream os = new FileOutputStream(file);
// 'J'
os.write((int)'J'); // 74
// 'P'
os.write('P'); // 80 (Automatically converted to int)
// '日' = 3 bytes UTF-8: [230, 151, 165]
os.write(230);
os.write(151);
os.write(165);
// '本' = 3 bytes UTF-8: [230, 156, 172]
os.write(230);
os.write(156);
os.write(172);
// '-'
os.write('-'); // 45 (Automatically converted to int)
// '八' = 3 bytes UTF-8: [229, 133, 171]
os.write(229);
os.write(133);
os.write(171);
// '洲' = 3 bytes UTF-8: [230, 180, 178]
os.write(230);
os.write(180);
os.write(178);
// Close
os.close();
}
}
Output:
out-file.txt
JP日本-八洲
Các file UTF-8 sử dụng 1, 2, 3 hoặc 4 bytes để lưu trữ một ký tự. Hình ảnh dưới đây cho thấy các bytes trên file vừa được tạo ra.
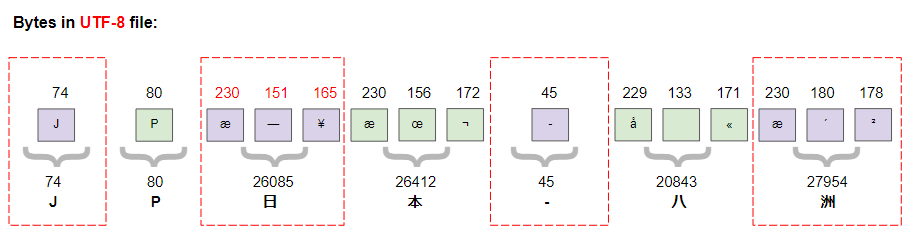
3. write(byte[])
public void write(byte[] b) throws IOException
Ghi một mảng byte vào OutputStream.
OutputStream_write_ex2.java
package org.o7planning.outputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_write_ex2 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileOutputStream
OutputStream writer = new FileOutputStream(file);
byte[] bytes = new byte[] { 'J', 'P', //
(byte)230, (byte)151, (byte)165, // '日' = 3 bytes UTF-8: [230, 151, 165]
(byte)230, (byte)156, (byte)172, // '本' = 3 bytes UTF-8: [230, 156, 172]
'-', //
(byte)229, (byte)133, (byte)171, // '八' = 3 bytes UTF-8: [229, 133, 171]
(byte)230, (byte)180, (byte)178, // '洲' = 3 bytes UTF-8: [230, 180, 178]
};
writer.write(bytes);
// Close
writer.close();
}
}
Output:
out-file.txt
JP日本-八洲
4. write(byte[], int, int)
public void write(byte b[], int offset, int len) throws IOException
Ghi một phần của một mảng byte vào OutputStream. Ghi các bytes từ chỉ số offset đến chỉ số offset+len.
OutputStream_write_ex3.java
package org.o7planning.outputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_write_ex3 {
public static void main(String[] args) throws IOException {
// ByteArrayOutputStream is a subclass of OutputStream.
OutputStream outputStream = new ByteArrayOutputStream();
byte[] bytes = new byte[] {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9'};
outputStream.write(bytes, 2, 5); // '2', ...'6'
String s = outputStream.toString();
System.out.println(s);
outputStream.close();
}
}
Output:
23456
5. close()
public void close() throws IOException
Phương thức close() được sử dụng để đóng stream này, phương thức flush() sẽ được gọi đầu tiên. Khi stream đã bị đóng, các lệnh gọi thêm write() hoặc flush() sẽ khiến IOException được ném ra. Đóng stream đã đóng trước đó không có tác dụng.
public interface Closeable extends AutoCloseable
Lớp Writer thi hành interface Closeable. Nếu bạn viết code theo quy tắc của AutoCloseable thì hệ thống sẽ tự đóng stream cho bạn mà không cần phải gọi trực tiếp phương thức close().
OutputStream_close_ex1.java
package org.o7planning.outputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_close_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
try (OutputStream writer = new FileOutputStream(file)) {
writer.write((int) '1');
writer.write('2');
writer.write((int) '3');
}
}
}
- Hướng dẫn và ví dụ Java Closeable
6. flush()
public void flush() throws IOException
Dữ liệu mà bạn ghi vào OutputStream đôi khi được lưu trữ một cách tạm thời trên bộ đệm, phương thức flush() được sử dụng để xả (flush) dữ liệu vào mục tiêu.
Bạn có đọc bài viết về BufferedOutputStream dưới đây để hiểu hơn về phương thức flush().
Nếu đích dự kiến của OutputStream này là một phần trừu tượng được cung cấp dưới điều hành, chẳng hạn như một tệp, thì việc xả stream chỉ đảm bảo những bytes đã ghi trước đó vào stream được chuyển tới hệ điều hành để ghi; nó không đảm bảo rằng chúng thực sự được ghi vào một thiết bị vật lý như ổ đĩa.
Các hướng dẫn Java IO
- Hướng dẫn và ví dụ Java CharArrayWriter
- Hướng dẫn và ví dụ Java FilterReader
- Hướng dẫn và ví dụ Java FilterWriter
- Hướng dẫn và ví dụ Java PrintStream
- Hướng dẫn và ví dụ Java BufferedReader
- Hướng dẫn và ví dụ Java BufferedWriter
- Hướng dẫn và ví dụ Java StringReader
- Hướng dẫn và ví dụ Java StringWriter
- Hướng dẫn và ví dụ Java PipedReader
- Hướng dẫn và ví dụ Java LineNumberReader
- Hướng dẫn và ví dụ Java PushbackReader
- Hướng dẫn và ví dụ Java PrintWriter
- Hướng dẫn sử dụng luồng vào ra nhị phân trong Java
- Hướng dẫn sử dụng luồng vào ra ký tự trong Java
- Hướng dẫn và ví dụ Java BufferedOutputStream
- Hướng dẫn và ví dụ Java ByteArrayOutputStream
- Hướng dẫn và ví dụ Java DataOutputStream
- Hướng dẫn và ví dụ Java PipedInputStream
- Hướng dẫn và ví dụ Java OutputStream
- Hướng dẫn và ví dụ Java ObjectOutputStream
- Hướng dẫn và ví dụ Java PushbackInputStream
- Hướng dẫn và ví dụ Java SequenceInputStream
- Hướng dẫn và ví dụ Java BufferedInputStream
- Hướng dẫn và ví dụ Java Reader
- Hướng dẫn và ví dụ Java Writer
- Hướng dẫn và ví dụ Java FileReader
- Hướng dẫn và ví dụ Java FileWriter
- Hướng dẫn và ví dụ Java CharArrayReader
- Hướng dẫn và ví dụ Java ByteArrayInputStream
- Hướng dẫn và ví dụ Java DataInputStream
- Hướng dẫn và ví dụ Java ObjectInputStream
- Hướng dẫn và ví dụ Java InputStreamReader
- Hướng dẫn và ví dụ Java OutputStreamWriter
- Hướng dẫn và ví dụ Java InputStream
- Hướng dẫn và ví dụ Java FileInputStream
Show More
- Hướng dẫn lập trình Java Servlet/JSP
- Các hướng dẫn Java New IO
- Các hướng dẫn Spring Cloud
- Các hướng dẫn Java Oracle ADF
- Các hướng dẫn Java Collections Framework
- Java cơ bản
- Các hướng dẫn Java Date Time
- Các thư viện mã nguồn mở Java
- Các hướng dẫn Java Web Services
- Các hướng dẫn Struts2 Framework
- Các hướng dẫn Spring Boot