Hướng dẫn và ví dụ Java DataInputStream
1. DataInputStream
DataInputStream được sử dụng để đọc các dữ liệu nguyên thuỷ từ một nguồn dữ liệu, đặc biệt các nguồn dữ liệu được ghi bởi DataOutputStream.
public class DataInputStream extends FilterInputStream implements DataInput
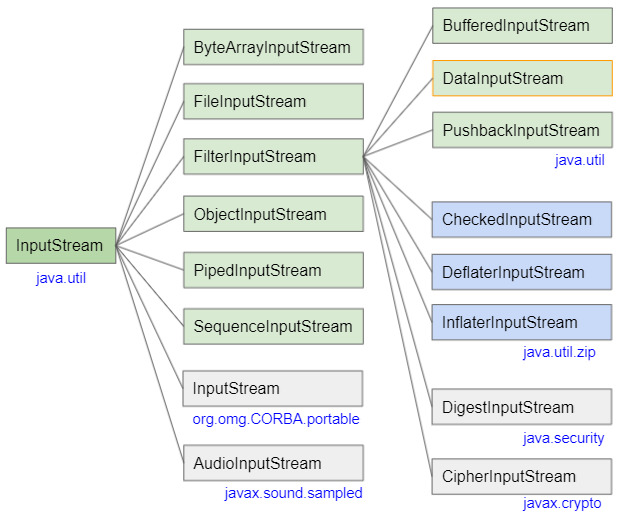
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- BufferedInputStream
- InputStream
- PushbackInputStream
- ObjectInputStream
- PipedInputStream
- SequenceInputStream
- AudioInputStream
- CheckedInputStream
- DeflaterInputStream
- InflaterInputStream
- CipherInputStream
- DigestInputStream
3. Methods
public final boolean readBoolean() throws IOException
public final byte readByte() throws IOException
public final int readUnsignedByte() throws IOException
public final short readShort() throws IOException
public final int readUnsignedShort() throws IOException
public final char readChar() throws IOException
public final int readInt() throws IOException
public final long readLong() throws IOException
public final float readFloat() throws IOException
public final double readDouble() throws IOException
public final String readUTF() throws IOException
public static final String readUTF(DataInput in) throws IOException
public final void readFully(byte[] b) throws IOException
public final void readFully(byte[] b, int off, int len) throws IOException
@Deprecated
public String readLine() throws IOException
Các phương thức khác thừa kế từ các lớp cha:
public int read() throws IOException
public int read(byte[] b) throws IOException
public int read(byte[] b, int off, int len) throws IOException
public byte[] readAllBytes() throws IOException
public byte[] readNBytes(int len) throws IOException
public int readNBytes(byte[] b, int off, int len) throws IOException
public long skip(long n) throws IOException
public int available() throws IOException
public void close() throws IOException
public synchronized void mark(int readlimit)
public synchronized void reset() throws IOException
public boolean markSupported()
public long transferTo(OutputStream out) throws IOException
public static InputStream nullInputStream()
4. Examples
DataInputStream thường được sử dụng để đọc các nguồn dữ liệu đã được ghi bởi DataOutputStream. Trong ví dụ này chúng ta sử dụng DataOutputStream ghi một bảng dữ liệu có cấu trúc giống như Excel vào 1 file, sau đó sử dụng DataInputStream để đọc file này.
OrderDate | Finished | Item | Units | UnitCost | Total |
2020-01-06 | Pencil | 95 | 1.99 | 189.05 | |
2020-01-23 | Binder | 50 | 19.99 | 999.50 | |
2020-02-09 | Pencil | 36 | 4.99 | 179.64 | |
2020-02-26 | Pen | 27 | 19.99 | 539.73 | |
2020-03-15 | Pencil | 56 | 2.99 | 167.44 |
Đầu tiên, chúng ta viết lớp Order mô phỏng dữ liệu của một dòng của bảng:
Order.java
package org.o7planning.datainputstream.ex;
import java.time.LocalDate;
public class Order {
private LocalDate orderDate;
private boolean finished;
private String item;
private int units;
private float unitCost;
private float total;
public Order(LocalDate orderDate, boolean finished, //
String item, int units, float unitCost, float total) {
this.orderDate = orderDate;
this.finished = finished;
this.item = item;
this.units = units;
this.unitCost = unitCost;
this.total = total;
}
public LocalDate getOrderDate() {
return orderDate;
}
public boolean isFinished() {
return finished;
}
public String getItem() {
return item;
}
public int getUnits() {
return units;
}
public float getUnitCost() {
return unitCost;
}
public float getTotal() {
return total;
}
}
Sử dụng DataOutputStream để ghi bảng dữ liệu trên vào một file:
WriteDataFile_example1.java
package org.o7planning.datainputstream.ex;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.time.LocalDate;
public class WriteDataFile_example1 {
// Windows: C:/somepath/data-file.txt
private static final String filePath = "/Volumes/Data/test/data-file.txt";
public static void main(String[] args) throws IOException {
Order[] orders = new Order[] { //
new Order(LocalDate.of(2020, 1, 6), true, "Pencil", 95, 1.99f, 189.05f),
new Order(LocalDate.of(2020, 1, 23), false, "Binder", 50, 19.99f, 999.50f),
new Order(LocalDate.of(2020, 2, 9), true, "Pencil", 36, 4.99f, 179.64f),
new Order(LocalDate.of(2020, 2, 26), false, "Pen", 27, 19.99f, 539.73f),
new Order(LocalDate.of(2020, 3, 15), true, "Pencil", 56, 2.99f, 167.44f) //
};
File outFile = new File(filePath);
outFile.getParentFile().mkdirs();
OutputStream outputStream = new FileOutputStream(outFile);
DataOutputStream dataOutputStream = new DataOutputStream(outputStream);
for (Order order : orders) {
dataOutputStream.writeUTF(order.getOrderDate().toString());
dataOutputStream.writeBoolean(order.isFinished());
dataOutputStream.writeUTF(order.getItem());
dataOutputStream.writeInt(order.getUnits());
dataOutputStream.writeFloat(order.getUnitCost());
dataOutputStream.writeFloat(order.getTotal());
}
dataOutputStream.close();
}
}
Sau khi chạy ví dụ trên chúng ta có một file dữ liệu với nội dung khá khó hiểu:
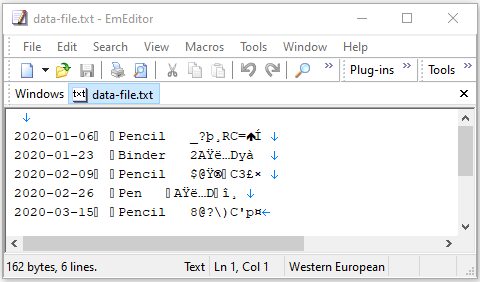
Tiếp theo, chúng ta sử dụng DataInputStream để đọc file nói trên.
ReadDataFile_example1.java
package org.o7planning.datainputstream.ex;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class ReadDataFile_example1 {
// Windows: C:/somepath/data-file.txt
private static final String filePath = "/Volumes/Data/test/data-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(filePath);
InputStream inputStream = new FileInputStream(file);
DataInputStream dataInputStream = new DataInputStream(inputStream);
int row = 0;
System.out.printf("|%3s | %-10s | %10s | %-15s | %8s| %10s | %10s |%n", //
"No", "Order Date", "Finished?", "Item", "Units", "Unit Cost", "Total");
System.out.printf("|%3s | %-10s | %10s | %-15s | %8s| %10s | %10s |%n", //
"--", "---------", "----------", "----------", "------", "---------", "---------");
while (dataInputStream.available() > 0) {
row++;
String orderDate = dataInputStream.readUTF();
boolean finished = dataInputStream.readBoolean();
String item = dataInputStream.readUTF();
int units = dataInputStream.readInt();
float unitCost = dataInputStream.readFloat();
float total = dataInputStream.readFloat();
System.out.printf("|%3d | %-10s | %10b | %-15s | %8d| %,10.2f | %,10.2f |%n", //
row, orderDate, finished, item, units, unitCost, total);
}
dataInputStream.close();
}
}
Output:
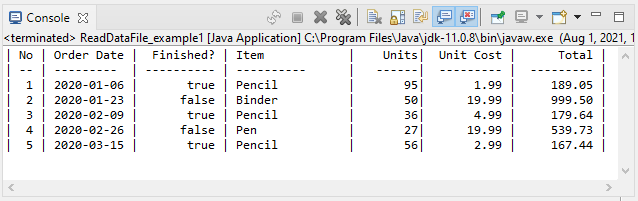
5. readUTF()
Phương thức readUTF() được sử dụng để đọc một chuỗi đã được mã hoá bằng "Modified UTF-8" (UTF-8 đã sửa đổi). Quy tắc đọc đối xứng với quy tắc ghi của phương thức DataOutput.writeUTF(String).
public final String readUTF() throws IOException
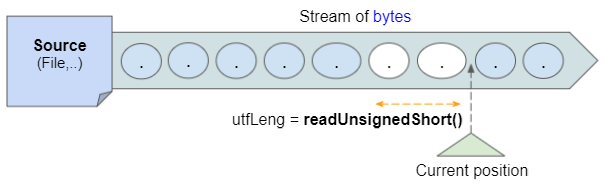
Tại vị trí con trỏ (cursor) trên dòng chảy các bytes (stream of bytes), DataInputStream sẽ đọc 2 bytes tiếp theo bằng phương thức readUnsignedShort() để xác định số bytes đã được sử dụng để lưu trữ chuỗi UTF-8 và nhận được kết quả là utfLeng.
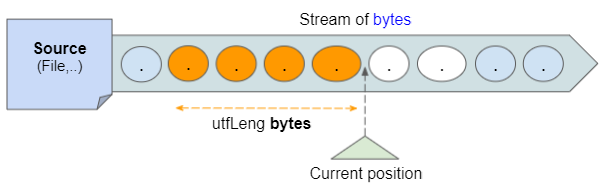
Sau đó, DataInputStream đọc utfLengbytes tiếp theo và chuyển đổi nó thành một chuỗi UTF-8.
- UTF-8
- DataOutputStream
6. readUTF(DataInput in)
Phương thức tĩnh readUTF(DataInput) được sử dụng để đọc một chuỗi "Modified UTF-8" từ một đối tượng DataInput đã chỉ định.
public static final String readUTF(DataInput in) throws IOException
7. readFully(byte[] buffer)
Đọc tối đa có thể các bytes từ DataInputStream này và gán vào mảng buffer đã chỉ định.
public final void readFully(byte[] buffer) throws IOException
Phương thức này sẽ chặn (block) cho đến khi một trong các điều kiện sau xảy ra:
- Đọc được buffer.lengthbytes.
- Đã tiến tới cuối stream.
- Một IOException xẩy ra (Khác với EOFException).
8. readFully(byte[] buffer, int off, int len)
Đọc tối đa có thể các bytes từ DataInputStream này và gán vào mảng buffer đã chỉ định từ chỉ số off đến chỉ số off+len-1.
public final void readFully(byte[] buffer, int off, int len) throws IOException
Phương thức này sẽ chặn (block) cho đến khi một trong các điều kiện sau xảy ra:
- Đọc được lenbytes.
- Đã tiến tới cuối stream.
- Một IOException xẩy ra (Khác với EOFException).
9. readBoolean()
Phương thức readBoolean() đọc một byte từ DataInputStream này, nếu kết quả khác 0 phương thức trả về true, ngược lại trả về false.
public final boolean readBoolean() throws IOException
10. readByte()
Phương thức readByte() đọc một byte từ DataInputStream này, giá trị trả về nằm trong khoảng -128 đến 127.
Nó thích hợp để đọc dữ liệu đã được ghi bởi phương thức DataOutput.writeByte(byte).
public final byte readByte() throws IOException
11. readUnsignedByte()
Phương thức readUnsignedShort() đọc 1 byte từ DataInputStream này và chuyển đổi nó thành một số nguyên 4 bytes không dấu. Giá trị từ 0 đến 255.
public final int readUnsignedByte() throws IOException
12. readShort()
Phương thức readShort() đọc 2 bytes từ DataInputStream này và chuyển đổi nó thành kiểu dữ liệu short.
public final short readShort() throws IOException
Quy tắc chuyển đổi:
return (short)((firstByte << 8) | (secondByte & 0xff));
13. readUnsignedShort()
Phương thức readUnsignedShort() đọc 2 bytes từ DataInputStream này và chuyển đổi nó thành một số nguyên 4 bytes không dấu. Giá trị từ 0 đến 65535.
public final int readUnsignedShort() throws IOException
Quy tắc chuyển đổi:
return (((firstByte & 0xff) << 8) | (secondByte & 0xff))
14. readChar()
Phương thức readChar() đọc 2 bytes từ DataInputStream này, và chuyển đổi nó thành kiểu dữ liệu char.
public final char readChar() throws IOException
Quy tắc chuyển đổi:
return (char)((firstByte << 8) | (secondByte & 0xff));
15. readInt()
Phương thức readInt() đọc 4 bytes từ DataInputStream này, và chuyển đổi nó thành kiểu dữ liệu int.
public final int readInt() throws IOException
Quy tắc chuyển đổi:
return (((firstByte & 0xff) << 24) | ((secondByte & 0xff) << 16)
| ((thirdByte & 0xff) << 8) | (fourthByte & 0xff));
16. readLong()
Phương thức readLong() đọc 8 bytes từ DataInputStream này và chuyển đổi nó thành kiểu dữ liệu long.
public final long readLong() throws IOException
Quy tắc chuyển đổi:
return (((long)(byte1 & 0xff) << 56) |
((long)(byte2 & 0xff) << 48) |
((long)(byte3 & 0xff) << 40) |
((long)(byte4 & 0xff) << 32) |
((long)(byte5 & 0xff) << 24) |
((long)(byte6 & 0xff) << 16) |
((long)(byte7 & 0xff) << 8) |
((long)(byte8 & 0xff)));
Các hướng dẫn Java IO
- Hướng dẫn và ví dụ Java CharArrayWriter
- Hướng dẫn và ví dụ Java FilterReader
- Hướng dẫn và ví dụ Java FilterWriter
- Hướng dẫn và ví dụ Java PrintStream
- Hướng dẫn và ví dụ Java BufferedReader
- Hướng dẫn và ví dụ Java BufferedWriter
- Hướng dẫn và ví dụ Java StringReader
- Hướng dẫn và ví dụ Java StringWriter
- Hướng dẫn và ví dụ Java PipedReader
- Hướng dẫn và ví dụ Java LineNumberReader
- Hướng dẫn và ví dụ Java PushbackReader
- Hướng dẫn và ví dụ Java PrintWriter
- Hướng dẫn sử dụng luồng vào ra nhị phân trong Java
- Hướng dẫn sử dụng luồng vào ra ký tự trong Java
- Hướng dẫn và ví dụ Java BufferedOutputStream
- Hướng dẫn và ví dụ Java ByteArrayOutputStream
- Hướng dẫn và ví dụ Java DataOutputStream
- Hướng dẫn và ví dụ Java PipedInputStream
- Hướng dẫn và ví dụ Java OutputStream
- Hướng dẫn và ví dụ Java ObjectOutputStream
- Hướng dẫn và ví dụ Java PushbackInputStream
- Hướng dẫn và ví dụ Java SequenceInputStream
- Hướng dẫn và ví dụ Java BufferedInputStream
- Hướng dẫn và ví dụ Java Reader
- Hướng dẫn và ví dụ Java Writer
- Hướng dẫn và ví dụ Java FileReader
- Hướng dẫn và ví dụ Java FileWriter
- Hướng dẫn và ví dụ Java CharArrayReader
- Hướng dẫn và ví dụ Java ByteArrayInputStream
- Hướng dẫn và ví dụ Java DataInputStream
- Hướng dẫn và ví dụ Java ObjectInputStream
- Hướng dẫn và ví dụ Java InputStreamReader
- Hướng dẫn và ví dụ Java OutputStreamWriter
- Hướng dẫn và ví dụ Java InputStream
- Hướng dẫn và ví dụ Java FileInputStream
Show More
- Hướng dẫn lập trình Java Servlet/JSP
- Các hướng dẫn Java New IO
- Các hướng dẫn Spring Cloud
- Các hướng dẫn Java Oracle ADF
- Các hướng dẫn Java Collections Framework
- Java cơ bản
- Các hướng dẫn Java Date Time
- Các thư viện mã nguồn mở Java
- Các hướng dẫn Java Web Services
- Các hướng dẫn Struts2 Framework
- Các hướng dẫn Spring Boot