Hướng dẫn và ví dụ Java PrintWriter
1. PrintWriter
PrintWriter là một lớp con của Writer, nó được sử dụng để in các dữ liệu có định dạng vào một OutputStream hoặc một Writer khác mà nó quản lý.
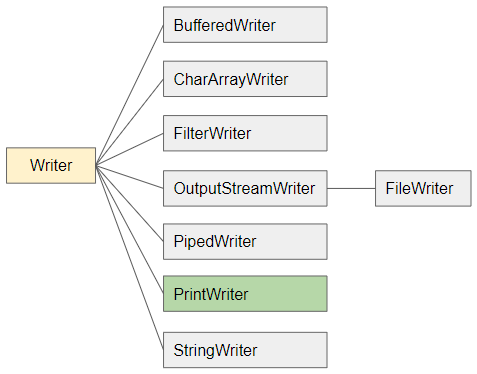
Các đặc điểm của PrintWriter:
Tất cả các phương thức của PrintWriter không ném ra các ngoại lệ I/O, để kiểm tra ngoại lệ có xẩy ra hay không bạn có thể gọi phương thức checkError().
Theo tuỳ chọn, PrintWriter có khả năng tự động xả (flush), nghĩa là phương thức flush() sẽ được gọi ngay sau khi gọi phương thức println(..) hoặc khi in một văn bản bao gồm ký tự '\n'.
2. PrintWriter Constructors
Các constructor để tạo ra đối tượng PrintWriter không tự động xả (flush):
public PrintWriter(File file)
public PrintWriter(File file, String csn)
public PrintWriter(File file, Charset charset)
public PrintWriter(OutputStream out)
public PrintWriter(Writer out)
public PrintWriter(String fileName)
public PrintWriter(String fileName, String csn)
public PrintWriter(String fileName, Charset charset)
Các constructor để tạo ra đối tượng PrintWriter với tuỳ chọn tự động xả (flush):
public PrintWriter(OutputStream out, boolean autoFlush)
public PrintWriter(OutputStream out, boolean autoFlush, Charset charset)
public PrintWriter(Writer out, boolean autoFlush)
Có khá nhiều constructor để khởi tạo một đối tượng PrintWriter, chúng ta xem điều gì xẩy ra khi tạo một PrintWriter trong một vài tình huống cụ thể:
PrintWriter(Writer)
Tạo một đối tượng PrintWriter để in dữ liệu có định dạng vào một Writer khác.
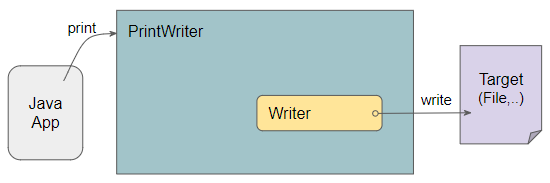
PrintWriter(OutputStream):
Tạo một đối tượng PrintWriter để in dữ liệu có định dạng vào một OutputStream.
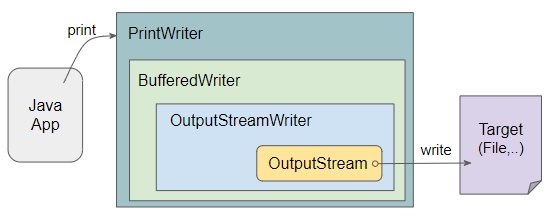
PrintWriter(File file) / PrintWriter(String fileName)
Tạo một đối tượng PrintWriter để in dữ liệu có định dạng vào một file.
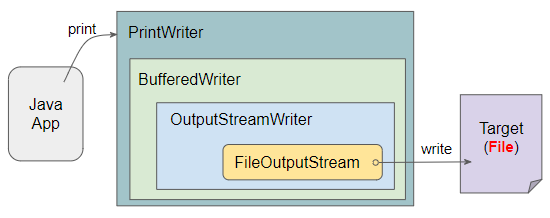
Nếu BufferedWriter tham gia vào cấu trúc của PrintWriter dữ liệu sẽ được ghi một cách tạm thời vào buffer (của BufferedWriter), nó sẽ bị đẩy xuống mục tiêu (Target) khi buffer đầy (Xem các hình ảnh minh hoạ ở trên). Bạn có thể chủ động đẩy dữ liệu vào mục tiêu bằng cách gọi phương thức PrintWriter.flush().
Nếu PrintWriter được tạo ra với tính năng autoFlush được bật, dữ liệu sẽ được đẩy vào mục tiêu mỗi khi phương thức PrintWriter.println(..) hoặc PrintWriter.format(..) được gọi.
3. PrintWriter Methods
Các phương thức của PrintWriter:
Methods of PrintWriter
public PrintWriter append(char c)
public PrintWriter append(CharSequence csq)
public PrintWriter append(CharSequence csq, int start, int end)
public PrintWriter format(String format, Object... args)
public PrintWriter format(Locale l, String format, Object... args)
public PrintWriter printf(String format, Object... args)
public PrintWriter printf(Locale l, String format, Object... args)
public boolean checkError()
protected void clearError()
protected void setError()
public void print(boolean b)
public void print(char c)
public void print(char[] s)
public void print(double d)
public void print(float f)
public void print(int i)
public void print(long l)
public void print(String s)
public void print(Object obj)
public void println()
public void println(boolean x)
public void println(char x)
public void println(char[] x)
public void println(double x)
public void println(float x)
public void println(int x)
public void println(long x)
public void println(String x)
public void println(Object x)
Các phương thức khác ghi đè (override) các phương thức của lớp cha và không ném ra ngoại lệ:
public void write(char[] buf)
public void write(char[] buf, int off, int len)
public void write(int c)
public void write(String s)
public void write(String s, int off, int len)
public void close()
public void flush()
4. Examples
Lấy nội dung dò lỗi (stack trace) từ một Exception:
GetStackTraceEx.java
package org.o7planning.printwriter.ex;
import java.io.PrintWriter;
import java.io.StringWriter;
public class GetStackTraceEx {
public static void main(String[] args) {
try {
int a = 100/0; // Exception occur here
} catch(Exception e) {
String s = getStackTrace(e);
System.err.println(s);
}
}
public static String getStackTrace(Throwable t) {
StringWriter stringWriter = new StringWriter();
// Create PrintWriter via PrintWriter(Writer) constructor.
PrintWriter pw = new PrintWriter(stringWriter);
// Call method: Throwable.printStackTrace(PrintWriter)
t.printStackTrace(pw);
pw.close();
String s = stringWriter.getBuffer().toString();
return s;
}
}
Output:
java.lang.ArithmeticException: / by zero
at org.o7planning.printwriter.ex.GetStackTraceEx.main(GetStackTraceEx.java:10)
PrintWriterEx1.java
package org.o7planning.printwriter.ex;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Locale;
public class PrintWriterEx1 {
// Windows: C:/SomeFolder/pw-out-test.txt
private static final String filePath = "/Volumes/Data/test/pw-out-test.txt";
public static void main(String[] args) throws IOException {
// Create a PrintWriter to write a file.
PrintWriter printWriter = new PrintWriter(filePath);
LocalDateTime now = LocalDateTime.now();
String empName = "Tran";
LocalDate hireDate = LocalDate.of(2000, 4, 23);
int salary = 10000;
printWriter.printf("# File generated on %1$tA, %1$tB %1$tY %tH:%tM:%tS %n", now, now, now);
printWriter.println(); // New line
printWriter.printf("Employee Name: %s%n", empName);
printWriter.printf("Hire date: %1$td.%1$tm.%1$tY %n", hireDate);
printWriter.printf(Locale.US, "Salary: $%,d %n", salary);
printWriter.close();
}
}
Output:
pw-out-test.txt
# File generated on Thursday, February 2021 01:31:22
Employee Name: Tran
Hire date: 23.04.2000
Salary: $10,000
Hai phương thức PrintStream.printf(..) và PrintWriter.printf(..) giống nhau về cách sử dụng, bạn có thể tham khảo thêm cách sử dụng của nó trong bài viết dưới đây.
5. checkError()
Phương thức checkError() trả về trạng thái lỗi của PrintWriter này, ngoài ra phương thức flush() cũng được gọi nếu PrintWriter này chưa bị đóng.
public boolean checkError()
Chú ý: Tất cả các phương thức của PrintWriter không ném ra IOException, nhưng một khi IOException xẩy ra trong nội bộ các phương thức thì trạng thái của nó được coi là lỗi.
Trạng thái lỗi của PrintWriter này chỉ bị xoá bỏ nếu gọi phương thức clearError() nhưng đây là một phương thức protected. Bạn có thể viết một lớp mở rộng từ PrintWriter và ghi đè (override) phương thức này nếu muốn sử dụng nó (Xem ví dụ bên dưới).
MyPrintWriter.java
package org.o7planning.printwriter.ex;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class MyPrintWriter extends PrintWriter {
public MyPrintWriter(File file) throws FileNotFoundException {
super(file);
}
@Override
public void clearError() {
super.clearError(); // Call protected method.
}
}
Ví dụ: Sử dụng phương thức checkError() để kiểm tra trạng thái lỗi của PrintWriter:
PrintWriter_checkError_ex1.java
package org.o7planning.printwriter.ex;
import java.io.File;
public class PrintWriter_checkError_ex1 {
// Windows: C:/SomeFolder/logFile.txt
private static final String logFilePath = "/Volumes/Data/test/logFile.txt";
public static void main(String[] args) throws Exception {
File logFile = new File(logFilePath);
MyPrintWriter mps = new MyPrintWriter(logFile);
int errorCount = 0;
while (true) {
// Write log..
mps.println("Some Log..");
Thread.sleep(1000);
// Check if IOException happened.
if (mps.checkError()) {
errorCount++;
mps.clearError();
if (errorCount > 10) {
sendAlertEmail();
break;
}
}
}
mps.close();
}
private static void sendAlertEmail() {
System.out.println("There is a problem in the Log system.");
}
}
6. print(..) *
Phương thức print(..) được sử dụng để in một giá trị nguyên thuỷ vào PrintWriter này.
public void print(boolean b)
public void print(char c)
public void print(char[] s)
public void print(double d)
public void print(float f)
public void print(int i)
public void print(long l)
public void print(String s)
Ví dụ:
PrintWriter_print_primitive_ex1.java
Writer swriter = new StringWriter();
PrintWriter ps = new PrintWriter(swriter);
ps.print(true);
ps.println();
ps.print(new char[] { 'a', 'b', 'c' });
ps.println();
ps.print("Text");
System.out.println(swriter.toString());
Output:
true
abc
Text
7. print(Object)
Chuyển một đối tượng thành một String bằng phương thức String.valueOf(Object), và in kết quả vào PrintWriter này.
public void print(Object obj) {
this.write(String.valueOf(obj));
}
Ví dụ:
PrintWriter_print_object_ex1.java
Writer writer = new StringWriter();
PrintWriter ps = new PrintWriter(writer);
Object obj1 = null;
ps.print(obj1);
ps.println();
Object obj2 = new Socket();
ps.print(obj2);
ps.println();
Object obj3 = Arrays.asList("One", "Two", "Three");
ps.print(obj3);
String content = writer.toString();
System.out.println(content);
Output:
null
Socket[unconnected]
[One, Two, Three]
8. println()
In ký tự xuống dòng vào PrintWriter này. Phương thức flush() cũng được gọi nếu PrintWriter này có chế độ tự động xả (auto flush).
public void println() {
print('\n');
if(autoFlush) this.flush();
}
9. println(..) *
Phương thức println(..) được sử dụng để in một giá trị nguyên thuỷ và một ký tự xuống dòng vào PrintWriter này. Phương thức flush() cũng được gọi nếu PrintWriter này có chế độ tự động xả (auto flush).
public void println(boolean x)
public void println(char x)
public void println(char[] x)
public void println(double x)
public void println(float x)
public void println(int x)
public void println(long x)
public void println(String x)
Ví dụ:
PrintWriter_println_primitive_ex1.java
OutputStream baos = new ByteArrayOutputStream();
PrintWriter ps = new PrintWriter(baos);
ps.println(true);
ps.println(new char[] { 'a', 'b', 'c' });
ps.println("Text");
ps.flush();
String content = baos.toString();
System.out.println(content);
Output:
true
abc
Text
10. println(Object)
Chuyển một đối tượng thành một String bằng phương thức String.valueOf(Object) sau đó in kết quả và ký tự xuống dòng vào PrintWriter này. Phương thức flush() cũng được gọi nếu PrintWriter này có chế độ tự động xả (auto flush). Phương thức này hoạt động giống như việc gọi phương thức print(Object) và sau đó là println().
public void println(Object x) {
this.write(String.valueOf(x));
this.write("\n");
if(this.autoFlush) this.flush();
}
Ví dụ:
PrintWriter_println_object_ex1.java
Writer swriter = new StringWriter();
PrintWriter ps = new PrintWriter(swriter);
Object obj1 = null;
ps.println(obj1);
Object obj2 = new Socket();
ps.println(obj2);
Object obj3 = Arrays.asList("One", "Two", "Three");
ps.println(obj3);
String content = swriter.toString();
System.out.println(content);
Output:
null
Socket[unconnected]
[One, Two, Three]
11. printf(..) *
Một phương pháp tiện lợi để ghi một chuỗi được định dạng vào PrintWriter này bằng cách sử dụng chuỗi định dạng và các đối số được chỉ định.
public PrintWriter printf(String format, Object... args)
public PrintWriter printf(Locale l, String format, Object... args)
Phương thức này hoạt động tương tự như đoạn code dưới đây:
String result = String.format(format, args);
printStream.print(result);
// Or:
String result = String.format(locale, format, args);
printStream.print(result);
Xem thêm:
12. format(..) *
Phương thức này hoạt động giống phương thức printf(..).
public PrintWriter format(String format, Object... args)
public PrintWriter format(Locale l, String format, Object... args)
Nó hoạt động giống như đoạn code dưới đây:
String result = String.format(format, args);
printStream.print(result);
// Or:
String result = String.format(locale, format, args);
printStream.print(result);
Xem thêm:
13. append(..) *
Các phương thức append(..) hoạt động giống như các phương thức print(..), điều khác biệt là nó trả về PrintWriter này, vì vậy bạn có thể tiếp tục gọi một phương thức tiếp theo thay vì kết thúc bởi dấu chấm phẩy ( ; ).
public PrintWriter append(char c)
public PrintWriter append(CharSequence csq)
public PrintWriter append(CharSequence csq, int start, int end)
Ví dụ:
PrintWriter_append_ex1.java
ByteArrayOutputStream baos = new ByteArrayOutputStream();
PrintWriter ps = new PrintWriter(baos);
ps.append("This").append(" is").append(' ').append('a').append(" Text");
ps.flush();
String text = baos.toString();
System.out.println(text);// This is a Text
Các hướng dẫn Java IO
- Hướng dẫn và ví dụ Java CharArrayWriter
- Hướng dẫn và ví dụ Java FilterReader
- Hướng dẫn và ví dụ Java FilterWriter
- Hướng dẫn và ví dụ Java PrintStream
- Hướng dẫn và ví dụ Java BufferedReader
- Hướng dẫn và ví dụ Java BufferedWriter
- Hướng dẫn và ví dụ Java StringReader
- Hướng dẫn và ví dụ Java StringWriter
- Hướng dẫn và ví dụ Java PipedReader
- Hướng dẫn và ví dụ Java LineNumberReader
- Hướng dẫn và ví dụ Java PushbackReader
- Hướng dẫn và ví dụ Java PrintWriter
- Hướng dẫn sử dụng luồng vào ra nhị phân trong Java
- Hướng dẫn sử dụng luồng vào ra ký tự trong Java
- Hướng dẫn và ví dụ Java BufferedOutputStream
- Hướng dẫn và ví dụ Java ByteArrayOutputStream
- Hướng dẫn và ví dụ Java DataOutputStream
- Hướng dẫn và ví dụ Java PipedInputStream
- Hướng dẫn và ví dụ Java OutputStream
- Hướng dẫn và ví dụ Java ObjectOutputStream
- Hướng dẫn và ví dụ Java PushbackInputStream
- Hướng dẫn và ví dụ Java SequenceInputStream
- Hướng dẫn và ví dụ Java BufferedInputStream
- Hướng dẫn và ví dụ Java Reader
- Hướng dẫn và ví dụ Java Writer
- Hướng dẫn và ví dụ Java FileReader
- Hướng dẫn và ví dụ Java FileWriter
- Hướng dẫn và ví dụ Java CharArrayReader
- Hướng dẫn và ví dụ Java ByteArrayInputStream
- Hướng dẫn và ví dụ Java DataInputStream
- Hướng dẫn và ví dụ Java ObjectInputStream
- Hướng dẫn và ví dụ Java InputStreamReader
- Hướng dẫn và ví dụ Java OutputStreamWriter
- Hướng dẫn và ví dụ Java InputStream
- Hướng dẫn và ví dụ Java FileInputStream
Show More
- Hướng dẫn lập trình Java Servlet/JSP
- Các hướng dẫn Java New IO
- Các hướng dẫn Spring Cloud
- Các hướng dẫn Java Oracle ADF
- Các hướng dẫn Java Collections Framework
- Java cơ bản
- Các hướng dẫn Java Date Time
- Các thư viện mã nguồn mở Java
- Các hướng dẫn Java Web Services
- Các hướng dẫn Struts2 Framework
- Các hướng dẫn Spring Boot