Phương thức String.format() và printf() trong Java
1. printf()
Trong lập trình Java, rất thường xuyên bạn bắt gặp đoạn mã System.out.printf(..). Thực ra, phương thức printf(..) được định nghĩa trong cả hai lớp PrintStream và PrintWriter, cách sử dụng của chúng là tương tự nhau, printf là viết tắt của "Print + Format".
printf methods
// Definition of the printf methods in the PrintWriter class:
public PrintStream printf(Locale l, String format, Object... args)
public PrintStream printf(String format, Object... args)
// Definition of the printf methods in PrintWriter class:
public PrintWriter printf(Locale l, String format, Object... args)
public PrintWriter format(String format, Object... args)
System.out.printf(..) là một trường hợp đặc biệt, được sử dụng để ghi một văn bản có định dạng ra màn hình Console, trong đó System.out thực chất là một đối tượng kiểu PrintStream.
System class
package java.lang;
public final class System {
public static final PrintStream out = ...;
}
Trước khi bắt đầu, hãy xem một ví dụ đơn giản:
Printf_ex1.java
package org.o7planning.printf.ex;
public class Printf_ex1 {
public static void main(String[] args) {
String firstName = "James";
String lastName = "Smith";
int age = 20;
System.out.printf("My name is %s %s, I'm %d years old.", firstName, lastName, age);
}
}
Output:
My name is James Smith, I'm 20 years old.
2. Quy tắc định dạng
%[flags][width][.precision]conversion-character
Các tham số: flags, width, precision là tuỳ chọn.
3. conversion-character
%[flags][width][.precision]conversion-character
conversion-character | Description | Type |
d | decimal integer | byte, short, int, long |
f | floating-point number | float, double |
b | Boolean | Object |
B | will uppercase the boolean | Object |
c | Character Capital | String |
C | will uppercase the letter | String |
s | String Capital | String |
S | will uppercase all the letters in the string | String |
h | hashcode - A hashcode is like an address. This is useful for printing a reference | Object |
n | newline - Platform specific newline character - use %n instead of \n for greater compatibility |
conversion-character: n
System.out.printf("One%nTwo%nThree");
Output:
One
Two
Three
conversion-character: s
System.out.printf("My name is %s %s", "James", "Smith");
Output:
My name is James Smith
conversion-character: S
System.out.printf("My name is %S %S", "James", "Smith");
Output:
My name is JAMES SMITH
conversion-character: b
System.out.printf("%b%n", null);
System.out.printf("%b%n", false);
System.out.printf("%b%n", 5.3);
System.out.printf("%b%n", "Any text");
System.out.printf("%b%n", new Object());
Output:
false
false
true
true
true
conversion-character: B
System.out.printf("%B%n", null);
System.out.printf("%B%n", false);
System.out.printf("%B%n", 5.3);
System.out.printf("%B%n", "Any text");
System.out.printf("%B%n", new Object());
Output:
FALSE
FALSE
TRUE
TRUE
TRUE
conversion-character: d
System.out.printf("There are %d teachers and %d students in the class", 2, 25);
Output:
There are 2 teachers and 25 students in the class
conversion-character: f
System.out.printf("Exchange rate today: EUR %f = USD %f", 1.0, 1.2059);
Output:
Exchange rate today: EUR 1.000000 = USD 1.205900
conversion-character: c
char ch = 'a';
System.out.printf("Code of '%c' character is %d", ch, (int)ch);
Output:
Code of 'a' character is 97
conversion-character: C
char ch = 'a';
System.out.printf("'%C' is the upper case of '%c'", ch, ch);
Output:
'A' is the upper case of 'a'
conversion-character: h
Printf_cc_h_ex.java
package org.o7planning.printf.ex;
public class Printf_cc_h_ex {
public static void main(String[] args) {
// h (Hashcode HEX)
Object myObject = new AnyObject("Something");
System.out.println("Hashcode: " + myObject.hashCode());
System.out.println("Identity Hashcode: " + System.identityHashCode(myObject));
System.out.println("Hashcode (HEX): " + Integer.toHexString(myObject.hashCode()));
System.out.println("toString : " + String.valueOf(myObject));
System.out.printf("Printf: %h", myObject);
}
}
class AnyObject {
private String val;
public AnyObject(String val) {
this.val = val;
}
@Override
public int hashCode() {
if (this.val == null || this.val.isEmpty()) {
return 0;
}
return 1000 + (int) this.val.charAt(0);
}
}
Output:
Hashcode: 1083
Identity Hashcode: 1579572132
Hashcode (HEX): 43b
toString : org.o7planning.printf.ex.AnyObject@43b
Printf: 43b
4. width
%[flags][width][.precision]conversion-character
width - chỉ định khoảng không gian (số ký tự) tối thiểu để giữ chỗ cho việc in ra nội dung của một đối số tương ứng.
printf(format, arg1, arg2,.., argN)
5. flags
%[flags][width][.precision]conversion-character
flag | Description |
- | left-justify (default is to right-justify ) |
+ | output a plus ( + ) or minus ( - ) sign for a numerical value |
0 | forces numerical values to be zero-padded (default is blank padding ) |
, | comma grouping separator (for numbers > 1000) |
space will display a minus sign if the number is negative or a space if it is positive |
flag: -
String[] fullNames = new String[] {"Tom", "Jerry", "Donald"};
float[] salaries = new float[] {1000, 1500, 1200};
// s
System.out.printf("|%-10s | %-30s | %-15s |%n", "No", "Full Name", "Salary");
for(int i=0; i< fullNames.length; i++) {
System.out.printf("|%-10d | %-30s | %15f |%n", (i+1), fullNames[i], salaries[i]);
}
Output:
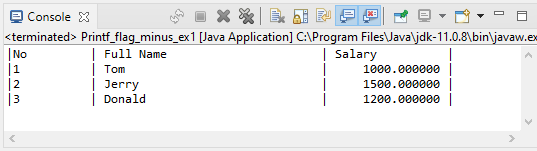
flag: +
// flag: +
System.out.printf("The world's economy increased by %+f percent in 2020. %n", -3.3);
System.out.printf("China's economy increased by %+f percent in 2020. %n", 2.3);
System.out.println();
// without flag: +
System.out.printf("The world's economy increased by %f percent in 2020. %n", -3.3);
System.out.printf("China's economy increased by %f percent in 2020. %n", 2.3);
Output:
The world's economy increased by -3.300000 percent in 2020.
China's economy increased by +2.300000 percent in 2020.
The world's economy increased by -3.300000 percent in 2020.
China's economy increased by 2.300000 percent in 2020.
flag: 0 (zero)
// flag: 0 & with: 20
System.out.printf("|%020f|%n", -3.1);
// without flag & with: 20
System.out.printf("|%20f|%n", -3.1);
// flag: - (left align) & with: 20
System.out.printf("|%-20f
Output:
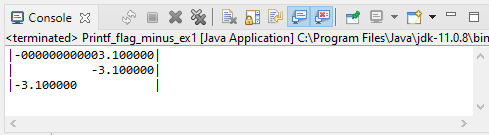
flag: , (comma)
// flag: ,
System.out.printf("%,f %n", 12345678.9);
// flag: ,
System.out.printf("%,f %n", -12345678.9);
Output:
12,345,678.900000
-12,345,678.900000
flag: (space)
// flag: (space)
System.out.printf("% f %n", 12345678.9);
System.out.printf("% f %n", -12345678.9);
System.out.println();
// flags: , (space + comma)
System.out.printf("% ,f %n", 12345678.9);
System.out.printf("% ,
Output:
12345678.900000
-12345678.900000
12,345,678.900000
-12,345,678.900000
6. precision
%[flags][width][.precision]conversion-character
System.out.printf("%f %n", 12345678.911);
System.out.printf("%f %n", -12345678.911);
System.out.println();
// flag: , (comma)
System.out.printf("%,f %n", 12345678.911);
// flag: , (comma)
System.out.printf("%,f %n", -12345678.911);
System.out.println();
// flags: , (space + comma)
System.out.printf("% ,f %n", 12345678.911);
// flags: , (space + comma)
System.out.printf("% ,f %n", -12345678.911);
System.out.println();
// flag: , (comma) & precision: .2
System.out.printf("%,.2f %n", 12345678.911);
// flag: , (comma) & precision: .3
System.out.printf("%,.3f %n", -12345678.911);
System.out.println();
// flags: , (space + comma) & precision: .2
System.out.printf("% ,.2f %n", 12345678.911);
// flags: , (space + comma) & precision: .3
System.out.printf("% ,.3f %n", -12345678.911);
Output:
12345678.911000
-12345678.911000
12,345,678.911000
-12,345,678.911000
12,345,678.911000
-12,345,678.911000
12,345,678.91
-12,345,678.911
12,345,678.91
-12,345,678.911
Java cơ bản
- Tùy biến trình biên dịch java xử lý Annotation của bạn (Annotation Processing Tool)
- Lập trình Java theo nhóm sử dụng Eclipse và SVN
- Hướng dẫn và ví dụ Java WeakReference
- Hướng dẫn và ví dụ Java PhantomReference
- Hướng dẫn nén và giải nén trong Java
- Cấu hình Eclipse để sử dụng JDK thay vì JRE
- Phương thức String.format() và printf() trong Java
- Cú pháp và các tính năng mới trong Java 5
- Cú pháp và các tính năng mới trong Java 8
- Hướng dẫn sử dụng biểu thức chính quy trong Java
- Hướng dẫn lập trình đa luồng trong Java - Java Multithreading
- Thư viện điều khiển các loại cơ sở dữ liệu khác nhau trong Java
- Hướng dẫn sử dụng Java JDBC kết nối cơ sở dữ liệu
- Lấy các giá trị của các cột tự động tăng khi Insert một bản ghi sử dụng JDBC
- Hướng dẫn và ví dụ Java Stream
- Functional Interface trong Java
- Giới thiệu về Raspberry Pi
- Hướng dẫn và ví dụ Java Predicate
- Abstract class và Interface trong Java
- Access modifier trong Java
- Hướng dẫn và ví dụ Java Enum
- Hướng dẫn và ví dụ Java Annotation
- So sánh và sắp xếp trong Java
- Hướng dẫn và ví dụ Java String, StringBuffer và StringBuilder
- Hướng dẫn xử lý ngoại lệ trong Java - Java Exception Handling
- Hướng dẫn và ví dụ Java Generics
- Thao tác với tập tin và thư mục trong Java
- Hướng dẫn và ví dụ Java BiPredicate
- Hướng dẫn và ví dụ Java Consumer
- Hướng dẫn và ví dụ Java BiConsumer
- Bắt đầu với Java cần những gì?
- Lịch sử của Java và sự khác biệt giữa Oracle JDK và OpenJDK
- Cài đặt Java trên Windows
- Cài đặt Java trên Ubuntu
- Cài đặt OpenJDK trên Ubuntu
- Cài đặt Eclipse
- Cài đặt Eclipse trên Ubuntu
- Học nhanh Java cho người mới bắt đầu
- Lịch sử của bit và byte trong khoa học máy tính
- Các kiểu dữ liệu trong Java
- Các toán tử Bitwise
- Câu lệnh rẽ nhánh (if else) trong Java
- Câu lệnh rẽ nhánh switch trong Java
- Vòng lặp trong Java
- Mảng (Array) trong Java
- JDK Javadoc định dạng CHM
- Thừa kế và đa hình trong Java
- Hướng dẫn và ví dụ Java Function
- Hướng dẫn và ví dụ Java BiFunction
- Ví dụ về Java encoding và decoding sử dụng Apache Base64
- Hướng dẫn và ví dụ Java Reflection
- Hướng dẫn gọi phương thức từ xa với Java RMI
- Hướng dẫn lập trình Java Socket
- Các nền tảng nào bạn nên chọn để lập trình ứng dụng Java Desktop?
- Hướng dẫn và ví dụ Java Commons IO
- Hướng dẫn và ví dụ Java Commons Email
- Hướng dẫn và ví dụ Java Commons Logging
- Tìm hiểu về Java System.identityHashCode, Object.hashCode và Object.equals
- Hướng dẫn và ví dụ Java SoftReference
- Hướng dẫn và ví dụ Java Supplier
- Lập trình Java hướng khía cạnh với AspectJ (AOP)
Show More
- Hướng dẫn lập trình Java Servlet/JSP
- Các hướng dẫn Java Collections Framework
- Java API cho HTML & XML
- Các hướng dẫn Java IO
- Các hướng dẫn Java Date Time
- Các hướng dẫn Spring Boot
- Các hướng dẫn Maven
- Các hướng dẫn Gradle
- Các hướng dẫn Java Web Services
- Các hướng dẫn lập trình Java SWT
- Các hướng dẫn lập trình JavaFX
- Các hướng dẫn Java Oracle ADF
- Các hướng dẫn Struts2 Framework
- Các hướng dẫn Spring Cloud