Hướng dẫn và ví dụ ReactJS Refs
1. React Refs
Trong ReactJS, refs được sử dụng để bạn tham chiếu (reference) tới một phần tử. Về cơ bản nên tránh sử dụng refs trong hầu hết các trường hợp. Tuy nhiên nó có ích khi bạn muốn truy cập vào các nút (node) của DOM hoặc các phần tử được tạo ra trong phương thức render().
Chú ý: Trong bài học này tôi sẽ tạo Refs theo phong cách của ReactJS phiên bản 16.3, bởi vì nó dễ dàng hơn cho việc sử dụng.
React.createRef()
Sử dụng phương thức React.createRef() để tạo Refs, sau đó đính (attach) nó vào các phần tử (Được tạo ra trong render() ) thông qua thuộc tính ref.
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.myRef = React.createRef();
}
render() {
return <div ref={this.myRef} />;
}
}
Khi mà một Ref được đính (attach) vào một phần tử được tạo ra trong phương thức render(). Bạn có thể tham chiếu tới đối tượng Node của phần tử này thông qua thuộc tính current của Ref.
const node = this.myRef.current;
Ví dụ:
refs-example.jsx
//
class SearchBox extends React.Component {
constructor(props) {
super(props);
this.state = {
searchText: "reactjs"
};
this.searchFieldRef = React.createRef();
}
clearAndFocus() {
this.setState({ searchText: "" });
// Focus to Input Field.
this.searchFieldRef.current.focus();
this.searchFieldRef.current.style.background = "#e8f8f5";
}
changeSearchText(event) {
var v = event.target.value;
this.setState((prevState, props) => {
return {
searchText: v
};
});
}
render() {
return (
<div className="search-box">
<input
value={this.state.searchText}
ref={this.searchFieldRef}
onChange={event => this.changeSearchText(event)}
/>
<button onClick={() => this.clearAndFocus()}>Clear And Focus</button>
<a href="">Reset</a>
</div>
);
}
}
// Render
ReactDOM.render(<SearchBox />, document.getElementById("searchbox1"));
refs-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Refs</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.search-box {
border:1px solid #cbbfab;
padding: 5px;
margin: 5px;
}
</style>
</head>
<body>
<h3>React Refs:</h3>
<div id="searchbox1"></div>
<script src="refs-example.jsx" type="text/babel"></script>
</body>
</html>
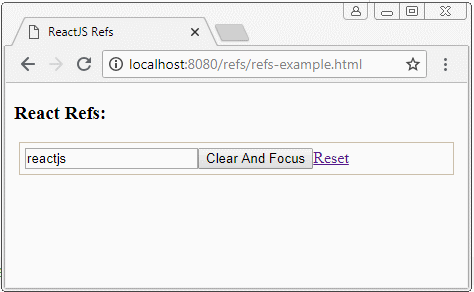
Các hướng dẫn ReactJS
- Hướng dẫn và ví dụ ReactJS props và state
- Xử lý sự kiện (Event) trong ReactJS
- Hướng dẫn và ví dụ ReactJS Component API
- Các phương thức trong vòng đời của ReactJS Component
- Hướng dẫn và ví dụ ReactJS Refs
- Hướng dẫn và ví dụ ReactJS Lists và Keys
- Hướng dẫn và ví dụ ReactJS Form
- Tìm hiểu về ReactJS Router với ví dụ tại phía Client
- Giới thiệu về Redux
- Ví dụ đơn giản với React và Redux tại phía Client
- Hướng dẫn sử dụng React-Transition-Group API
- Bắt đầu nhanh với ReactJS trên môi trường NodeJS
- Tìm hiểu về ReactJS Router với một ví dụ cơ bản (NodeJS)
- Ví dụ React-Transition-Group Transition (NodeJS)
- Ví dụ React-Transition-Group CSSTransition (NodeJS)
- Giới thiệu về ReactJS
- Cài đặt React Plugin cho trình soạn thảo Atom
- Tạo một HTTP Server đơn giản với NodeJS
- Bắt đầu nhanh với ReactJS
Show More