Hướng dẫn sử dụng Spring MVC và Velocity
1. Giới thiệu
Tài liệu được viết dựa trên:
Spring MVC 4.x
Eclipse 4.6 (NEON)
Apache Velocity là một bộ phân tích mẫu (template) viết trên Java, nó cung cấp một ngôn ngữ template để tham chiếu vào các đối tượng Java, và hiển thị dữ liệu Java. Mục đích của nó là làm tách bạch giữa 2 tầng hiện thị và tầng dữ liệu (presentation tier and business tiers) trong mô hình MVC (Model-View -Controller).
Velocity là một project mã nguồn mở được cung cấp bởi Apache. Nó được phát hành theo giấy phép của Apache.
Velocity là một project mã nguồn mở được cung cấp bởi Apache. Nó được phát hành theo giấy phép của Apache.
Như vậy về bản chất Velocity có thể dụng trên tầng View để hiển thị dữ liệu. Velocity là một ngôn ngữ mẫu (template).
Bạn có thể tham khảo thêm tài liệu về Velocity tại:
- TODO
Chú ý: Bắt đầu từ phiên bản 4.3 Spring không còn hỗ trợ Velocity, và Velocity sẽ bị loại bỏ khỏi Spring từ phiên bản 5 (Trong tương lai)Ở phía dưới đây là một cuộc hội thoại, có vẻ giống một cuộc cãi vã giữa các nhà phát triển Velocity (Apache) và các nhà phát triển Spring, xoay quanh vấn đề vì sao Velocity không còn được hỗ trợ trong nền tảng của Spring nữa.
2. Tạo Maven Project
- File/New/Other...
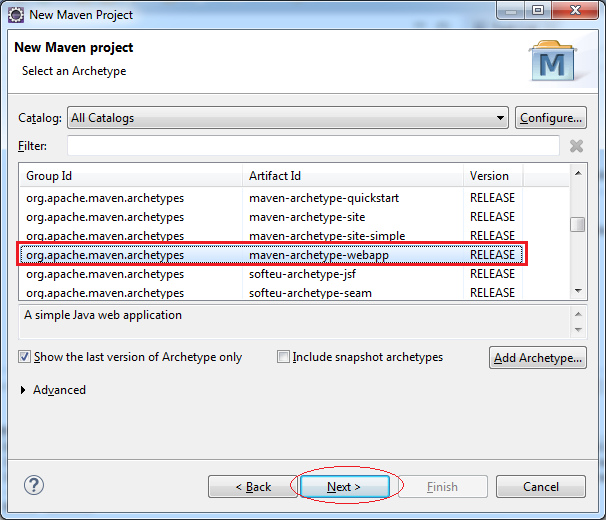
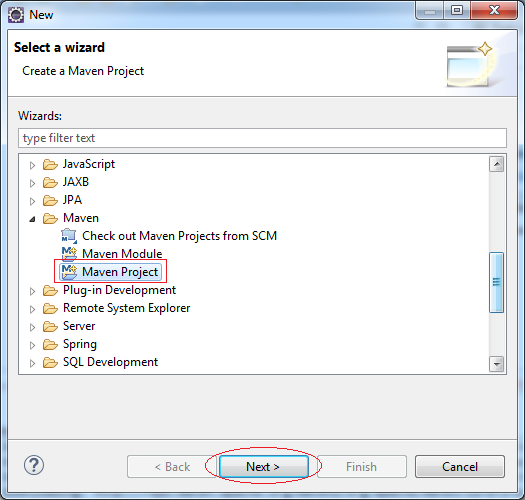
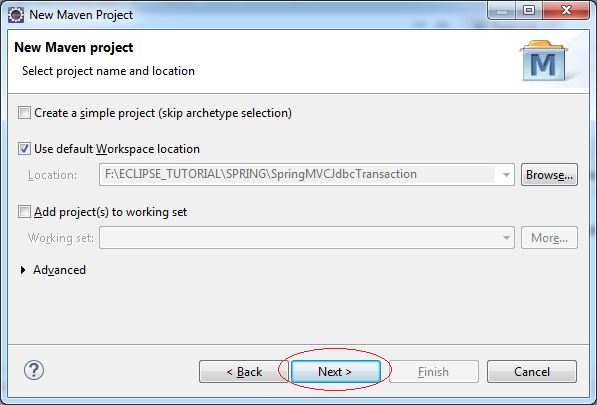
Nhập vào:
- Group Id: org.o7planning
- Artifact Id: SpringMVCVelocity
- Package: org.o7planning.tutorial.springmvcvelocity
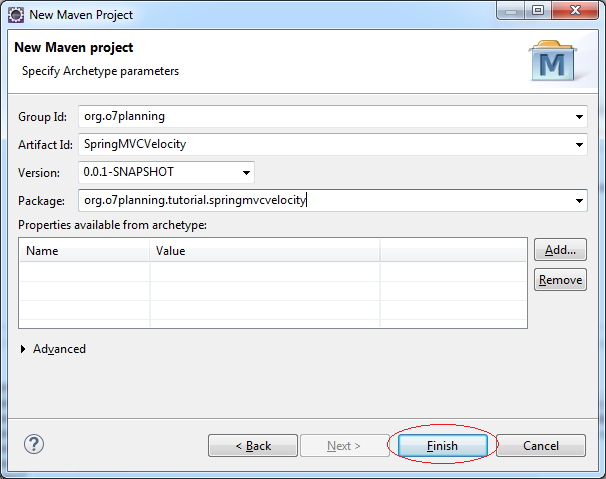
Project đã được tạo ra:
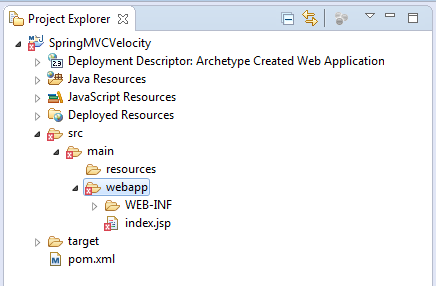
Bạn đừng lo lắng với thông báo lỗi khi Project vừa được tạo ra. Lý do là bạn chưa khai báo thư viện Servlet.
Eclipse 4.4 (Luna) tạo ra project Maven có thể sai cấu trúc. Bạn cần phải kiểm tra.
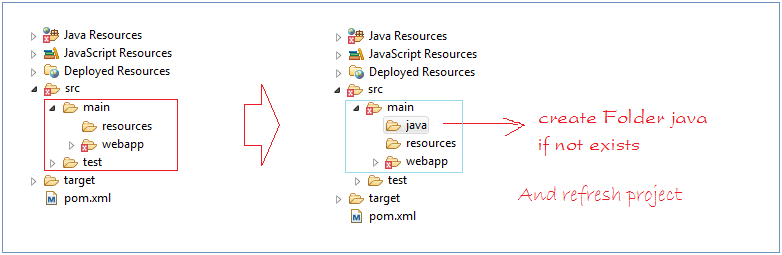
3. Khai báo Maven
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringMVCVelocity</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCVelocity Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring framework START -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- contains: VelocityEngineFactory -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-context-support -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>4.1.4.RELEASE</version>
</dependency>
<!-- Spring framework END -->
<!-- Servlet API -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.velocity/velocity -->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity</artifactId>
<version>1.7</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.apache.velocity/velocity-tools -->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity-tools</artifactId>
<version>2.0</version>
</dependency>
</dependencies>
<build>
<finalName>SpringMVCVelocity</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default - /SpringMVCVelocity : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
4. Cấu hình Spring
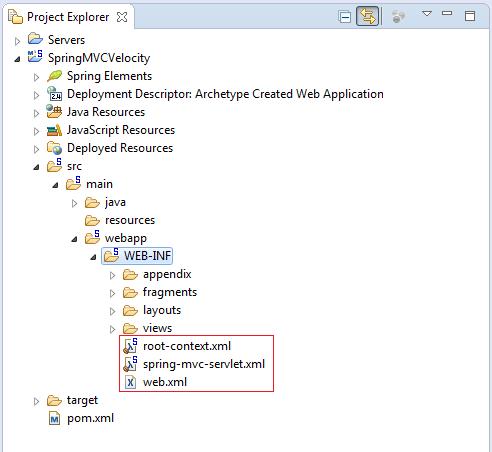
web.xml
<web-app id="WebApp_ID" version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>Archetype Created Web Application</display-name>
<servlet>
<servlet-name>spring-mvc</servlet-name>
<servlet-class>
org.springframework.web.servlet.DispatcherServlet
</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring-mvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- Other XML Configuration -->
<!-- Load by Spring ContextLoaderListener -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>
/WEB-INF/root-context.xml,
/WEB-INF/spring-mvc-servlet.xml
</param-value>
</context-param>
<!-- Spring ContextLoaderListener -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
</web-app>
spring-mvc-servlet.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd">
<!-- Register the annotated components in the container eg : annotated controllers -->
<context:component-scan base-package="org.o7planning.tutorial.springmvcvelocity.*" />
<!-- Tell the container that we are going to use annotations -->
<context:annotation-config />
<bean id="velocityConfig"
class="org.springframework.web.servlet.view.velocity.VelocityConfigurer">
<property name="resourceLoaderPath">
<value>/</value>
</property>
</bean>
<bean id="viewResolver"
class="org.springframework.web.servlet.view.velocity.VelocityLayoutViewResolver">
<property name="cache" value="true" />
<property name="prefix" value="/WEB-INF/views/" />
<property name="layoutUrl" value="/WEB-INF/layouts/layout.vm" />
<property name="suffix" value=".vm" />
</bean>
</beans>
root-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Empty -->
</beans>
5. Views
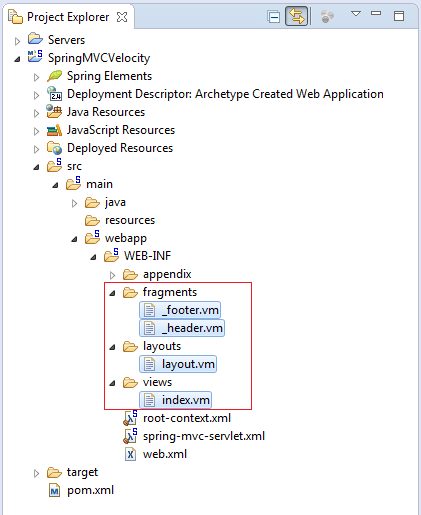
/WEB-INF/fragments/_header.vm
<div style="background: #E0E0E0; height: 80px; padding: 5px;">
<div style="float: left">
<h1>My Site</h1>
</div>
<div style="float: right; padding: 10px;">
Search <input name="search">
</div>
</div>
/WEB-INF/fragments/_footer.vm
<div
style="background: #E0E0E0; text-align: center; padding: 5px; margin-top: 10px;">
@Copyright mysite.com
</div>
/WEB-INF/layouts/layout.vm
<html>
<head>
<title>Spring & Velocity</title>
</head>
<body>
<div>
#parse("/WEB-INF/fragments/_header.vm")
</div>
<div>
<!-- View abc.vm is inserted here -->
<!-- Noi dung View abc.vm se duoc tren tai day -->
$screen_content
</div>
<div>
#parse("/WEB-INF/fragments/_footer.vm")
</div>
</body>
</html>
$screen_content: Là vị trí các views.vm sẽ được trèn vào.
/WEB-INF/views/index.vm
<h1>Index</h1>
<h2>Department list</h2>
<table border="1">
<tr>
<th>Dept No</th>
<th>Dept Name</th>
</tr>
#foreach($dept in $departments)
<tr>
<td>$dept.deptNo</td>
<td>$dept.deptName</td>
</tr>
#end
</table>
6. Java Classes
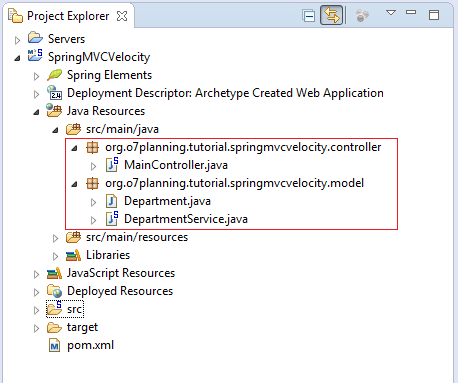
Department.java
package org.o7planning.tutorial.springmvcvelocity.model;
public class Department {
private Integer deptId;
private String deptNo;
private String deptName;
private String location;
public Department() {
}
public Department(Integer deptId, String deptName, String location) {
this.deptId = deptId;
this.deptNo = "D" + deptId;
this.deptName = deptName;
this.location = location;
}
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
public String getDeptNo() {
return deptNo;
}
public void setDeptNo(String deptNo) {
this.deptNo = deptNo;
}
public String getDeptName() {
return deptName;
}
public void setDeptName(String deptName) {
this.deptName = deptName;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
}
DepartmentService.java
package org.o7planning.tutorial.springmvcvelocity.model;
import java.util.ArrayList;
import java.util.List;
import org.springframework.stereotype.Service;
@Service
public class DepartmentService {
public List<Department> listDepartment() {
List<Department> list = new ArrayList<Department>();
list.add(new Department(1, "Operations", "Chicago"));
list.add(new Department(2, "HR", "Hanoi"));
return list;
}
}
Spring coi các class được chú thích bởi @Service, @Controller là các Bean. Nó được tự động tiêm vào một Bean khác thông qua chú thích @Autowired.
MainController.java
package org.o7planning.tutorial.springmvcvelocity.controller;
import java.util.List;
import org.o7planning.tutorial.springmvcvelocity.model.Department;
import org.o7planning.tutorial.springmvcvelocity.model.DepartmentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MainController {
@Autowired
private DepartmentService deptService;
@RequestMapping(value = { "/", "/welcome" }, method = RequestMethod.GET)
public String welcomePage(Model model) {
List<Department> list = deptService.listDepartment();
model.addAttribute("departments", list);
return "index";
}
}
Các hướng dẫn Spring MVC
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Cài đặt Spring Tool Suite cho Eclipse
- Hướng dẫn lập trình Spring MVC cho người mới bắt đầu - Hello Spring 4 MVC
- Cấu hình các nguồn dữ liệu tĩnh trong Spring MVC
- Hướng dẫn sử dụng Spring MVC Interceptor
- Tạo ứng dụng web đa ngôn ngữ với Spring MVC
- Hướng dẫn Upload File với Spring MVC
- Ứng dụng Java Web login đơn giản sử dụng Spring MVC, Spring Security và Spring JDBC
- Hướng dẫn sử dụng Spring MVC Security với Hibernate
- Hướng dẫn sử dụng Spring MVC Security và Spring JDBC (XML Config)
- Đăng nhập bằng mạng xã hội trong Spring MVC với Spring Social Security
- Hướng dẫn sử dụng Spring MVC và Velocity
- Hướng dẫn sử dụng Spring MVC với FreeMarker
- Sử dụng Template trong Spring MVC với Apache Tiles
- Hướng dẫn sử dụng Spring MVC và Spring JDBC Transaction
- Sử dụng nhiều DataSource trong Spring MVC
- Hướng dẫn sử dụng Spring MVC, Hibernate và Spring Transaction Manager
- Hướng dẫn sử dụng Spring MVC Form và Hibernate
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Tạo một ứng dụng Java Web bán hàng sử dụng Spring MVC và Hibernate
- Ví dụ CRUD đơn giản với Spring MVC RESTful Web Service
- Triển khai ứng dụng Spring MVC trên Oracle WebLogic Server
Show More