Sử dụng Template trong Spring MVC với Apache Tiles
1. Giới thiệu
Tài liệu hướng dẫn này được viết dựa trên:
- Eclipse 4.6 NEON(ok for Eclipse 4.5 MARS)
- Spring MVC 4.x
- Apache Tiles 3.x
2. Apache Tiles là gì?
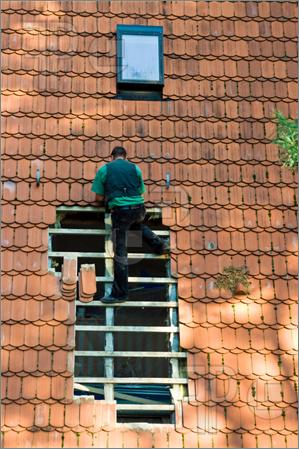
Apache Titles lấy lý tưởng từ việc xếp các viên ngói lại với nhau để tạo nên mái nhà.
Một trang web của bạn được coi là một mái nhà, nó được ghép lại từ các viên ngói, một viên ngói ở đây là một file jsp (jsp fragment). Apache Titles giúp bạn định nghĩa ra một khuôn mẫu (Template) để ghép các thành phần (các mảnh jsp) lại với nhau để thành một trang web.
Một trang web của bạn được coi là một mái nhà, nó được ghép lại từ các viên ngói, một viên ngói ở đây là một file jsp (jsp fragment). Apache Titles giúp bạn định nghĩa ra một khuôn mẫu (Template) để ghép các thành phần (các mảnh jsp) lại với nhau để thành một trang web.
Hãy xem một template minh họa:
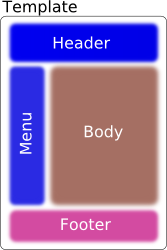
classic.jsp
<%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles"%>
<html>
<head>
<title><tiles:getAsString name="title" /></title>
</head>
<body>
<table width="100%">
<tr>
<td colspan="2">
<tiles:insertAttribute name="header" />
</td>
</tr>
<tr>
<td width="20%" nowrap="nowrap">
<tiles:insertAttribute name="menu" />
</td>
<td width="80%">
<tiles:insertAttribute name="body" />
</td>
</tr>
<tr>
<td colspan="2">
<tiles:insertAttribute name="footer" />
</td>
</tr>
</table>
</body>
</html>
4. Tạo Maven Project
Trên Eclipse chọn
- File/New/Other...
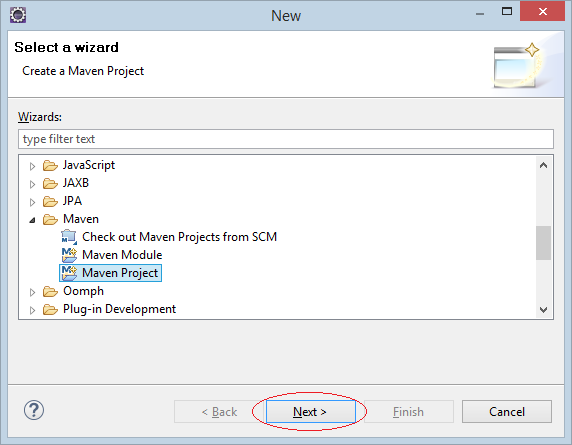
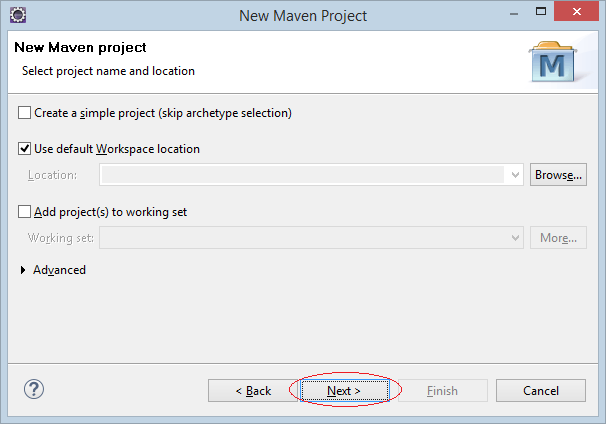
Chọn nguyên mẫu (archetype) "maven-archetype-webapp".
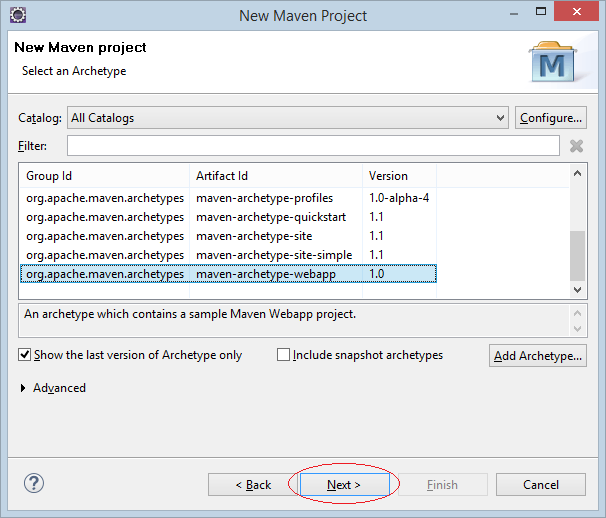
Nhập vào:
- Group Id: org.o7planning
- Artifact Id: SpringMVCTiles
- Package: org.o7planning.tutorial.springmvctiles
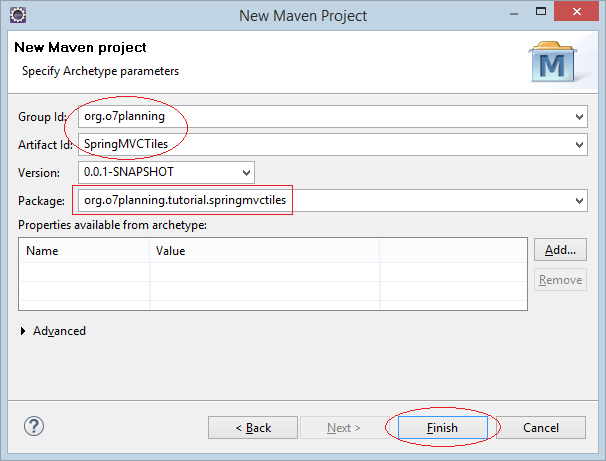
Đây là cấu trúc Project được tạo ra:
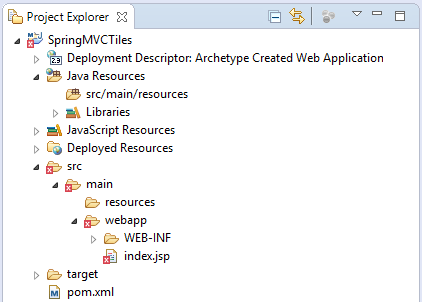
Đảm bảo rằng bạn sử dụng Java >= 6.
Project properties:
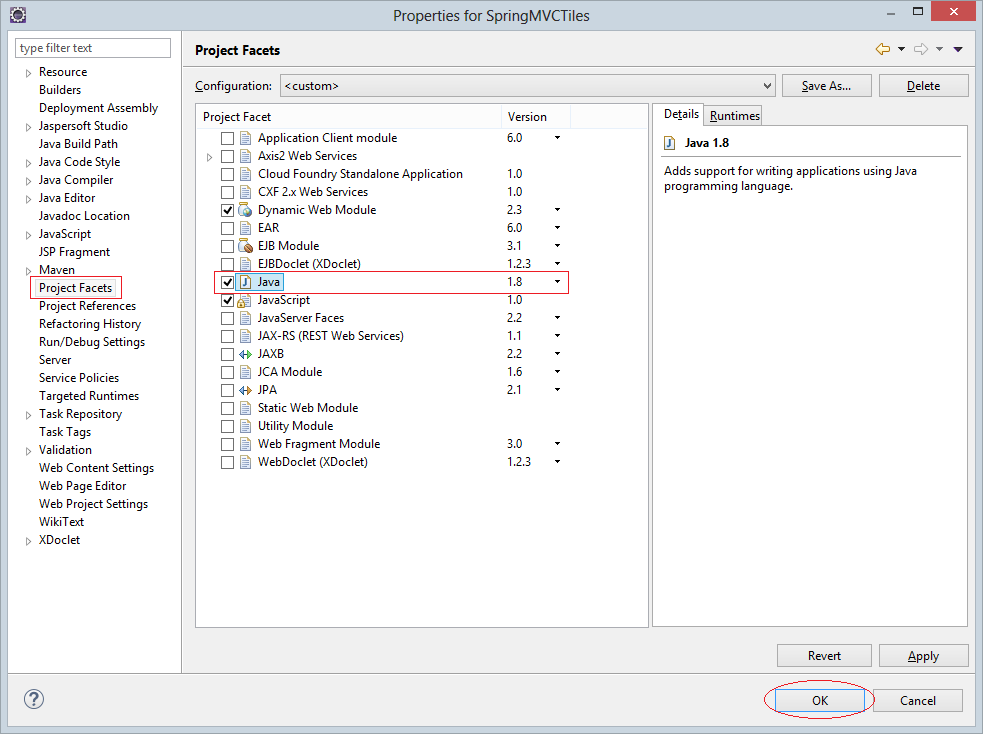
Bạn đừng lo lắng với thông báo lỗi khi Project vừa được tạo ra. Lý do là bạn chưa khai báo thư viện Servlet.
Eclipse tạo ra project Maven có thể sai cấu trúc. Bạn cần phải kiểm tra.
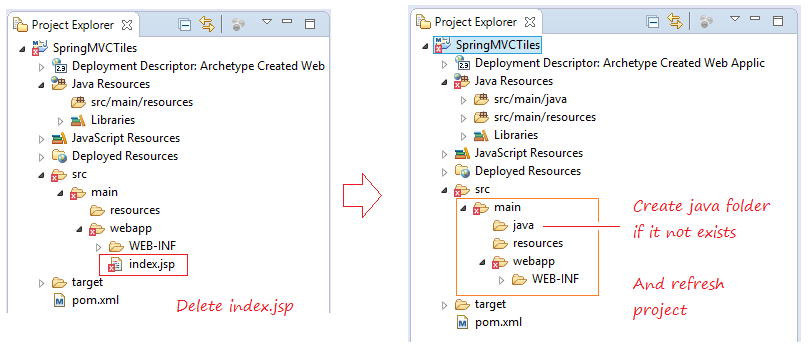
5. Khai báo maven & web.xml
Đảm bảo rằng bạn đang sử dụng Servlet API >= 3
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>SpringMVCTiles</display-name>
</web-app>
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringMVCTiles</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCTiles Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Servlet Library -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- JSP API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
<!-- Servlet JSP JSTL API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp.jstl/javax.servlet.jsp.jstl-api -->
<dependency>
<groupId>javax.servlet.jsp.jstl</groupId>
<artifactId>javax.servlet.jsp.jstl-api</artifactId>
<version>1.2.1</version>
</dependency>
<!-- Spring dependencies -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- Tiles API -->
<!-- http://mvnrepository.com/artifact/org.apache.tiles/tiles-api -->
<dependency>
<groupId>org.apache.tiles</groupId>
<artifactId>tiles-api</artifactId>
<version>3.0.7</version>
</dependency>
<!-- Tiles Core -->
<!-- http://mvnrepository.com/artifact/org.apache.tiles/tiles-core -->
<dependency>
<groupId>org.apache.tiles</groupId>
<artifactId>tiles-core</artifactId>
<version>3.0.7</version>
</dependency>
<!-- Tiles Servlet -->
<!-- http://mvnrepository.com/artifact/org.apache.tiles/tiles-servlet -->
<dependency>
<groupId>org.apache.tiles</groupId>
<artifactId>tiles-servlet</artifactId>
<version>3.0.7</version>
</dependency>
<!-- Tiles JSP -->
<!-- http://mvnrepository.com/artifact/org.apache.tiles/tiles-jsp -->
<dependency>
<groupId>org.apache.tiles</groupId>
<artifactId>tiles-jsp</artifactId>
<version>3.0.7</version>
</dependency>
</dependencies>
<build>
<finalName>SpringMVCTiles</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.3</version>
<!-- Must update Maven Project -->
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default - /SpringMVCTiles : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
6. Cấu hình Spring MVC
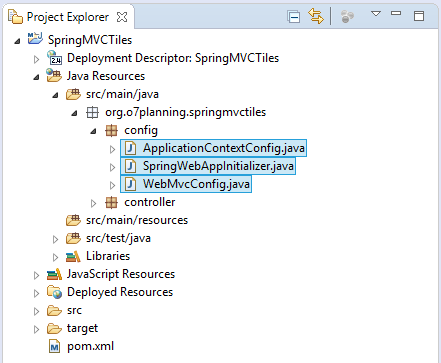
SpringWebAppInitializer.java
package org.o7planning.springmvctiles.config;
import javax.servlet.FilterRegistration;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRegistration;
import org.springframework.web.WebApplicationInitializer;
import org.springframework.web.context.support.AnnotationConfigWebApplicationContext;
import org.springframework.web.filter.CharacterEncodingFilter;
import org.springframework.web.servlet.DispatcherServlet;
public class SpringWebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
AnnotationConfigWebApplicationContext appContext = new AnnotationConfigWebApplicationContext();
appContext.register(ApplicationContextConfig.class);
ServletRegistration.Dynamic dispatcher = servletContext.addServlet("SpringDispatcher",
new DispatcherServlet(appContext));
dispatcher.setLoadOnStartup(1);
dispatcher.addMapping("/");
// UtF8 Charactor Filter.
FilterRegistration.Dynamic fr = servletContext.addFilter("encodingFilter", CharacterEncodingFilter.class);
fr.setInitParameter("encoding", "UTF-8");
fr.setInitParameter("forceEncoding", "true");
fr.addMappingForUrlPatterns(null, true, "/*");
}
}
Để Spring có thể tương tác với Apache Titles bạn cần phải khai báo 2 Spring BEAN là viewResolver và tilesConfigurer.
ApplicationContextConfig.java
package org.o7planning.springmvctiles.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.ViewResolver;
import org.springframework.web.servlet.view.UrlBasedViewResolver;
import org.springframework.web.servlet.view.tiles3.TilesConfigurer;
import org.springframework.web.servlet.view.tiles3.TilesView;
@Configuration
@ComponentScan("org.o7planning.springmvctiles.*")
public class ApplicationContextConfig {
@Bean(name = "viewResolver")
public ViewResolver getViewResolver() {
UrlBasedViewResolver viewResolver = new UrlBasedViewResolver();
// TilesView 3
viewResolver.setViewClass(TilesView.class);
return viewResolver;
}
@Bean(name = "tilesConfigurer")
public TilesConfigurer getTilesConfigurer() {
TilesConfigurer tilesConfigurer = new TilesConfigurer();
// TilesView 3
tilesConfigurer.setDefinitions("/WEB-INF/tiles.xml");
return tilesConfigurer;
}
}
WebMvcConfig.java
package org.o7planning.springmvctiles.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
@EnableWebMvc
public class WebMvcConfig extends WebMvcConfigurerAdapter {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
// Default..
}
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
}
7. Cấu hình Apache Tiles
tiles.xml định nghĩa các trang hoàn chỉnh. Một trang hoàn chỉnh được ghép bởi các mảnh jsp khác nhau.
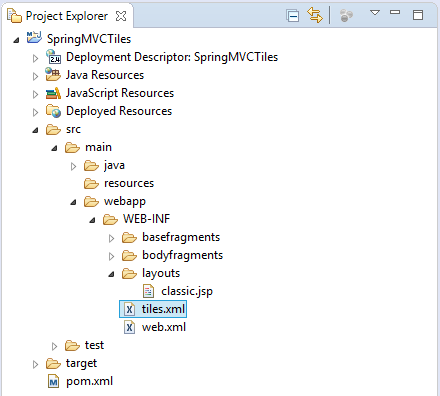
/WEB-INF/tiles.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE tiles-definitions PUBLIC
"-//Apache Software Foundation//DTD Tiles Configuration 3.0//EN"
"http://tiles.apache.org/dtds/tiles-config_3_0.dtd">
<tiles-definitions>
<!-- Base Define -->
<definition name="base.definition"
template="/WEB-INF/layouts/classic.jsp">
<put-attribute name="title" value="" />
<put-attribute name="header" value="/WEB-INF/basefragments/_header.jsp" />
<put-attribute name="menu" value="/WEB-INF/basefragments/_menu.jsp" />
<put-attribute name="body" value="" />
<put-attribute name="footer" value="/WEB-INF/basefragments/_footer.jsp" />
</definition>
<!-- Home Page -->
<definition name="homePage" extends="base.definition">
<put-attribute name="title" value="Home Page" />
<put-attribute name="body" value="/WEB-INF/bodyfragments/_home.jsp" />
</definition>
<!-- ContactUs Page -->
<definition name="contactusPage" extends="base.definition">
<put-attribute name="title" value="Contact Us" />
<put-attribute name="body" value="/WEB-INF/bodyfragments/_contactus.jsp" />
</definition>
</tiles-definitions>
8. Tiles Layout
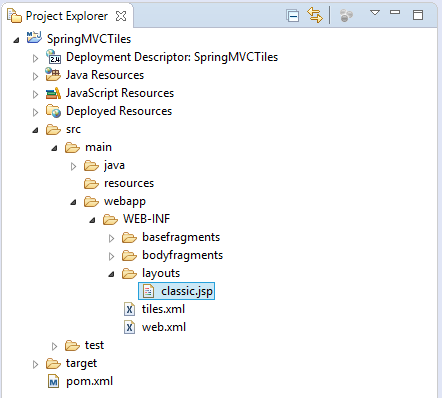
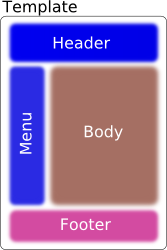
/WEB-INF/layouts/classic.jsp
<%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles"%>
<html>
<head>
<title><tiles:getAsString name="title" /></title>
</head>
<body>
<table width="100%">
<tr>
<td colspan="2">
<tiles:insertAttribute name="header" />
</td>
</tr>
<tr>
<td width="20%" nowrap="nowrap">
<tiles:insertAttribute name="menu" />
</td>
<td width="80%">
<tiles:insertAttribute name="body" />
</td>
</tr>
<tr>
<td colspan="2">
<tiles:insertAttribute name="footer" />
</td>
</tr>
</table>
</body>
</html>
9. JSP Fragments
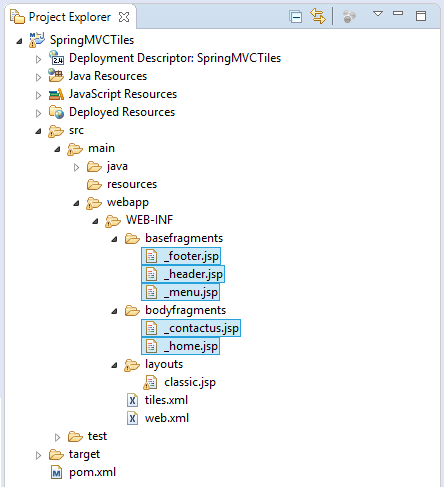
_header.jsp
<div style="background: #E0E0E0; height: 55px; padding: 5px;">
<div style="float: left">
<h1>My Site</h1>
</div>
<div style="float: right; padding: 10px; text-align: right;">
Search <input name="search">
</div>
</div>
_menu.jsp
<div style="padding: 5px;">
<ul>
<li><a href="${pageContext.request.contextPath}/">Home</a></li>
<li><a href="${pageContext.request.contextPath}/contactus">Contact Us</a></li>
</ul>
</div>
_footer.jsp
<div
style="background: #E0E0E0; text-align: center; padding: 5px; margin-top: 10px;">
@Copyright o7planning.org
</div>
_home.jsp
<h2>Hi All</h2>
This is Home Page
_contactus.jsp
Contact Us: o7planning.org
<br>
Address: ${address}
<br>
Phone: ${phone}
<br>
Email: ${email}
10. Spring MVC Controller
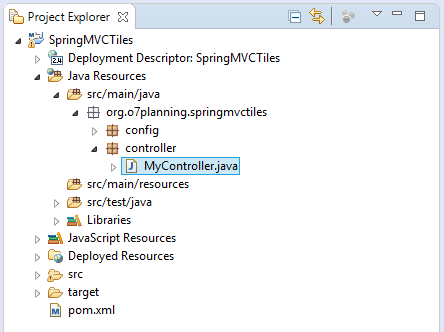
MyController.java
package org.o7planning.springmvctiles.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MyController {
@RequestMapping(value = { "/", "/home" }, method = RequestMethod.GET)
public String homePage(Model model) {
return "homePage";
}
@RequestMapping(value = { "/contactus" }, method = RequestMethod.GET)
public String contactusPage(Model model) {
model.addAttribute("address", "Vietnam");
model.addAttribute("phone", "...");
model.addAttribute("email", "...");
return "contactusPage";
}
}
11. Chạy ứng dụng
Trong lần đầu tiên, trước khi chạy ứng dụng bạn cần phải build toàn bộ project.
Nhấn phải chuột vào project chọn:
- Run As/Maven install
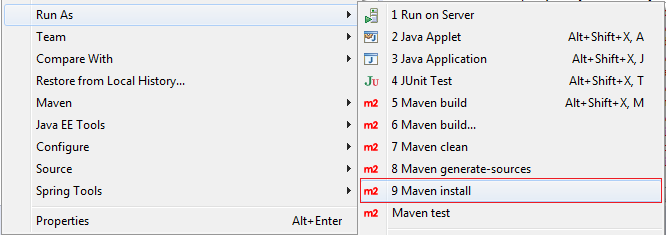
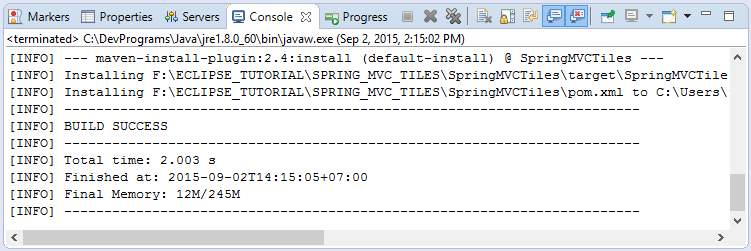
Cấu hình để chạy ứng dụng:
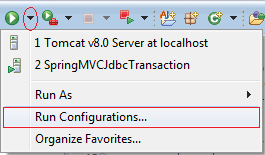
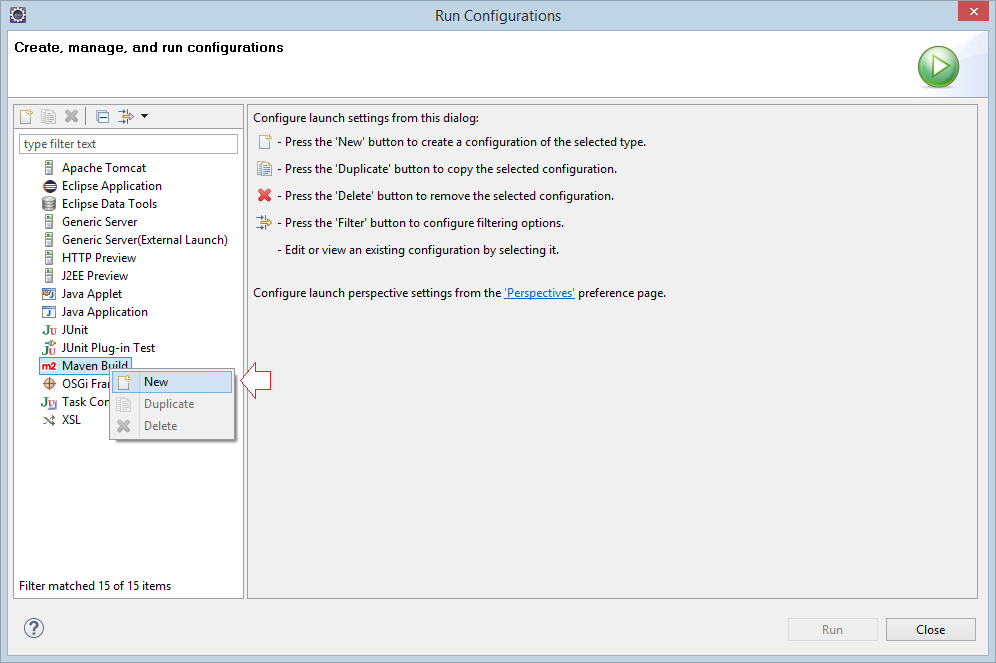
Nhập vào:
- Name: Run SpringMVCTiles
- Base directory: ${workspace_loc:/SpringMVCTiles}
- Goals: tomcat7:run
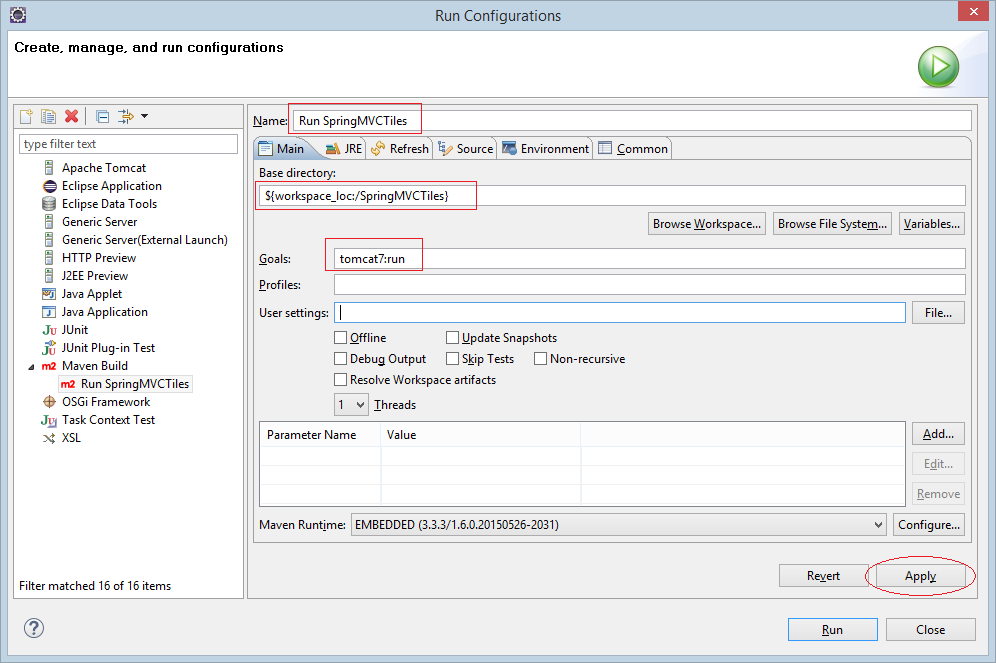
Nhấn Run để chạy:
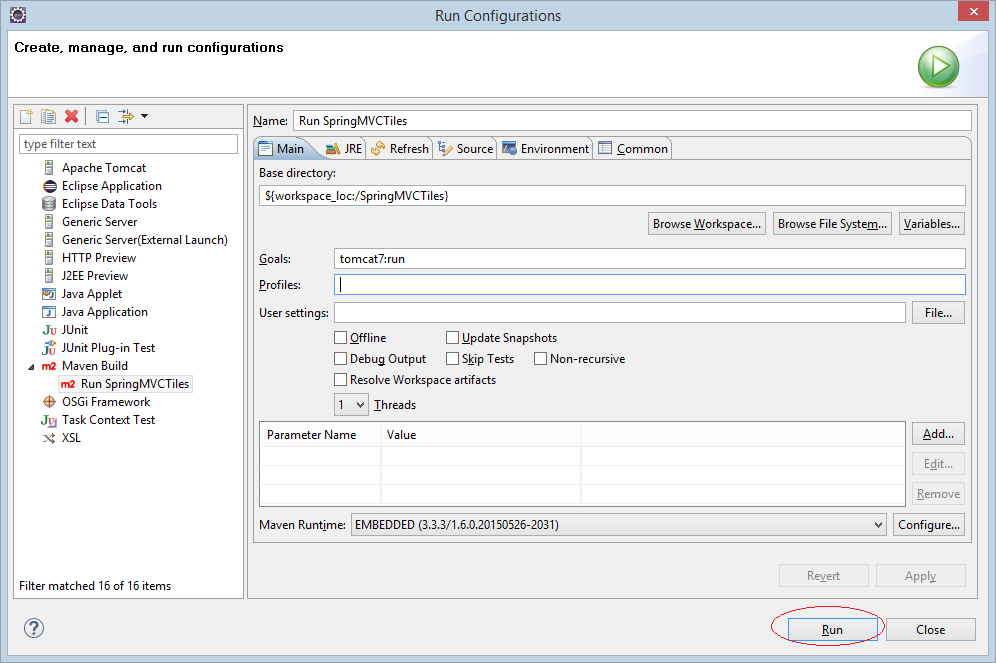
Trong lần chạy đầu tiên sẽ hơi lâu do chương trình download các thư viện tomcat plugin về để chạy.
Mọi thứ đã hoàn thành:
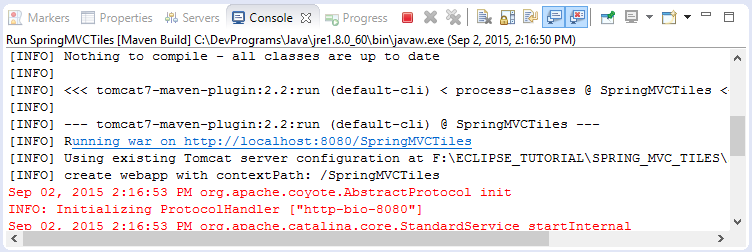
Chạy URL:
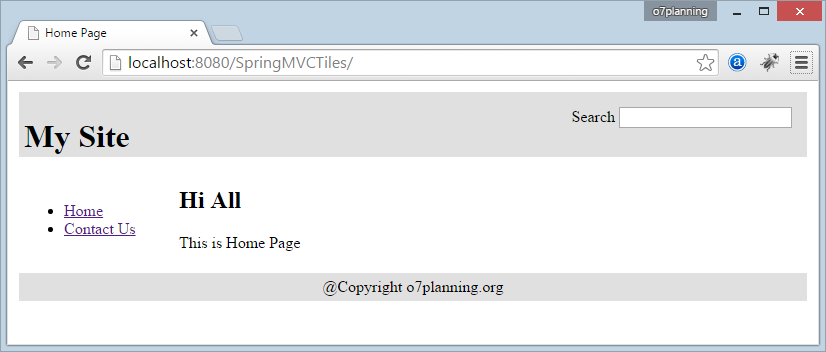
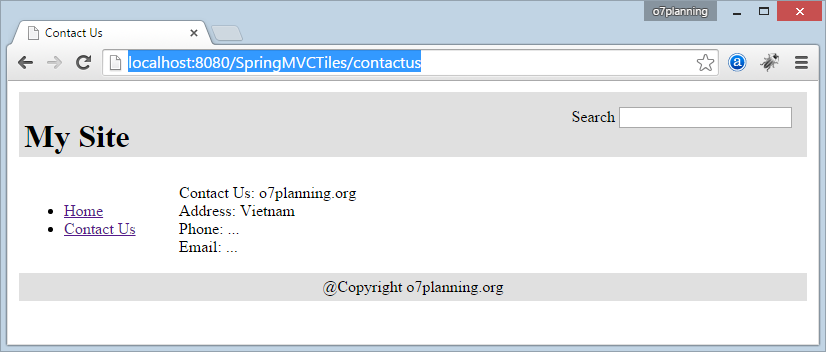
Các hướng dẫn Spring MVC
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Hướng dẫn lập trình Spring MVC cho người mới bắt đầu - Hello Spring 4 MVC
- Cài đặt Spring Tool Suite cho Eclipse
- Cấu hình các nguồn dữ liệu tĩnh trong Spring MVC
- Hướng dẫn sử dụng Spring MVC Interceptor
- Tạo ứng dụng web đa ngôn ngữ với Spring MVC
- Hướng dẫn Upload File với Spring MVC
- Ứng dụng Java Web login đơn giản sử dụng Spring MVC, Spring Security và Spring JDBC
- Hướng dẫn sử dụng Spring MVC Security với Hibernate
- Hướng dẫn sử dụng Spring MVC Security và Spring JDBC (XML Config)
- Đăng nhập bằng mạng xã hội trong Spring MVC với Spring Social Security
- Hướng dẫn sử dụng Spring MVC và Velocity
- Hướng dẫn sử dụng Spring MVC với FreeMarker
- Sử dụng Template trong Spring MVC với Apache Tiles
- Hướng dẫn sử dụng Spring MVC và Spring JDBC Transaction
- Sử dụng nhiều DataSource trong Spring MVC
- Hướng dẫn sử dụng Spring MVC, Hibernate và Spring Transaction Manager
- Hướng dẫn sử dụng Spring MVC Form và Hibernate
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Tạo một ứng dụng Java Web bán hàng sử dụng Spring MVC và Hibernate
- Ví dụ CRUD đơn giản với Spring MVC RESTful Web Service
- Triển khai ứng dụng Spring MVC trên Oracle WebLogic Server
Show More