Cấu hình các nguồn dữ liệu tĩnh trong Spring MVC
1. Mục tiêu của tài liệu
Tài liệu được viết dựa trên:
Eclipse 4.6 (NEON)
Spring 4 MVC
Trong tài liệu này tôi sẽ hướng dẫn bạn cấu hình các nguồn dữ liệu tĩnh trong Spring MVC, các nguồn dữ liệu tĩnh thông thường là các file ảnh (image), các file css, và javascript,...
Spring MVC cho phép bạn ánh xạ giữa một URL với một vị trí thực tế của nguồn dữ liệu. Bạn có thể xem hình minh họa dưới đây:
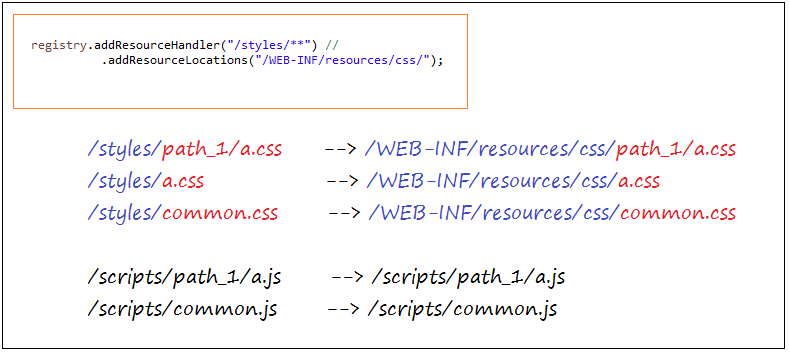
2. Tạo Maven Project
- File/New/Other..
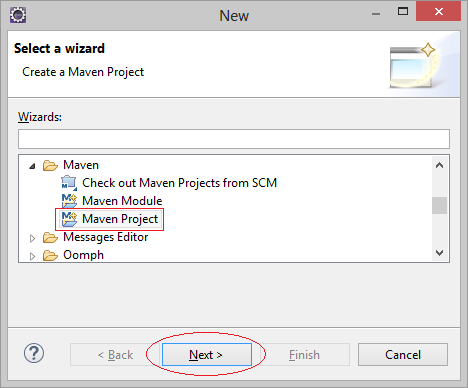
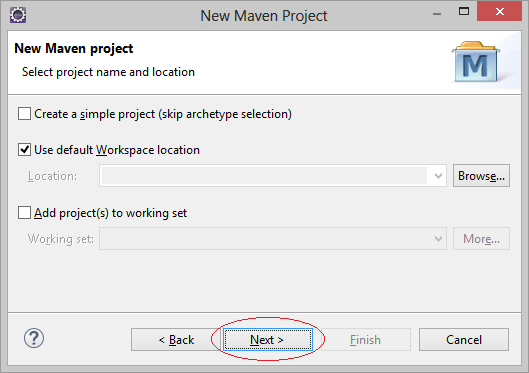
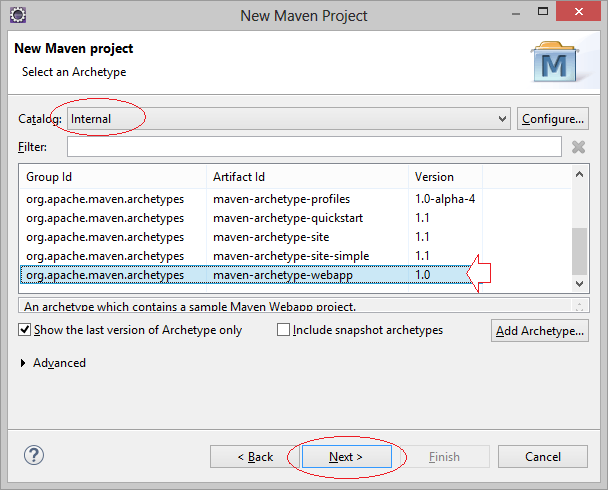
Nhập vào:
- Group ID: org.o7planning
- Artifact ID: SpringMVCStaticResource
- Package: org.o7planning.tutorial.springmvcresource
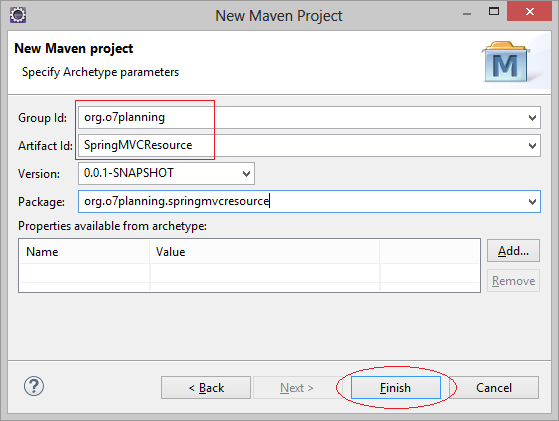
Project đã được tạo ra.
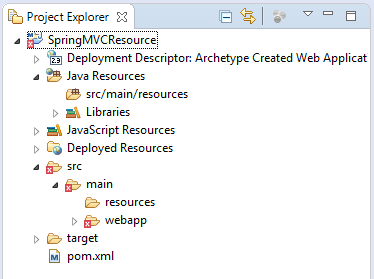
Bạn đừng lo lắng với thông báo lỗi khi Project vừa được tạo ra. Lý do là bạn chưa khai báo thư viện Servlet.
Chú ý:
Eclipse tạo ra project Maven có thể sai cấu trúc. Bạn cần phải kiểm tra lại và sửa lỗi.
Eclipse tạo ra project Maven có thể sai cấu trúc. Bạn cần phải kiểm tra lại và sửa lỗi.
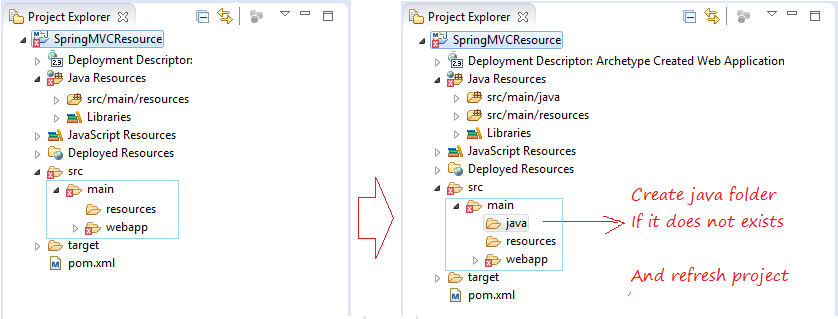
Đảm bảo rằng project của bạn sử dụng Java >=6.
Project properties:
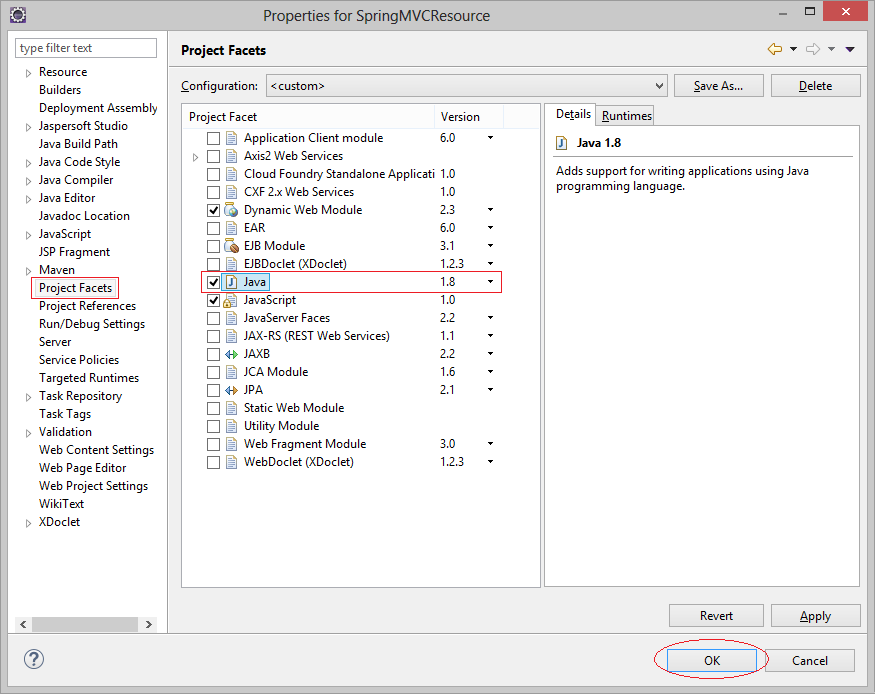
3. Cấu hình Maven & web.xml
Cấu hình web.xml sử dụng Web App >= 3.
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>SpringMVCResource</display-name>
</web-app>
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringMVCResource</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SpringMVCResource Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Servlet API -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- Jstl for jsp page -->
<!-- http://mvnrepository.com/artifact/javax.servlet/jstl -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- JSP API -->
<!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.2</version>
<scope>provided</scope>
</dependency>
<!-- Spring dependencies -->
<!-- http://mvnrepository.com/artifact/org.springframework/spring-core -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-web -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.3.RELEASE</version>
</dependency>
</dependencies>
<build>
<finalName>SpringMVCResource</finalName>
<plugins>
<!-- Config: Maven Tomcat Plugin -->
<!-- http://mvnrepository.com/artifact/org.apache.tomcat.maven/tomcat7-maven-plugin -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
<!-- Config: contextPath and Port (Default - /SpringMVCResource : 8080) -->
<!--
<configuration>
<path>/</path>
<port>8899</port>
</configuration>
-->
</plugin>
</plugins>
</build>
</project>
4. Cấu hình Spring MVC
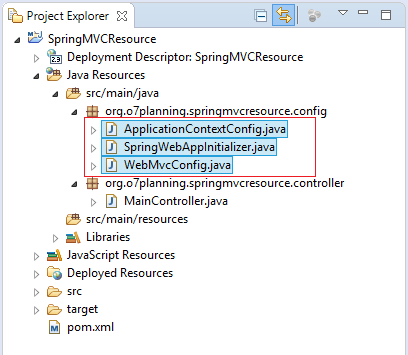
SpringWebAppInitializer.java
package org.o7planning.springmvcresource.config;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRegistration;
import org.springframework.web.WebApplicationInitializer;
import org.springframework.web.context.support.AnnotationConfigWebApplicationContext;
import org.springframework.web.servlet.DispatcherServlet;
public class SpringWebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
AnnotationConfigWebApplicationContext appContext = new AnnotationConfigWebApplicationContext();
appContext.register(ApplicationContextConfig.class);
ServletRegistration.Dynamic dispatcher = servletContext.addServlet("SpringDispatcher",
new DispatcherServlet(appContext));
dispatcher.setLoadOnStartup(1);
dispatcher.addMapping("/");
}
}
ApplicationContextConfig.java
package org.o7planning.springmvcresource.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@ComponentScan("org.o7planning.springmvcresource.*")
public class ApplicationContextConfig {
@Bean(name = "viewResolver")
public InternalResourceViewResolver getViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix("/WEB-INF/pages/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
}
WebMvcConfig.java
package org.o7planning.springmvcresource.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
@EnableWebMvc
public class WebMvcConfig extends WebMvcConfigurerAdapter {
// Cấu hình để sử dụng các file nguồn tĩnh (html, image, ..)
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
// Css resource.
registry.addResourceHandler("/styles/**") //
.addResourceLocations("/WEB-INF/resources/css/").setCachePeriod(31556926);
}
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
}
Một số chú ý:
Cấu hình Static Resource:
// Css resource.
registry.addResourceHandler("/styles/**") //
.addResourceLocations("/WEB-INF/resources/css/");
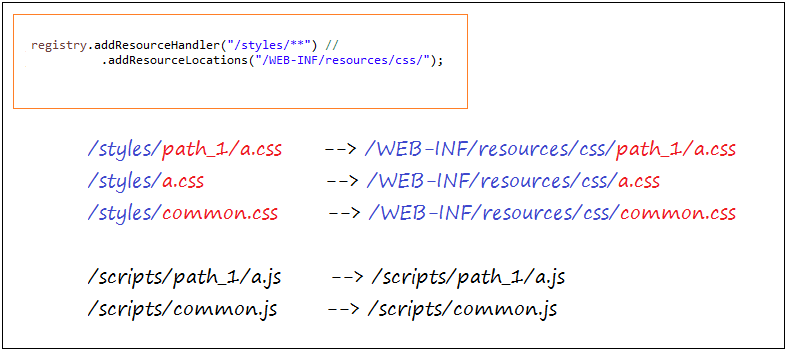
5. Spring Controllers
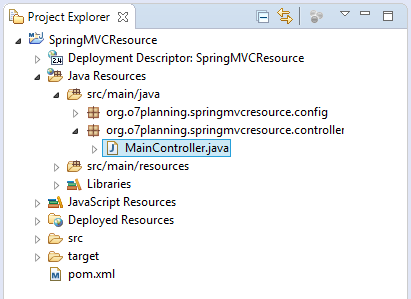
MyController.java
package org.o7planning.springmvcresource.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@RequestMapping(value = "/staticResourceTest")
public String staticResource(Model model) {
return "staticResourceTest";
}
}
6. Static Resource & Views
Static Resource
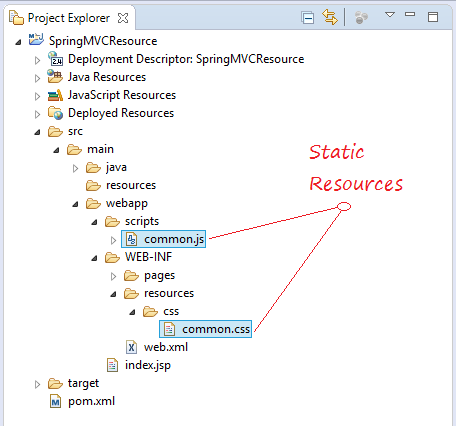
scripts/common.js
function sayHello() {
alert("Hello from JavaScript");
}
/WEB-INF/resource/css/commons.css
.button {
font-size: 20px;
background: #ccc;
}
.red-text {
color: red;
font-size: 30px;
}
.green-text {
color: green;
font-size: 20px;
}
Views
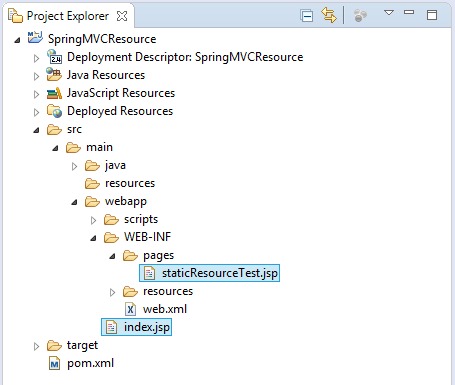
staticResourceTest.jsp
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Spring MVC Resource example</title>
<script type="text/javascript"
src="${pageContext.request.contextPath}/scripts/common.js"></script>
<link rel="stylesheet" type="text/css"
href="${pageContext.request.contextPath}/styles/common.css">
</head>
<body>
<pre>
Config: /styles/** ==> /WEB-INF/resources/css/
----------------------------------------------
/styles/common.css ==> /WEB-INF/resources/css/common.css
/styles/path1/abc.css ==> /WEB-INF/resources/css/path1/abc.css
/styles/path1/path2/abc.css ==> /WEB-INF/resources/css/path1/path2/abc.css
</pre>
<div class="red-text">Red text</div>
<br>
<div class="green-text">Green text</div>
<br>
<input type="button" class="button" onclick="sayHello();"
value="Click me!">
</body>
</html>
index.jsp
<html>
<body>
<a href="staticResourceTest">staticResourceTest</a>
</body>
</html>
Các hướng dẫn Spring MVC
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Cài đặt Spring Tool Suite cho Eclipse
- Hướng dẫn lập trình Spring MVC cho người mới bắt đầu - Hello Spring 4 MVC
- Cấu hình các nguồn dữ liệu tĩnh trong Spring MVC
- Hướng dẫn sử dụng Spring MVC Interceptor
- Tạo ứng dụng web đa ngôn ngữ với Spring MVC
- Hướng dẫn Upload File với Spring MVC
- Ứng dụng Java Web login đơn giản sử dụng Spring MVC, Spring Security và Spring JDBC
- Hướng dẫn sử dụng Spring MVC Security với Hibernate
- Hướng dẫn sử dụng Spring MVC Security và Spring JDBC (XML Config)
- Đăng nhập bằng mạng xã hội trong Spring MVC với Spring Social Security
- Hướng dẫn sử dụng Spring MVC và Velocity
- Hướng dẫn sử dụng Spring MVC với FreeMarker
- Sử dụng Template trong Spring MVC với Apache Tiles
- Hướng dẫn sử dụng Spring MVC và Spring JDBC Transaction
- Sử dụng nhiều DataSource trong Spring MVC
- Hướng dẫn sử dụng Spring MVC, Hibernate và Spring Transaction Manager
- Hướng dẫn sử dụng Spring MVC Form và Hibernate
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Tạo một ứng dụng Java Web bán hàng sử dụng Spring MVC và Hibernate
- Ví dụ CRUD đơn giản với Spring MVC RESTful Web Service
- Triển khai ứng dụng Spring MVC trên Oracle WebLogic Server
Show More