Tạo ứng dụng web đa ngôn ngữ với Spring MVC
1. Mục tiêu của tài liệu
Đôi khi bạn cần làm một website đa ngữ, một website đa ngữ giúp nó tới được với nhiều đối tượng người dùng hơn. Website đa ngữ cũng được biết tới với tên gọi Internationalization (i18n) (Quốc tế hóa), nó trái ngược với Localization (L10n) (Nội địa hóa).
Chú ý: Internationalization là một từ có 18 ký tự, ký tự đầu tiên là i và ký tự cuối cùng là n, vì vậy nó thường được viết tắt là i18n.
Spring cung cấp các hỗ trợ mở rộng cho quốc tế hóa (Internationalization) (i18n) thông qua việc sử dụng Spring Interceptor, Locale Resolvers và Resource Bundles cho các địa phương khác nhau.
Trong tài liệu này tôi hướng dẫn bạn làm một website đa ngữ đơn giản sử dụng Spring MVC.
Bạn có thể xem trước ví dụ sẽ làm:
Trong ví dụ này thông tin địa phương (Locale) nằm trên tham số của URL. Thông tin Locale sẽ được lưu trữ lại ở Cookie, các trang tiếp theo người dùng không phải lựa chọn lại ngôn ngữ.
- http://localhost:8080/SpringMVCInternationalization/login1?lang=vi
- http://localhost:8080/SpringMVCInternationalization/login1?lang=fr
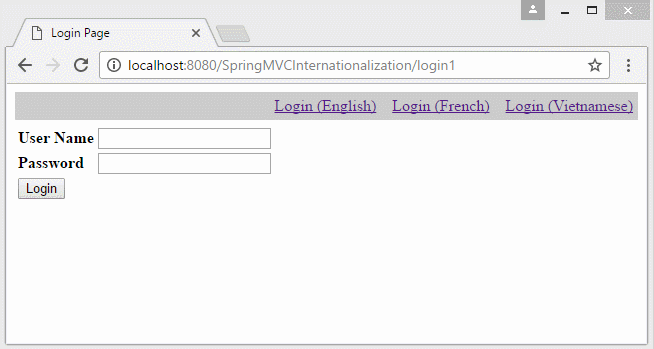
Một ví dụ khác với thông tin Locale nằm trên URL:
- http://localhost:8080/SpringMVCInternationalization/vi/login2
- http://localhost:8080/SpringMVCInternationalization/en/login2
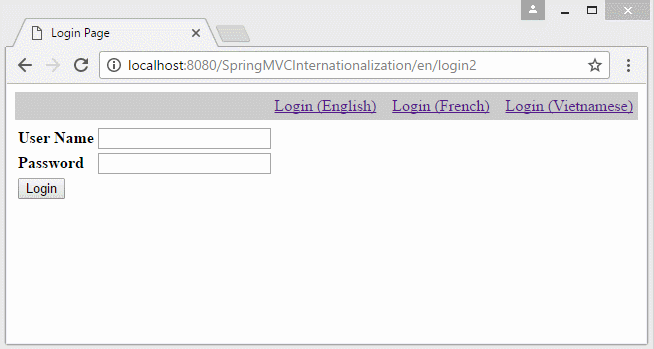
2. Tạo Maven Project
- File/New/Other..
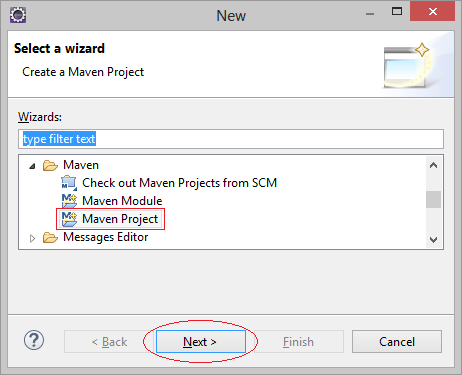
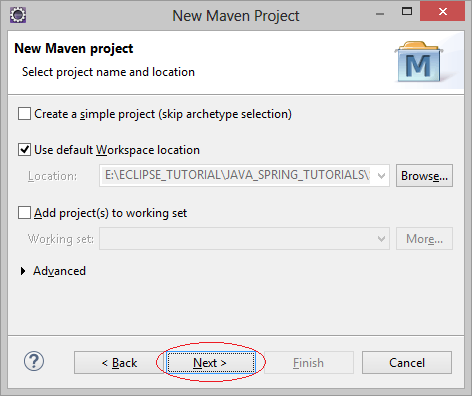
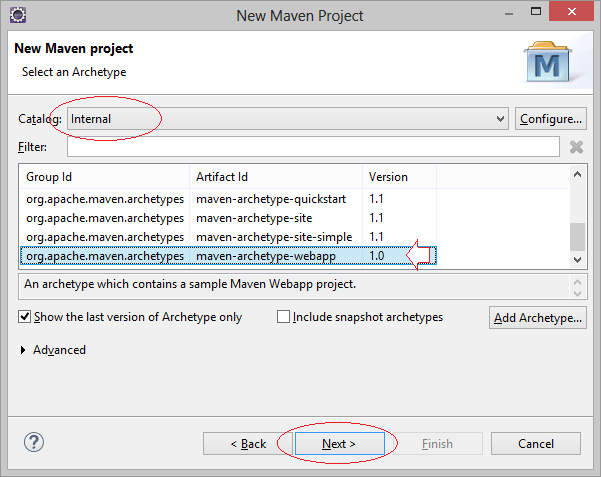
- Group Id: org.o7planning
- Artifact Id: SpringMVCInternationalization
- Package: org.o7planning.springmvcinternationalization
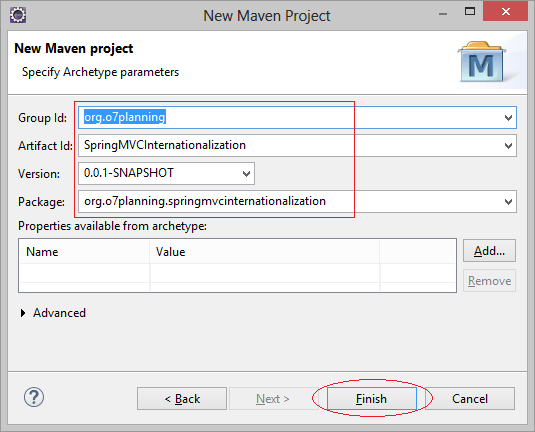
Project đã được tạo ra:
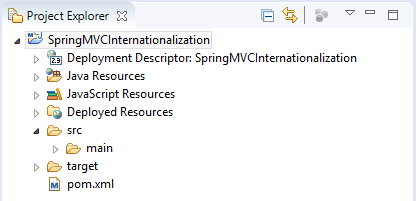
Đảm bảo project của bạn sử dụng Java >= 6.
Project Properties:
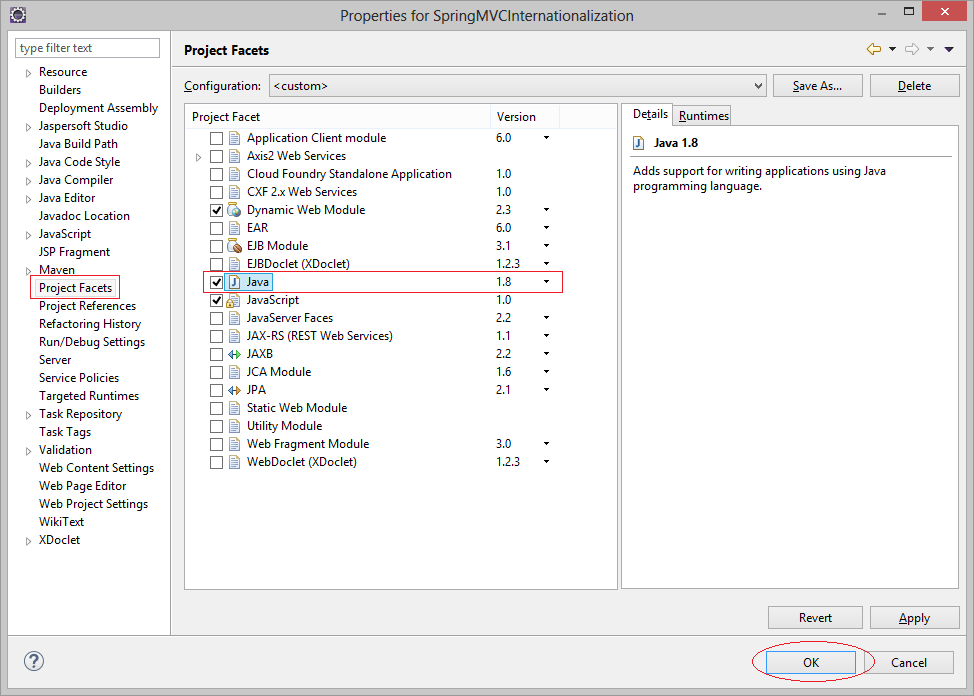
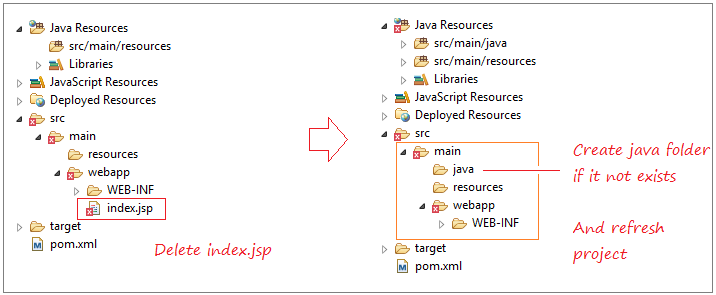
3. Message Resources
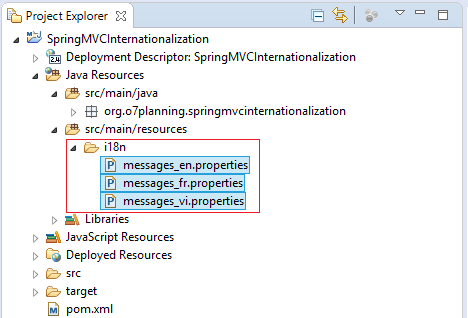
Ở đây tôi tạo ra 3 file properties cho các ngôn ngữ tiếng Anh, Pháp và Việt Nam. Các file này sẽ được tải (load), và quản lý bởi messageResource Bean.
i18n/messages_en.properties
#Generated by Eclipse Messages Editor (Eclipse Babel)
label.password = Password
label.submit = Login
label.title = Login Page
label.userName = User Name
i18n/messages_fr.properties
#Generated by Eclipse Messages Editor (Eclipse Babel)
label.password = Mot de passe
label.submit = Connexion
label.title = Connectez-vous page
label.userName = Nom d'utilisateur
i18n/messages_vi.properties
#Generated by Eclipse Messages Editor (Eclipse Babel)
label.password = M\u1EADt kh\u1EA9u
label.submit = \u0110\u0103ng nh\u1EADp
label.title = Trang \u0111\u0103ng nh\u1EADp
label.userName = T\u00EAn ng\u01B0\u1EDDi d\u00F9ng
Eclipse hỗ trợ bạn sửa đổi nội dung các file này bằng cách sử dụng "Message Editor".
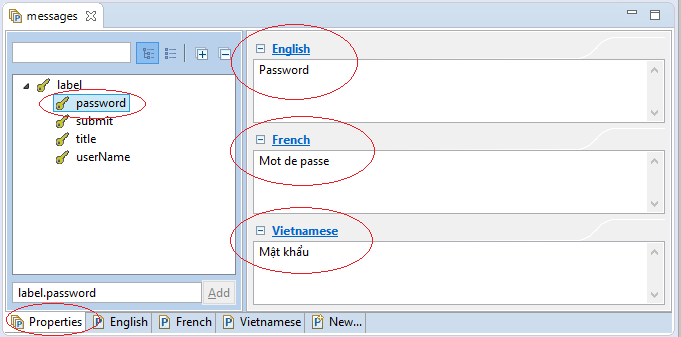
4. Cấu hình Spring MVC
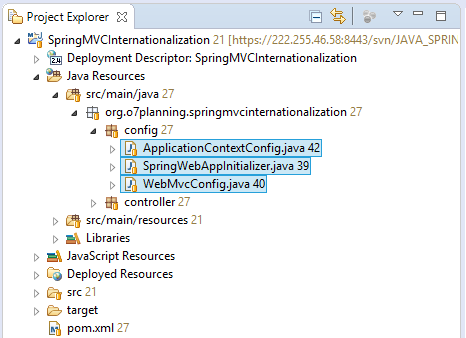
Bạn đang làm một website đa ngôn ngữ, vì vậy bạn cần sử dụng mã hóa UTF-8.
SpringWebAppInitializer.java
package org.o7planning.springmvcinternationalization.config;
import javax.servlet.FilterRegistration;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRegistration;
import org.springframework.web.WebApplicationInitializer;
import org.springframework.web.context.support.AnnotationConfigWebApplicationContext;
import org.springframework.web.filter.CharacterEncodingFilter;
import org.springframework.web.servlet.DispatcherServlet;
public class SpringWebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
AnnotationConfigWebApplicationContext appContext = new AnnotationConfigWebApplicationContext();
appContext.register(ApplicationContextConfig.class);
ServletRegistration.Dynamic dispatcher = servletContext.addServlet("SpringDispatcher",
new DispatcherServlet(appContext));
dispatcher.setLoadOnStartup(1);
dispatcher.addMapping("/");
// UtF8 Charactor Filter.
FilterRegistration.Dynamic fr = servletContext.addFilter("encodingFilter", CharacterEncodingFilter.class);
fr.setInitParameter("encoding", "UTF-8");
fr.setInitParameter("forceEncoding", "true");
fr.addMappingForUrlPatterns(null, true, "/*");
}
}
Bạn cần khai báo 2 Spring BEAN là localeResolver và messageResource.
localeResolver - Chỉ định cách lấy thông tin địa phương (Locale) mà người dùng sẽ sử dụng. CookieLocaleResolver sẽ đọc thông tin Locale từ Cookie, để biết người dùng trước đó đã sử dụng trang web với ngôn ngữ nào.
messageResource - Sẽ tải nội dung các file properties.
localeResolver - Chỉ định cách lấy thông tin địa phương (Locale) mà người dùng sẽ sử dụng. CookieLocaleResolver sẽ đọc thông tin Locale từ Cookie, để biết người dùng trước đó đã sử dụng trang web với ngôn ngữ nào.
messageResource - Sẽ tải nội dung các file properties.
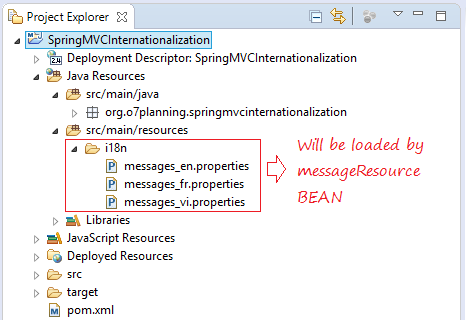
ApplicationContextConfig.java
package org.o7planning.springmvcinternationalization.config;
import org.springframework.context.MessageSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.support.ReloadableResourceBundleMessageSource;
import org.springframework.web.servlet.LocaleResolver;
import org.springframework.web.servlet.i18n.CookieLocaleResolver;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@ComponentScan("org.o7planning.springmvcinternationalization.*")
public class ApplicationContextConfig {
@Bean(name = "viewResolver")
public InternalResourceViewResolver getViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix("/WEB-INF/pages/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
@Bean(name = "messageSource")
public MessageSource getMessageResource() {
ReloadableResourceBundleMessageSource messageResource= new ReloadableResourceBundleMessageSource();
// Đọc vào file i18n/messages_xxx.properties
// Ví dụ: i18n/message_en.properties
messageResource.setBasename("classpath:i18n/messages");
messageResource.setDefaultEncoding("UTF-8");
return messageResource;
}
@Bean(name = "localeResolver")
public LocaleResolver getLocaleResolver() {
CookieLocaleResolver resolver= new CookieLocaleResolver();
resolver.setCookieDomain("myAppLocaleCookie");
// 60 phút.
resolver.setCookieMaxAge(60*60);
return resolver;
}
}
Trước khi request được xử lý bởi Controller, nó phải đi qua các Interceptors, ở đây bạn cần đăng ký LocaleChangeInterceptor, Interceptor này xử lý các thay đổi Locale từ phía người dùng.
WebMvcConfig.java
package org.o7planning.springmvcinternationalization.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
import org.springframework.web.servlet.i18n.LocaleChangeInterceptor;
@Configuration
@EnableWebMvc
public class WebMvcConfig extends WebMvcConfigurerAdapter {
// Cấu hình để sử dụng các file nguồn tĩnh (html, image, ..)
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
// Default..
}
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
@Override
public void addInterceptors(InterceptorRegistry registry) {
LocaleChangeInterceptor localeInterceptor = new LocaleChangeInterceptor();
localeInterceptor.setParamName("lang");
registry.addInterceptor(localeInterceptor).addPathPatterns("/*");
}
}
5. Controller & Views
MainController.java
package org.o7planning.springmvcinternationalization.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@RequestMapping(value = "/login1")
public String login1(Model model) {
return "login1";
}
}
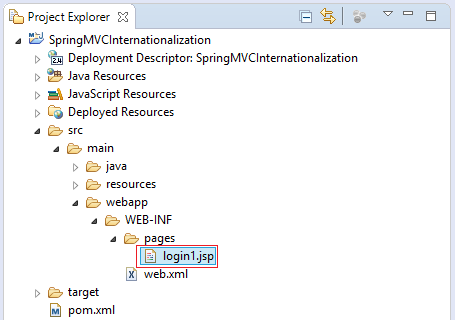
login1.jsp
<%@taglib uri="http://www.springframework.org/tags" prefix="spring"%>
<%@ page session="false"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title><spring:message code="label.title" /></title>
</head>
<body>
<div style="text-align: right;padding:5px;margin:5px 0px;background:#ccc;">
<a href="${pageContext.request.contextPath}/login1?lang=en">Login (English)</a>
<a href="${pageContext.request.contextPath}/login1?lang=fr">Login (French)</a>
<a href="${pageContext.request.contextPath}/login1?lang=vi">Login (Vietnamese)</a>
</div>
<form method="post" action="">
<table>
<tr>
<td>
<strong>
<spring:message code="label.userName" />
</strong>
</td>
<td><input name="userName" /></td>
</tr>
<tr>
<td>
<strong>
<spring:message code="label.password" />
</strong>
</td>
<td><input name="password" /></td>
</tr>
<tr>
<td colspan="2">
<spring:message code="label.submit" var="labelSubmit"></spring:message>
<input type="submit" value="${labelSubmit}" />
</td>
</tr>
</table>
</form>
</body>
</html>
7. Thông tin Locale trên URL
Trong trường hợp bạn muốn xây dựng một website đa ngữ mà thông tin Locale nằm trên URL. Bạn cần thay đổi một vài cấu hình:
- http://localhost:8080/SpringMVCInternationalization/vi/login2
- http://localhost:8080/SpringMVCInternationalization/en/login2
Tạo 2 lớp UrlLocaleInterceptor và UrlLocaleResolver.
UrlLocaleInterceptor.java
package org.o7planning.springmvcinternationalization.interceptor;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.LocaleResolver;
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
import org.springframework.web.servlet.support.RequestContextUtils;
public class UrlLocaleInterceptor extends HandlerInterceptorAdapter {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
LocaleResolver localeResolver = RequestContextUtils.getLocaleResolver(request);
if (localeResolver == null) {
throw new IllegalStateException("No LocaleResolver found: not in a DispatcherServlet request?");
}
// Lấy ra thông tin Locale từ LocaleResolver
Locale locale = localeResolver.resolveLocale(request);
localeResolver.setLocale(request, response, locale);
return true;
}
}
UrlLocaleResolver.java
package org.o7planning.springmvcinternationalization.resolver;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.LocaleResolver;
public class UrlLocaleResolver implements LocaleResolver {
private static final String URL_LOCALE_ATTRIBUTE_NAME = "URL_LOCALE_ATTRIBUTE_NAME";
@Override
public Locale resolveLocale(HttpServletRequest request) {
// ==> /SpringMVCInternationalization/en/...
// ==> /SpringMVCInternationalization/fr/...
// ==> /SpringMVCInternationalization/WEB-INF/pages/...
String uri = request.getRequestURI();
System.out.println("URI=" + uri);
String prefixEn = request.getServletContext().getContextPath() + "/en/";
String prefixFr = request.getServletContext().getContextPath() + "/fr/";
String prefixVi = request.getServletContext().getContextPath() + "/vi/";
Locale locale = null;
// English
if (uri.startsWith(prefixEn)) {
locale = Locale.ENGLISH;
}
// French
else if (uri.startsWith(prefixFr)) {
locale = Locale.FRANCE;
}
// Vietnamese
else if (uri.startsWith(prefixVi)) {
locale = new Locale("vi", "VN");
}
if (locale != null) {
request.getSession().setAttribute(URL_LOCALE_ATTRIBUTE_NAME, locale);
}
if (locale == null) {
locale = (Locale) request.getSession().getAttribute(URL_LOCALE_ATTRIBUTE_NAME);
if (locale == null) {
locale = Locale.ENGLISH;
}
}
return locale;
}
@Override
public void setLocale(HttpServletRequest request, HttpServletResponse response, Locale locale) {
// Nothing
}
}
Sửa đổi lớp ApplicationContextConfig:
ApplicationContextConfig.java
package org.o7planning.springmvcinternationalization.config;
import org.o7planning.springmvcinternationalization.resolver.UrlLocaleResolver;
import org.springframework.context.MessageSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.support.ReloadableResourceBundleMessageSource;
import org.springframework.web.servlet.LocaleResolver;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
@Configuration
@ComponentScan("org.o7planning.springmvcinternationalization.*")
public class ApplicationContextConfig {
@Bean(name = "viewResolver")
public InternalResourceViewResolver getViewResolver() {
InternalResourceViewResolver viewResolver = new InternalResourceViewResolver();
viewResolver.setPrefix("/WEB-INF/pages/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
@Bean(name = "messageSource")
public MessageSource getMessageResource() {
ReloadableResourceBundleMessageSource messageResource= new ReloadableResourceBundleMessageSource();
// Đọc vào file i18n/messages_xxx.properties
// Ví dụ: i18n/message_en.properties
messageResource.setBasename("classpath:i18n/messages");
messageResource.setDefaultEncoding("UTF-8");
return messageResource;
}
// To solver URL like:
// /SpringMVCInternationalization/en/login2
// /SpringMVCInternationalization/vi/login2
// /SpringMVCInternationalization/fr/login2
@Bean(name = "localeResolver")
public LocaleResolver getLocaleResolver() {
LocaleResolver resolver= new UrlLocaleResolver();
return resolver;
}
}
Thay đổi lại cấu hình Interceptor trong WebMvcConfig:
WebMvcConfig.java
package org.o7planning.springmvcinternationalization.config;
import org.o7planning.springmvcinternationalization.interceptor.UrlLocaleInterceptor;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.DefaultServletHandlerConfigurer;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
@Configuration
@EnableWebMvc
public class WebMvcConfig extends WebMvcConfigurerAdapter {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
// Default..
}
@Override
public void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) {
configurer.enable();
}
@Override
public void addInterceptors(InterceptorRegistry registry) {
UrlLocaleInterceptor localeInterceptor = new UrlLocaleInterceptor();
registry.addInterceptor(localeInterceptor).addPathPatterns("/en/*", "/fr/*", "/vi/*");
}
}
Controller:
MainController.java
package org.o7planning.springmvcinternationalization.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@RequestMapping(value = "/{locale:en|fr|vi}/login2")
public String login2(Model model) {
return "login2";
}
}
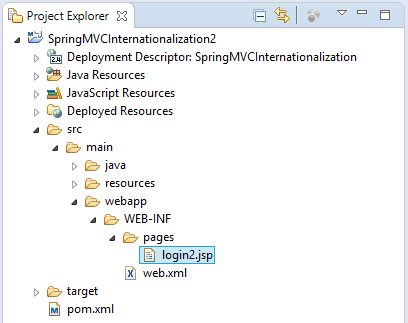
login2.jsp
<%@taglib uri="http://www.springframework.org/tags" prefix="spring"%>
<%@ page session="false"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title><spring:message code="label.title" /></title>
</head>
<body>
<div style="text-align: right;padding:5px;margin:5px 0px;background:#ccc;">
<a href="${pageContext.request.contextPath}/en/login2">Login (English)</a>
<a href="${pageContext.request.contextPath}/fr/login2">Login (French)</a>
<a href="${pageContext.request.contextPath}/vi/login2">Login (Vietnamese)</a>
</div>
<form method="post" action="">
<table>
<tr>
<td>
<strong>
<spring:message code="label.userName" />
</strong>
</td>
<td><input name="userName" /></td>
</tr>
<tr>
<td>
<strong>
<spring:message code="label.password" />
</strong>
</td>
<td><input name="password" /></td>
</tr>
<tr>
<td colspan="2">
<spring:message code="label.submit" var="labelSubmit"></spring:message>
<input type="submit" value="${labelSubmit}" />
</td>
</tr>
</table>
</form>
</body>
</html>
Chạy ứng dụng:
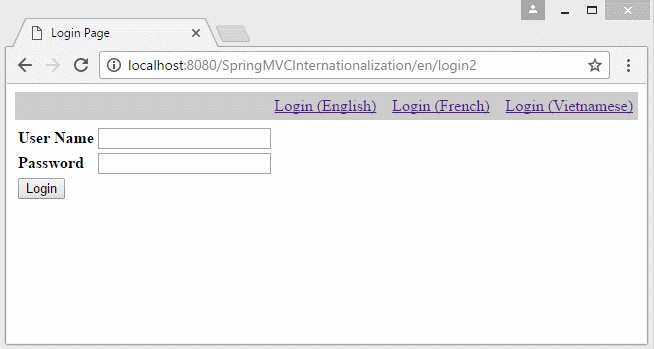
8. Web đa ngữ với nội dung trong DB
Ví dụ về một Website đa ngôn ngữ ở trên có thể làm bạn chưa hài lòng. Bạn mong muốn có một website tin tức với nhiều ngôn ngữ, và nội dung lưu trữ trong Database. Một giải pháp mà bạn có thể sử dụng là sử dụng nhiều Datasource. Mà mỗi datasource là một database chứa nội dung của một ngôn ngữ.
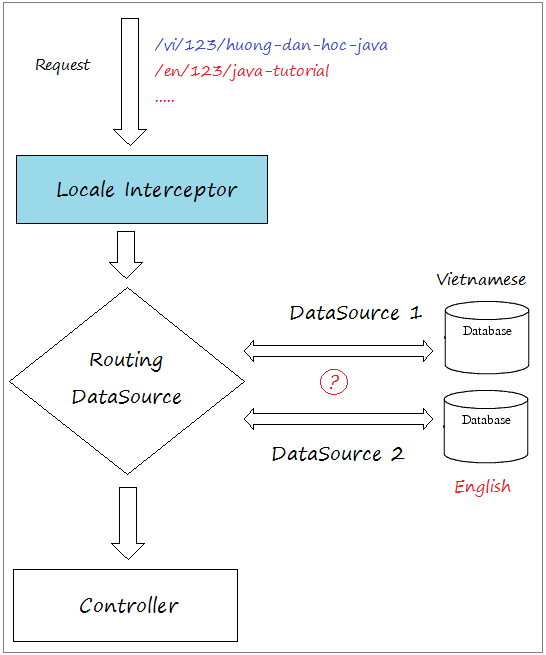
Bạn có thể tham khảo thêm tại:
Các hướng dẫn Spring MVC
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Cài đặt Spring Tool Suite cho Eclipse
- Hướng dẫn lập trình Spring MVC cho người mới bắt đầu - Hello Spring 4 MVC
- Cấu hình các nguồn dữ liệu tĩnh trong Spring MVC
- Hướng dẫn sử dụng Spring MVC Interceptor
- Tạo ứng dụng web đa ngôn ngữ với Spring MVC
- Hướng dẫn Upload File với Spring MVC
- Ứng dụng Java Web login đơn giản sử dụng Spring MVC, Spring Security và Spring JDBC
- Hướng dẫn sử dụng Spring MVC Security với Hibernate
- Hướng dẫn sử dụng Spring MVC Security và Spring JDBC (XML Config)
- Đăng nhập bằng mạng xã hội trong Spring MVC với Spring Social Security
- Hướng dẫn sử dụng Spring MVC và Velocity
- Hướng dẫn sử dụng Spring MVC với FreeMarker
- Sử dụng Template trong Spring MVC với Apache Tiles
- Hướng dẫn sử dụng Spring MVC và Spring JDBC Transaction
- Sử dụng nhiều DataSource trong Spring MVC
- Hướng dẫn sử dụng Spring MVC, Hibernate và Spring Transaction Manager
- Hướng dẫn sử dụng Spring MVC Form và Hibernate
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Tạo một ứng dụng Java Web bán hàng sử dụng Spring MVC và Hibernate
- Ví dụ CRUD đơn giản với Spring MVC RESTful Web Service
- Triển khai ứng dụng Spring MVC trên Oracle WebLogic Server
Show More