Tạo dự án Java OSGi với Maven và Tycho
1. Giới thiệu
Tài liệu này được viết dựa trên:
- Eclipse 3.4 (LUNA)
- Tycho 0.20.0
Trước hết phải đảm bảo rằng bạn đã cài đặt Tycho vào Eclipse. Nếu chưa cài bạn có thể xem hướng dẫn tại đây:
2. Tạo nhanh OSGi Project (SimpleOSGi)
Trước hết chúng ta tạo nhanh một OSGi project theo đúng cách thông thường.
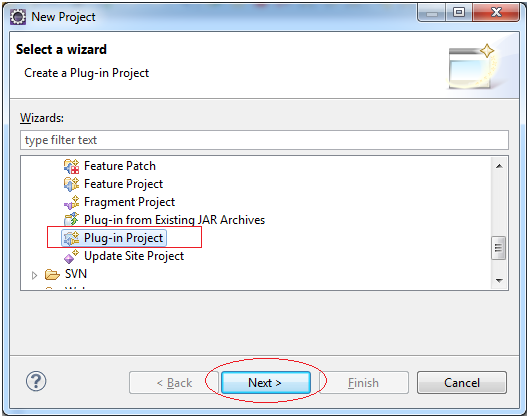
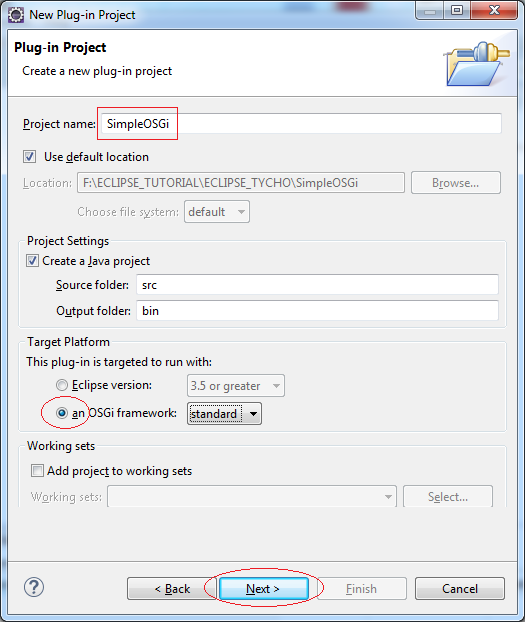
Nhập vào:
- Version: 2.0.0.qualifier
- Activator: org.o7planning.tutorial.simpleosgi.Activator
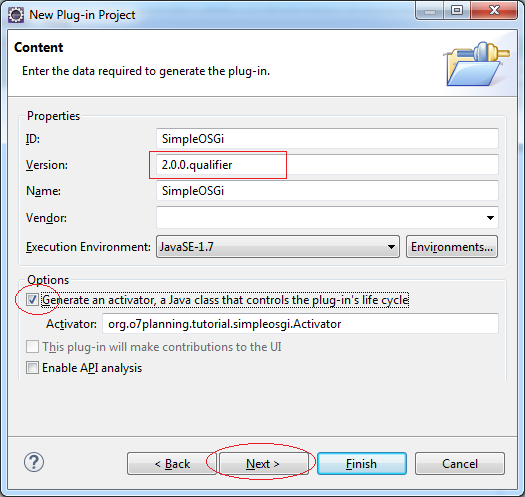
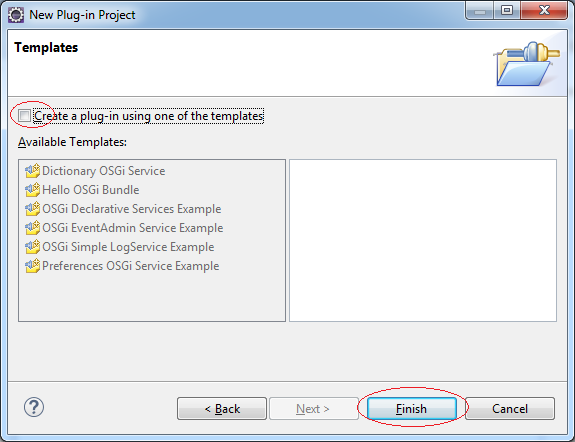
Add:
- org.eclipse.osgi
- org.eclipse.equinox.console
- org.apache.felix.gogo.command
- org.apache.felix.gogo.runtime
- org.apache.felix.gogo.shell
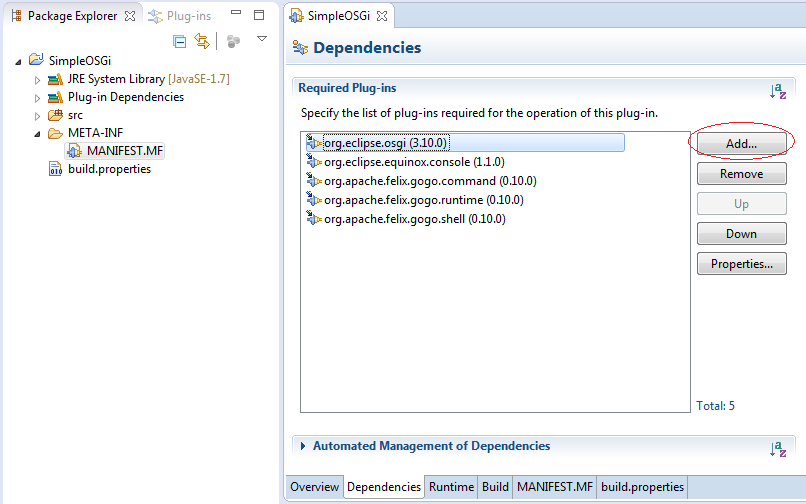
Activator.java
package org.o7planning.tutorial.simpleosgi;
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
public class Activator implements BundleActivator {
private static BundleContext context;
static BundleContext getContext() {
return context;
}
public void start(BundleContext bundleContext) throws Exception {
Activator.context = bundleContext;
System.out.println("SimpleOSGi Started");
}
public void stop(BundleContext bundleContext) throws Exception {
Activator.context = null;
System.out.println("SimpleOSGi Stopped");
}
}
3. Chạy OSGi (SimpleOSGi)
Chúng ta sẽ cấu hình nhanh và chạy OSGi này, để thấy rằng tình trạng của OSGi vừa tạo ra là tốt.
Nhấn phải vào project SimpleOSGi, chọn "Run As/Run Configuration.."
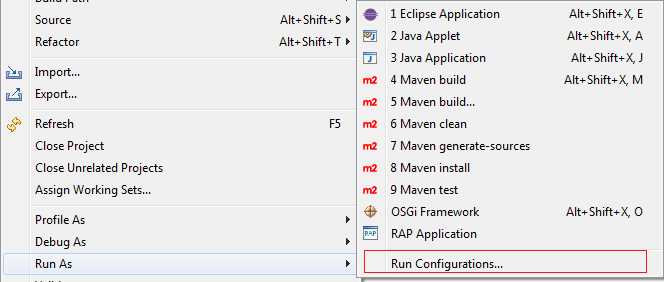
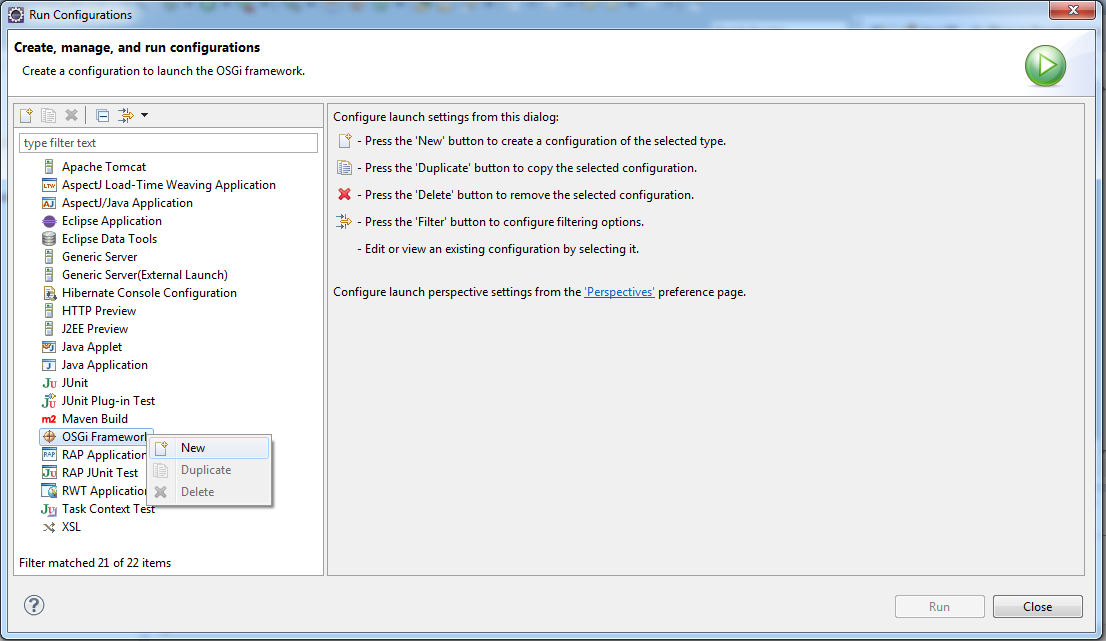
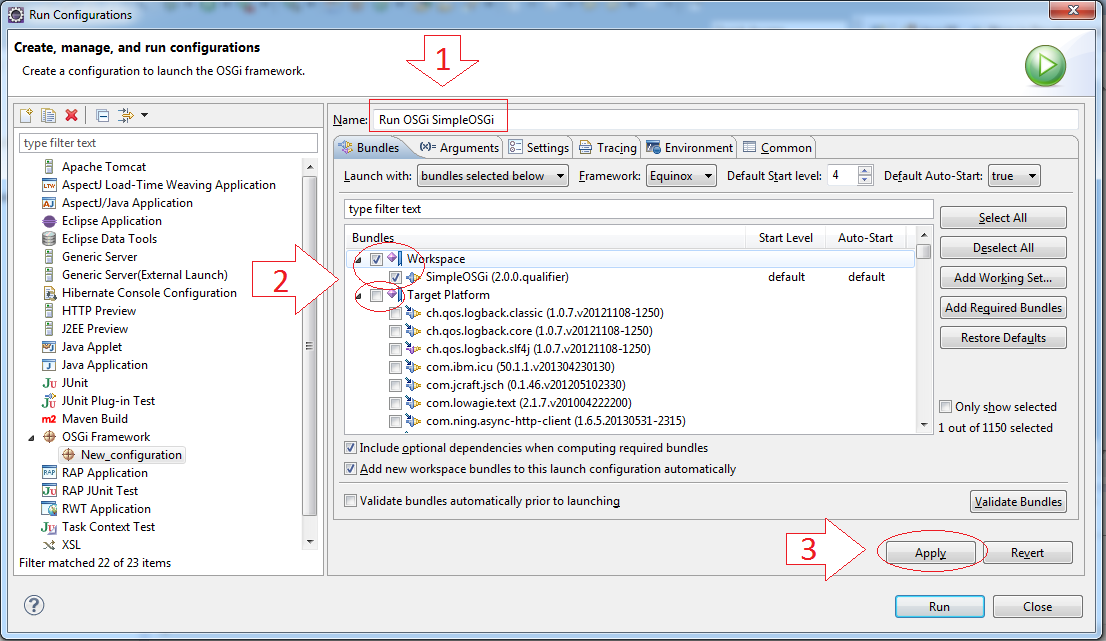
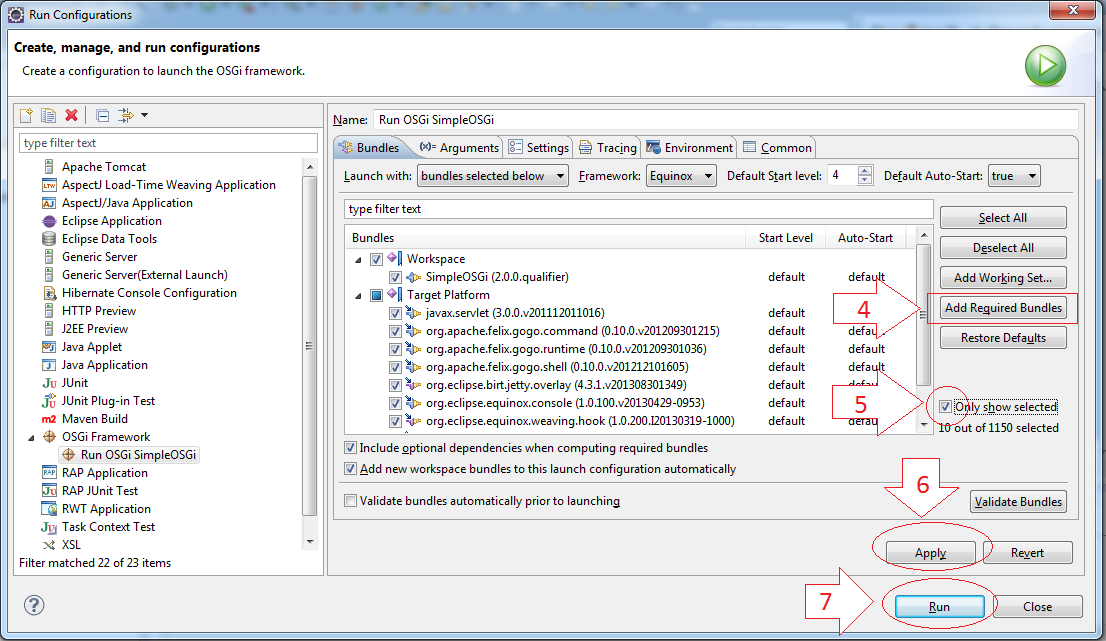
Chạy OSGi cho kết quả thành công.
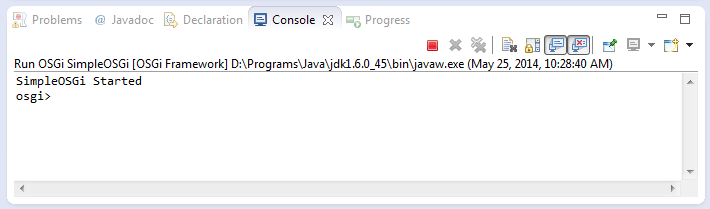
4. Tạo nhanh một Maven Project (SimpleMaven)
Tiếp theo chúng ta sẽ tạo nhanh một Maven Project. Mục tiêu để sau này chúng ta cùng build 2 project Maven Project & Osgi Project sử dụng Maven & Tycho. Và thấy rằng cả 2 project này được đối xử như nhau.
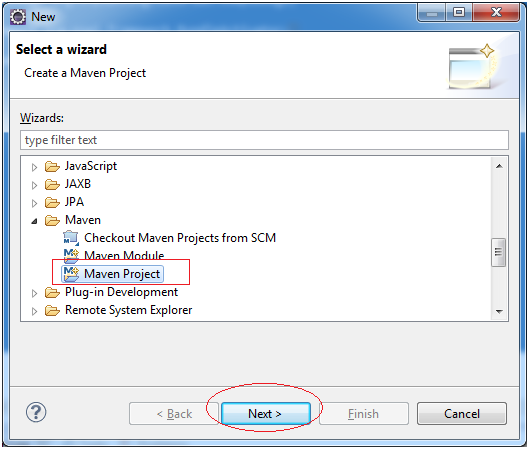
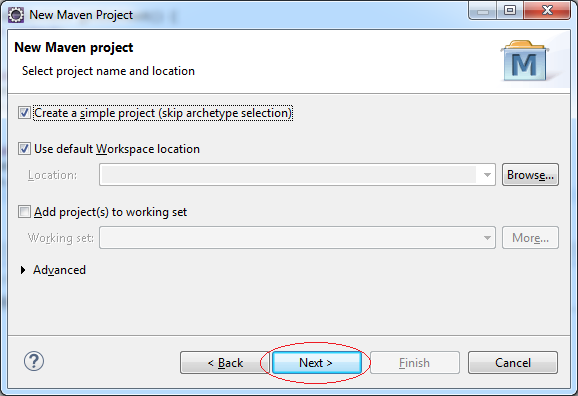
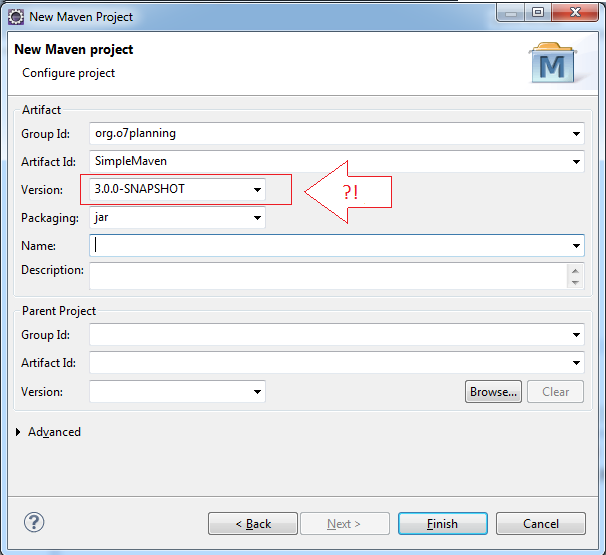
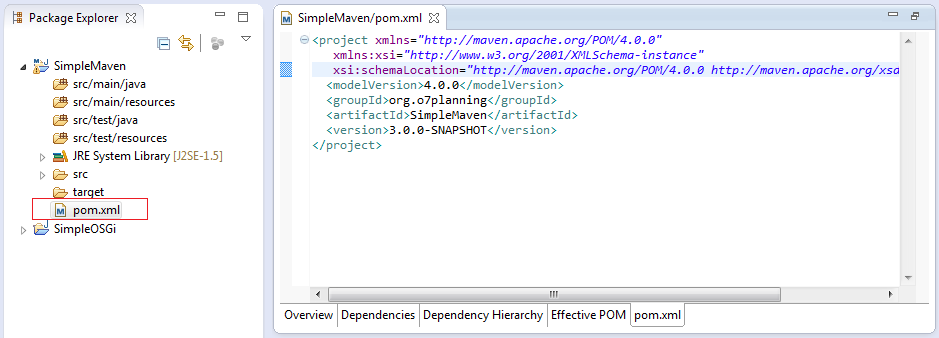
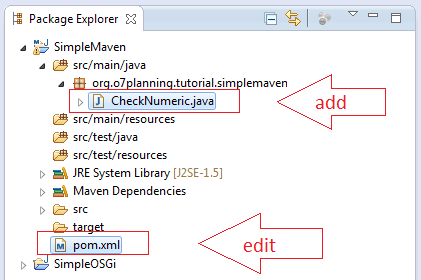
CheckNumeric.java
package org.o7planning.tutorial.simplemaven;
import org.apache.commons.lang3.StringUtils;
public class CheckNumeric {
public static void main(String[] args) {
String text1 = "0123a4";
String text2 = "01234";
boolean result1 = StringUtils.isNumeric(text1);
boolean result2 = StringUtils.isNumeric(text2);
System.out.println(text1 + " is a numeric? " + result1);
System.out.println(text1 + " is a numeric? " + result2);
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMaven</artifactId>
<version>3.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
</project>
5. Tạo MavenParent Project
Mục đích của project này để cấu hình Maven & Tycho để build 2 project trên (SimpleOSGi & SimpleMaven)
Tạo một Project java thông thường
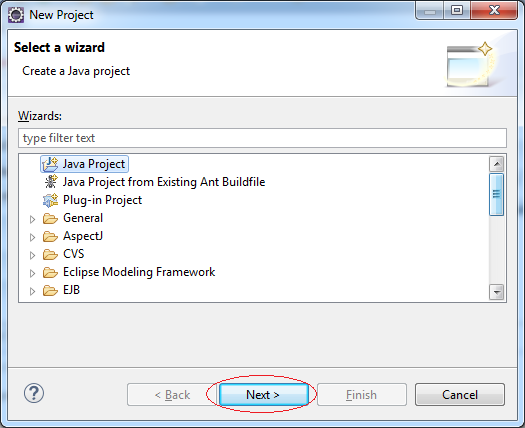
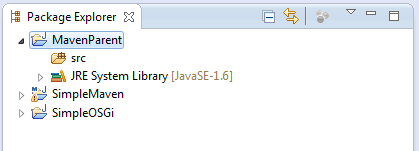
6. Cấu hình trên OSGi project (SimpleOSGi)
Trước hết chúng ta chuyển SimpleOSGi project thành Maven project. Như vậy lúc này SimpleOSGi vừa là một OSGi project vừa là Maven project.
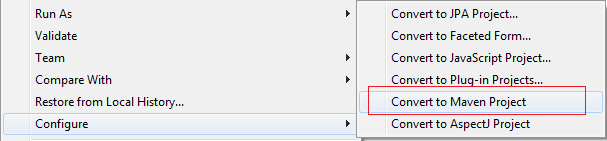
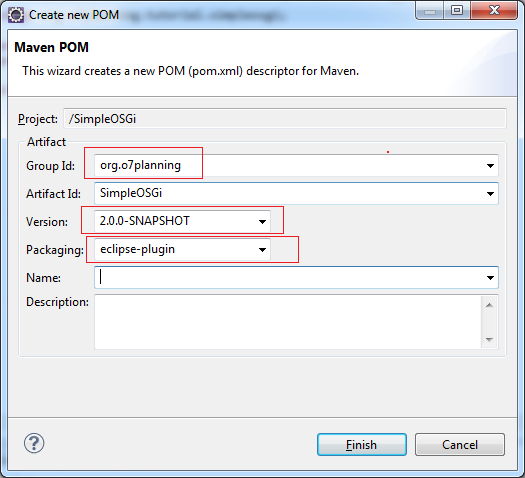
Project đã được chuyển đổi thành Maven project, và có thông báo lỗi, bạn không cần phải lo lắng về điều đó.
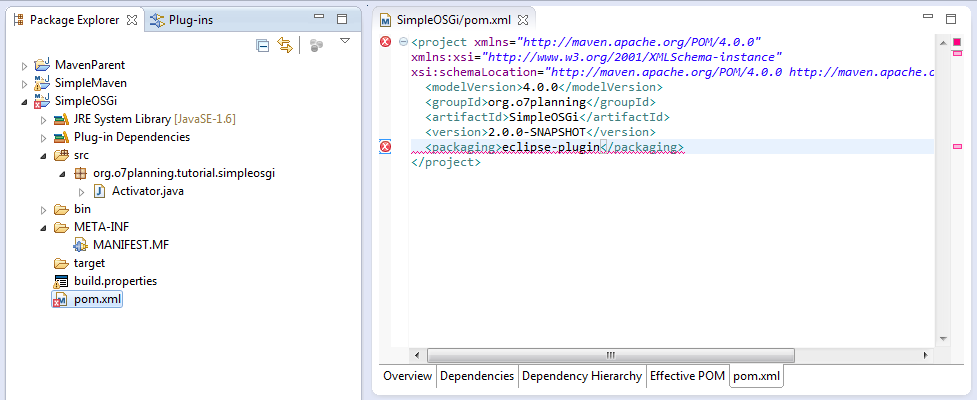
Chú ý rằng nếu OSGi của bạn đánh version AAA và có đuôi qualifier, thì trên Maven bạn cũng phải cấu hình trên file pom.xml, project có version AAA và đuôi là SNAPSHOT. Giống như hình minh họa dưới đây.
Mở file pom.xml và cấu hình lại như sau:
SimpleOSGi/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleOSGi</artifactId>
<version>2.0.0-SNAPSHOT</version>
<packaging>eclipse-plugin</packaging>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
7. Cấu hình trên Maven Project (SimpleMaven)
Mở file pom.xml trên project SimpleMaven thêm đoạn code sau:
**
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
SimpleMaven/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMaven</artifactId>
<version>3.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
8. Cấu hình trên Maven Parent
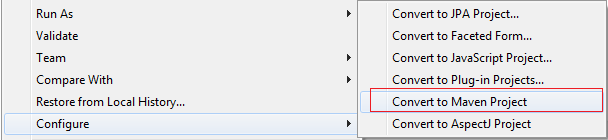
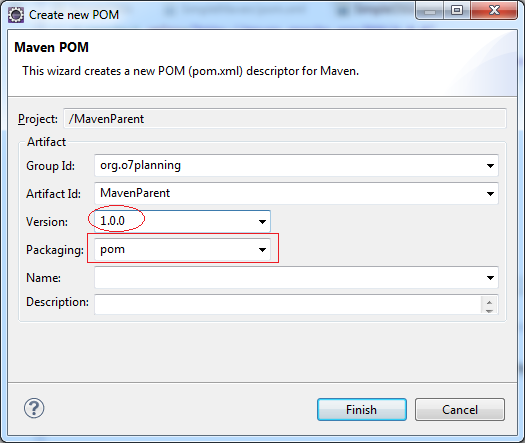
MavenParent/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<packaging>pom</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<!-- 0.22.0 : Target location type: Directory is not supported -->
<tycho-version>0.22.0</tycho-version>
</properties>
<repositories>
<!-- Select the P2 repository to be used when resolving dependencies -->
<repository>
<id>eclipse-luna</id>
<layout>p2</layout>
<url>http://download.eclipse.org/releases/luna</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>tycho-snapshots</id>
<url>https://oss.sonatype.org/content/groups/public/</url>
</pluginRepository>
</pluginRepositories>
<build>
<plugins>
<plugin>
<!-- enable tycho build extension -->
<groupId>org.eclipse.tycho</groupId>
<artifactId>tycho-maven-plugin</artifactId>
<version>${tycho-version}</version>
<extensions>true</extensions>
<configuration>
<dependency-resolution>
<optionalDependencies>ignore</optionalDependencies>
</dependency-resolution>
</configuration>
</plugin>
<plugin>
<groupId>org.eclipse.tycho</groupId>
<artifactId>tycho-versions-plugin</artifactId>
<version>${tycho-version}</version>
<configuration>
<dependency-resolution>
<optionalDependencies>ignore</optionalDependencies>
</dependency-resolution>
</configuration>
</plugin>
<!--
<plugin>
<groupId>org.eclipse.tycho</groupId>
<artifactId>target-platform-configuration</artifactId>
<version>${tycho-version}</version>
<configuration>
<target>
<artifact>
<groupId>org.o7planning</groupId>
<artifactId>TargetPlatform</artifactId>
<version>1.0.0</version>
</artifact>
</target>
</configuration>
</plugin>
-->
</plugins>
</build>
<modules>
<module>../SimpleMaven</module>
<module>../SimpleOSGi</module>
</modules>
</project>
Công nghệ của Eclipse
- Làm sao để có các thư viện mã nguồn mở Java dưới dạng OSGi
- Cài đặt Tycho cho Eclipse
- Hướng dẫn lập trình Java OSGi cho người mới bắt đầu
- Tạo dự án Java OSGi với Maven và Tycho
- Cài đặt WindowBuilder cho Eclipse
- Các nền tảng nào bạn nên chọn để lập trình ứng dụng Java Desktop?
- Lập trình ứng dụng Java Desktop sử dụng SWT
- Hướng dẫn và ví dụ Eclipse JFace
- Cài đặt e4 Tools Developer Resources cho Eclipse
- Đóng gói và triển khai ứng dụng Desktop SWT/RCP
- Cài đặt Eclipse RAP Target Platform
- Cài đặt EMF cho Eclipse
- Cài đặt RAP e4 Tooling cho Eclipse
- Tạo Eclipse RAP Widget từ ClientScripting widget
- Cài đặt GEF cho Eclipse
- Hướng dẫn lập trình Eclipse RAP cho người mới bắt đầu - Ứng dụng Workbench (trước e4)
- Hướng dẫn lập trình Eclipse RCP 3 cho người mới bắt đầu - Ứng dụng Workbench
- Ứng dụng Eclipse RCP 3 đơn giản - Tương tác View và Editor
- Hướng dẫn lập trình Eclipse RCP 4 cho người mới bắt đầu - Ứng dụng e4 Workbench
- Cài đặt RAP Tools cho Eclipse
- Hướng dẫn lập trình Eclipse RAP cho người mới bắt đầu - Ứng dụng cơ bản
- Hướng dẫn lập trình Eclipse RAP cho người mới bắt đầu - Ứng dụng e4 Workbench
- Đóng gói và triển khai ứng dụng Eclipse RAP
Show More
Các hướng dẫn Maven
- Cài đặt Maven cho Eclipse
- Hướng dẫn sử dụng Maven cho người mới bắt đầu
- Quản lý các phụ thuộc trong Maven
- Xây dựng dự án nhiều Module với Maven
- Chạy ứng dụng Java web Maven trên Tomcat Maven Plugin
- Chạy ứng dụng Java Web Maven trên Jetty Maven Plugin
- Cài đặt Tycho cho Eclipse
- Tạo dự án Java OSGi với Maven và Tycho
- Tạo Maven Web App Project rỗng trong Eclipse
- Tương tác OSGi và AspectJ
Show More