Hướng dẫn sử dụng Spring Boot và Thymeleaf
1. Thymeleaf là gì?
Thymeleaf là một Java XML/XHTML/HTML5 Template Engine nó có thể làm việc với cả hai môi trường Web và môi trường không phải Web. Nó phù hợp hơn khi được sử dụng để phục vụ XHTML/HTML5 trên tầng View (View Layer) của ứng dụng Web dựa trên kiến trúc MVC. Nó có thể xử lý bất kỳ một file XML nào, thậm trí trên các môi trường offline (Không trực tuyến). Nó hỗ trợ đầy đủ để tương tác với Spring Framework.
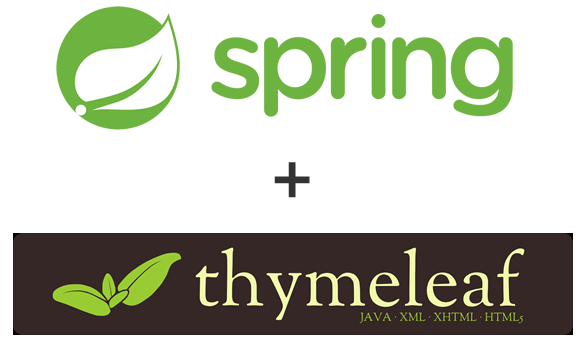
Các file mẫu (Template file) của Thymeleaf bản chất chỉ là một file văn bản thông thường, có định dạng XML/XHTML/HTML5. Thymeleaf Engine (Bộ máy Thymeleaf) sẽ đọc một file mẫu (template file) và kết hợp với các đối tượng Java để tạo ra (generate) một tài liệu khác.
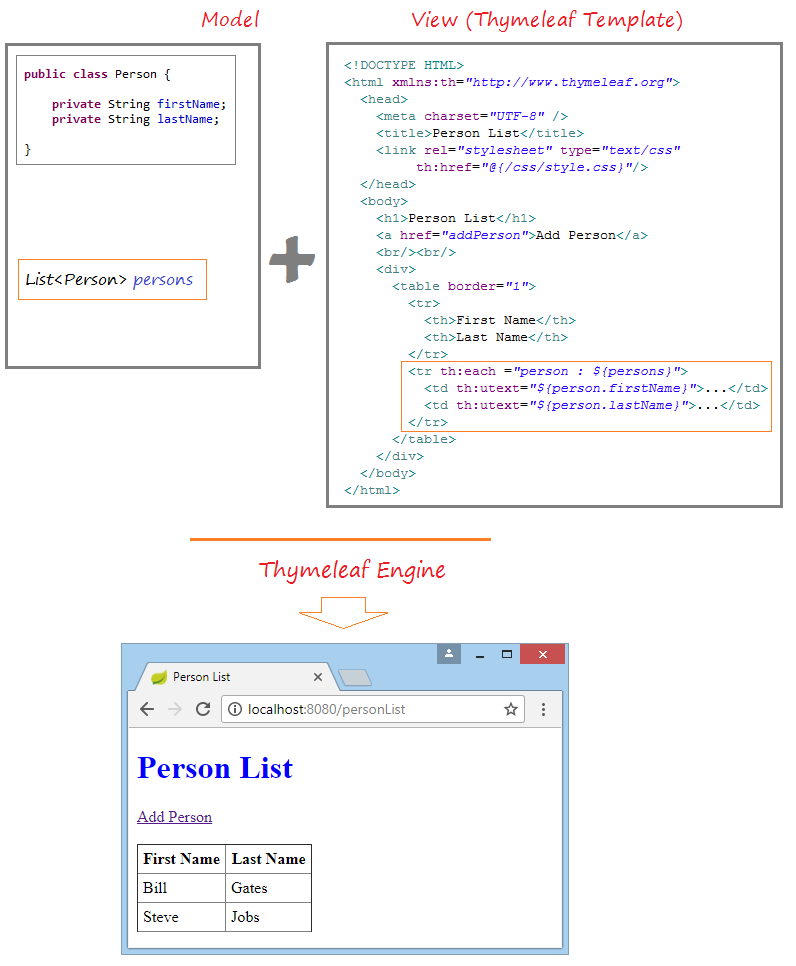
Thymeleaf có thể sử dụng để thay thế cho JSP trên tầng View (View Layer) của ứng dụng Web MVC. Thymeleaf là phần mềm mã nguồn mở, được cấp phép theo giấy phép Apache 2.0.
Dưới đây là hình ảnh của ứng dụng mà chúng ta sẽ thực hiện trong bài học này:
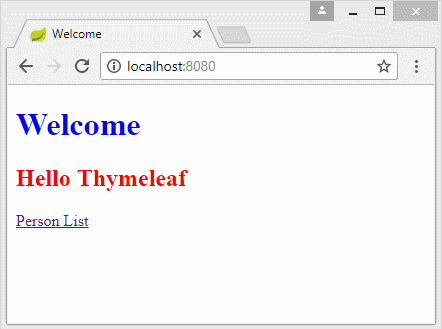
Xem thêm:
- Các hướng dẫn Thymeleaf
2. Tạo ứng dụng
Trên Eclipse chọn:
- File/New/Other...
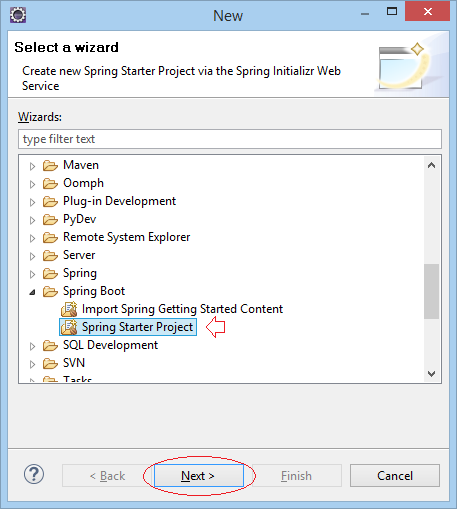
Nhập vào:
- Name: SpringBootThymeleaf
- Group: org.o7planning
- Artifact: SpringBootThymeleaf
- Description: Spring Boot and Thymeleaf
- Package: org.o7planning.thymeleaf
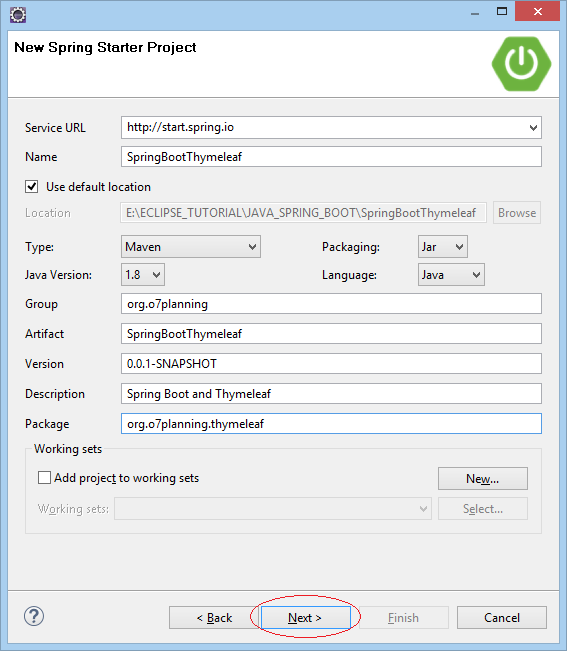
Lựa chọn 2 công nghệ Web và Thymeleaf.
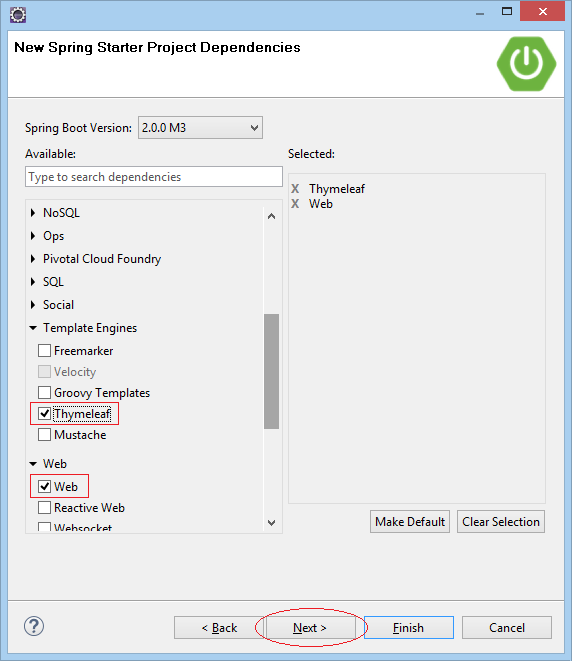
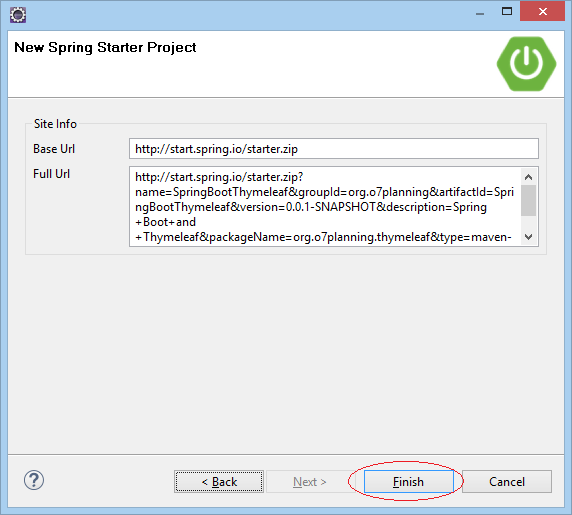
Project của bạn đã được tạo ra:
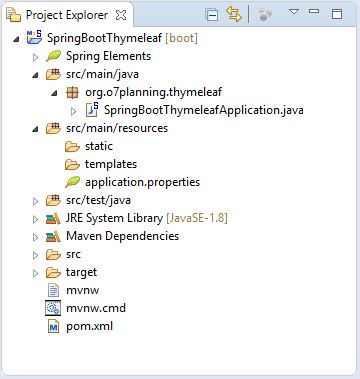
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootThymeleaf</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootThymeleaf</name>
<description>Spring Boot and Thymeleaf</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. Thymeleaf Template
Thymeleaf Template là các file mẫu. Nội dung của nó theo định dạng XML/XHTML/HTML5. Chúng ta sẽ tạo ra 3 file và đặt tại thư mục src/main/resources/templates:
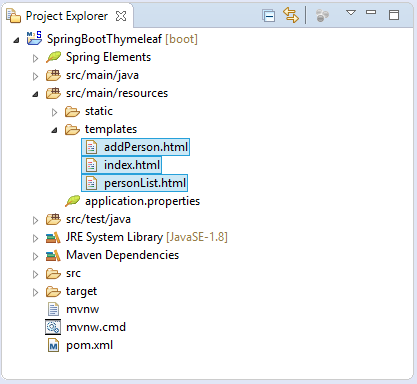
index.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Welcome</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Welcome</h1>
<h2 th:utext="${message}">..!..</h2>
<!--
In Thymeleaf the equivalent of
JSP's ${pageContext.request.contextPath}/edit.html
would be @{/edit.html}
-->
<a th:href="@{/personList}">Person List</a>
</body>
</html>
personList.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Person List</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Person List</h1>
<a href="addPerson">Add Person</a>
<br/><br/>
<div>
<table border="1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr th:each ="person : ${persons}">
<td th:utext="${person.firstName}">...</td>
<td th:utext="${person.lastName}">...</td>
</tr>
</table>
</div>
</body>
</html>
addPerson.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8" />
<title>Add Person</title>
<link rel="stylesheet" type="text/css" th:href="@{/css/style.css}"/>
</head>
<body>
<h1>Create a Person:</h1>
<!--
In Thymeleaf the equivalent of
JSP's ${pageContext.request.contextPath}/edit.html
would be @{/edit.html}
-->
<form th:action="@{/addPerson}"
th:object="${personForm}" method="POST">
First Name:
<input type="text" th:field="*{firstName}" />
<br/>
Last Name:
<input type="text" th:field="*{lastName}" />
<br/>
<input type="submit" value="Create" />
</form>
<br/>
<!-- Check if errorMessage is not null and not empty -->
<div th:if="${errorMessage}" th:utext="${errorMessage}"
style="color:red;font-style:italic;">
...
</div>
</body>
</html>
Ở trên tôi đã tạo ra 3 file HTML. Các file HTML ở trên cần phải phù hợp với các tiêu chuẩn của XML, tất cả các thẻ (tag) đều phải có mở và đóng. Chẳng hạn:
<div>A div tag</div>
<br />
<meta charset="UTF-8" />
Tất cả các file HTML cần phải khai báo sử dụng Thymeleaf Namespace:
<!-- Thymeleaf Namespace -->
<html xmlns:th="http://www.thymeleaf.org">
Trong các file mẫu (Template file)có các Thymeleaf Marker (Các đánh dấu của Thymeleaf), chúng là các chỉ dẫn giúp Thymeleaf Engine chế biến dữ liệu.
Thymeleaf Engine phân tích file mẫu (Template file), và kết hợp với các dữ liệu Java để tạo ra (generate) một tài liệu mới.
Thymeleaf Engine phân tích file mẫu (Template file), và kết hợp với các dữ liệu Java để tạo ra (generate) một tài liệu mới.
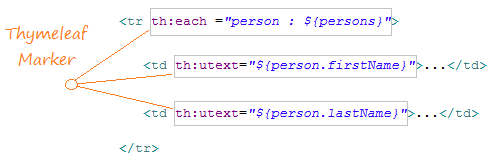
Dưới đây là các ví dụ để sử dụng Context-Path trong Thymeleaf:<!-- Example 1: --> <a th:href="@{/mypath/abc.html}">A Link</a> Output: ==> <a href="/my-context-path/mypath/abc.html">A Link</a> <!-- Example 2: --> <form th:action="@{/mypath/abc.html}" th:object="${personForm}" method="POST"> Output: ==> <form action="/my-context-path/mypath/abc.html" method="POST">
4. Static Resource & Properties File
Với các nguồn dữ liệu tĩnh (Static Resource), chẳng hạn các file css, javascript, image,.. bạn cần đặt chúng vào thư mục src/main/resources/static hoặc các thư mục con của nó.
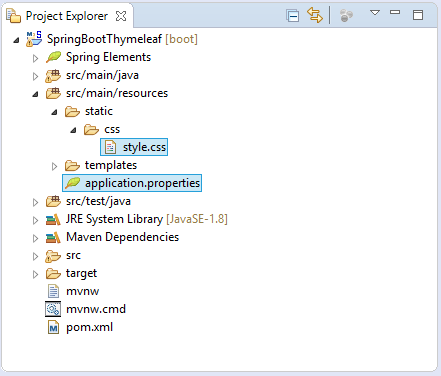
style.css
h1 {
color:#0000FF;
}
h2 {
color:#FF0000;
}
table {
border-collapse: collapse;
}
table th, table td {
padding: 5px;
}
application.properties
spring.thymeleaf.cache=false
welcome.message=Hello Thymeleaf
error.message=First Name & Last Name is required!
5. Các lớp Model, Form, Controller
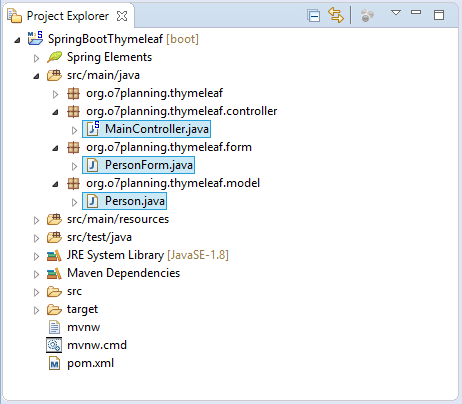
Person.java
package org.o7planning.thymeleaf.model;
public class Person {
private String firstName;
private String lastName;
public Person() {
}
public Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
Lớp PersonForm đại diện cho dữ liệu của FORM khi bạn tạo mới một Person trên trang addPerson.
PersonForm.java
package org.o7planning.thymeleaf.form;
public class PersonForm {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
MainController là một lớp Controller, nó xử lý yêu cầu của người dùng và điều khiển luồng đi (flow) của ứng dụng.
MainController.java
package org.o7planning.thymeleaf.controller;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.thymeleaf.form.PersonForm;
import org.o7planning.thymeleaf.model.Person;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MainController {
private static List<Person> persons = new ArrayList<Person>();
static {
persons.add(new Person("Bill", "Gates"));
persons.add(new Person("Steve", "Jobs"));
}
// Được tiêm vào (inject) từ application.properties.
@Value("${welcome.message}")
private String message;
@Value("${error.message}")
private String errorMessage;
@RequestMapping(value = { "/", "/index" }, method = RequestMethod.GET)
public String index(Model model) {
model.addAttribute("message", message);
return "index";
}
@RequestMapping(value = { "/personList" }, method = RequestMethod.GET)
public String personList(Model model) {
model.addAttribute("persons", persons);
return "personList";
}
@RequestMapping(value = { "/addPerson" }, method = RequestMethod.GET)
public String showAddPersonPage(Model model) {
PersonForm personForm = new PersonForm();
model.addAttribute("personForm", personForm);
return "addPerson";
}
@RequestMapping(value = { "/addPerson" }, method = RequestMethod.POST)
public String savePerson(Model model, //
@ModelAttribute("personForm") PersonForm personForm) {
String firstName = personForm.getFirstName();
String lastName = personForm.getLastName();
if (firstName != null && firstName.length() > 0 //
&& lastName != null && lastName.length() > 0) {
Person newPerson = new Person(firstName, lastName);
persons.add(newPerson);
return "redirect:/personList";
}
model.addAttribute("errorMessage", errorMessage);
return "addPerson";
}
}
Các hướng dẫn Spring Boot
- Cài đặt Spring Tool Suite cho Eclipse
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Hướng dẫn lập trình Spring Boot cho người mới bắt đầu
- Các thuộc tính thông dụng của Spring Boot
- Hướng dẫn sử dụng Spring Boot và Thymeleaf
- Hướng dẫn sử dụng Spring Boot và FreeMarker
- Hướng dẫn sử dụng Spring Boot và Groovy
- Hướng dẫn sử dụng Spring Boot và Mustache
- Hướng dẫn sử dụng Spring Boot và JSP
- Hướng dẫn sử dụng Spring Boot, Apache Tiles, JSP
- Sử dụng Logging trong Spring Boot
- Giám sát ứng dụng với Spring Boot Actuator
- Tạo ứng dụng web đa ngôn ngữ với Spring Boot
- Sử dụng nhiều ViewResolver trong Spring Boot
- Sử dụng Twitter Bootstrap trong Spring Boot
- Hướng dẫn và ví dụ Spring Boot Interceptor
- Hướng dẫn sử dụng Spring Boot, Spring JDBC và Spring Transaction
- Hướng dẫn và ví dụ Spring JDBC
- Hướng dẫn sử dụng Spring Boot, JPA và Spring Transaction
- Hướng dẫn sử dụng Spring Boot và Spring Data JPA
- Hướng dẫn sử dụng Spring Boot, Hibernate và Spring Transaction
- Tương tác Spring Boot, JPA và cơ sở dữ liệu H2
- Hướng dẫn sử dụng Spring Boot và MongoDB
- Sử dụng nhiều DataSource với Spring Boot và JPA
- Sử dụng nhiều DataSource với Spring Boot và RoutingDataSource
- Tạo ứng dụng Login với Spring Boot, Spring Security, Spring JDBC
- Tạo ứng dụng Login với Spring Boot, Spring Security, JPA
- Tạo ứng dụng đăng ký tài khoản với Spring Boot, Spring Form Validation
- Ví dụ OAuth2 Social Login trong Spring Boot
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Ví dụ CRUD Restful Web Service với Spring Boot
- Ví dụ Spring Boot Restful Client với RestTemplate
- Ví dụ CRUD với Spring Boot, REST và AngularJS
- Bảo mật Spring Boot RESTful Service sử dụng Basic Authentication
- Bảo mật Spring Boot RESTful Service sử dụng Auth0 JWT
- Ví dụ Upload file với Spring Boot
- Ví dụ Download file với Spring Boot
- Ví dụ Upload file với Spring Boot và jQuery Ajax
- Ví dụ Upload file với Spring Boot và AngularJS
- Tạo ứng dụng Web bán hàng với Spring Boot, Hibernate
- Hướng dẫn và ví dụ Spring Email
- Tạo ứng dụng Chat đơn giản với Spring Boot và Websocket
- Triển khai ứng dụng Spring Boot trên Tomcat Server
- Triển khai ứng dụng Spring Boot trên Oracle WebLogic Server
- Cài đặt chứng chỉ SSL miễn phí Let's Encrypt cho Spring Boot
- Cấu hình Spring Boot chuyển hướng HTTP sang HTTPS
- Tìm nạp dữ liệu với Spring Data JPA DTO Projections
Show More