Hướng dẫn sử dụng Spring Boot và FreeMarker
1. FreeMarker là gì?
FreeMarker là một Template Engine (Bộ máy phân tích mẫu), nó được cung cấp bởi Apache như một thư viện Java mã nguồn mở. FreeMarker đọc các file mẫu và kết hợp với các đối tượng Java để tạo ra (generate) một văn bản đầu ra (Html, email, ..).
Các file mẫu (Template file) của FreeMarker bản chất chỉ là một file văn bản theo định dạng được giới thiệu bởi FreeMarker, đó là FreeMarker Template Language (FTL).
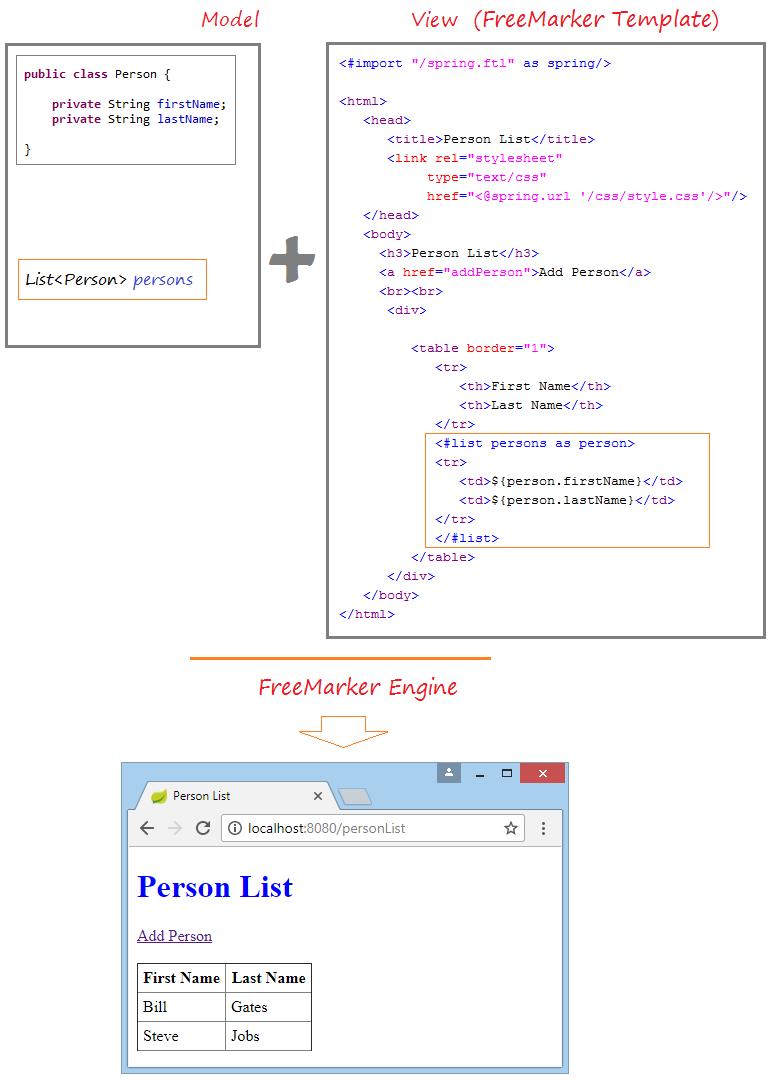
FreeMarker có thể được sử dụng để thay thế cho JSP trên tầng View (View Layer) của ứng dụng Web MVC.
Dưới đây là hình ảnh của ứng dụng mà chúng ta sẽ thực hiện trong bài học này:
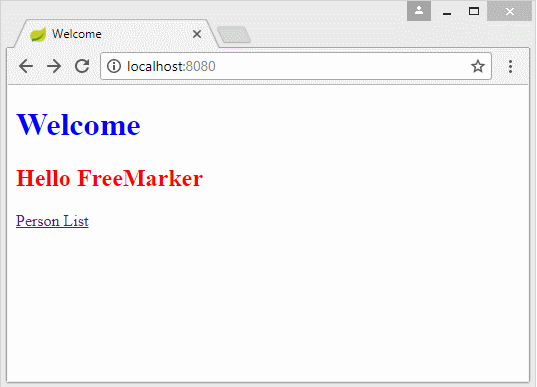
2. Tạo ứng dụng
Trên Eclipse chọn:
- File/New/Other...
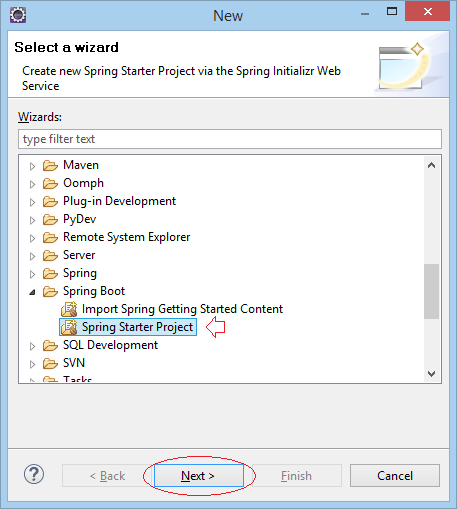
Nhập vào:
- Name: SpringBootFreeMarker
- Group: org.o7planning
- Artifact: SpringBootFreeMarker
- Description: Spring Boot and FreeMarker
- Package: org.o7planning.freemarker
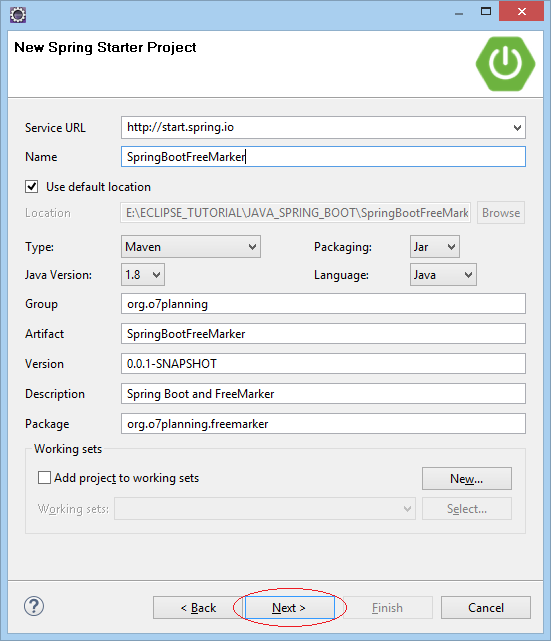
Lựa chọn 2 công nghệ Web và FreeMarker.
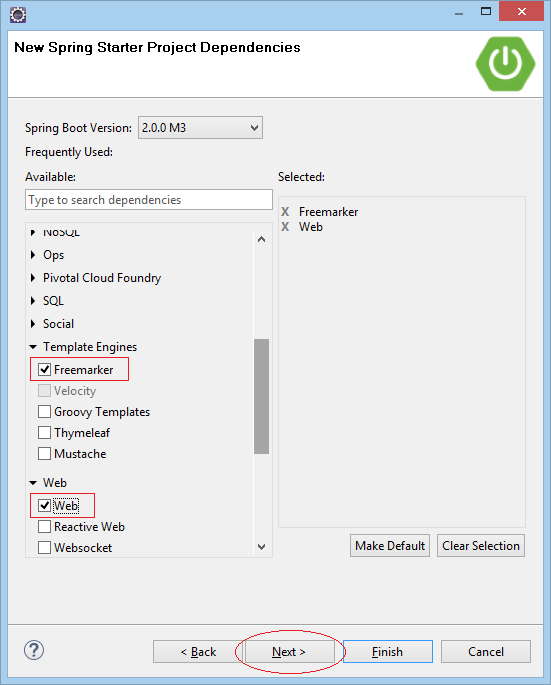
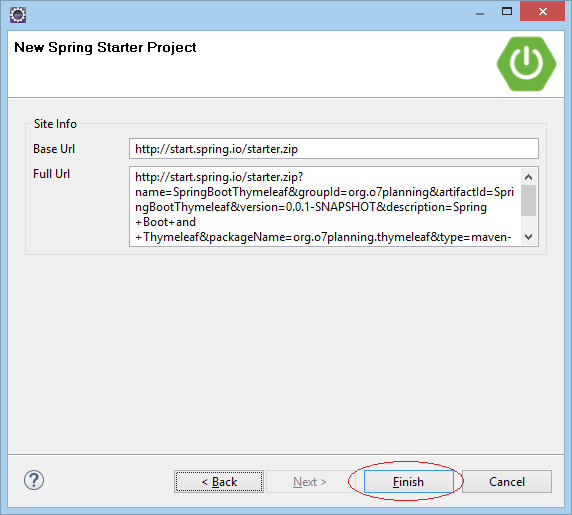
Project của bạn đã được tạo ra:
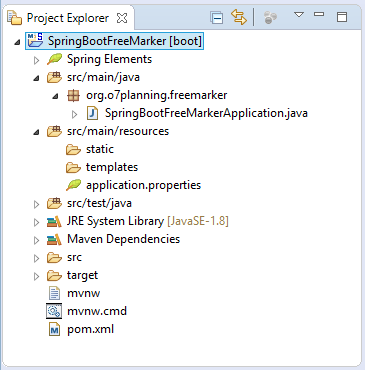
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootFreeMarker</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootFreeMarker</name>
<description>Spring Boot and FreeMarker</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. FreeMarker Template
Chúng ta sẽ tạo ra 3 file mẫu (Template file) và đặt tại thư mục src/main/resources/templates:
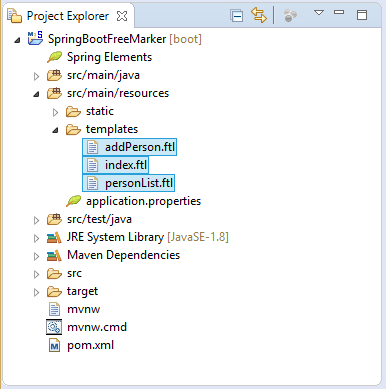
index.flt
<#import "/spring.ftl" as spring/>
<!DOCTYPE HTML>
<html>
<head>
<meta charset="UTF-8" />
<title>Welcome</title>
<link rel="stylesheet"
type="text/css" href="<@spring.url '/css/style.css'/>"/>
</head>
<body>
<h1>Welcome</h1>
<#if message??>
<h2>${message}</h2>
</#if>
<a href="<@spring.url '/personList'/>">Person List</a>
</body>
</html>
personList.flt
<#import "/spring.ftl" as spring/>
<html>
<head>
<title>Person List</title>
<link rel="stylesheet"
type="text/css"
href="<@spring.url '/css/style.css'/>"/>
</head>
<body>
<h3>Person List</h3>
<a href="addPerson">Add Person</a>
<br><br>
<div>
<table border="1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<#list persons as person>
<tr>
<td>${person.firstName}</td>
<td>${person.lastName}</td>
</tr>
</#list>
</table>
</div>
</body>
</html>
addPerson.flt
<#import "/spring.ftl" as spring/>
<html>
<head>
<title>Add Person</title>
<link rel="stylesheet"
type="text/css" href="<@spring.url '/css/style.css'/>"/>
</head>
<body>
<#if errorMessage??>
<div style="color:red;font-style:italic;">
${errorMessage}
</div>
</#if>
<div>
<fieldset>
<legend>Add Person</legend>
<form name="person" action="" method="POST">
First Name: <@spring.formInput "personForm.firstName" "" "text"/> <br/>
Last Name: <@spring.formInput "personForm.lastName" "" "text"/> <br/>
<input type="submit" value="Create" />
</form>
</fieldset>
</div>
</body>
</html>
Tất cả các file mẫu cần phải khai báo một "FreeMarker Macros".
<!-- FreeMarker Macros -->
<#import "/spring.ftl" as spring/>
Trong các file mẫu (Template file)có các FreeMarker Marker (Các đánh dấu của FreeMarker), chúng là các chỉ dẫn giúp FreeMarker Engine chế biến dữ liệu.
FreeMarker Engine phân tích file mẫu (Template file), và kết hợp với các dữ liệu Java để tạo ra (generate) một tài liệu mới.
FreeMarker Engine phân tích file mẫu (Template file), và kết hợp với các dữ liệu Java để tạo ra (generate) một tài liệu mới.
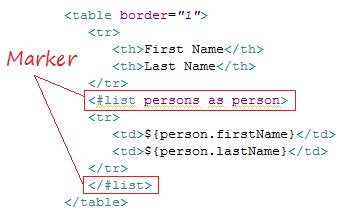
Dưới đây là các ví dụ để sử dụng Context-Path trong FreeMarker:<!-- Example 1: --> <a href="<@spring.url '/mypath/abc.html'/>">A Link</a> Output: ==> <a href="/my-context-path/mypath/abc.html">A Link</a> <!-- Example 2: --> <form action="<@spring.url '/mypath/abc.html'/>" method="POST"> Output: ==> <form action="/my-context-path/mypath/abc.html" method="POST">
4. Static Resource & Properties File
Với các nguồn dữ liệu tĩnh (Static Resource), chẳng hạn các file css, javascript, image,.. bạn cần đặt chúng vào thư mục src/main/resources/static hoặc các thư mục con của nó.
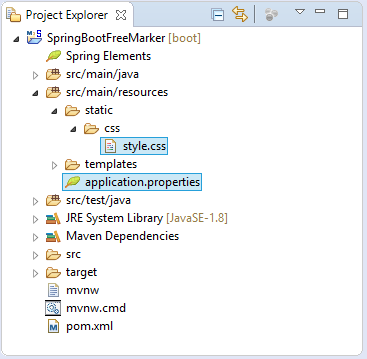
style.css
h1 {
color:#0000FF;
}
h2 {
color:#FF0000;
}
table {
border-collapse: collapse;
}
table th, table td {
padding: 5px;
}
application.properties
welcome.message=Hello FreeMarker
error.message=First Name & Last Name is required!
5. Các lớp Model, Form, Controller
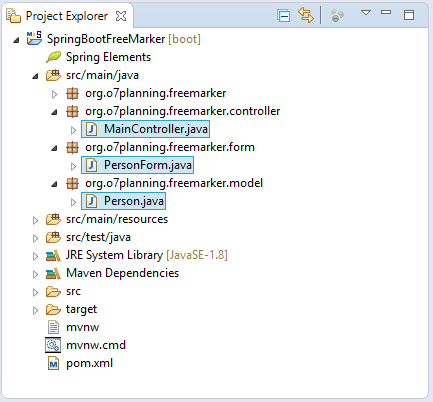
Person.java
package org.o7planning.freemarker.model;
public class Person {
private String firstName;
private String lastName;
public Person() {
}
public Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
Lớp PersonForm đại diện cho dữ liệu của FORM khi bạn tạo mới một Person trên trang addPerson.
PersonForm.java
package org.o7planning.freemarker.form;
public class PersonForm {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
MainController là một lớp Controller, nó xử lý yêu cầu của người dùng và điều khiển luồng đi (flow) của ứng dụng.
MainController.java
package org.o7planning.freemarker.controller;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.freemarker.form.PersonForm;
import org.o7planning.freemarker.model.Person;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MainController {
private static List<Person> persons = new ArrayList<Person>();
static {
persons.add(new Person("Bill", "Gates"));
persons.add(new Person("Steve", "Jobs"));
}
// Được tiêm vào (inject) từ application.properties.
@Value("${welcome.message}")
private String message;
@Value("${error.message}")
private String errorMessage;
@RequestMapping(value = { "/", "/index" }, method = RequestMethod.GET)
public String index(Model model) {
model.addAttribute("message", message);
return "index";
}
@RequestMapping(value = { "/personList" }, method = RequestMethod.GET)
public String personList(Model model) {
model.addAttribute("persons", persons);
return "personList";
}
@RequestMapping(value = { "/addPerson" }, method = RequestMethod.GET)
public String addPersonForm(Model model) {
PersonForm personForm = new PersonForm();
model.addAttribute("personForm", personForm);
return "addPerson";
}
@RequestMapping(value = { "/addPerson" }, method = RequestMethod.POST)
public String addPersonSave(Model model, //
@ModelAttribute("personForm") PersonForm personForm) {
String firstName = personForm.getFirstName();
String lastName = personForm.getLastName();
if (firstName != null && firstName.length() > 0 //
&& lastName != null && lastName.length() > 0) {
Person newPerson = new Person(firstName, lastName);
persons.add(newPerson);
return "redirect:/personList";
}
String error = "First Name & Last Name is required!";
model.addAttribute("errorMessage", error);
return "addPerson";
}
}
Các hướng dẫn Spring Boot
- Cài đặt Spring Tool Suite cho Eclipse
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Hướng dẫn lập trình Spring Boot cho người mới bắt đầu
- Các thuộc tính thông dụng của Spring Boot
- Hướng dẫn sử dụng Spring Boot và Thymeleaf
- Hướng dẫn sử dụng Spring Boot và FreeMarker
- Hướng dẫn sử dụng Spring Boot và Groovy
- Hướng dẫn sử dụng Spring Boot và Mustache
- Hướng dẫn sử dụng Spring Boot và JSP
- Hướng dẫn sử dụng Spring Boot, Apache Tiles, JSP
- Sử dụng Logging trong Spring Boot
- Giám sát ứng dụng với Spring Boot Actuator
- Tạo ứng dụng web đa ngôn ngữ với Spring Boot
- Sử dụng nhiều ViewResolver trong Spring Boot
- Sử dụng Twitter Bootstrap trong Spring Boot
- Hướng dẫn và ví dụ Spring Boot Interceptor
- Hướng dẫn sử dụng Spring Boot, Spring JDBC và Spring Transaction
- Hướng dẫn và ví dụ Spring JDBC
- Hướng dẫn sử dụng Spring Boot, JPA và Spring Transaction
- Hướng dẫn sử dụng Spring Boot và Spring Data JPA
- Hướng dẫn sử dụng Spring Boot, Hibernate và Spring Transaction
- Tương tác Spring Boot, JPA và cơ sở dữ liệu H2
- Hướng dẫn sử dụng Spring Boot và MongoDB
- Sử dụng nhiều DataSource với Spring Boot và JPA
- Sử dụng nhiều DataSource với Spring Boot và RoutingDataSource
- Tạo ứng dụng Login với Spring Boot, Spring Security, Spring JDBC
- Tạo ứng dụng Login với Spring Boot, Spring Security, JPA
- Tạo ứng dụng đăng ký tài khoản với Spring Boot, Spring Form Validation
- Ví dụ OAuth2 Social Login trong Spring Boot
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Ví dụ CRUD Restful Web Service với Spring Boot
- Ví dụ Spring Boot Restful Client với RestTemplate
- Ví dụ CRUD với Spring Boot, REST và AngularJS
- Bảo mật Spring Boot RESTful Service sử dụng Basic Authentication
- Bảo mật Spring Boot RESTful Service sử dụng Auth0 JWT
- Ví dụ Upload file với Spring Boot
- Ví dụ Download file với Spring Boot
- Ví dụ Upload file với Spring Boot và jQuery Ajax
- Ví dụ Upload file với Spring Boot và AngularJS
- Tạo ứng dụng Web bán hàng với Spring Boot, Hibernate
- Hướng dẫn và ví dụ Spring Email
- Tạo ứng dụng Chat đơn giản với Spring Boot và Websocket
- Triển khai ứng dụng Spring Boot trên Tomcat Server
- Triển khai ứng dụng Spring Boot trên Oracle WebLogic Server
- Cài đặt chứng chỉ SSL miễn phí Let's Encrypt cho Spring Boot
- Cấu hình Spring Boot chuyển hướng HTTP sang HTTPS
- Tìm nạp dữ liệu với Spring Data JPA DTO Projections
Show More