Hướng dẫn sử dụng Spring Boot và Groovy
1. Groovy là gì?
Apache Groovy là một ngôn ngữ lập trình hướng đối tượng (Object-oriented programming language), chạy trên các máy ảo Java. Nó là một ngôn ngữ động (dynamic language) với các tính năng tương tự như Python, Ruby, Perl. Nó có thể được sử dụng như một ngôn ngữ kịch bản cho nền tảng Java, và được biên dịch thành mã bycode của máy ảo Java, tương tác với mã Java và các thư viện. Groovy sử dụng cú pháp kiểu ngoặc nhọn { } giống như Java. Hầu hết các mã Java cũng có cú pháp hợp lệ với Groovy, mặc dù ngữ nghĩa có thể khác nhau.
Trong bài học này tất nhiên tôi không tập trung vào giới thiệu ngôn ngữ Groovy cũng như không sử dụng ngôn ngữ này. Nhưng Groovy cung cấp một template (mẫu) để tạo ra các tài liệu HTML, và đây mới là chủ đề mà chúng ta thảo luận trong bài học.
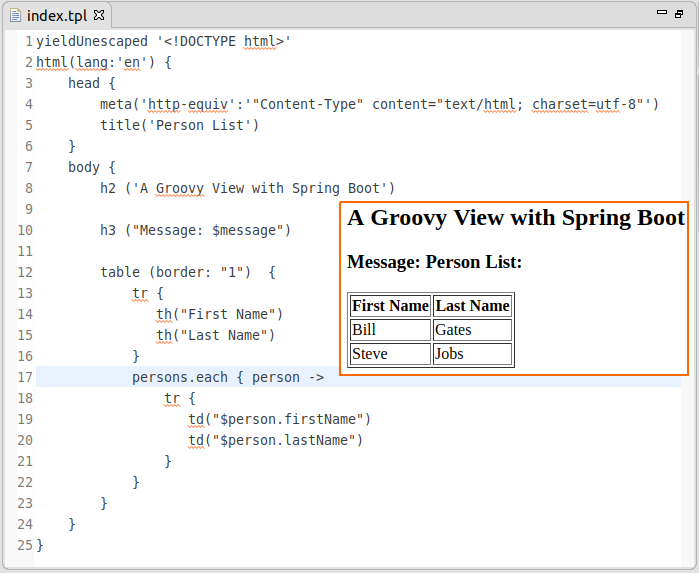
Mục tiêu của bài học này là tạo một ứng dụng Java Web sử dụng Spring Boot và sử dụng Groovy Template cho tầng View, tất nhiên đối với tầng View bạn có thể lựa chọn các công nghệ khác như JSP, Thymeleaf...
2. Tạo dự án Spring Boot
Trên Eclipse tạo một dự án Spring Boot:
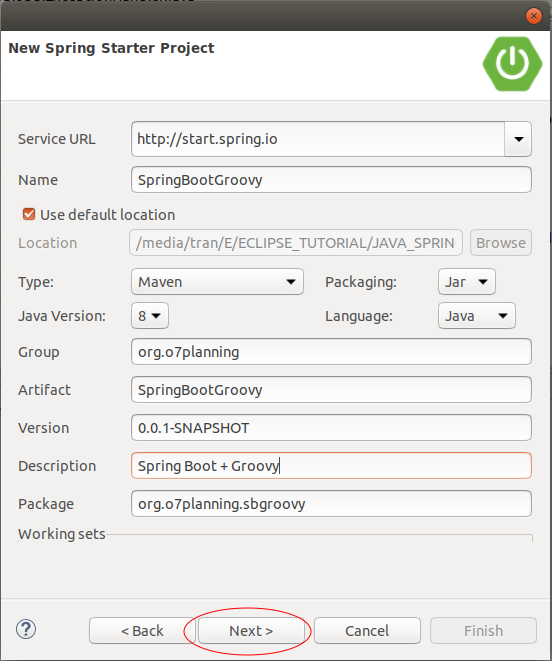
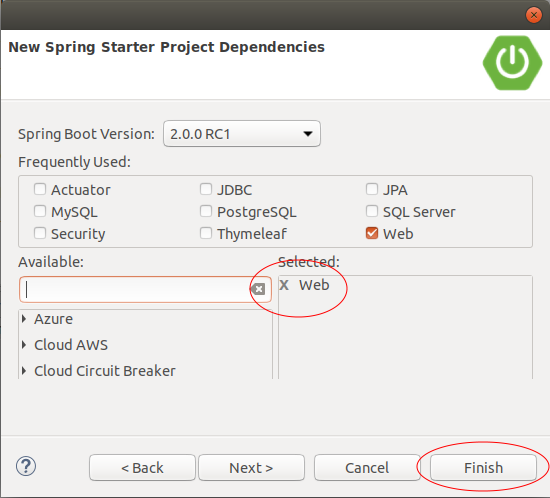
Để sử dụng Groovy bạn cần thêm phụ thuộc spring-boot-starter-groovy-templates vào project của bạn.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-groovy-templates</artifactId>
</dependency>
Nội dung đầy đủ của tập tin pom.xml:
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SpringBootGroovy</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>SpringBootGroovy</name>
<description>Spring Boot + Groovy</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-groovy-templates</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SpringBootGroovyApplication.java
package org.o7planning.sbgroovy;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootGroovyApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootGroovyApplication.class, args);
}
}
3. Controller, Groovy Template
Tạo một tập tin index.tpl trong thư mục templates.
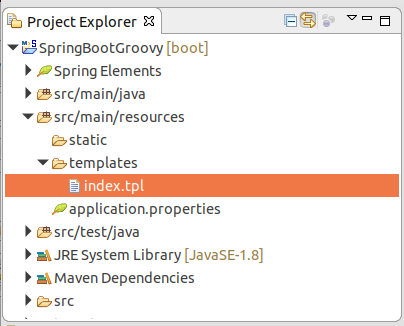
index.tpl
yieldUnescaped '<!DOCTYPE html>'
html(lang:'en') {
head {
meta('http-equiv':'"Content-Type" content="text/html; charset=utf-8"')
title('Person List')
}
body {
h2 ('A Groovy View with Spring Boot')
h3 ("Message: $message")
table (border: "1") {
tr {
th("First Name")
th("Last Name")
}
persons.each { person ->
tr {
td("$person.firstName")
td("$person.lastName")
}
}
}
}
}
MainController.java
package org.o7planning.sbgroovy.controller;
import java.util.ArrayList;
import java.util.List;
import org.o7planning.sbgroovy.model.Person;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
private static List<Person> persons = new ArrayList<Person>();
static {
persons.add(new Person("Bill", "Gates"));
persons.add(new Person("Steve", "Jobs"));
}
@RequestMapping(value = "/")
public String handleRequest(Model model) {
String message = "Person List:";
model.addAttribute("message", message);
model.addAttribute("persons", persons);
return "index";
}
}
Person.java
package org.o7planning.sbgroovy.model;
public class Person {
private String firstName;
private String lastName;
public Person() {
}
public Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
Hình dưới đây giải thích mối quan hệ giữa Controller và Groovy View:
TODO Image.
Các hướng dẫn Spring Boot
- Cài đặt Spring Tool Suite cho Eclipse
- Hướng dẫn lập trình Spring cho người mới bắt đầu
- Hướng dẫn lập trình Spring Boot cho người mới bắt đầu
- Các thuộc tính thông dụng của Spring Boot
- Hướng dẫn sử dụng Spring Boot và Thymeleaf
- Hướng dẫn sử dụng Spring Boot và FreeMarker
- Hướng dẫn sử dụng Spring Boot và Groovy
- Hướng dẫn sử dụng Spring Boot và Mustache
- Hướng dẫn sử dụng Spring Boot và JSP
- Hướng dẫn sử dụng Spring Boot, Apache Tiles, JSP
- Sử dụng Logging trong Spring Boot
- Giám sát ứng dụng với Spring Boot Actuator
- Tạo ứng dụng web đa ngôn ngữ với Spring Boot
- Sử dụng nhiều ViewResolver trong Spring Boot
- Sử dụng Twitter Bootstrap trong Spring Boot
- Hướng dẫn và ví dụ Spring Boot Interceptor
- Hướng dẫn sử dụng Spring Boot, Spring JDBC và Spring Transaction
- Hướng dẫn và ví dụ Spring JDBC
- Hướng dẫn sử dụng Spring Boot, JPA và Spring Transaction
- Hướng dẫn sử dụng Spring Boot và Spring Data JPA
- Hướng dẫn sử dụng Spring Boot, Hibernate và Spring Transaction
- Tương tác Spring Boot, JPA và cơ sở dữ liệu H2
- Hướng dẫn sử dụng Spring Boot và MongoDB
- Sử dụng nhiều DataSource với Spring Boot và JPA
- Sử dụng nhiều DataSource với Spring Boot và RoutingDataSource
- Tạo ứng dụng Login với Spring Boot, Spring Security, Spring JDBC
- Tạo ứng dụng Login với Spring Boot, Spring Security, JPA
- Tạo ứng dụng đăng ký tài khoản với Spring Boot, Spring Form Validation
- Ví dụ OAuth2 Social Login trong Spring Boot
- Chạy các nhiệm vụ nền theo lịch trình trong Spring
- Ví dụ CRUD Restful Web Service với Spring Boot
- Ví dụ Spring Boot Restful Client với RestTemplate
- Ví dụ CRUD với Spring Boot, REST và AngularJS
- Bảo mật Spring Boot RESTful Service sử dụng Basic Authentication
- Bảo mật Spring Boot RESTful Service sử dụng Auth0 JWT
- Ví dụ Upload file với Spring Boot
- Ví dụ Download file với Spring Boot
- Ví dụ Upload file với Spring Boot và jQuery Ajax
- Ví dụ Upload file với Spring Boot và AngularJS
- Tạo ứng dụng Web bán hàng với Spring Boot, Hibernate
- Hướng dẫn và ví dụ Spring Email
- Tạo ứng dụng Chat đơn giản với Spring Boot và Websocket
- Triển khai ứng dụng Spring Boot trên Tomcat Server
- Triển khai ứng dụng Spring Boot trên Oracle WebLogic Server
- Cài đặt chứng chỉ SSL miễn phí Let's Encrypt cho Spring Boot
- Cấu hình Spring Boot chuyển hướng HTTP sang HTTPS
- Tìm nạp dữ liệu với Spring Data JPA DTO Projections
Show More