Hướng dẫn và ví dụ Java PhantomReference
1. PhantomReference
Trong bài viết này, chúng ta sẽ thảo luận về lớp PhantomReference, trước khi bắt đầu tôi khuyến nghị bạn nên tìm hiểu về lớp WeakReference và SoftReference. Cả 3 lớp này có các đặc điểm cơ bản giống nhau và không cần thiết phải được đề cập lại một lần nữa.
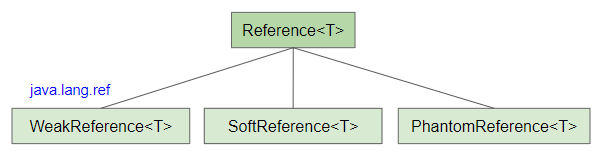
PhantomReference Constructors
PhantomReference(T innerObject, ReferenceQueue<? super T> queue)
PhantomReference chỉ có duy nhất một constructor. Để tạo một đối tượng PhantomReference bạn phải cung cấp cho 2 tham số:
- innerObject: Đối tượng sẽ được gói bên trong đối tượng PhantomReference.
- queue: Một hàng đợi được sử dụng để lưu trữ đối tượng PhantomReference này khi đối tượng innerObject của nó bị GC xoá khỏi bộ nhớ.
ReferenceQueue<AnyObject> queue = new ReferenceQueue<>();
AnyObject innerObject = new AnyObject("Obj1");
PhantomReference phantomRef = new PhantomReference(innerObject, queue);
Tất cả các phương thức của PhantomReference được thừa kế từ lớp cha.
// Methods inherited from parent.
public T get()
public void clear()
public boolean isEnqueued()
public boolean enqueue()
Phương thức phantomReference.get() luôn luôn trả về null, mục đích là ngăn chặn việc truy cập hoặc ý muốn hồi sinh một đối tượng gần như đã bị loại bỏ.
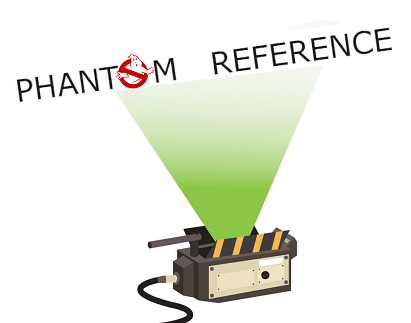
Có thể bạn đang ngạc nhiên về các đặc điểm của PhantomReference và câu hỏi của bạn lúc này là PhantomReference được sử dụng cho mục đích gì?
PhantomReference phantomRef = new PhantomReference(innerObject, queue);
Về cơ bản PhantomReference cung cấp cho bạn khả năng xác định chính xác khi nào đối tượng innerObject của nó bị xoá khỏi bộ nhớ. Phương thức phantomRef.isEnqueued() trả về true nghĩa là đối tượng innerObject đã bị xoá bỏ khỏi bộ nhớ. Khi đối tượng innerObject bị xoá khỏi bộ nhớ thì đối tượng phantomRef sẽ được đặt vào hàng đợi (queue).
Ví dụ: Nếu bạn cần cấp phát bộ nhớ để xử lý các tệp video lớn thì việc sử dụng PhantomReference là một lựa chọn đúng đắn. Ban đầu sử dụng PhantomReference cấp phát bộ nhớ để xử lý video đầu tiên, sau đó bạn cần kiểm tra để đảm bảo rằng bộ nhớ đó đã được giải phóng trước khi tiếp tục cấp phát bộ nhớ để xử lý tệp video tiếp theo. Điều này làm giảm bớt nguy cơ nhận được OutOfMemoryError.
Lớp VideoProcessor mô phỏng việc xử lý một tệp video kích thước lớn:
VideoProcessor.java
package org.o7planning.phantomreference.ex;
public class VideoProcessor {
private String video;
public VideoProcessor(String video) {
this.video = video;
System.out.println("\nNew VideoProcessor: " + this.video);
}
public void process() {
System.out.println(" >>> Processing video: " + this.video);
try {
Thread.sleep(2000);
} catch (InterruptedException e) { }
System.out.println(" >>> Completed processing video: " + this.video);
}
// !IMPORTANT: Do not override finalize() method.
// (Java9+: If you override this method, PhantomReference will not work!!)
// @Override
// protected void finalize() throws Throwable {
// System.out.println("VideoProcessor is being removed from memory\n");
// super.finalize();
// }
}
PhantomReferenceEx1.java
package org.o7planning.phantomreference.ex;
import java.lang.ref.PhantomReference;
import java.lang.ref.Reference;
import java.lang.ref.ReferenceQueue;
public class PhantomReferenceEx1 {
public static void main(String[] args) {
String[] videos = new String[] { "video1.mp4", "video2.mp4", "video3.mp4" };
ReferenceQueue<VideoProcessor> queue = new ReferenceQueue<VideoProcessor>();
for (String video : videos) {
VideoProcessor videoProcessor = new VideoProcessor(video);
PhantomReference<VideoProcessor> phantomRef = new PhantomReference<>(videoProcessor, queue);
videoProcessor.process();
videoProcessor = null;
// Call GC:
System.gc();
while (true) {
boolean isEnqueued = phantomRef.isEnqueued();
System.out.println(" phantomRef.isEnqueued: " + isEnqueued);
if (!isEnqueued) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
}
continue;
}
break;
}
}
System.out.println("\nObjects in the queue:");
Reference<? extends VideoProcessor> ref= null;
while((ref = queue.poll())!= null) {
System.out.println(ref);
}
}
}
Output:
New VideoProcessor: video1.mp4
>>> Processing video: video1.mp4
>>> Completed processing video: video1.mp4
phantomRef.isEnqueued: false
phantomRef.isEnqueued: true
New VideoProcessor: video2.mp4
>>> Processing video: video2.mp4
>>> Completed processing video: video2.mp4
phantomRef.isEnqueued: false
phantomRef.isEnqueued: true
New VideoProcessor: video3.mp4
>>> Processing video: video3.mp4
>>> Completed processing video: video3.mp4
phantomRef.isEnqueued: false
phantomRef.isEnqueued: true
Objects in the queue:
java.lang.ref.PhantomReference@5e265ba4
java.lang.ref.PhantomReference@156643d4
java.lang.ref.PhantomReference@123a439b
Java cơ bản
- Bắt đầu với Java cần những gì?
- Lịch sử của Java và sự khác biệt giữa Oracle JDK và OpenJDK
- Cài đặt Java trên Windows
- Cài đặt Java trên Ubuntu
- Cài đặt OpenJDK trên Ubuntu
- Cài đặt Eclipse
- Cài đặt Eclipse trên Ubuntu
- Cấu hình Eclipse để sử dụng JDK thay vì JRE
- Học nhanh Java cho người mới bắt đầu
- Lịch sử của bit và byte trong khoa học máy tính
- Các kiểu dữ liệu trong Java
- Các toán tử Bitwise
- Câu lệnh rẽ nhánh (if else) trong Java
- Câu lệnh rẽ nhánh switch trong Java
- Vòng lặp trong Java
- Mảng (Array) trong Java
- JDK Javadoc định dạng CHM
- Thừa kế và đa hình trong Java
- Abstract class và Interface trong Java
- Access modifier trong Java
- Hướng dẫn và ví dụ Java Enum
- Hướng dẫn và ví dụ Java Annotation
- Tùy biến trình biên dịch java xử lý Annotation của bạn (Annotation Processing Tool)
- So sánh và sắp xếp trong Java
- Hướng dẫn và ví dụ Java String, StringBuffer và StringBuilder
- Phương thức String.format() và printf() trong Java
- Hướng dẫn xử lý ngoại lệ trong Java - Java Exception Handling
- Hướng dẫn và ví dụ Java Generics
- Tìm hiểu về Java System.identityHashCode, Object.hashCode và Object.equals
- Hướng dẫn và ví dụ Java WeakReference
- Hướng dẫn và ví dụ Java SoftReference
- Hướng dẫn và ví dụ Java PhantomReference
- Thao tác với tập tin và thư mục trong Java
- Cú pháp và các tính năng mới trong Java 5
- Cú pháp và các tính năng mới trong Java 8
- Hướng dẫn sử dụng biểu thức chính quy trong Java
- Hướng dẫn lập trình đa luồng trong Java - Java Multithreading
- Thư viện điều khiển các loại cơ sở dữ liệu khác nhau trong Java
- Hướng dẫn sử dụng Java JDBC kết nối cơ sở dữ liệu
- Lấy các giá trị của các cột tự động tăng khi Insert một bản ghi sử dụng JDBC
- Hướng dẫn nén và giải nén trong Java
- Hướng dẫn và ví dụ Java Reflection
- Hướng dẫn gọi phương thức từ xa với Java RMI
- Hướng dẫn lập trình Java Socket
- Hướng dẫn và ví dụ Java Stream
- Functional Interface trong Java
- Hướng dẫn và ví dụ Java Predicate
- Hướng dẫn và ví dụ Java BiPredicate
- Hướng dẫn và ví dụ Java Consumer
- Hướng dẫn và ví dụ Java BiConsumer
- Hướng dẫn và ví dụ Java Supplier
- Hướng dẫn và ví dụ Java Function
- Hướng dẫn và ví dụ Java BiFunction
- Lập trình Java hướng khía cạnh với AspectJ (AOP)
- Lập trình Java theo nhóm sử dụng Eclipse và SVN
- Các nền tảng nào bạn nên chọn để lập trình ứng dụng Java Desktop?
- Ví dụ về Java encoding và decoding sử dụng Apache Base64
- Hướng dẫn và ví dụ Java Commons IO
- Hướng dẫn và ví dụ Java Commons Email
- Hướng dẫn và ví dụ Java Commons Logging
- Giới thiệu về Raspberry Pi
Show More
- Hướng dẫn lập trình Java Servlet/JSP
- Các hướng dẫn Java Collections Framework
- Java API cho HTML & XML
- Các hướng dẫn Java IO
- Các hướng dẫn Java Date Time
- Các hướng dẫn Spring Boot
- Các hướng dẫn Maven
- Các hướng dẫn Gradle
- Các hướng dẫn Java Web Services
- Các hướng dẫn lập trình Java SWT
- Các hướng dẫn lập trình JavaFX
- Các hướng dẫn Java Oracle ADF
- Các hướng dẫn Struts2 Framework
- Các hướng dẫn Spring Cloud