Hướng dẫn và ví dụ JavaFX StackPane Layout
1. StackPane Layout
StackPane là một bộ chứa, nó có thể chứa các thành phần giao diện khác, các thành phần con được sếp chồng nên nhau, tại một thời điểm bạn chỉ có thể nhìn thấy thành phần con nằm ở trên cùng của stack.
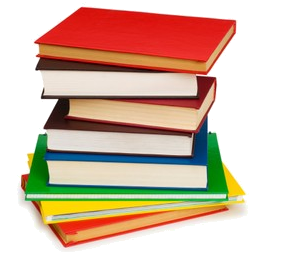
Các thành phần con được thêm vào mới nhất sẽ nằm ở phía trên cùng của Stack.
// Thêm một thành phần con vào Stack.
stackPane.getChildren().add(newChild);
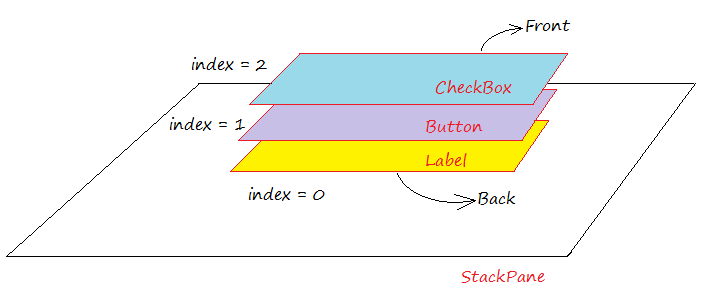
Bạn có thể đưa một thành phần con bất kỳ nên phía trước (front) của Stack thông qua phương thức toFront(), hoặc đưa thành phần con xuống phía cuối của stack thông qua phương thức toBack().
// Tất cả các thành phần con của StackPane
ObservableList<Node> childs = stackPane.getChildren();
if (childs.size() > 1) {
// Thành phần trên cùng.
Node topNode = childs.get(childs.size()-1);
topNode.toBack();
}
2. Ví dụ với StackPane
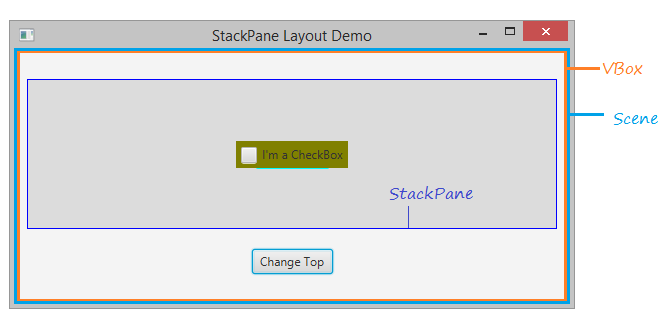
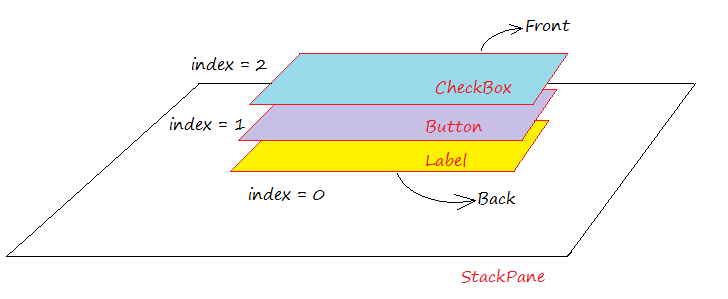
StackPaneDemo.java
package org.o7planning.javafx.stackpane;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class StackPaneDemo extends Application {
private StackPane stackPane;
@Override
public void start(Stage primaryStage) throws Exception {
VBox root = new VBox();
// StackPane
stackPane = new StackPane();
// Thêm Label vào StackPane
Label label = new Label("I'm a Label");
label.setStyle("-fx-background-color:yellow");
label.setPadding(new Insets(5,5,5,5));
stackPane.getChildren().add(label);
// Thêm Button vào StackPane
Button button = new Button("I'm a Button");
button.setStyle("-fx-background-color: cyan");
button.setPadding(new Insets(5,5,5,5));
stackPane.getChildren().add(button);
// Thêm CheckBox vào StackPane
CheckBox checkBox = new CheckBox("I'm a CheckBox");
checkBox.setOpacity(1);
checkBox.setStyle("-fx-background-color:olive");
checkBox.setPadding(new Insets(5,5,5,5));
stackPane.getChildren().add(checkBox);
//
stackPane.setPrefSize(300, 150);
stackPane.setStyle("-fx-background-color: Gainsboro;-fx-border-color: blue;");
//
root.getChildren().add(stackPane);
Button controlButton = new Button("Change Top");
root.getChildren().add(controlButton);
root.setAlignment(Pos.CENTER);
VBox.setMargin(stackPane, new Insets(10, 10, 10, 10));
VBox.setMargin(controlButton, new Insets(10, 10, 10, 10));
//
Scene scene = new Scene(root, 550, 250);
primaryStage.setTitle("StackPane Layout Demo");
primaryStage.setScene(scene);
controlButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
changeTop();
}
});
primaryStage.show();
}
private void changeTop() {
ObservableList<Node> childs = this.stackPane.getChildren();
if (childs.size() > 1) {
//
Node topNode = childs.get(childs.size()-1);
topNode.toBack();
}
}
public static void main(String[] args) {
launch(args);
}
}
Chạy ví dụ:
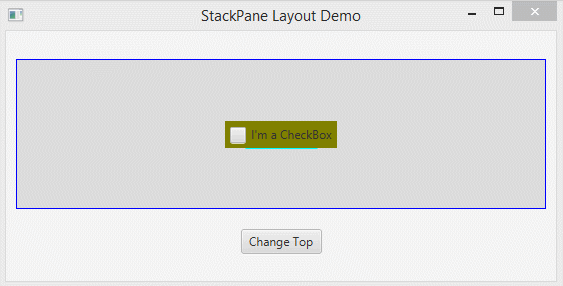
Thông thường bạn chỉ hiển thị thành phần con phía trên cùng của StackPane, và ẩn các thành phần con khác. Sử dụng phương thức Node.setVisible(visible). Xem ví dụ minh họa:
StackPaneDemo2.java
package org.o7planning.javafx.stackpane;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class StackPaneDemo2 extends Application {
private StackPane stackPane;
@Override
public void start(Stage primaryStage) throws Exception {
VBox root = new VBox();
// StackPane
stackPane = new StackPane();
// Thêm Label vào StackPane
Label label = new Label("I'm a Label");
label.setStyle("-fx-background-color:yellow");
label.setPadding(new Insets(5,5,5,5));
// Ẩn label này.
label.setVisible(false);
stackPane.getChildren().add(label);
// Thêm Button vào StackPane
Button button = new Button("I'm a Button");
button.setStyle("-fx-background-color: cyan");
button.setPadding(new Insets(5,5,5,5));
// Ẩn button này.
button.setVisible(false);
stackPane.getChildren().add(button);
// Thêm CheckBox vào StackPane
CheckBox checkBox = new CheckBox("I'm a CheckBox");
checkBox.setOpacity(1);
checkBox.setStyle("-fx-background-color:olive");
checkBox.setPadding(new Insets(5,5,5,5));
// Hiển thị checkBox (mặc định).
checkBox.setVisible(true);
stackPane.getChildren().add(checkBox);
//
stackPane.setPrefSize(300, 150);
stackPane.setStyle("-fx-background-color: Gainsboro;-fx-border-color: blue;");
//
root.getChildren().add(stackPane);
Button controlButton = new Button("Change Top");
root.getChildren().add(controlButton);
root.setAlignment(Pos.CENTER);
VBox.setMargin(stackPane, new Insets(10, 10, 10, 10));
VBox.setMargin(controlButton, new Insets(10, 10, 10, 10));
//
Scene scene = new Scene(root, 550, 250);
primaryStage.setTitle("StackPane Layout Demo 2");
primaryStage.setScene(scene);
controlButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
changeTop();
}
});
primaryStage.show();
}
private void changeTop() {
ObservableList<Node> childs = this.stackPane.getChildren();
if (childs.size() > 1) {
//
Node topNode = childs.get(childs.size()-1);
// Node này sẽ được đưa lên phía trước.
Node newTopNode = childs.get(childs.size()-2);
topNode.setVisible(false);
topNode.toBack();
newTopNode.setVisible(true);
}
}
public static void main(String[] args) {
launch(args);
}
}
Chạy ví dụ:
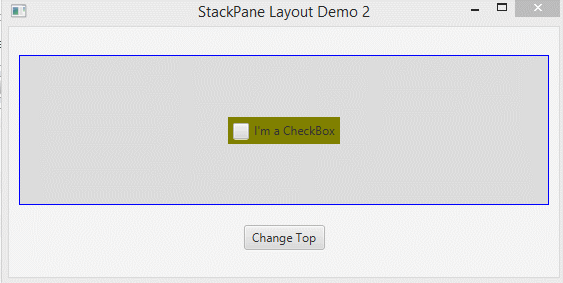
Các hướng dẫn lập trình JavaFX
- Cài đặt e(fx)clipse cho Eclipse (Bộ công cụ lập trình JavaFX)
- Cài đặt JavaFX Scene Builder cho Eclipse
- Hướng dẫn lập trình JavaFX cho người mới bắt đầu - Hello JavaFX
- Hướng dẫn và ví dụ JavaFX FlowPane Layout
- Hướng dẫn và ví dụ JavaFX TilePane Layout
- Hướng dẫn và ví dụ JavaFX HBox, VBox Layout
- Hướng dẫn và ví dụ JavaFX BorderPane Layout
- Hướng dẫn và ví dụ JavaFX AnchorPane Layout
- Hướng dẫn và ví dụ JavaFX GridPane Layout
- Hướng dẫn và ví dụ JavaFX StackPane Layout
- Hướng dẫn và ví dụ JavaFX TitledPane
- Hướng dẫn và ví dụ JavaFX ScrollPane
- Hướng dẫn và ví dụ JavaFX Accordion
- Hướng dẫn và ví dụ JavaFX ListView
- Hướng dẫn và ví dụ JavaFX Group
- Hướng dẫn và ví dụ JavaFX ComboBox
- Hướng dẫn và ví dụ JavaFX WebView và WebEngine
- Hướng dẫn và ví dụ JavaFX HTMLEditor
- Hướng dẫn và ví dụ JavaFX TableView
- Hướng dẫn và ví dụ JavaFX TreeView
- Hướng dẫn và ví dụ JavaFX TreeTableView
- Hướng dẫn và ví dụ JavaFX Menu
- Hướng dẫn và ví dụ JavaFX ContextMenu
- Hướng dẫn và ví dụ JavaFX Image và ImageView
- Hướng dẫn và ví dụ JavaFX Label
- Hướng dẫn và ví dụ JavaFX Hyperlink
- Hướng dẫn và ví dụ JavaFX Button
- Hướng dẫn và ví dụ JavaFX ToggleButton
- Hướng dẫn và ví dụ JavaFX RadioButton
- Hướng dẫn và ví dụ JavaFX MenuButton và SplitMenuButton
- Hướng dẫn và ví dụ JavaFX TextField
- Hướng dẫn và ví dụ JavaFX PasswordField
- Hướng dẫn và ví dụ JavaFX TextArea
- Hướng dẫn và ví dụ JavaFX Slider
- Hướng dẫn và ví dụ JavaFX Spinner
- Hướng dẫn và ví dụ JavaFX ProgressBar và ProgressIndicator
- Hướng dẫn và ví dụ JavaFX ChoiceBox
- Hướng dẫn và ví dụ JavaFX Tooltip
- Hướng dẫn và ví dụ JavaFX DatePicker
- Hướng dẫn và ví dụ JavaFX ColorPicker
- Hướng dẫn và ví dụ JavaFX FileChooser và DirectoryChooser
- Mở một cửa sổ (window) mới trong JavaFX
- Hướng dẫn và ví dụ JavaFX Alert Dialog
- Hướng dẫn và ví dụ JavaFX TextInputDialog
- Hướng dẫn và ví dụ JavaFX ChoiceDialog
- Hướng dẫn và ví dụ JavaFX PieChart
- Hướng dẫn và ví dụ JavaFX AreaChart và StackedAreaChart
- Hướng dẫn và ví dụ JavaFX BarChart và StackedBarChart
- Hướng dẫn và ví dụ JavaFX Line
- Hướng dẫn và ví dụ JavaFX Rectangle và Ellipse
- Các hiệu ứng (effects) trong JavaFX
- Hướng dẫn sử dụng các phép biến hình (Transformations) trong JavaFX
Show More