Hướng dẫn và ví dụ JavaFX HTMLEditor
1. JavaFX HTMLEditor
JavaFX HTMLEditor là một trình soạn thảo văn bản có đầy đủ các chức năng của một rich text editor. Thực hiện của nó là dựa trên các tính năng chỉnh sửa tài liệu của HTML5 và bao gồm các chức năng chỉnh sửa như sau:
- Các định dạng văn bản như chữ đậm (bold), chữ nghiêng (italic), gạch chân (underline), và gạch ngang
- Sét đặt khối văn bản (Paragraph) như định dạng, font, và kích thước.
- Mầu chữ và mầu nền
- Thụt văn bản (Text indent)
- Chấm đầu dòng và đánh chỉ số danh sách (Bulleted and numbered lists)
- Căn lề văn bản (Text alignment).
- Thêm thanh ngang (<hr> - horizontal rule).
- Sao chép và dán một đoạn văn bản
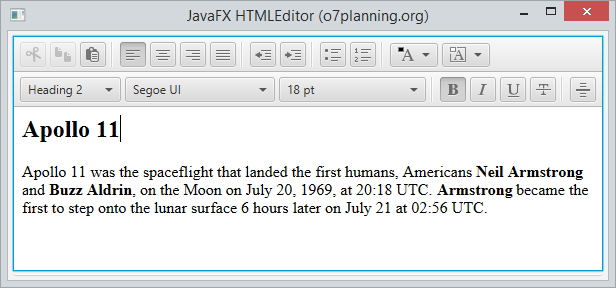
// Tạo HTMLEditor
HTMLEditor htmlEditor = new HTMLEditor();
htmlEditor.setPrefHeight(245);
String INITIAL_TEXT = "<h2>Apollo 11</h2>" //
+ "Apollo 11 was the spaceflight that landed the first humans,"//
+ " Americans <a href='http://en.wikipedia.org/wiki/Neil_Armstrong'>Neil Armstrong</a>"
+ " and <a href='http://en.wikipedia.org/wiki/Buzz_Aldrin'>Buzz Aldrin</a>,"//
+ " on the Moon on July 20, 1969, at 20:18 UTC."//
+ " <b>Armstrong</b> became the first to step onto"//
+ " the lunar surface 6 hours later on July 21 at 02:56 UTC.";
// Set HTML
htmlEditor.setHtmlText(INITIAL_TEXT);
// Get HTML
String html = htmlEditor.getHtmlText();
2. Ví dụ HTMLEditor
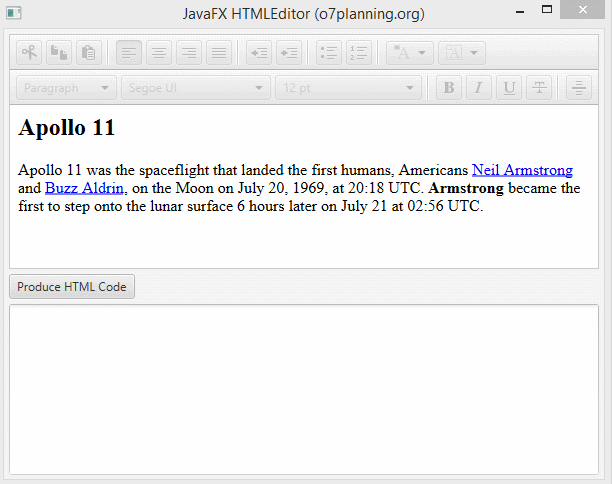
HTMLEditorDemo.java
package org.o7planning.javafx.htmleditor;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.scene.web.HTMLEditor;
import javafx.stage.Stage;
public class HTMLEditorDemo extends Application {
@Override
public void start(Stage stage) {
HTMLEditor htmlEditor = new HTMLEditor();
htmlEditor.setPrefHeight(245);
String INITIAL_TEXT = "<h2>Apollo 11</h2>" //
+ "Apollo 11 was the spaceflight that landed the first humans,"//
+ " Americans <a href='http://en.wikipedia.org/wiki/Neil_Armstrong'>Neil Armstrong</a>"
+ " and <a href='http://en.wikipedia.org/wiki/Buzz_Aldrin'>Buzz Aldrin</a>,"//
+ " on the Moon on July 20, 1969, at 20:18 UTC."//
+ " <b>Armstrong</b> became the first to step onto"//
+ " the lunar surface 6 hours later on July 21 at 02:56 UTC.";
htmlEditor.setHtmlText(INITIAL_TEXT);
Button showHTMLButton = new Button("Produce HTML Code");
TextArea textArea = new TextArea();
textArea.setWrapText(true);
//
showHTMLButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textArea.setText(htmlEditor.getHtmlText());
}
});
VBox root = new VBox();
root.setPadding(new Insets(5));
root.setSpacing(5);
root.getChildren().addAll(htmlEditor, showHTMLButton, textArea);
Scene scene = new Scene(root, 600, 450);
stage.setTitle("JavaFX HTMLEditor (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
3. HTMLEditor và WebView
HTMLEditor là một trình soạn thảo HTML trong khi đó WebView là một trình duyệt mini. Bạn có thể chỉnh sửa nội dung HTML trên HTMLEditor và hiển thị nó trên WebView. Hãy xem một ví dụ minh họa:
Xem thêm WebView:
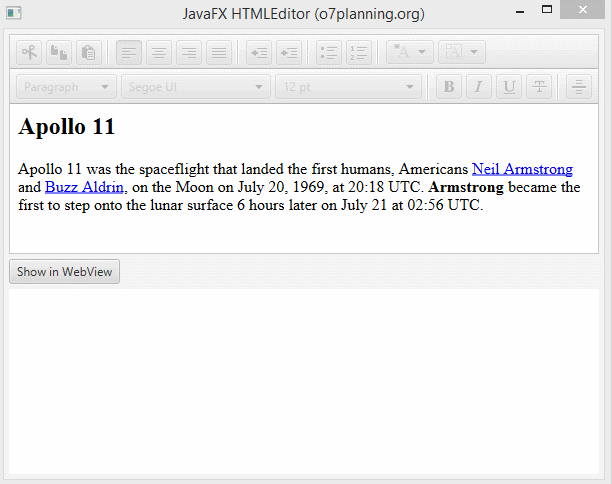
HTMLEditorWebViewDemo.java
package org.o7planning.javafx.htmleditor;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.scene.web.HTMLEditor;
import javafx.scene.web.WebEngine;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
public class HTMLEditorWebViewDemo extends Application {
@Override
public void start(Stage stage) {
// HTML Editor.
HTMLEditor htmlEditor = new HTMLEditor();
htmlEditor.setPrefHeight(245);
htmlEditor.setMinHeight(220);
String INITIAL_TEXT = "<h2>Apollo 11</h2>" //
+ "Apollo 11 was the spaceflight that landed the first humans,"//
+ " Americans <a href='http://en.wikipedia.org/wiki/Neil_Armstrong'>Neil Armstrong</a>"
+ " and <a href='http://en.wikipedia.org/wiki/Buzz_Aldrin'>Buzz Aldrin</a>,"//
+ " on the Moon on July 20, 1969, at 20:18 UTC."//
+ " <b>Armstrong</b> became the first to step onto"//
+ " the lunar surface 6 hours later on July 21 at 02:56 UTC.";
htmlEditor.setHtmlText(INITIAL_TEXT);
Button showHTMLButton = new Button("Show in WebView");
// WebView
WebView webView = new WebView();
WebEngine webEngine = webView.getEngine();
//
showHTMLButton.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
webEngine.loadContent(htmlEditor.getHtmlText(), "text/html");
}
});
VBox root = new VBox();
root.setPadding(new Insets(5));
root.setSpacing(5);
root.getChildren().addAll(htmlEditor, showHTMLButton, webView);
Scene scene = new Scene(root, 600, 450);
stage.setTitle("JavaFX HTMLEditor (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Các hướng dẫn lập trình JavaFX
- Mở một cửa sổ (window) mới trong JavaFX
- Hướng dẫn và ví dụ JavaFX ChoiceDialog
- Hướng dẫn và ví dụ JavaFX Alert Dialog
- Hướng dẫn và ví dụ JavaFX TextInputDialog
- Cài đặt e(fx)clipse cho Eclipse (Bộ công cụ lập trình JavaFX)
- Cài đặt JavaFX Scene Builder cho Eclipse
- Hướng dẫn lập trình JavaFX cho người mới bắt đầu - Hello JavaFX
- Hướng dẫn và ví dụ JavaFX FlowPane Layout
- Hướng dẫn và ví dụ JavaFX TilePane Layout
- Hướng dẫn và ví dụ JavaFX HBox, VBox Layout
- Hướng dẫn và ví dụ JavaFX BorderPane Layout
- Hướng dẫn và ví dụ JavaFX AnchorPane Layout
- Hướng dẫn và ví dụ JavaFX TitledPane
- Hướng dẫn và ví dụ JavaFX Accordion
- Hướng dẫn và ví dụ JavaFX ListView
- Hướng dẫn và ví dụ JavaFX Group
- Hướng dẫn và ví dụ JavaFX ComboBox
- Hướng dẫn sử dụng các phép biến hình (Transformations) trong JavaFX
- Các hiệu ứng (effects) trong JavaFX
- Hướng dẫn và ví dụ JavaFX GridPane Layout
- Hướng dẫn và ví dụ JavaFX StackPane Layout
- Hướng dẫn và ví dụ JavaFX ScrollPane
- Hướng dẫn và ví dụ JavaFX WebView và WebEngine
- Hướng dẫn và ví dụ JavaFX HTMLEditor
- Hướng dẫn và ví dụ JavaFX TableView
- Hướng dẫn và ví dụ JavaFX TreeView
- Hướng dẫn và ví dụ JavaFX TreeTableView
- Hướng dẫn và ví dụ JavaFX Menu
- Hướng dẫn và ví dụ JavaFX ContextMenu
- Hướng dẫn và ví dụ JavaFX Image và ImageView
- Hướng dẫn và ví dụ JavaFX Label
- Hướng dẫn và ví dụ JavaFX Hyperlink
- Hướng dẫn và ví dụ JavaFX Button
- Hướng dẫn và ví dụ JavaFX ToggleButton
- Hướng dẫn và ví dụ JavaFX RadioButton
- Hướng dẫn và ví dụ JavaFX MenuButton và SplitMenuButton
- Hướng dẫn và ví dụ JavaFX TextField
- Hướng dẫn và ví dụ JavaFX PasswordField
- Hướng dẫn và ví dụ JavaFX TextArea
- Hướng dẫn và ví dụ JavaFX Slider
- Hướng dẫn và ví dụ JavaFX Spinner
- Hướng dẫn và ví dụ JavaFX ProgressBar và ProgressIndicator
- Hướng dẫn và ví dụ JavaFX ChoiceBox
- Hướng dẫn và ví dụ JavaFX Tooltip
- Hướng dẫn và ví dụ JavaFX DatePicker
- Hướng dẫn và ví dụ JavaFX ColorPicker
- Hướng dẫn và ví dụ JavaFX FileChooser và DirectoryChooser
- Hướng dẫn và ví dụ JavaFX PieChart
- Hướng dẫn và ví dụ JavaFX AreaChart và StackedAreaChart
- Hướng dẫn và ví dụ JavaFX BarChart và StackedBarChart
- Hướng dẫn và ví dụ JavaFX Line
- Hướng dẫn và ví dụ JavaFX Rectangle và Ellipse
Show More