Hướng dẫn và ví dụ JavaFX TextField
1. JavaFX TextField
Class TextField thực hiện một điều khiển giao diện người dùng (UI Control) chấp nhận và hiển thị đầu vào văn bản. Nó cung cấp khả năng tiếp nhận đầu vào văn bản từ một người sử dụng. Một class tương tự khác là PasswordField cho phép người dùng nhập mật khẩu, lớp này mở rộng lớp TextInput
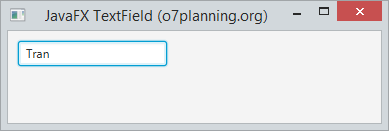
Xem một vài phương thức hữu ích bạn có thể sử dụng với TextField.
- clear() - Xóa bỏ văn bản trên TextField.
- copy() - Copy đoạn text đang được bôi đen trên TextField vào Clipboard.
- cut() - Cắt một đoạn text đang được bôi đen trên TextField và lưu vào Clipboard, đồng thời di chuyển vị trí con trỏ.
- paste() - Dán nội dung text trên Clipboard vào TextField tại vị trí con trỏ, thay thế đoạn text đang được bôi đen nếu có.
2. Ví dụ TextField
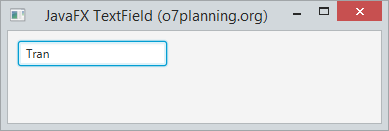
TextFieldDemo.java
package org.o7planning.javafx.textfield;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class TextFieldDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
TextField textField = new TextField("Tran");
textField.setMinWidth(120);
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.getChildren().add(textField);
Scene scene = new Scene(root, 200, 100);
primaryStage.setTitle("JavaFX TextField (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
3. Các phương thức hữu ích
Ví dụ dưới đây minh họa sử dụng các phương thức clear(),copy(), paste(), cut(), chúng là các phương thức hữu ích của TextField.
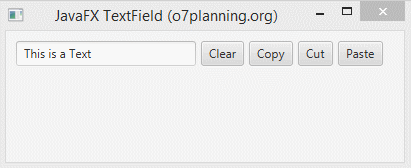
TextFieldDemo2.java
package org.o7planning.javafx.textfield;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class TextFieldDemo2 extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
TextField textField = new TextField("This is a Text");
textField.setMinWidth(180);
// Clear
Button buttonClear = new Button("Clear");
buttonClear.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.clear();
}
});
// Copy
Button buttonCopy = new Button("Copy");
// Nhấn vào nút này không làm mất tiêu điểm (Focus) của thành phần khác.
buttonCopy.setFocusTraversable(false);
buttonCopy.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.copy();
}
});
// Cut
Button buttonCut = new Button("Cut");
// Nhấn vào nút này không làm mất tiêu điểm (Focus) của thành phần khác.
buttonCut.setFocusTraversable(false);
buttonCut.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.cut();
}
});
// Paste
Button buttonPaste = new Button("Paste");
buttonPaste.setFocusTraversable(false);
buttonPaste.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
textField.paste();
}
});
FlowPane root = new FlowPane();
root.setPadding(new Insets(10));
root.setVgap(5);
root.setHgap(5);
root.getChildren().addAll(textField, buttonClear,
buttonCopy, buttonCut, buttonPaste);
Scene scene = new Scene(root, 200, 100);
primaryStage.setTitle("JavaFX TextField (o7planning.org)");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
Các hướng dẫn lập trình JavaFX
- Mở một cửa sổ (window) mới trong JavaFX
- Hướng dẫn và ví dụ JavaFX ChoiceDialog
- Hướng dẫn và ví dụ JavaFX Alert Dialog
- Hướng dẫn và ví dụ JavaFX TextInputDialog
- Cài đặt e(fx)clipse cho Eclipse (Bộ công cụ lập trình JavaFX)
- Cài đặt JavaFX Scene Builder cho Eclipse
- Hướng dẫn lập trình JavaFX cho người mới bắt đầu - Hello JavaFX
- Hướng dẫn và ví dụ JavaFX FlowPane Layout
- Hướng dẫn và ví dụ JavaFX TilePane Layout
- Hướng dẫn và ví dụ JavaFX HBox, VBox Layout
- Hướng dẫn và ví dụ JavaFX BorderPane Layout
- Hướng dẫn và ví dụ JavaFX AnchorPane Layout
- Hướng dẫn và ví dụ JavaFX TitledPane
- Hướng dẫn và ví dụ JavaFX Accordion
- Hướng dẫn và ví dụ JavaFX ListView
- Hướng dẫn và ví dụ JavaFX Group
- Hướng dẫn và ví dụ JavaFX ComboBox
- Hướng dẫn sử dụng các phép biến hình (Transformations) trong JavaFX
- Các hiệu ứng (effects) trong JavaFX
- Hướng dẫn và ví dụ JavaFX GridPane Layout
- Hướng dẫn và ví dụ JavaFX StackPane Layout
- Hướng dẫn và ví dụ JavaFX ScrollPane
- Hướng dẫn và ví dụ JavaFX WebView và WebEngine
- Hướng dẫn và ví dụ JavaFX HTMLEditor
- Hướng dẫn và ví dụ JavaFX TableView
- Hướng dẫn và ví dụ JavaFX TreeView
- Hướng dẫn và ví dụ JavaFX TreeTableView
- Hướng dẫn và ví dụ JavaFX Menu
- Hướng dẫn và ví dụ JavaFX ContextMenu
- Hướng dẫn và ví dụ JavaFX Image và ImageView
- Hướng dẫn và ví dụ JavaFX Label
- Hướng dẫn và ví dụ JavaFX Hyperlink
- Hướng dẫn và ví dụ JavaFX Button
- Hướng dẫn và ví dụ JavaFX ToggleButton
- Hướng dẫn và ví dụ JavaFX RadioButton
- Hướng dẫn và ví dụ JavaFX MenuButton và SplitMenuButton
- Hướng dẫn và ví dụ JavaFX TextField
- Hướng dẫn và ví dụ JavaFX PasswordField
- Hướng dẫn và ví dụ JavaFX TextArea
- Hướng dẫn và ví dụ JavaFX Slider
- Hướng dẫn và ví dụ JavaFX Spinner
- Hướng dẫn và ví dụ JavaFX ProgressBar và ProgressIndicator
- Hướng dẫn và ví dụ JavaFX ChoiceBox
- Hướng dẫn và ví dụ JavaFX Tooltip
- Hướng dẫn và ví dụ JavaFX DatePicker
- Hướng dẫn và ví dụ JavaFX ColorPicker
- Hướng dẫn và ví dụ JavaFX FileChooser và DirectoryChooser
- Hướng dẫn và ví dụ JavaFX PieChart
- Hướng dẫn và ví dụ JavaFX AreaChart và StackedAreaChart
- Hướng dẫn và ví dụ JavaFX BarChart và StackedBarChart
- Hướng dẫn và ví dụ JavaFX Line
- Hướng dẫn và ví dụ JavaFX Rectangle và Ellipse
Show More