Hướng dẫn và ví dụ JavaFX Alert Dialog
1. JavaFX Alert
Lớp Alert (cảnh báo) là một lớp con của lớp Dialog (Hộp thoại) và cung cấp hỗ trợ cho một số loại dialog được xây dựng trước có thể dễ dàng hiển thị cho người dùng để nhắc nhở cho một phản hồi. Do đó, đối với nhiều người dùng, lớp Alert là lớp thích hợp nhất cho nhu cầu của họ (trái với việc sử dụng Dialog trực tiếp). Ngoài ra, nếu muốn nhắc người dùng nhập văn bản hoặc chọn từ danh sách tùy chọn (list of options) sẽ được phục vụ tốt hơn bằng cách sử dụng TextInputDialog và ChoiceDialog tương ứng.
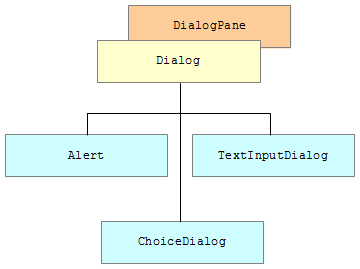
Alert dialog theo mặc định là một cửa sổ có thể thức (modelity) là Modelity.WINDOW_MODAL. Tuy nhiên bạn có thể thay đổi bằng cách sử dụng phương thức alert.initModality(Modality).
Mặc định cửa sổ nào mở ra một Alert sẽ là cửa sổ cha (cửa sổ sở hữu) của Alert đó.
Bạn có thể xem thêm giải thích về Modelity trong các bài viết dưới đây:
- Hướng dẫn và ví dụ JavaFX Dialog
Dưới đây là hình ảnh minh họa cấu trúc của cửa sổ Alert:
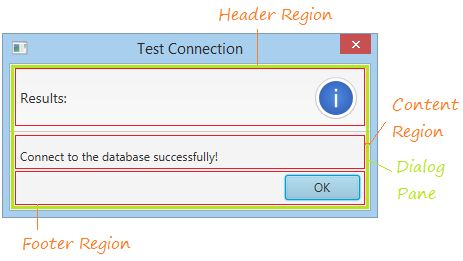
Header Region (Vùng Header):
Vùng này sử dụng để hiện thị một thông báo ngắn, và biểu tượng (icon).
Content Region (Vùng nội dung):
Mặc định Content Region hiển thị một Label, mà bạn có thể sét đăt nội dung text cho Label này thông qua phương thức alert.setContentText(String). Bạn cũng có thể hiển thị một Node khác trong Content Region thông qua alert.getDialogPane().setContent(Node).
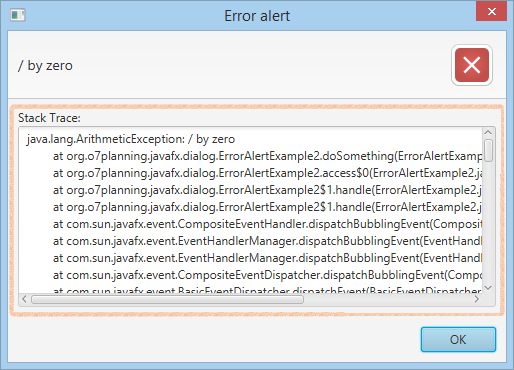
Footer Region (Vùng Footer):
Vùng này được sử dụng để hiển thị các Button, bạn có thể tùy biến các button sẽ được hiển thị.
2. Information Alert
Information Alert (Hộp thông tin) là một cửa sổ dialog hiển thị một thông tin. Dưới đây là một hình ảnh về một Information Alert tiêu chuẩn:
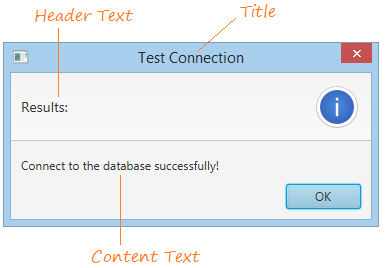
Hình ảnh về một Information Alert với Header Text mặc định:
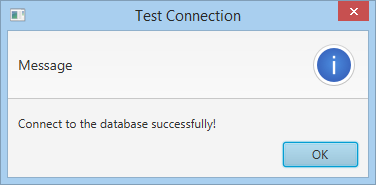
Hình ảnh một Information Alert không có Header Text:
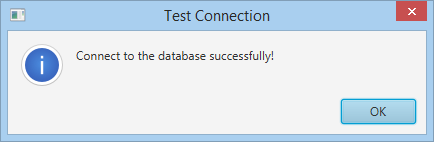
Ví dụ:
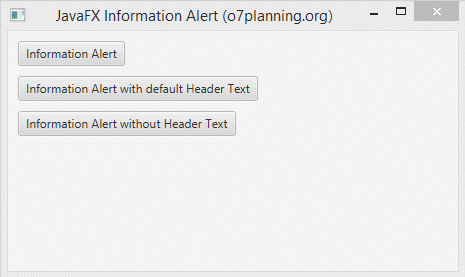
InformationAlertExample.java
package org.o7planning.javafx.alert;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class InformationAlertExample extends Application {
// Hiển thị Information Alert với Header Text
private void showAlertWithHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
alert.setHeaderText("Results:");
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
// Hiển thị Information Alert với Header Text mặc định
private void showAlertWithDefaultHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
// alert.setHeaderText("Results:");
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
// Hiển thị Information Alert không có Header Text
private void showAlertWithoutHeaderText() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Test Connection");
// Header Text: null
alert.setHeaderText(null);
alert.setContentText("Connect to the database successfully!");
alert.showAndWait();
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Information Alert");
Button button2 = new Button("Information Alert with default Header Text");
Button button3 = new Button("Information Alert without Header Text");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithHeaderText();
}
});
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithDefaultHeaderText();
}
});
button3.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithoutHeaderText();
}
});
root.getChildren().addAll(button1, button2, button3);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Information Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
3. Warning Alert
Warning Alert rất giống với một Information Alert, ngoại trừ biểu tượng của nó và mục đích sử dụng. Warning Alert sử dụng để cảnh báo với người dùng những nguy cơ hoặc vấn đề tiềm ẩn, mặc dù có thể nó không xẩy ra.
Hình ảnh một Warning Alert tiêu chuẩn:
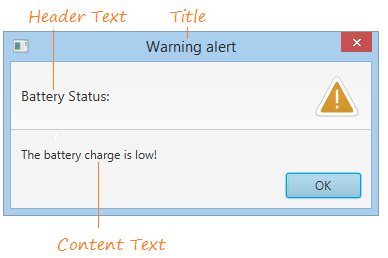
Hình ảnh một Warning Alert với Header Text mặc định:
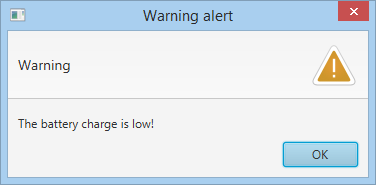
Hình ảnh một Warning Alert không có Header Text:
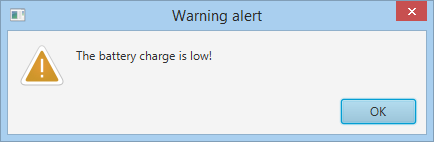
Ví dụ:
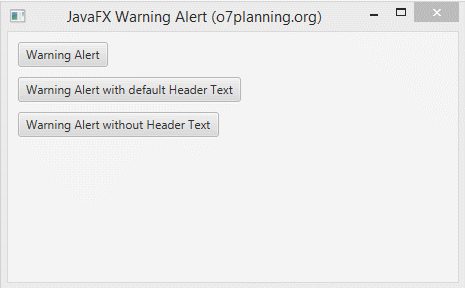
WarningAlertExample.java
package org.o7planning.javafx.alert;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class WarningAlertExample extends Application {
// Hiển thị Warning Alert với Header Text
private void showAlertWithHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
alert.setHeaderText("Battery Status:");
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
// Hiển thị Warning Alert với Header Text mặc định
private void showAlertWithDefaultHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
// alert.setHeaderText("Battery Status:");
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
// Hiển thị Warning Alert không có Header Text
private void showAlertWithoutHeaderText() {
Alert alert = new Alert(AlertType.WARNING);
alert.setTitle("Warning alert");
// Header Text: null
alert.setHeaderText(null);
alert.setContentText("The battery charge is low!");
alert.showAndWait();
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Warning Alert");
Button button2 = new Button("Warning Alert with default Header Text");
Button button3 = new Button("Warning Alert without Header Text");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithHeaderText();
}
});
button2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithDefaultHeaderText();
}
});
button3.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showAlertWithoutHeaderText();
}
});
root.getChildren().addAll(button1, button2, button3);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Warning Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
4. Error Alert
Error Alert rất giống với Informtion Alert và Warning Alert, ngoại trừ biểu tượng (icon) và mục đích sử dụng. Error Alert được sử dụng để thông báo một vấn đề nghiêm trọng đã xẩy ra.
Để tạo một Error Alert bạn cần sử dụng AlertType.ERROR.
* Error Alert *
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("Error alert");
alert.setHeaderText("Can not add user");
alert.setContentText("The 'abc' user does not exists!");
alert.showAndWait();
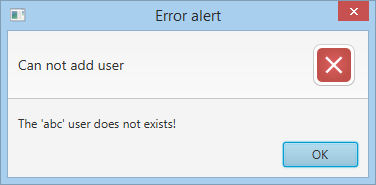
Trong thực tế khi một lỗi xẩy ra, bạn hiển thị một Error Alert cho người dùng biết, nó bao gồm các thông tin ngắn gọn. Và có thể bao gồm cả các thông tin chi tiết về lỗi, trong trường hợp này bạn cần sét đặt nội dung tùy biến cho Content Region.
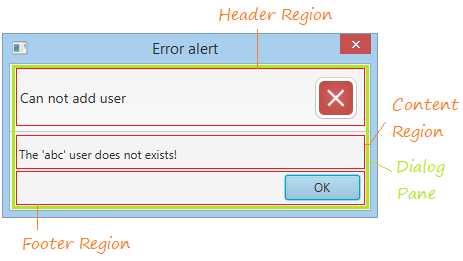
Ví dụ:
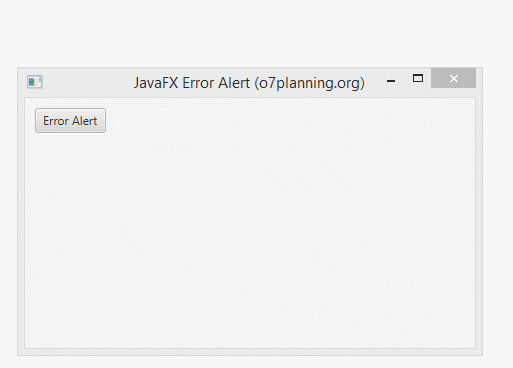
ErrorAlertExample2.java
package org.o7planning.javafx.alert;
import java.io.PrintWriter;
import java.io.StringWriter;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ErrorAlertExample2 extends Application {
private void showError(Exception e) {
Alert alert = new Alert(AlertType.ERROR);
alert.setTitle("Error alert");
alert.setHeaderText(e.getMessage());
VBox dialogPaneContent = new VBox();
Label label = new Label("Stack Trace:");
String stackTrace = this.getStackTrace(e);
TextArea textArea = new TextArea();
textArea.setText(stackTrace);
dialogPaneContent.getChildren().addAll(label, textArea);
// Sét đặt nội dung cho Dialog Pane
alert.getDialogPane().setContent(dialogPaneContent);
alert.showAndWait();
}
private void doSomething() {
try {
// Lỗi xẩy ra tại đây.
int a = 100 / 0;
} catch (Exception e) {
showError(e);
}
}
private String getStackTrace(Exception e) {
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
e.printStackTrace(pw);
String s = sw.toString();
return s;
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
Button button1 = new Button("Error Alert");
button1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
doSomething();
}
});
root.getChildren().addAll(button1);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Error Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
5. Confirmation Alert
Confirmation Alert hiển thị để yêu cầu người dùng xác nhận một hành động sẽ được thực hiện hoặc không. Mặc định Confirmation Alert sẽ có 2 lựa chọn cho người dùng là OK hoặc Cancel.
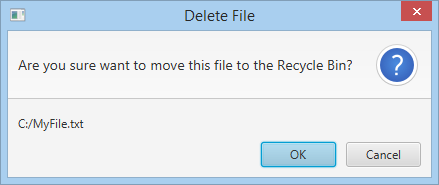
Ví dụ:
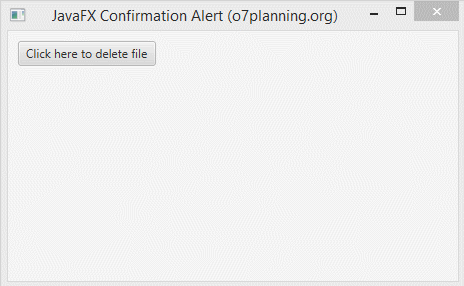
ConfirmationAlertExample.java
package org.o7planning.javafx.alert;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ConfirmationAlertExample extends Application {
private Label label;
private void showConfirmation() {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Delete File");
alert.setHeaderText("Are you sure want to move this file to the Recycle Bin?");
alert.setContentText("C:/MyFile.txt");
// option != null.
Optional<ButtonType> option = alert.showAndWait();
if (option.get() == null) {
this.label.setText("No selection!");
} else if (option.get() == ButtonType.OK) {
this.label.setText("File deleted!");
} else if (option.get() == ButtonType.CANCEL) {
this.label.setText("Cancelled!");
} else {
this.label.setText("-");
}
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Click here to delete file");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showConfirmation();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Confirmation Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
6. Tùy biến các Button
Alert dialog cho phép bạn tùy biến các button hiển thị trên Footer Region, dưới đây là một ví dụ:
Ví dụ:
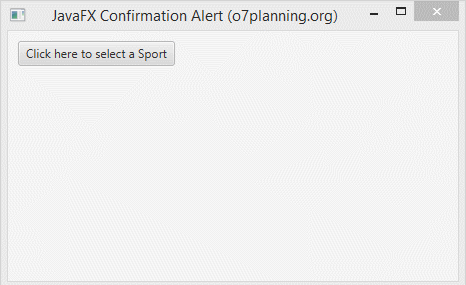
ConfirmationAlertExample2.java
package org.o7planning.javafx.alert;
import java.util.Optional;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ConfirmationAlertExample2 extends Application {
private Label label;
private void showConfirmation() {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Select");
alert.setHeaderText("Choose the sport you like:");
ButtonType football = new ButtonType("Football");
ButtonType badminton = new ButtonType("Badminton");
ButtonType volleyball = new ButtonType("Volleyball");
// Loại bỏ các ButtonType mặc định
alert.getButtonTypes().clear();
alert.getButtonTypes().addAll(football, badminton, volleyball);
// option != null.
Optional<ButtonType> option = alert.showAndWait();
if (option.get() == null) {
this.label.setText("No selection!");
} else if (option.get() == football) {
this.label.setText("You like Football");
} else if (option.get() == badminton) {
this.label.setText("You like Badminton");
} else if (option.get() == volleyball) {
this.label.setText("You like Volleyball");
} else {
this.label.setText("-");
}
}
@Override
public void start(Stage stage) {
VBox root = new VBox();
root.setPadding(new Insets(10));
root.setSpacing(10);
this.label = new Label();
Button button = new Button("Click here to select a Sport");
button.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
showConfirmation();
}
});
root.getChildren().addAll(button, label);
Scene scene = new Scene(root, 450, 250);
stage.setTitle("JavaFX Confirmation Alert (o7planning.org)");
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
Các hướng dẫn lập trình JavaFX
- Cài đặt e(fx)clipse cho Eclipse (Bộ công cụ lập trình JavaFX)
- Cài đặt JavaFX Scene Builder cho Eclipse
- Hướng dẫn lập trình JavaFX cho người mới bắt đầu - Hello JavaFX
- Hướng dẫn và ví dụ JavaFX FlowPane Layout
- Hướng dẫn và ví dụ JavaFX TilePane Layout
- Hướng dẫn và ví dụ JavaFX HBox, VBox Layout
- Hướng dẫn và ví dụ JavaFX BorderPane Layout
- Hướng dẫn và ví dụ JavaFX AnchorPane Layout
- Hướng dẫn và ví dụ JavaFX GridPane Layout
- Hướng dẫn và ví dụ JavaFX StackPane Layout
- Hướng dẫn và ví dụ JavaFX TitledPane
- Hướng dẫn và ví dụ JavaFX ScrollPane
- Hướng dẫn và ví dụ JavaFX Accordion
- Hướng dẫn và ví dụ JavaFX ListView
- Hướng dẫn và ví dụ JavaFX Group
- Hướng dẫn và ví dụ JavaFX ComboBox
- Hướng dẫn và ví dụ JavaFX WebView và WebEngine
- Hướng dẫn và ví dụ JavaFX HTMLEditor
- Hướng dẫn và ví dụ JavaFX TableView
- Hướng dẫn và ví dụ JavaFX TreeView
- Hướng dẫn và ví dụ JavaFX TreeTableView
- Hướng dẫn và ví dụ JavaFX Menu
- Hướng dẫn và ví dụ JavaFX ContextMenu
- Hướng dẫn và ví dụ JavaFX Image và ImageView
- Hướng dẫn và ví dụ JavaFX Label
- Hướng dẫn và ví dụ JavaFX Hyperlink
- Hướng dẫn và ví dụ JavaFX Button
- Hướng dẫn và ví dụ JavaFX ToggleButton
- Hướng dẫn và ví dụ JavaFX RadioButton
- Hướng dẫn và ví dụ JavaFX MenuButton và SplitMenuButton
- Hướng dẫn và ví dụ JavaFX TextField
- Hướng dẫn và ví dụ JavaFX PasswordField
- Hướng dẫn và ví dụ JavaFX TextArea
- Hướng dẫn và ví dụ JavaFX Slider
- Hướng dẫn và ví dụ JavaFX Spinner
- Hướng dẫn và ví dụ JavaFX ProgressBar và ProgressIndicator
- Hướng dẫn và ví dụ JavaFX ChoiceBox
- Hướng dẫn và ví dụ JavaFX Tooltip
- Hướng dẫn và ví dụ JavaFX DatePicker
- Hướng dẫn và ví dụ JavaFX ColorPicker
- Hướng dẫn và ví dụ JavaFX FileChooser và DirectoryChooser
- Mở một cửa sổ (window) mới trong JavaFX
- Hướng dẫn và ví dụ JavaFX Alert Dialog
- Hướng dẫn và ví dụ JavaFX TextInputDialog
- Hướng dẫn và ví dụ JavaFX ChoiceDialog
- Hướng dẫn và ví dụ JavaFX PieChart
- Hướng dẫn và ví dụ JavaFX AreaChart và StackedAreaChart
- Hướng dẫn và ví dụ JavaFX BarChart và StackedBarChart
- Hướng dẫn và ví dụ JavaFX Line
- Hướng dẫn và ví dụ JavaFX Rectangle và Ellipse
- Các hiệu ứng (effects) trong JavaFX
- Hướng dẫn sử dụng các phép biến hình (Transformations) trong JavaFX
Show More