Hàm setTimeout và setInterval trong JavaScript
Trong ECMAScript, setTimeout(func, time) và setInterval(func,time) là hai hàm khá giống nhau, nó hẹn giờ để thực hiện một nhiệm vụ nào đó. Trong bài học này tôi sẽ thảo luận lần lượt về từng hàm này.
1. Hàm setTimeout()
Hàm setTimeout(func, delay) thiết lập một khoảng thời gian 'delay' mili giây, khi khoảng thời gian này trôi qua hàm func sẽ được gọi duy nhất một lần.
Chú ý: 1 giây = 1000 mili giây.
Cú pháp:
setTimeout(func, delay)
- func: Hàm này sẽ được gọi sau khoảng thời gian 'delay' mili giây.
- delay: Khoảng thời gian trễ (Tính bằng mili giây)
Dưới đây là ví dụ đơn giản với hàm setTimeout(). Sau 3 giây kể từ khi người dùng nhấn vào nút "Show greeting" một lời chào sẽ hiển thị.
setTimeout-example.html
<!DOCTYPE html>
<html>
<head>
<title>setTimeout function</title>
<script type="text/javascript">
function greeting() {
alert("Hello Everyone!");
}
function startAction() {
setTimeout(greeting, 3000); // 3 seconds.
}
</script>
</head>
<body>
<h2>setTimeout Function</h2>
<button onclick="startAction()">Show greeting</button>
</body>
</html>
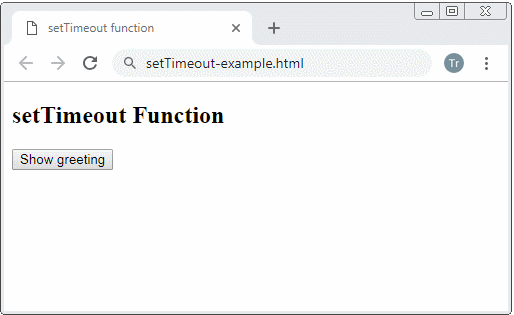
Một ví dụ khác với hàm setTimeout(), bạn có thể chạy ví dụ này trên môi trường NodeJS:
setTimeout-example.js
console.log("3");
console.log("2");
console.log("1");
console.log("Call setTimeout!");
setTimeout( function() {
console.log("Hello Everyone!");
}, 3000); // 3 seconds
console.log("End!");
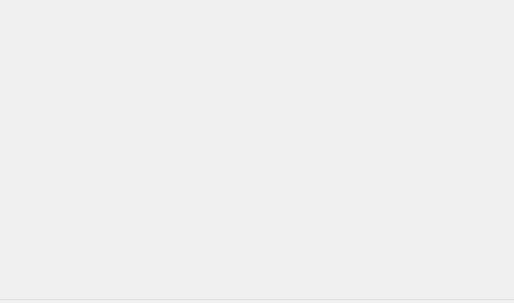
clearTimeout()
Giả sử bạn gọi hàm setTimeout() để hẹn giờ thực hiện một nhiệm vụ nào đó, trong khi nhiệm vụ chưa bắt đầu thực hiện bạn có thể hủy nhiệm vụ đó bằng cách gọi hàm clearTimeout().
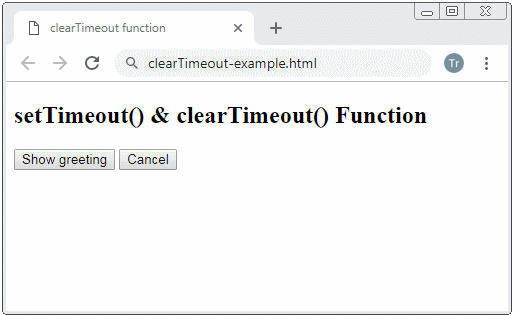
clearTimeout-example.html
<!DOCTYPE html>
<html>
<head>
<title>clearTimeout function</title>
<script type="text/javascript">
var myTask = null;
function greeting() {
alert("Hello Everyone!");
myTask = null;
}
function startAction() {
if(!myTask) {
myTask = setTimeout(greeting, 3000); // 3 seconds.
}
}
function cancelAction() {
if(myTask) {
clearTimeout(myTask);
myTask = null;
}
}
</script>
</head>
<body>
<h2>setTimeout() & clearTimeout() Function</h2>
<button onclick="startAction()">Show greeting</button>
<button onclick="cancelAction()">Cancel</button>
</body>
</html>
2. Hàm setInterval()
Hàm setInterval(func, delay) thiết lập một khoảng thời gian 'delay' mili giây, sau mỗi 'delay' mili giây hàm func lại được gọi.
Cú pháp:
setInterval(func, delay)
- func: Hàm này sẽ được gọi sau mỗi khoảng thời gian 'delay' mili giây.
- delay: Khoảng thời gian trễ (Tính bằng mili giây)
Dưới đây là ví dụ đơn giản với hàm setInterval(). Sau 3 giây kể từ khi người dùng nhấn vào nút "Show greeting" một lời chào sẽ hiển thị, và nó lại hiển thị tiếp sau 3 giây,..
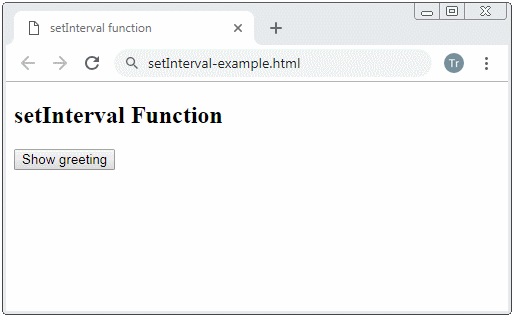
setInterval-example.html
<!DOCTYPE html>
<html>
<head>
<title>setInterval function</title>
<script type="text/javascript">
function greeting() {
alert("Hello Everyone!");
}
function startAction() {
setInterval(greeting, 3000); // 3 seconds.
}
</script>
</head>
<body>
<h2>setInterval Function</h2>
<button onclick="startAction()">Show greeting</button>
</body>
</html>
Một ví dụ khác với hàm setInterval(), bạn có thể chạy ví dụ này trên môi trường NodeJS:
setInterval-example.js
console.log("3");
console.log("2");
console.log("1");
console.log("Call setInterval!");
setInterval( function() {
console.log("Hello Everyone!");
}, 3000); // 3 seconds
console.log("End!");
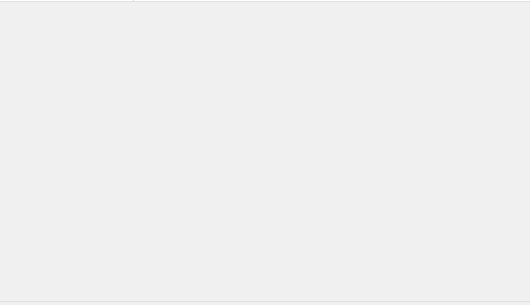
clearInterval()
Giả sử bạn gọi hàm setInterval() để hẹn giờ thực hiện một nhiệm vụ nào đó, bạn có thể hủy nhiệm vụ đó bằng cách gọi hàm clearInterval().
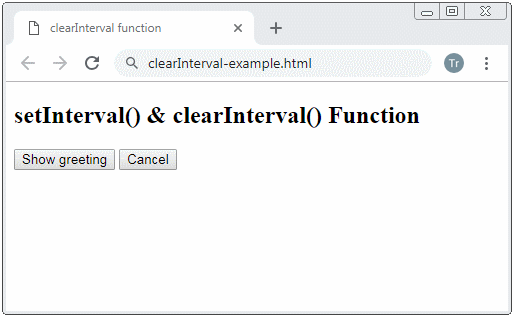
clearInterval-example.html
<!DOCTYPE html>
<html>
<head>
<title>clearInterval function</title>
<script type="text/javascript">
var myTask = null;
function greeting() {
alert("Hello Everyone!");
}
function startAction() {
if(!myTask) {
myTask = setInterval(greeting, 3000); // 3 seconds.
}
}
function cancelAction() {
if(myTask) {
clearInterval(myTask);
myTask = null;
}
}
</script>
</head>
<body>
<h2>setInterval() & clearInterval() Function</h2>
<button onclick="startAction()">Show greeting</button>
<button onclick="cancelAction()">Cancel</button>
</body>
</html>
Các hướng dẫn ECMAScript, Javascript
- Giới thiệu về Javascript và ECMAScript
- Bắt đầu nhanh với Javascript
- Hộp thoại Alert, Confirm, Prompt trong Javascript
- Bắt đầu nhanh với JavaScript
- Biến (Variable) trong JavaScript
- Các toán tử Bitwise
- Mảng (Array) trong JavaScript
- Vòng lặp trong JavaScript
- Hàm trong JavaScript
- Hướng dẫn và ví dụ JavaScript Number
- Hướng dẫn và ví dụ JavaScript Boolean
- Hướng dẫn và ví dụ JavaScript String
- Câu lệnh rẽ nhánh if/else trong JavaScript
- Câu lệnh rẽ nhánh switch trong JavaScript
- Hướng dẫn xử lý lỗi trong JavaScript
- Hướng dẫn và ví dụ JavaScript Date
- Hướng dẫn và ví dụ JavaScript Module
- Lịch sử phát triển của module trong JavaScript
- Hàm setTimeout và setInterval trong JavaScript
- Hướng dẫn và ví dụ Javascript Form Validation
- Hướng dẫn và ví dụ JavaScript Web Cookie
- Từ khóa void trong JavaScript
- Lớp và đối tượng trong JavaScript
- Kỹ thuật mô phỏng lớp và kế thừa trong JavaScript
- Thừa kế và đa hình trong JavaScript
- Tìm hiểu về Duck Typing trong JavaScript
- Hướng dẫn và ví dụ JavaScript Symbol
- Hướng dẫn JavaScript Set Collection
- Hướng dẫn JavaScript Map Collection
- Tìm hiểu về JavaScript Iterable và Iterator
- Hướng dẫn sử dụng biểu thức chính quy trong JavaScript
- Hướng dẫn và ví dụ JavaScript Promise, Async Await
- Hướng dẫn và ví dụ Javascript Window
- Hướng dẫn và ví dụ Javascript Console
- Hướng dẫn và ví dụ Javascript Screen
- Hướng dẫn và ví dụ Javascript Navigator
- Hướng dẫn và ví dụ Javascript Geolocation API
- Hướng dẫn và ví dụ Javascript Location
- Hướng dẫn và ví dụ Javascript History API
- Hướng dẫn và ví dụ Javascript Statusbar
- Hướng dẫn và ví dụ Javascript Locationbar
- Hướng dẫn và ví dụ Javascript Scrollbars
- Hướng dẫn và ví dụ Javascript Menubar
- Hướng dẫn xử lý JSON trong JavaScript
- Xử lý sự kiện (Event) trong Javascript
- Hướng dẫn và ví dụ Javascript MouseEvent
- Hướng dẫn và ví dụ Javascript WheelEvent
- Hướng dẫn và ví dụ Javascript KeyboardEvent
- Hướng dẫn và ví dụ Javascript FocusEvent
- Hướng dẫn và ví dụ Javascript InputEvent
- Hướng dẫn và ví dụ Javascript ChangeEvent
- Hướng dẫn và ví dụ Javascript DragEvent
- Hướng dẫn và ví dụ Javascript HashChangeEvent
- Hướng dẫn và ví dụ Javascript URL Encoding
- Hướng dẫn và ví dụ Javascript FileReader
- Hướng dẫn và ví dụ Javascript XMLHttpRequest
- Hướng dẫn và ví dụ Javascript Fetch API
- Phân tích XML trong Javascript với DOMParser
- Giới thiệu về Javascript HTML5 Canvas API
- Làm nổi bật Code với thư viện Javascript SyntaxHighlighter
- Polyfill là gì trong khoa học lập trình?
Show More