Hướng dẫn và ví dụ Javascript InputEvent
1. InputEvent
InputEvent là một interface đại diện cho các sự kiện xẩy ra khi người dùng nhập dữ liệu trên các phần tử có thể sửa đổi (editable element) chẳng hạn như <input>, <textarea> hoặc các phần tử với thuộc tính (attribute) contenteditable="true".
InputEvent là một interface con của UIEvent.
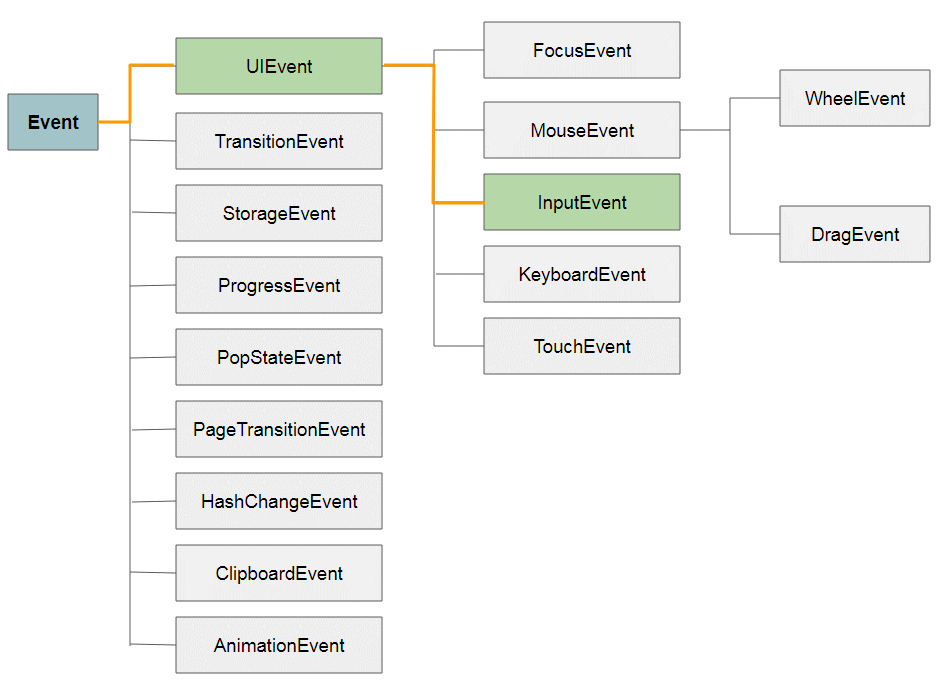
Các sự kiện:
Event | Mô tả |
input | Sự kiện xẩy ra khi một phần tử nhận được đầu vào từ người dùng. |
Các trình duyệt hỗ trợ InputEvent:

Ví dụ, các phần tử hỗ trợ sự kiện input:
inputevents-elements-example.html
<!DOCTYPE html>
<html>
<head>
<title>InputEvent Example</title>
<meta charset="UTF-8">
<style>
*[contenteditable='true'] {
height:30px;padding:5px;border:1px solid #ccd;
}
</style>
<script>
// Note: event.data is not supported in Firefox, IE
function inputHandler(evt) {
showLog(evt.data);
}
function showLog(text) {
var logDiv = document.getElementById("log-div");
logDiv.innerHTML = logDiv.innerHTML + " .. " + text;
}
</script>
</head>
<body>
<h3>InputEvent example</h3>
<p style="color:red">Note: event.data is not supported in Firefox, IE</p>
<h3>Input element</h3>
<input type="text" oninput ="inputHandler(event)"/>
<h3>Textarea element</h3>
<textarea oninput ="inputHandler(event)" rows="5" cols="30"></textarea>
<h3>Elements with contenteditable='true'</h3>
<div oninput ="inputHandler(event)" contenteditable="true"></div>
<p id="log-div"></p>
</body>
</html>
Events: input vs change
Bạn cũng có thể cân nhắc sử dụng sự kiện change vì nó khá giống với sự kiện input:
- Sự kiện change hỗ trợ phần tử <select>, nó xẩy ra khi người dùng thay đổi lựa chọn (option) trên <select>.
- Đối với các phần tử <input> (text, number, email, password, tel, time, week, month, date, datetime-local, search, url), <textarea>: Sự kiện input xẩy ra ngay sau khi giá trị phần tử thay đổi. Trong khi sự kiện change xẩy ra khi giá trị phần tử thay đổi và phần tử mất focus.
2. Properties, Methods
InputEvent là interface con của UIEvent nên nó thừa hưởng tất cả các phương thức, thuộc tính (property) từ UIEvent. Ngoài ra nó có thêm các phương thức và thuộc tính (property) riêng của nó.
Properties:
Property | Mô tả |
data | Trả về các ký tự được chèn vào. Đây có thể là một chuỗi trống nếu thay đổi không chèn vào văn bản (chẳng hạn như khi xóa các ký tự). |
dataTransfer | Trả về một đối tượng DataTransfer chứa thông tin về dữ liệu richtext hoặc plaintext được thêm vào hoặc xóa khỏi nội dung của phần tử. |
inputType | Trả về loại thay đổi, chẳng hạn như 'inserting', 'deleting', 'formatting', ... |
isComposing | Trả về true/false cho biết nếu sự kiện này được kích hoạt sau sự kiện compositionstart và trước sự kiện compositionend. |
Các giá trị có thể của inputType:
- insertText
- insertReplacementText
- insertLineBreak
- insertParagraph
- insertOrderedList
- insertUnorderedList
- insertHorizontalRule
- insertFromYank
- insertFromDrop
- insertFromPaste
- insertTranspose
- insertCompositionText
- insertFromComposition
- insertLink
- deleteByComposition
- deleteCompositionText
- deleteWordBackward
- deleteWordForward
- deleteSoftLineBackward
- deleteSoftLineForward
- deleteEntireSoftLine
- deleteHardLineBackward
- deleteHardLineForward
- deleteByDrag
- deleteByCut
- deleteByContent
- deleteContentBackward
- deleteContentForward
- historyUndo
- historyRedo
- formatBold
- formatItalic
- formatUnderline
- formatStrikethrough
- formatSuperscript
- formatSubscript
- formatJustifyFull
- formatJustifyCenter
- formatJustifyRight
- formatJustifyLeft
- formatIndent
- formatOutdent
- formatRemove
- formatSetBlockTextDirection
- formatSetInlineTextDirection
- formatBackColor
- formatFontColor
- formatFontName
Methods:
Method | Mô tả |
getTargetRanges() | Trả về một mảng chứa các phạm vi mục tiêu sẽ bị ảnh hưởng bởi sự kiện này. |
Chú ý: Mặc dù InputEvent được hỗ trợ bởi tất cả các trình duyệt nhưng một vài thuộc tính (property) và phương thức của InputEvent không được hỗ trợ trong một vài trình duyệt, vì vậy bạn nên cân nhắc khi sử dụng chúng.
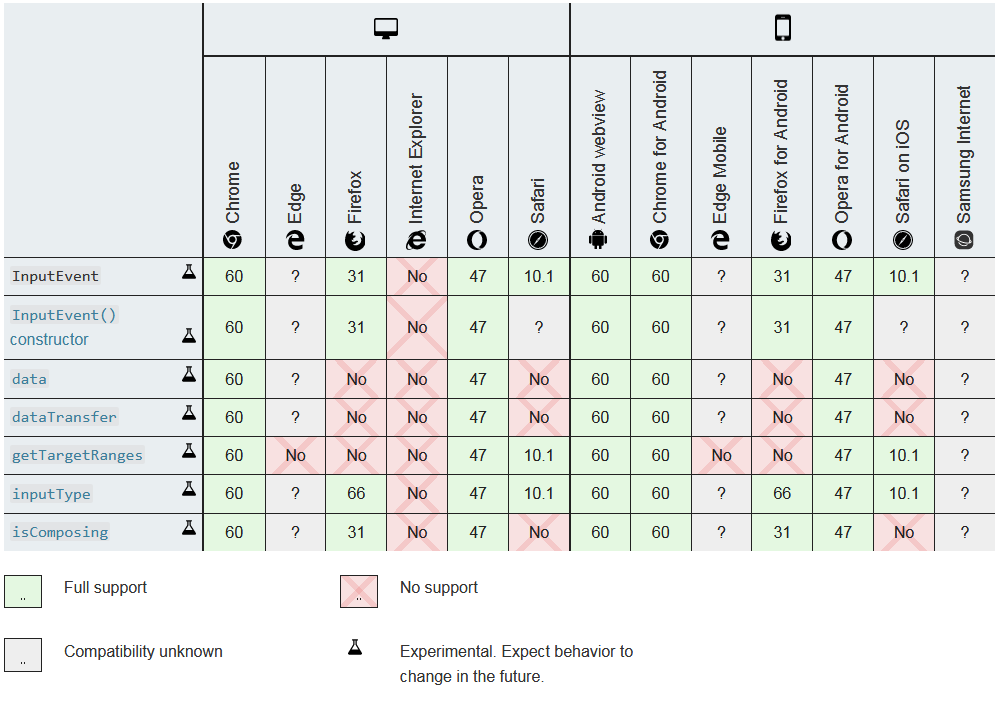
Ví dụ:
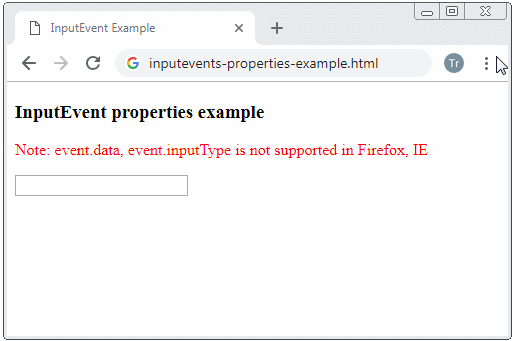
inputevents-properties-example.html
<!DOCTYPE html>
<html>
<head>
<title>InputEvent Example</title>
<meta charset="UTF-8">
<script>
// Note: event.data, event.inputType is not supported in Firefox, IE
function inputHandler(evt) {
showLog(evt.data +" - " + evt.inputType);
}
function showLog(text) {
var logDiv = document.getElementById("log-div");
logDiv.innerHTML = text;
}
</script>
</head>
<body>
<h3>InputEvent properties example</h3>
<p style="color:red">Note: event.data, event.inputType is not supported in Firefox, IE</p>
<input type="text" oninput ="inputHandler(event)"/>
<p id="log-div"></p>
</body>
</html>
Các hướng dẫn ECMAScript, Javascript
- Giới thiệu về Javascript và ECMAScript
- Bắt đầu nhanh với Javascript
- Hộp thoại Alert, Confirm, Prompt trong Javascript
- Bắt đầu nhanh với JavaScript
- Biến (Variable) trong JavaScript
- Các toán tử Bitwise
- Mảng (Array) trong JavaScript
- Vòng lặp trong JavaScript
- Hàm trong JavaScript
- Hướng dẫn và ví dụ JavaScript Number
- Hướng dẫn và ví dụ JavaScript Boolean
- Hướng dẫn và ví dụ JavaScript String
- Câu lệnh rẽ nhánh if/else trong JavaScript
- Câu lệnh rẽ nhánh switch trong JavaScript
- Hướng dẫn xử lý lỗi trong JavaScript
- Hướng dẫn và ví dụ JavaScript Date
- Hướng dẫn và ví dụ JavaScript Module
- Lịch sử phát triển của module trong JavaScript
- Hàm setTimeout và setInterval trong JavaScript
- Hướng dẫn và ví dụ Javascript Form Validation
- Hướng dẫn và ví dụ JavaScript Web Cookie
- Từ khóa void trong JavaScript
- Lớp và đối tượng trong JavaScript
- Kỹ thuật mô phỏng lớp và kế thừa trong JavaScript
- Thừa kế và đa hình trong JavaScript
- Tìm hiểu về Duck Typing trong JavaScript
- Hướng dẫn và ví dụ JavaScript Symbol
- Hướng dẫn JavaScript Set Collection
- Hướng dẫn JavaScript Map Collection
- Tìm hiểu về JavaScript Iterable và Iterator
- Hướng dẫn sử dụng biểu thức chính quy trong JavaScript
- Hướng dẫn và ví dụ JavaScript Promise, Async Await
- Hướng dẫn và ví dụ Javascript Window
- Hướng dẫn và ví dụ Javascript Console
- Hướng dẫn và ví dụ Javascript Screen
- Hướng dẫn và ví dụ Javascript Navigator
- Hướng dẫn và ví dụ Javascript Geolocation API
- Hướng dẫn và ví dụ Javascript Location
- Hướng dẫn và ví dụ Javascript History API
- Hướng dẫn và ví dụ Javascript Statusbar
- Hướng dẫn và ví dụ Javascript Locationbar
- Hướng dẫn và ví dụ Javascript Scrollbars
- Hướng dẫn và ví dụ Javascript Menubar
- Hướng dẫn xử lý JSON trong JavaScript
- Xử lý sự kiện (Event) trong Javascript
- Hướng dẫn và ví dụ Javascript MouseEvent
- Hướng dẫn và ví dụ Javascript WheelEvent
- Hướng dẫn và ví dụ Javascript KeyboardEvent
- Hướng dẫn và ví dụ Javascript FocusEvent
- Hướng dẫn và ví dụ Javascript InputEvent
- Hướng dẫn và ví dụ Javascript ChangeEvent
- Hướng dẫn và ví dụ Javascript DragEvent
- Hướng dẫn và ví dụ Javascript HashChangeEvent
- Hướng dẫn và ví dụ Javascript URL Encoding
- Hướng dẫn và ví dụ Javascript FileReader
- Hướng dẫn và ví dụ Javascript XMLHttpRequest
- Hướng dẫn và ví dụ Javascript Fetch API
- Phân tích XML trong Javascript với DOMParser
- Giới thiệu về Javascript HTML5 Canvas API
- Làm nổi bật Code với thư viện Javascript SyntaxHighlighter
- Polyfill là gì trong khoa học lập trình?
Show More