Hướng dẫn và ví dụ Javascript Scrollbars
1. window.scrollbars
Thuộc tính (property) window.scrollbars trả về một đối tượng Scrollbars đại diện cho các thanh cuộn (scroll bars) bao quanh Viewport của trình duyệt. Tuy nhiên hầu như bạn không thể tương tác với Scrollbars thông qua Javascript vì nó có rất ít API cho bạn.
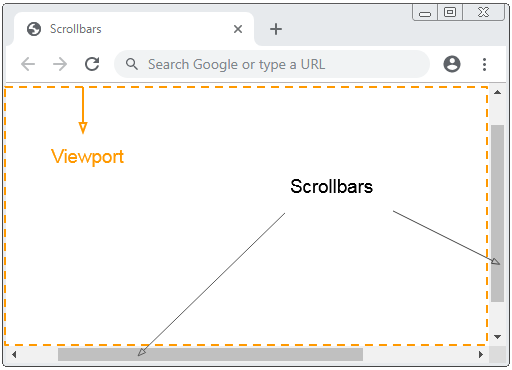
window.scrollbars
// Or Simple:
scrollbars
scrollbars.visible
Đối tượng Scrollbars có duy nhất một thuộc tính (property) là visible. Tuy nhiên thuộc tính này không đáng tin cậy, scrollbars.visible trả về giá trị true điều đó không có nghĩa là bạn đang nhìn thấy các thanh cuộn.
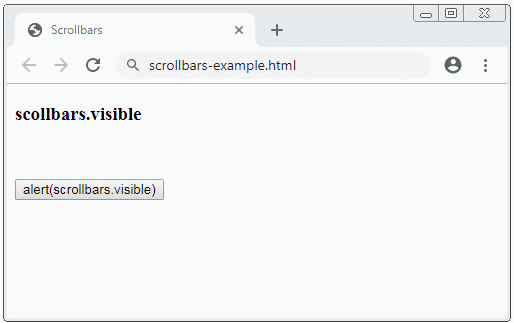
scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
</head>
<body>
<h3>scollbars.visible</h3>
<br/><br/>
<button onclick="alert(scrollbars.visible)">
alert(scrollbars.visible)
</button>
</body>
</html>
Example:
Ví dụ, sử dụng phương thức window.open(..) mở ra một cửa sổ mới không có thanh cuộn:
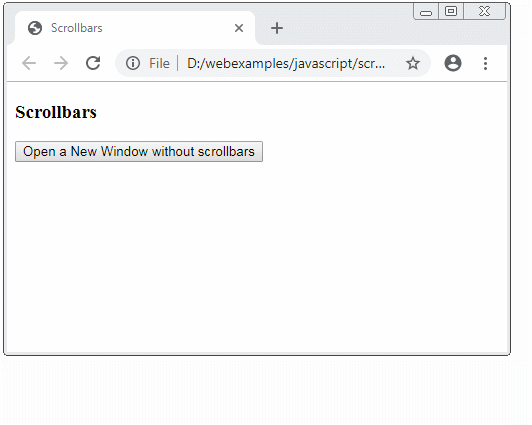
open-new-window-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<script>
function openNewWindow() {
var winFeature = 'scrollbars=no,resizable=yes';
// Open a New Windows.
window.open('some-page.html','MyWinName',winFeature);
}
</script>
</head>
<body>
<h3>Scrollbars</h3>
<button onclick="openNewWindow()">
Open a New Window without scrollbars
</button>
</body>
</html>
some-page.html
<!DOCTYPE html>
<html>
<head>
<title>Some Page</title>
<meta charset="UTF-8">
<style>
/** CHROME: Make the scroll bar not appear */
body {
overflow: hidden;
}
</style>
</head>
<body>
<h3>Some Page</h3>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
<p>1 2 3 4 5 6</p>
<p>1 2 3 4 5 6 7</p>
</body>
</html>
Example:
Ví dụ, Làm sao để các thanh cuộn không bao giờ hiển thị?
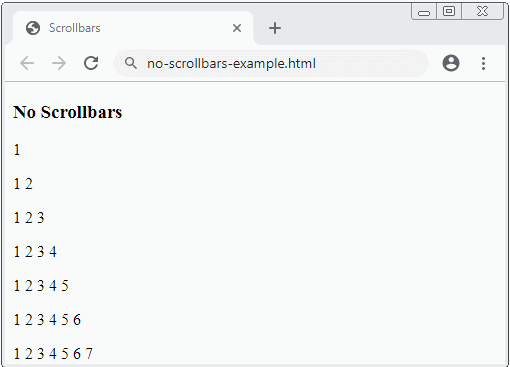
no-scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<style>
body {
overflow: hidden;
}
</style>
</head>
<body>
<h3>No Scrollbars</h3>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
<p>1 2 3 4 5 6</p>
<p>1 2 3 4 5 6 7</p>
</body>
</html>
2. Phát hiện thanh cuộn
Đôi khi bạn muốn phát hiện (detect) thanh cuộn của 1 phần tử nào đó có đang hiển thị hay không? Hoặc muốn phát hiện thanh cuộn của Viewport có đang hiển thị hay không? Dưới đây là một thư viện nhỏ, nó có ích cho bạn trong trường hợp này.
scrollbars-utils.js
// Private Function
// @axis: 'X', 'Y', 'x', 'y'
function __isScrollbarShowing__(domNode, axis, computedStyles) {
axis = axis.toUpperCase();
var type;
if(axis === 'Y') {
type = 'Height';
} else {
type = 'Width';
}
var scrollType = 'scroll' + type;
var clientType = 'client' + type;
var overflowAxis = 'overflow' + axis;
var hasScroll = domNode[scrollType] > domNode[clientType];
// Check the overflow and overflowY properties for "auto" and "visible" values
var cStyle = computedStyles || window.getComputedStyle(domNode)
return hasScroll && (cStyle[overflowAxis] == "visible"
|| cStyle[overflowAxis] == "auto"
)
|| cStyle[overflowAxis] == "scroll";
}
// @domNode: Optional.
function isScrollbarXShowing(domNode) {
if(!domNode) {
domNode = document.documentElement;
}
return __isScrollbarShowing__(domNode, 'x');
}
// @domNode: Optional.
function isScrollbarYShowing(domNode) {
if(!domNode) {
domNode = document.documentElement;
}
return __isScrollbarShowing__(domNode, 'y');
}
// Scrollbar X or Scrollbar Y is showing?
// @domNode: Optional.
function isScrollbarShowing(domNode) {
return isScrollbarXShowing(domNode) || isScrollbarYShowing(domNode);
}
Ví dụ, sử dụng thư viện trên để phát hiện thanh cuộn của Viewport.
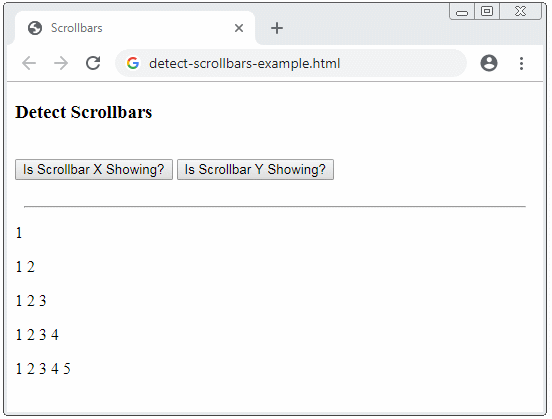
detect-scollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<script src="scrollbars-utils.js"></script>
</head>
<body>
<h3>Detect Scrollbars</h3>
<br/>
<button onclick="alert(isScrollbarXShowing())">
Is Scrollbar X Showing?
</button>
<button onclick="alert(isScrollbarYShowing())">
Is Scrollbar Y Showing?
</button>
<br/><br/>
<hr style="width: 500px;"/>
<p>1</p>
<p>1 2</p>
<p>1 2 3</p>
<p>1 2 3 4</p>
<p>1 2 3 4 5</p>
</body>
</html>
Ví dụ, phát hiện (detect) thanh cuộn của phần tử <div>:
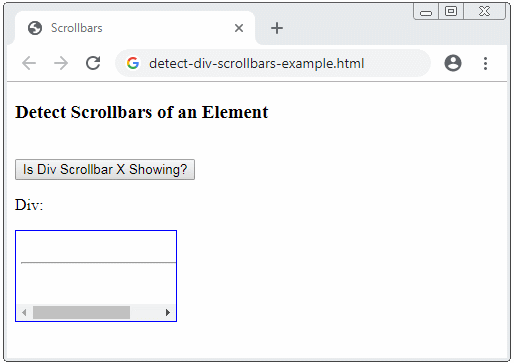
detect-div-scrollbars-example.html
<!DOCTYPE html>
<html>
<head>
<title>Scrollbars</title>
<meta charset="UTF-8">
<style>
#my-div {
border: 1px solid blue;
overflow-x: auto;
padding: 5px;
width: 150px;
height: 80px;
}
</style>
<script src="scrollbars-utils.js"></script>
<script>
function detectScrollbarXOfDiv() {
var domNode = document.getElementById("my-div");
var scX = isScrollbarXShowing(domNode);
var scY = isScrollbarYShowing(domNode);
alert("Is Div Scrollbar X Showing? " + scX);
}
</script>
</head>
<body>
<h3>Detect Scrollbars of an Element</h3>
<br/>
<button onclick="detectScrollbarXOfDiv()">
Is Div Scrollbar X Showing?
</button>
<p>Div:</p>
<div id="my-div">
<br>
<hr style="width:200px"/>
<br>
</div>
</body>
</html>
Các hướng dẫn ECMAScript, Javascript
- Giới thiệu về Javascript và ECMAScript
- Bắt đầu nhanh với Javascript
- Hộp thoại Alert, Confirm, Prompt trong Javascript
- Bắt đầu nhanh với JavaScript
- Biến (Variable) trong JavaScript
- Các toán tử Bitwise
- Mảng (Array) trong JavaScript
- Vòng lặp trong JavaScript
- Hàm trong JavaScript
- Hướng dẫn và ví dụ JavaScript Number
- Hướng dẫn và ví dụ JavaScript Boolean
- Hướng dẫn và ví dụ JavaScript String
- Câu lệnh rẽ nhánh if/else trong JavaScript
- Câu lệnh rẽ nhánh switch trong JavaScript
- Hướng dẫn xử lý lỗi trong JavaScript
- Hướng dẫn và ví dụ JavaScript Date
- Hướng dẫn và ví dụ JavaScript Module
- Lịch sử phát triển của module trong JavaScript
- Hàm setTimeout và setInterval trong JavaScript
- Hướng dẫn và ví dụ Javascript Form Validation
- Hướng dẫn và ví dụ JavaScript Web Cookie
- Từ khóa void trong JavaScript
- Lớp và đối tượng trong JavaScript
- Kỹ thuật mô phỏng lớp và kế thừa trong JavaScript
- Thừa kế và đa hình trong JavaScript
- Tìm hiểu về Duck Typing trong JavaScript
- Hướng dẫn và ví dụ JavaScript Symbol
- Hướng dẫn JavaScript Set Collection
- Hướng dẫn JavaScript Map Collection
- Tìm hiểu về JavaScript Iterable và Iterator
- Hướng dẫn sử dụng biểu thức chính quy trong JavaScript
- Hướng dẫn và ví dụ JavaScript Promise, Async Await
- Hướng dẫn và ví dụ Javascript Window
- Hướng dẫn và ví dụ Javascript Console
- Hướng dẫn và ví dụ Javascript Screen
- Hướng dẫn và ví dụ Javascript Navigator
- Hướng dẫn và ví dụ Javascript Geolocation API
- Hướng dẫn và ví dụ Javascript Location
- Hướng dẫn và ví dụ Javascript History API
- Hướng dẫn và ví dụ Javascript Statusbar
- Hướng dẫn và ví dụ Javascript Locationbar
- Hướng dẫn và ví dụ Javascript Scrollbars
- Hướng dẫn và ví dụ Javascript Menubar
- Hướng dẫn xử lý JSON trong JavaScript
- Xử lý sự kiện (Event) trong Javascript
- Hướng dẫn và ví dụ Javascript MouseEvent
- Hướng dẫn và ví dụ Javascript WheelEvent
- Hướng dẫn và ví dụ Javascript KeyboardEvent
- Hướng dẫn và ví dụ Javascript FocusEvent
- Hướng dẫn và ví dụ Javascript InputEvent
- Hướng dẫn và ví dụ Javascript ChangeEvent
- Hướng dẫn và ví dụ Javascript DragEvent
- Hướng dẫn và ví dụ Javascript HashChangeEvent
- Hướng dẫn và ví dụ Javascript URL Encoding
- Hướng dẫn và ví dụ Javascript FileReader
- Hướng dẫn và ví dụ Javascript XMLHttpRequest
- Hướng dẫn và ví dụ Javascript Fetch API
- Phân tích XML trong Javascript với DOMParser
- Giới thiệu về Javascript HTML5 Canvas API
- Làm nổi bật Code với thư viện Javascript SyntaxHighlighter
- Polyfill là gì trong khoa học lập trình?
Show More