Hướng dẫn và ví dụ Javascript FocusEvent
1. FocusEvent
FocusEvent là một interface đại diện cho các sự kiện liên quan tới focus, cụ thể là 4 sự kiện focus, focusin, blur, focusout.
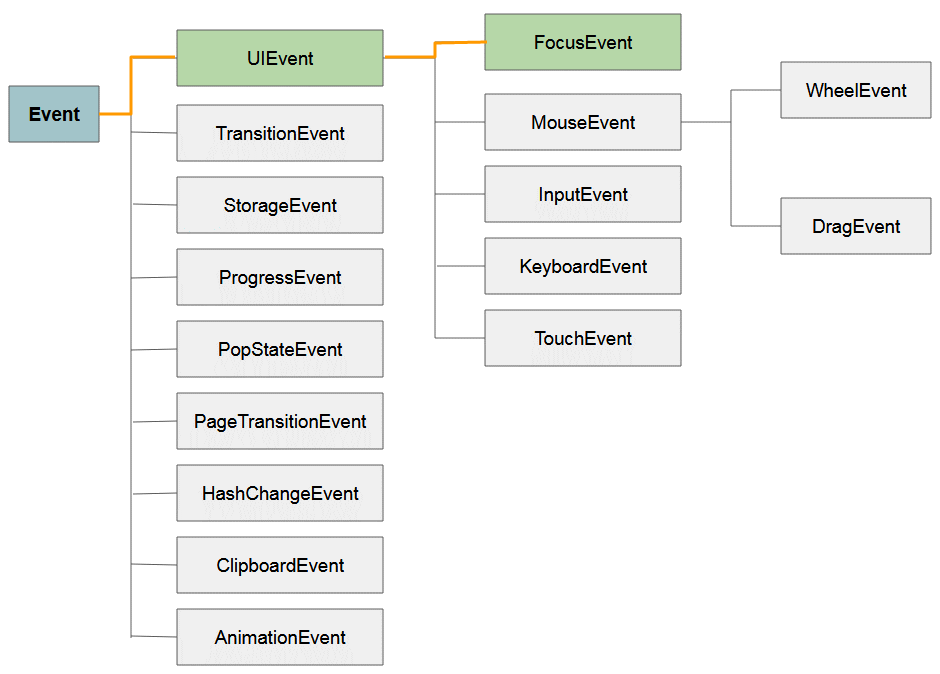
- Hướng dẫn và ví dụ Javascript UiEvent
Các thuộc tính (property):
Property | Mô tả |
relatedTarget | Trả về phần tử liên quan tới phần tử đã kích hoạt sự kiện. |
Nếu bạn nhẩy đến một phần tử sau đó nhẩy ra ngoài (focus vào 1 phần tử khác), sẽ có 4 sự kiện xẩy ra theo thứ tự lần lượt là:
- focus
- focusin
- blue
- focusout
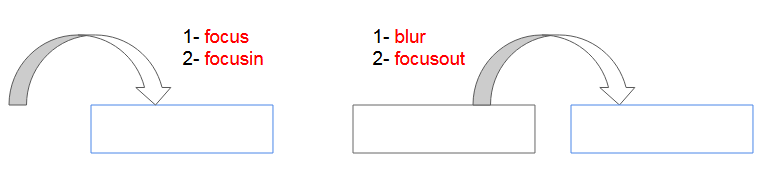
Sự kiện focus xẩy ra trước focusin, thực tế là chúng xẩy ra rất rất gần nhau, có thể coi là xẩy ra đồng thời. Tương tự như vậy, sự kiện blur xẩy ra trước focusout, thực tế chúng xẩy ra rất rất gần nhau, có thể coi là xẩy ra đồng thời.
focus
Sự kiện focus xẩy ra khi bạn đang ở một nơi khác và nhẩy vào một phần tử, chẳng hạn phần tử <input> để chuẩn bị nhập nội dung cho phần tử này.
blur
Sự kiện blur xẩy ra khi bạn nhẩy ra khỏi một phần tử (focus vào một phần tử khác).
focusevent-example.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<script>
function focusHandler(evt) {
showLog("focus");
}
function blurHandler(evt) {
showLog("blur");
}
function showLog(msg) {
var oldHtml= document.getElementById("log-div").innerHTML;
document.getElementById("log-div").innerHTML=oldHtml + " .. "+ msg;
}
</script>
</head>
<body>
<h3>Test events: focus, blur</h3>
<input type="text"
onfocus="focusHandler(event)"
onblur="blurHandler(event)" />
<br><br>
<div id="log-div" style="padding:10px;border:1px solid #ccd;"></div>
</body>
</html>
2. focusin, focusout
Các sự kiện focus, blur được thiết kế dành cho các phần tử "có thể focus" (focusable), chẳng hạn như <input>, <textarea>,.. Trong khi đó sự kiện focusin, focusout được thiết kế dành cho các phần tử có thể chứa các phần tử khác chẳng hạn như <div>, <span>, <form>,..
focus vs focusin
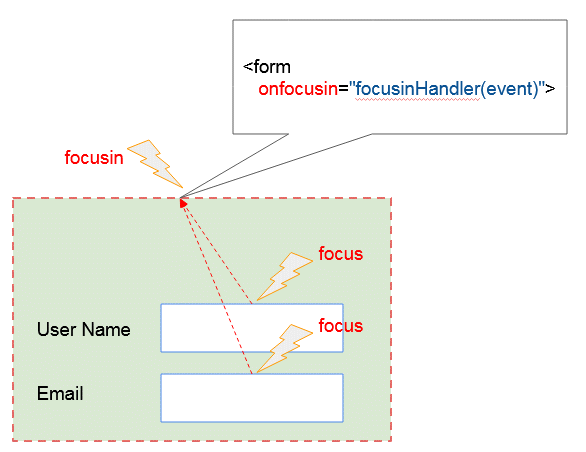
Giả sử bạn có một <form> với 2 phần tử con <input>. Khi người dùng nhẩy vào một trong các phần tử <input> khi đó sự kiện focus sẽ xẩy ra. Sự kiện focus tại phần tử con sẽ tạo ra sự kiện focusin tại các phần tử cha. Như vậy sự kiện focusin sẽ xẩy ra tại phần tử <form>. Chú ý: Mặc dù sự kiện focusin xẩy ra trên phần tử cha, nhưng event.target trả về phần tử con (Phần tử phát ra sự kiện focus).
blur vs focusout
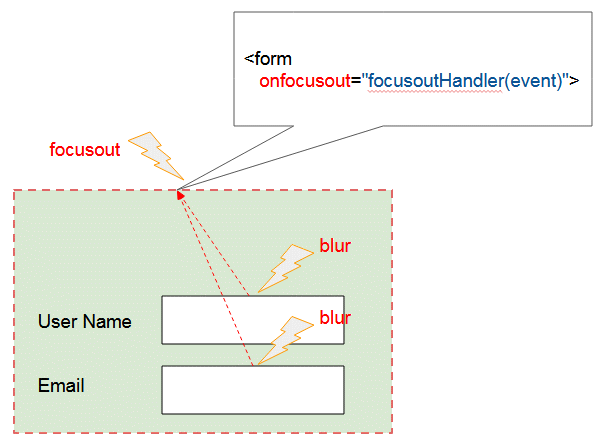
Giả sử bạn có một <form> với 2 phần tử con <input>. Khi người dùng nhẩy ra khỏi phần tử <input> khi đó sự kiện blur sẽ xẩy ra. Sự kiện blur tại phần tử con sẽ tạo ra sự kiện focusout tại các phần tử cha. Như vậy sự kiện focusout sẽ xẩy ra tại phần tử <form>. Chú ý: Mặc dù sự kiện focusout xẩy ra trên phần tử cha, nhưng event.target trả về phần tử con (Phần tử phát ra sự kiện blur).
Example: focusin & focusout
focusin-focusout-example.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<meta charset="utf-8" />
<script src="focusin-focusout-example.js"></script>
<style>
form {
border: 1px solid #ccc;
padding: 10px;
display: inline-block;
}
</style>
</head>
<body>
<h3>Events: focusin & focusout</h3>
<p>Focus to 'input' and Blur to it</p>
<form onfocusin="focusinHandler(event)" onfocusout="focusoutHandler(event)">
User Name
<input type="text" name="username" />
<br>
<br> Email
<input type="text" name="email" />
</form>
</body>
</html>
focusin-focusout-example.js
function focusinHandler(evt) {
// <input>
evt.target.style.border="1px solid blue";
// <form>
evt.target.parentElement.style.border="1px solid red";
}
function focusoutHandler(evt) {
// <input>
evt.target.style.border="1px solid black";
// <form>
evt.target.parentElement.style.border="1px solid black";
}
Bạn gặp vấn đề với onfocusout (???)
Với các phần tử <input>, <textarea>,.. bạn chỉ cần xử lý sự kiện focus, blur. Thuộc tính (attribute) onfocusout không hoạt động đối với phần tử này trên một vài trình duyệt (Tôi đã kiểm tra vấn đề này trên trình duyệt Firefox, Chrome).
<input type="text"
onfocus="focusHandler(event)"
onblur="blurHandler(event)"
onfocusin="focusinHandler(event)"onforcusout="focusoutHandler(event)"
/>
attr-onfocusout-not-working.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<script>
function focusHandler(evt) {
showLog("focus");
}
function focusinHandler(evt) {
showLog("focusin");
}
function focusoutHandler(evt) {
showLog("focusout");
}
function blurHandler(evt) {
showLog("blur");
}
function showLog(msg) {
var oldHtml= document.getElementById("log-div").innerHTML;
document.getElementById("log-div").innerHTML=oldHtml + " .. "+ msg;
}
</script>
</head>
<body>
<h3>Why does the 'onfocusout' attribute not work with 'input'?</h3>
<input type="text"
onfocus="focusHandler(event)"
onblur="blurHandler(event)"
onfocusin="focusinHandler(event)"
onforcusout="focusoutHandler(event)" />
<br><br>
<div id="log-div" style="padding:10px;border:1px solid #ccd;"></div>
</body>
</html>
Mặc dù thuộc tính (attribute) onfocusout không hoạt động với phần tử <input>, <textarea>,.. trên một vài trình duyệt. Nhưng nếu muốn bạn vẫn có thể đăng ký sự kiện focusout cho chúng theo cách dưới đây:
// Javascript Code:
var myInput = document.getElementById("my-input");
myInput.addEventListener("focus", focusHandler);
myInput.addEventListener("focusin", focusinHandler);
myInput.addEventListener("focusout", focusoutHandler);
myInput.addEventListener("blur", blurHandler);
Xem ví dụ đầy đủ:
focusevents-addEventListener-example.html
<!DOCTYPE html>
<html>
<head>
<title>FocusEvent</title>
<meta charset="utf-8"/>
</head>
<body>
<h3>Test events: focus, focusin, focusout, blur</h3>
<input id="my-input" type="text" />
<br><br>
<div id="log-div" style="padding:10px;border:1px solid #ccd;"></div>
<script src="focusevents-addEventListener-example.js"></script>
</body>
</html>
focusevents-addEventListener-example.js
function focusHandler(evt) {
showLog("focus");
}
function focusinHandler(evt) {
showLog("focusin");
}
function focusoutHandler(evt) {
showLog("focusout");
}
function blurHandler(evt) {
showLog("blur");
}
function showLog(msg) {
var oldHtml= document.getElementById("log-div").innerHTML;
document.getElementById("log-div").innerHTML=oldHtml + " .. "+ msg;
}
// Javascript Code:
var myInput = document.getElementById("my-input");
myInput.addEventListener("focus", focusHandler);
myInput.addEventListener("focusin", focusinHandler);
myInput.addEventListener("focusout", focusoutHandler);
myInput.addEventListener("blur", blurHandler);
Các hướng dẫn ECMAScript, Javascript
- Giới thiệu về Javascript và ECMAScript
- Bắt đầu nhanh với Javascript
- Hộp thoại Alert, Confirm, Prompt trong Javascript
- Bắt đầu nhanh với JavaScript
- Biến (Variable) trong JavaScript
- Các toán tử Bitwise
- Mảng (Array) trong JavaScript
- Vòng lặp trong JavaScript
- Hàm trong JavaScript
- Hướng dẫn và ví dụ JavaScript Number
- Hướng dẫn và ví dụ JavaScript Boolean
- Hướng dẫn và ví dụ JavaScript String
- Câu lệnh rẽ nhánh if/else trong JavaScript
- Câu lệnh rẽ nhánh switch trong JavaScript
- Hướng dẫn xử lý lỗi trong JavaScript
- Hướng dẫn và ví dụ JavaScript Date
- Hướng dẫn và ví dụ JavaScript Module
- Lịch sử phát triển của module trong JavaScript
- Hàm setTimeout và setInterval trong JavaScript
- Hướng dẫn và ví dụ Javascript Form Validation
- Hướng dẫn và ví dụ JavaScript Web Cookie
- Từ khóa void trong JavaScript
- Lớp và đối tượng trong JavaScript
- Kỹ thuật mô phỏng lớp và kế thừa trong JavaScript
- Thừa kế và đa hình trong JavaScript
- Tìm hiểu về Duck Typing trong JavaScript
- Hướng dẫn và ví dụ JavaScript Symbol
- Hướng dẫn JavaScript Set Collection
- Hướng dẫn JavaScript Map Collection
- Tìm hiểu về JavaScript Iterable và Iterator
- Hướng dẫn sử dụng biểu thức chính quy trong JavaScript
- Hướng dẫn và ví dụ JavaScript Promise, Async Await
- Hướng dẫn và ví dụ Javascript Window
- Hướng dẫn và ví dụ Javascript Console
- Hướng dẫn và ví dụ Javascript Screen
- Hướng dẫn và ví dụ Javascript Navigator
- Hướng dẫn và ví dụ Javascript Geolocation API
- Hướng dẫn và ví dụ Javascript Location
- Hướng dẫn và ví dụ Javascript History API
- Hướng dẫn và ví dụ Javascript Statusbar
- Hướng dẫn và ví dụ Javascript Locationbar
- Hướng dẫn và ví dụ Javascript Scrollbars
- Hướng dẫn và ví dụ Javascript Menubar
- Hướng dẫn xử lý JSON trong JavaScript
- Xử lý sự kiện (Event) trong Javascript
- Hướng dẫn và ví dụ Javascript MouseEvent
- Hướng dẫn và ví dụ Javascript WheelEvent
- Hướng dẫn và ví dụ Javascript KeyboardEvent
- Hướng dẫn và ví dụ Javascript FocusEvent
- Hướng dẫn và ví dụ Javascript InputEvent
- Hướng dẫn và ví dụ Javascript ChangeEvent
- Hướng dẫn và ví dụ Javascript DragEvent
- Hướng dẫn và ví dụ Javascript HashChangeEvent
- Hướng dẫn và ví dụ Javascript URL Encoding
- Hướng dẫn và ví dụ Javascript FileReader
- Hướng dẫn và ví dụ Javascript XMLHttpRequest
- Hướng dẫn và ví dụ Javascript Fetch API
- Phân tích XML trong Javascript với DOMParser
- Giới thiệu về Javascript HTML5 Canvas API
- Làm nổi bật Code với thư viện Javascript SyntaxHighlighter
- Polyfill là gì trong khoa học lập trình?
Show More