Hướng dẫn và ví dụ Flutter ListTile
Trong Flutter, ListTile là một thành phần giao diện người dùng, nó có cấu trúc giống hình minh hoạ dưới đây:
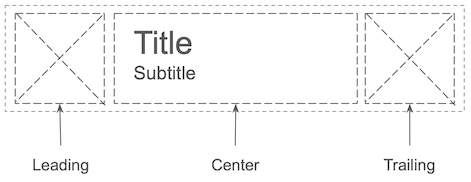
ListTile
ListTile có chiều rộng lấp đầy chiều rộng của phần tử cha và vùng "Center" của nó có xu hướng mở rộng chiều rộng nhiều nhất có thể.
ListTile có thể được sử dụng một cách độc lập hoặc được sử dụng như một phần tử của ListView.
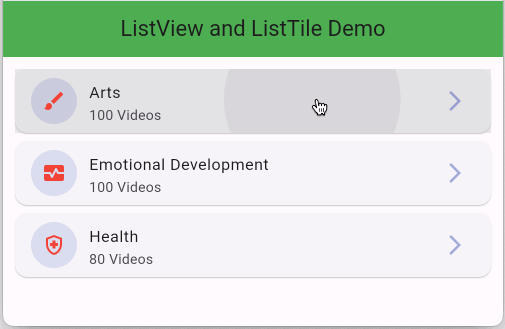
- Flutter ExpansionTile
1. ListTile
Constructor của ListTile:
ListTile({
Key key,
Widget leading,
Widget title,
Widget subtitle,
Widget trailing,
bool isThreeLine: false,
bool dense,
VisualDensity visualDensity,
ShapeBorder shape,
EdgeInsetsGeometry contentPadding,
bool enabled: true,
GestureTapCallback onTap,
GestureLongPressCallback onLongPress,
MouseCursor mouseCursor,
bool selected: false,
Color focusColor,
Color hoverColor,
FocusNode focusNode,
bool autofocus: false,
});
2. Ví dụ đơn giản
Ví dụ dưới đây là một ListTile đơn giản với hai trạng thái "được lựa chọn" và "không được lựa chọn".
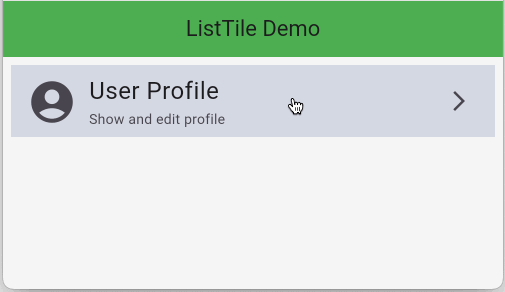
listtile_ex1.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key});
@override
State<StatefulWidget> createState() {
return _MyHomePageState();
}
}
class _MyHomePageState extends State<MyHomePage> {
bool _selected = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ListTile Demo'),
backgroundColor: Colors.green,
),
backgroundColor: Colors.grey[100],
body: Padding(
padding: const EdgeInsets.all(8.0),
child: Container(
decoration: BoxDecoration(color: Colors.indigo.withAlpha(30)),
child: ListTile(
leading: const Icon(
Icons.account_circle,
size: 50,
),
title: const Text(
'User Profile',
style: TextStyle(fontSize: 24),
),
trailing: const Icon(Icons.arrow_forward_ios),
subtitle: const Text('Show and edit profile'),
selected: _selected,
onTap: () {
_selected = !_selected;
setState(() {});
},
),
),
),
);
}
}
3. Sử dụng ListTile với Card và ListView
Bạn có thể sử dụng ListTile cùng với ListView và Card. Trong đó:
- ListTile được bao bọc trong một Card.
- ListView được sử dụng để hiển thị danh sách các Card(s).
Một ListTile được bao bọc bởi một Card:

category_tile.dart
import 'package:flutter/material.dart';
class CategoryTile extends StatelessWidget {
String categoryTitle;
int videoCount;
Icon icon;
CategoryTile({
required this.categoryTitle,
required this.videoCount,
required this.icon,
});
@override
Widget build(BuildContext context) {
return Card(
color: Colors.white,
child: ListTile(
leading: Container(
width: 46,
height: 46,
decoration: ShapeDecoration(
color: Colors.indigo.withAlpha(40),
shape: const CircleBorder(),
),
child: icon,
),
title: Text(categoryTitle),
subtitle: Text("$videoCount Videos"),
trailing: Icon(
Icons.arrow_forward_ios,
color: Colors.indigo.withAlpha(120),
),
onTap: () {},
),
);
}
}
Một ListView hiển thị danh sách các Card(s):
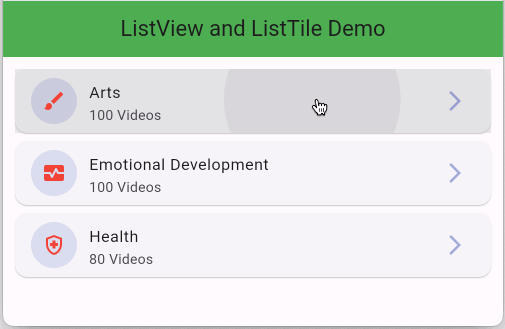
listtile_ex2.dart
import 'package:flutter/material.dart';
import 'package:listtile/category_tile.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ListView and ListTile Demo'),
backgroundColor: Colors.green,
),
backgroundColor: Colors.grey[120],
body: Padding(
padding: const EdgeInsets.all(8.0),
child: ListView(
children: [
CategoryTile(
categoryTitle: 'Arts',
videoCount: 100,
icon: Icon(Icons.brush, color: Colors.red),
),
CategoryTile(
categoryTitle: 'Emotional Development',
videoCount: 100,
icon: Icon(Icons.monitor_heart, color: Colors.red),
),
CategoryTile(
categoryTitle: 'Health',
videoCount: 80,
icon: Icon(Icons.health_and_safety_outlined, color: Colors.red),
),
],
),
),
);
}
}
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More