Hướng dẫn và ví dụ Flutter Banner
1. Flutter Banner
Trong Flutter, Banner là thông điệp chéo (diagonal message) hiển thị ở phía trên bề mặt và tại một góc của một Widget khác. Banner thường được sử dụng để trang trí và làm nổi bật một thông điệp liên quan tới một Widget này.
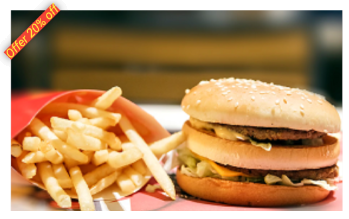
Banner Constructor:
Banner Constructor
const Banner(
{Key key,
Widget child,
@required String message,
TextDirection textDirection,
@required BannerLocation location,
TextDirection layoutDirection,
Color color: _kColor,
TextStyle textStyle: _kTextStyle}
)
2. Banner Example
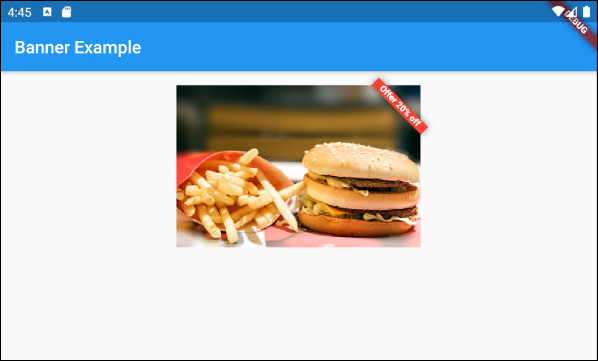
main.dart (ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Banner Example"),
),
body: Container(
padding: EdgeInsets.all(16),
child: Align(
alignment: Alignment.topCenter,
child: Banner (
message: 'Offer 20% off',
location: BannerLocation.topEnd,
color: Colors.red,
child: Container(
height: 186,
width: 280,
child: Image.network(
'https://raw.githubusercontent.com/o7planning/rs/master/flutter/fast_food.png',
fit: BoxFit.cover,
),
),
),
),
)
);
}
}
3. child
Property child được sử dụng để định nghĩa nội dung nằm dưới "banner". Trong hầu hết các trường hợp sử dụng nó là một Widget chứa một hình ảnh.
Widget child
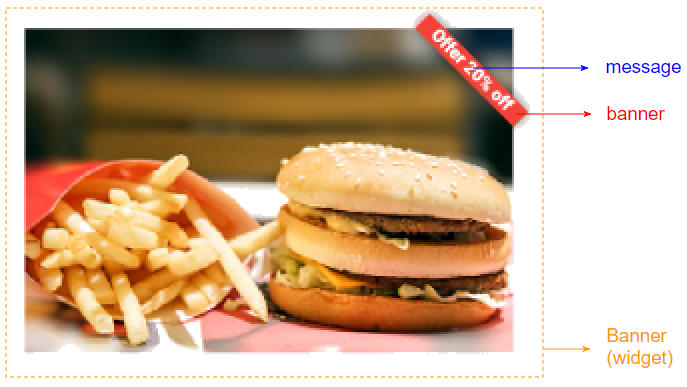
child cũng có thể là một nội dung hỗn hợp bao gồm hình ảnh và văn bản:
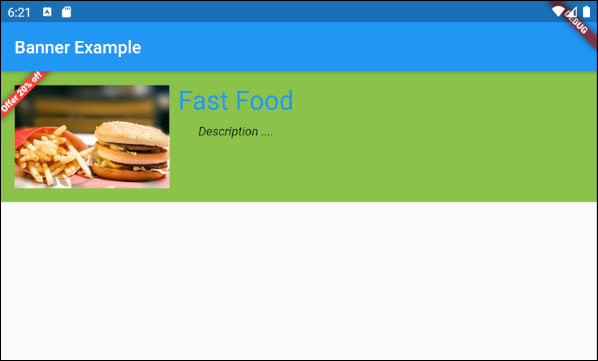
main.dart (child ex2)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold (
appBar: AppBar(
title: Text("Banner Example"),
),
body: Banner (
message: 'Offer 20% off',
location: BannerLocation.topStart,
color: Colors.red,
child: Container(
height: 150,
width: double.infinity,
color: Colors.lightGreen,
child: Padding (
padding: EdgeInsets.all(16),
child: Row (
children: [
Image.network (
"https://raw.githubusercontent.com/o7planning/rs/master/flutter/fast_food.png"
),
SizedBox(width: 10),
Column (
children: [
Text("Fast Food",style: TextStyle(fontSize: 30, color: Colors.blue)),
SizedBox(height: 10),
Text("Description ....", style: TextStyle(fontStyle: FontStyle.italic))
],
)
],
),
),
),
)
);
}
}
4. message
Property message định nghĩa một thông điệp hiển thị trên "banner".

@required String message
5. layoutDirection
Property layoutDirection được sử dụng để chỉ định hướng của bố cục. Mặc định là giá trị của nó là TextDirection.ltr (Left to Right).
TextDirection layoutDirection
// Enum values:
TextDirection.ltr // Left to Right (Default)
TextDirection.rtl // Right to Left
Khái niệm Layout Direction (Hướng của bố cục) giúp tạo ra ứng dụng phù hợp với các ngôn ngữ và nền văn hóa khác nhau. Cụ thể tiếng Anh được viết từ trái sang phải, trong khi đó tiếng Ả Rập được viết từ phải sang trái.
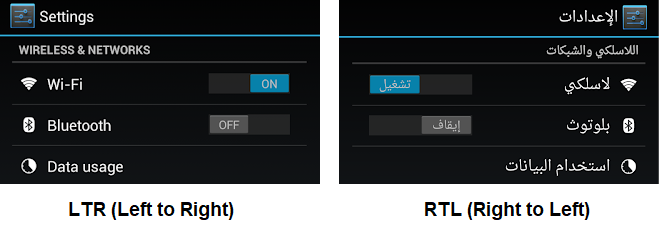
6. location
Property location được sử dụng để chỉ định vị trí hiển thị "banner", nó có thể nhận một trong 4 giá trị sau:
- BannerLocation.topStart
- BannerLocation.topEnd
- BannerLocation.bottomStart
- BannerLocation.bottomEnd
@required BannerLocation location
Nếu hướng của bố cục (Layout Direction) là từ Trái sang Phải:
- layoutDirection: TextDirection.ltr (Default)
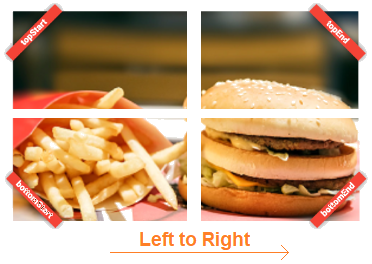
Nếu hướng của bố cục (Layout Direction) là từ Phải sang Trái:
- layoutDirection: TextDirection.rtl
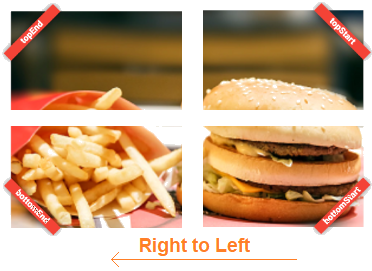
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More