Navigation và Routing trong Flutter
1. Route Transition
Flutter đưa ra khái niệm "Route Transition" (Sự chuyển đổi lộ trình) để mô tả hành động bạn nhẩy từ màn hình thứ nhất tới màn hình thứ hai, và quá trình chuyển đổi (transition) này có thể bao gồm một hiệu ứng hoạt hình để mang lại cảm nhận tốt đối với người dùng. Trong bài viết này tôi sẽ trình bày một vài cách để đạt được điều đó.
Để đơn giản bạn có thể xem hình minh hoạ dưới đây, sau đó chúng ta sẽ phân tích cách bước để thực hiện nó:
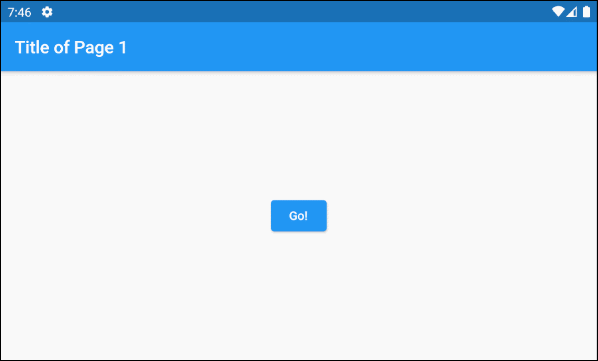
Ban đầu người dùng đứng tại trang thứ nhất (Page1) và thực hiện một hành động nào đó, chẳng hạn nhấn vào một button để nhẩy tới trang thứ hai (Page2), một hiệu ứng hoạt hình có thể xuất hiện trong quá trình chuyển đổi giữa hai trang, tuy nhiên trong ví dụ đơn giản này tôi không tạo ra hiệu ứng hoạt hình.
main.dart (ex 1)
import 'package:flutter/material.dart';
main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: Page1(),
);
}
}
class Page1 extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Title of Page 1"),
),
body: Center(
child: ElevatedButton(
child: Text('Go!'),
onPressed: () {
Navigator.of(context).push(_createRoute());
},
),
),
);
}
}
Route _createRoute() {
return PageRouteBuilder(
pageBuilder: (BuildContext context, Animation<double> animation,//
Animation<double> secondaryAnimation) {
return Page2();
},
transitionsBuilder: (BuildContext context, Animation<double> animation, //
Animation<double> secondaryAnimation, Widget child) {
return child;
},
);
}
class Page2 extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Title of Page 2"),
),
body: Center(
child: Text('Page 2'),
),
backgroundColor: Colors.lightGreen[100],
);
}
}
Điều quan trọng nhất bạn cần tạo ra một đối tượng Route (Lộ trình) để mô tả cách trang thứ nhất được thay thế bởi trang thứ hai. Lớp PageRouteBuilder là một lớp hậu duệ của Route, nó được sử dụng cho ví dụ đầu tiên vì nó dễ dàng sử dụng.
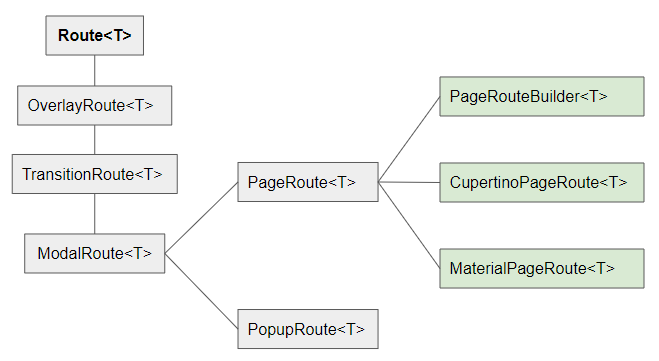
_createRoute()
Route _createRoute() {
return PageRouteBuilder(
pageBuilder: (BuildContext context, Animation<double> animation,//
Animation<double> secondaryAnimation) {
return Page2();
},
transitionsBuilder: (BuildContext context, Animation<double> animation, //
Animation<double> secondaryAnimation, Widget child) {
return child;
},
);
}
Sau đó sử dụng Navigator (bộ điều hướng) thực thi Route (lộ trình).
Navigator.of(context).push(_createRoute());
Tiếp tục với ví dụ trên, chúng ta thêm hiệu ứng hoạt hình SlideTransition vào giữa quá trình chuyển đổi từ trang thứ nhất sang trang thứ hai:
(ex 1b)
Route _createRoute() {
return PageRouteBuilder(
pageBuilder: (BuildContext context, Animation<double> animation,//
Animation<double> secondaryAnimation) {
return Page2();
},
transitionsBuilder: (BuildContext context, Animation<double> animation, //
Animation<double> secondaryAnimation, Widget child) {
return SlideTransition(
position: new Tween<Offset>(
begin: const Offset(-1.0, 0.0),
end: Offset.zero,
).animate(animation),
child: new SlideTransition(
position: new Tween<Offset>(
begin: Offset.zero,
end: const Offset(-1.0, 0.0),
).animate(secondaryAnimation),
child: child,
),
);
},
);
}
Và nhận được kết quả:
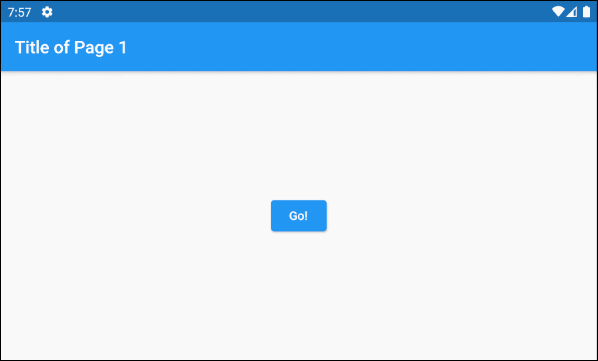
Và ví dụ tiếp theo với hiệu ứng hoạt hình ScaleTransition:
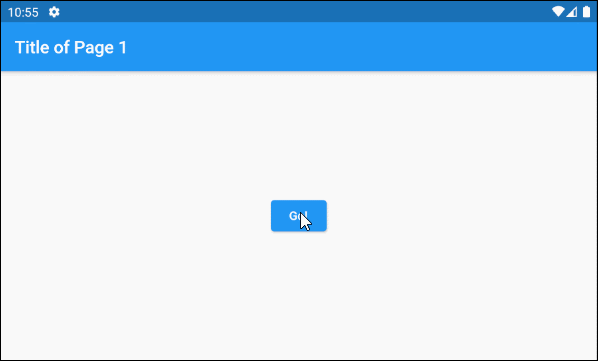
(ex 1c)
Route _createRoute() {
return PageRouteBuilder(
pageBuilder: (BuildContext context, Animation<double> animation,//
Animation<double> secondaryAnimation) {
return Page2();
},
transitionsBuilder: (BuildContext context, Animation<double> animation, //
Animation<double> secondaryAnimation, Widget child) {
return new ScaleTransition(
scale: new Tween<double>(
begin: 0.0,
end: 1.0,
).animate(
CurvedAnimation(
parent: animation,
curve: Interval(
0.00,
0.50,
curve: Curves.easeInCirc,
),
),
),
child: child
);
},
);
}
- Hướng dẫn và ví dụ Flutter SlideTransition
- Hướng dẫn và ví dụ Flutter ScaleTransition
2. Named Route
Trong Flutter bạn cũng có thể định nghĩa một bản đồ (Map) chứa các trang chính của ứng dụng và tên tương ứng của chúng, sau đó sử dụng Navigator để di chuyển tới các trang khác nhau dựa trên các tên này.
Trước hết hãy xem trước kết quả của ví dụ:
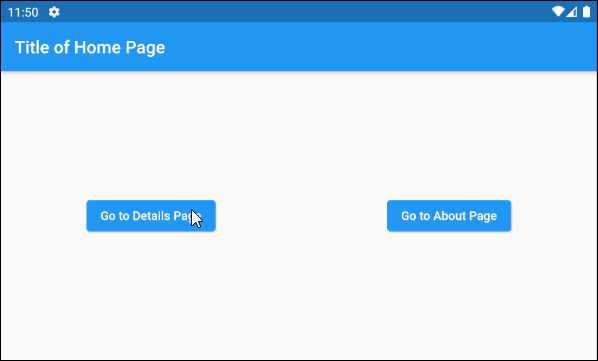
main.dart (ex 4)
import 'package:flutter/material.dart';
main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
initialRoute: '/home',
routes: <String, WidgetBuilder>{
'/home': (BuildContext context) => Home(),
'/details': (BuildContext context) => Details(),
'/about': (BuildContext context) => About(),
},
);
}
}
class Home extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Title of Home Page"),
),
body: Center(
child: Row (
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
ElevatedButton(
child: Text('Go to Details Page'),
onPressed: () {
Navigator.of(context).pushNamed('/details');
},
),
ElevatedButton(
child: Text('Go to About Page'),
onPressed: () {
Navigator.of(context).pushNamed('/about');
},
),
],
)
),
);
}
}
class Details extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Title of Details Page"),
),
body: Center(
child: ElevatedButton(
child: Text('Close'),
onPressed: () {
// Close page and pass a value back to previous page
Navigator.of(context).pop();
},
),
),
backgroundColor: Colors.lightGreen[100],
);
}
}
class About extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Title of About Page"),
),
body: Center(
child: ElevatedButton(
child: Text('Close'),
onPressed: () {
// Close page
Navigator.of(context).pop();
},
),
),
backgroundColor: Colors.cyan[100],
);
}
}
Trong ví dụ này chúng ta sử dụng property routes của MaterialApp để định nghĩa một route Map chứa các trang chính của ứng dụng và tên tương ứng của chúng.
// Map<String, WidgetBuilder> routes
MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
initialRoute: '/home',
routes: <String, WidgetBuilder>{
'/home': (BuildContext context) => Home(),
'/details': (BuildContext context) => Details(),
'/about': (BuildContext context) => About(),
},
);
Và nhẩy tới các trang khác nhau dựa trên tên của route.
Navigator.of(context).pushNamed('/details');
Quay trở lại trang trước:
// Close page (Back to previous page)
Navigator.of(context).pop();
// Close page and pass a value back to previous page.
Navigator.of(context).pop("Some Value");
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More