Ví dụ Flutter Navigator pushNamedAndRemoveUntil
pushNamedAndRemoveUntil là một phương thức tĩnh của lớp Navigator. Trong bài học này chúng ta sẽ xem xét cách sử dụng phương thức này thông qua một vài ví dụ.
1. pushNamedAndRemoveUntil
typedef RoutePredicate = bool Function(Route<dynamic> route);
@optionalTypeArgs
static Future<T?> pushNamedAndRemoveUntil<T extends Object?>(
BuildContext context,
String newRouteName,
RoutePredicate predicate,
{Object? arguments}
)
Các phương thức Navigator.pushAndRemoveUntil() và Navigator.pushNamedAndRemoveUntil() được sử dụng để nhẩy đến một màn hình mới đồng thời loại bỏ một vài tuyến đường đã đi qua trong lịch sử cho tới khi RoutePredicate trả về true.
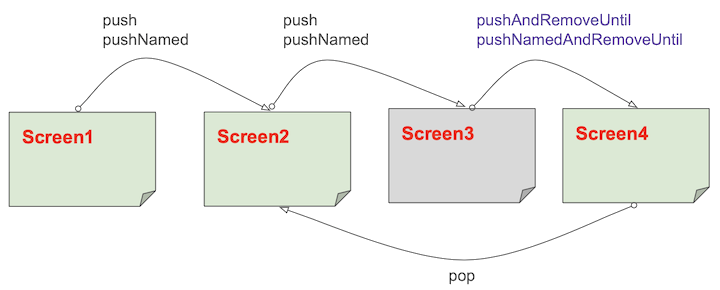
Navigator.pushNamedAndRemoveUntil(
context,
RouteNames.routeD4, // "/routeD4"
(Route<dynamic> route) {
String? routeName= route.settings.name;
return routeName == RouteNames.routeD2;
},
);
Ví dụ, bạn tạo ra một ứng dụng tương tự Facebook hoặc Instagram, người dùng sau khi xem nhiều trang (màn hình) họ đăng xuất ra khỏi ứng dụng. Sau khi đăng xuất, ứng dụng nhẩy tới màn hình Home và việc loại bỏ lịch sử các tuyến đường (route) và người dùng đã trải qua trước đó là điều cần thiết.
Navigator.of(context).pushNamedAndRemoveUntil(
'/home',
(Route<dynamic> route) => false,
);
// OR:
Navigator.pushNamedAndRemoveUntil(
context,
'/home',
(Route<dynamic> route) => false,
);
2. Ví dụ pushNamedAndRemoveUntil()
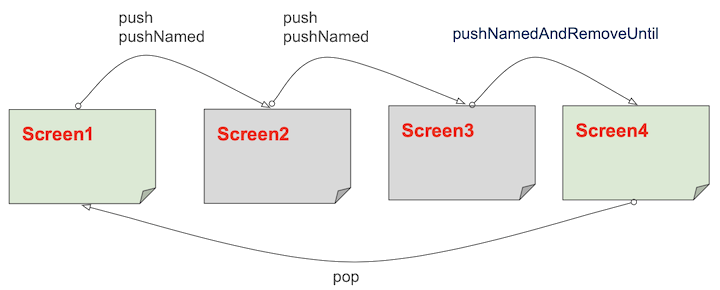
Trong ví dụ này, chúng ta sử dụng phương thức pushNamedAndRemoveUntil() để nhẩy từ màn hình 3 tới màn hình 4, đồng thời loại bỏ lịch sử các tuyến đường ngoại trừ màn hình 1.
//
// Code of Screen D3
//
Navigator.pushNamedAndRemoveUntil(
context,
RouteNames.routeD4, // "/routeD4"
(Route<dynamic> route) => route.isFirst,
);
Code đầy đủ của ví dụ:
push_named_and_remove_until_ex.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class RouteNames {
static const String routeD1 = "/screenD1";
static const String routeD2 = "/screenD2";
static const String routeD3 = "/screenD3";
static const String routeD4 = "/screenD4";
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Navigator Demo (pushNamed)',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
initialRoute: RouteNames.routeD1,
routes: {
RouteNames.routeD1: (context) => const ScreenD1(),
RouteNames.routeD2: (context) => const ScreenD2(),
RouteNames.routeD3: (context) => const ScreenD3(),
RouteNames.routeD4: (context) => const ScreenD4(),
},
);
}
}
class ScreenD1 extends StatelessWidget {
const ScreenD1({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: [
const Text("This is Screen D1"),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
Navigator.pushNamed(context, RouteNames.routeD2);
},
child: const Text("Go to Screen D2 >>"),
),
],
),
),
);
}
}
class ScreenD2 extends StatelessWidget {
const ScreenD2({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: [
const Text(
"This is Screen D2",
style: TextStyle(
color: Colors.red,
),
),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
Navigator.pushNamed(context, RouteNames.routeD3);
},
child: const Text("Go to Screen D3 >>"),
),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
Navigator.pop(context); // Back!!
},
child: const Text("<< Back"),
),
],
),
),
);
}
}
class ScreenD3 extends StatelessWidget {
const ScreenD3({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: [
const Text(
"This is Screen D3",
style: TextStyle(
color: Colors.red,
),
),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
Navigator.pushNamedAndRemoveUntil(
context,
RouteNames.routeD4, // "/routeD4"
(Route<dynamic> route) => route.isFirst,
);
},
child: const Text("Go to Screen D4 >>"),
),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
Navigator.pop(context); // Back!!
},
child: const Text("<< Back"),
),
],
),
),
);
}
}
class ScreenD4 extends StatelessWidget {
const ScreenD4({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: [
const Text(
"This is Screen D4",
style: TextStyle(
color: Colors.red,
),
),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
Navigator.pop(context); // Back!!
},
child: const Text("<< Back"),
),
],
),
),
);
}
}
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More