Bài thực hành Flutter thiết kế trang Profile với Stack
Trong bài thực hành Flutter này, chúng ta sẽ thiết kế một trang profile giống hình minh hoạ dưới đây.
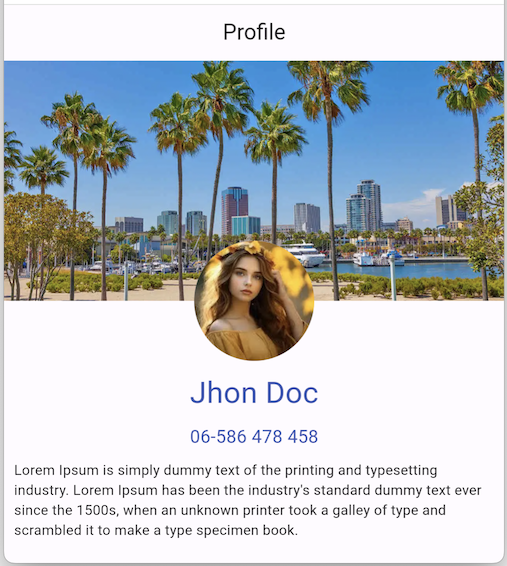
Các hình ảnh được sử dụng trong bài thực hành này:
1. Phác thảo thiết kế và gợi ý
Để đơn giản chúng ta sẽ phân chia trang profile thành 2 phần (section), tương đương với 2 lớp:
- UserAvatarSection
- UserInfoSection
UserAvatarSection
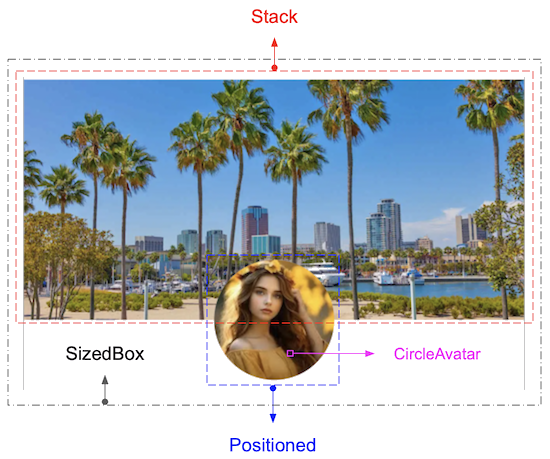
Stack(
clipBehavior: Clip.none,
children: [
Container(
// Background Image
),
Positioned(
bottom: -60,
left: 0,
right: 0,
CircleAvatar(
radius: 60,
// Background Image
),
),
],
)
UserInfoSection
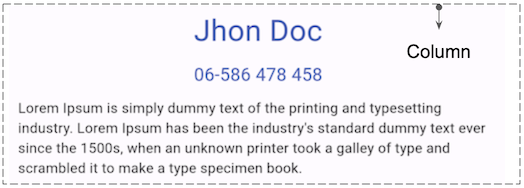
2. UserData
Tạo lớp UserData mô phỏng người dùng.
_user_data.dart
class UserData {
final String backgroundImageUrl;
final String userImageUrl;
final String fullName;
final String phone;
final String description;
UserData(
{required this.backgroundImageUrl,
required this.userImageUrl,
required this.fullName,
required this.phone,
required this.description});
}
var user1 = UserData(
backgroundImageUrl:
'https://raw.githubusercontent.com/o7planning/rs/master/flutter/long-beach.webp',
userImageUrl:
'https://raw.githubusercontent.com/o7planning/rs/master/flutter/users/user2.webp',
fullName: 'Jhon Doc',
phone: '06-586 478 458',
description:
"Lorem Ipsum is simply dummy text of the printing and typesetting industry. "
"Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, "
"when an unknown printer took a galley of type and scrambled it to make a type specimen book.",
);
3. UserAvatarSection
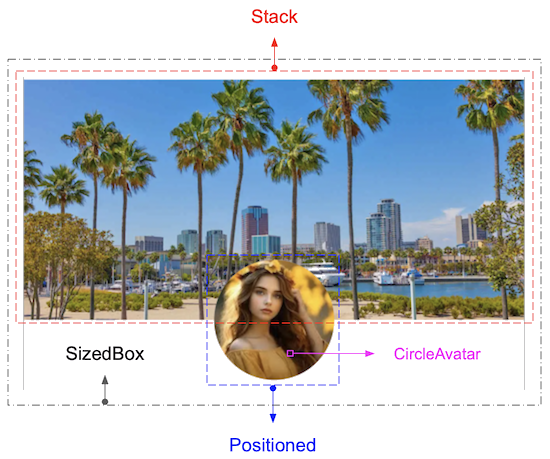
Chú ý: Để ảnh nền của CircleAvatar khớp (fit) với kích thước của CircleAvatar bạn có thể đặt nó trong một Center.
user_avatar_section.dart
import 'package:flutter/material.dart';
import '_user_data.dart';
class UserAvatarSection extends StatelessWidget {
final UserData userData;
const UserAvatarSection({super.key, required this.userData});
@override
Widget build(BuildContext context) {
return SizedBox(
height: 240,
child: Stack(
clipBehavior: Clip.none,
children: [
Container(
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(userData.backgroundImageUrl),
fit: BoxFit.fitWidth,
),
),
),
Positioned(
bottom: -60,
left: 0,
right: 0,
// Need a Center widget to make background image fit with CircleAvatar
child: Center(
child: CircleAvatar(
radius: 60,
backgroundImage: NetworkImage(userData.userImageUrl),
),
),
)
],
),
);
}
}
4. UserInfoSection
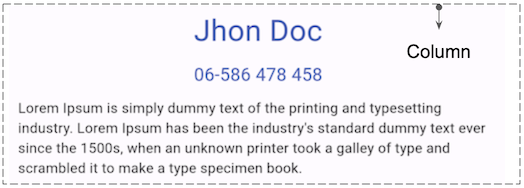
user_info_section.dart
import 'package:flutter/material.dart';
import '_user_data.dart';
class UserInfoSection extends StatelessWidget {
final UserData userData;
const UserInfoSection({required this.userData, super.key});
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text(
userData.fullName,
style: const TextStyle(
fontSize: 30,
color: Colors.indigo,
),
),
const SizedBox(height: 10),
Text(
userData.phone,
style: const TextStyle(
fontSize: 18,
color: Colors.indigo,
),
),
const SizedBox(height: 10),
Text(userData.description),
],
),
);
}
}
5. UserProfileScreen
Lắp ghép tất cả các phần (section) đã thiết kết ở trên để tạo ra trang Profile.
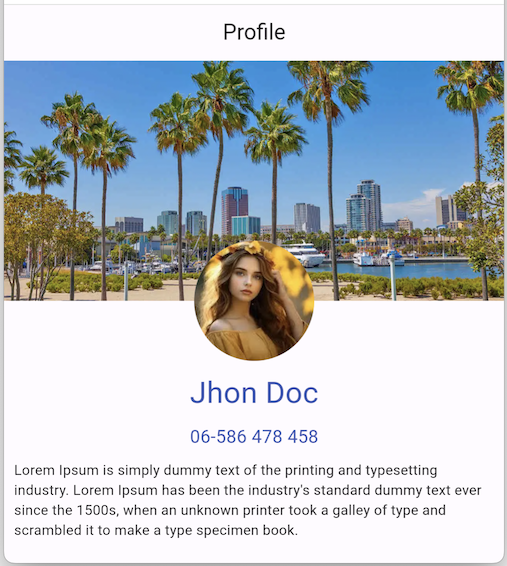
user_profile_screen.dart
import 'package:flutter/material.dart';
import 'user_avatar_section.dart';
import 'user_info_section.dart';
import '_user_data.dart';
class UserProfileScreen extends StatelessWidget {
final UserData userData;
const UserProfileScreen({super.key, required this.userData});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Profile'),
),
body: SingleChildScrollView(
child: Column(
children: [
UserAvatarSection(userData: userData),
const SizedBox(height: 60),
UserInfoSection(userData: userData)
],
),
),
);
}
}
6. MyApp
main.dart
import 'dart:async';
import 'package:flutter/material.dart';
import 'user_profile_screen.dart';
import '_user_data.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: UserProfileScreen(userData: user1),
);
}
}
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More