Hướng dẫn và ví dụ Flutter Column
1. Column
Column là một widget hiển thị các widget con của nó trên một cột. Một biến thể khác là Row, hiển thị các widget con của nó trên một hàng.
Để làm cho một widget con của Column có thể mở rộng lấp đầy khoảng không gian thẳng đứng sẵn có bạn có thể gói nó bên trong một đối tượng Expanded.
Column đặt các con của nó trên một cột và không thể cuộn, nếu bạn muốn có một bộ chứa (container) tương tự và có thể cuộn được hãy cân nhắc sử dụng ListView.
Column Constructor:
Column Constructor
Column(
{Key key,
List<Widget> children: const <Widget>[],
MainAxisAlignment mainAxisAlignment: MainAxisAlignment.start,
MainAxisSize mainAxisSize: MainAxisSize.max,
CrossAxisAlignment crossAxisAlignment: CrossAxisAlignment.center,
TextDirection textDirection,
VerticalDirection verticalDirection: VerticalDirection.down,
TextBaseline textBaseline
}
)
2. children
Property children được sử dụng để định nghĩa một danh sách các widget con của Column.
Bạn có thể thêm các widget con vào children, hoặc loại bỏ các widget ra khỏi children, tuy nhiên bạn phải tuân thủ một quy tắc sẽ được đề cập trong mục "Add/Remove Children".
List<Widget> children: const <Widget>[]
Chúng ta bắt đầu với ví dụ đầu tiên, một Column với 4 widget con:
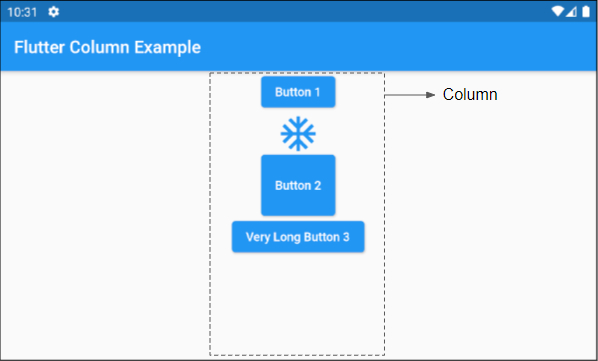
main.dart (children ex1)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Column Example")
),
body: Center(
child: Column (
children: [
ElevatedButton(child: Text("Button 1"), onPressed:(){}),
Icon(Icons.ac_unit, size: 48, color: Colors.blue),
ElevatedButton(
child: Text("Button 2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size.square(70))
)
),
ElevatedButton(child: Text("Very Long Button 3"), onPressed:(){}),
]
)
),
);
}
}
Một vài loại widget con với hệ số flex > 0 có khả năng mở rộng chiều cao của nó để lấp đầy không gian còn lại theo chiều thẳng đứng chẳng hạn Expanded, Spacer,.. chúng thường được sử dụng để điều chỉnh khoảng cách giữa các widget con của Column, dưới đây là một ví dụ như vậy:
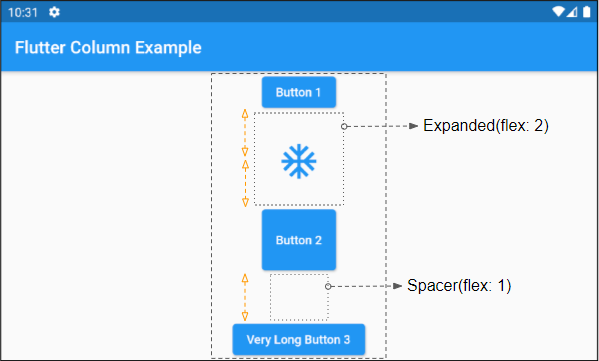
children (ex2)
Column (
children: [
ElevatedButton(child: Text("Button 1"), onPressed:(){}),
Expanded (
flex: 2,
child: Icon(Icons.ac_unit, size: 48, color: Colors.blue)
),
ElevatedButton(
child: Text("Button 2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size.square(70))
)
),
Spacer(flex: 1),
ElevatedButton(child: Text("Very Long Button 3"), onPressed:(){}),
]
)
3. Add/Remove children
Bạn có thể thêm một vài widget con vào một Column hoặc loại bỏ một vài widget con ra khỏi Column, và cách làm như dưới đây có thể mang đến một kết quả không như mong đợi:
** Not Working! **
class SomeWidgetState extends State<SomeWidget> {
List<Widget> _children;
void initState() {
_children = [];
}
void someHandler() {
setState(() {
_children.add(newWidget);
});
}
Widget build(BuildContext context) {
// Reusing `List<Widget> _children` here is problematic.
return Column(children: this._children);
}
}
Để giải quyết vấn đề nêu trên bạn cần tuân thủ các quy tắc sau:
- Các widget con cần được chỉ định giá trị Key một cách rõ ràng, điều này giúp cho Flutter nhận biết các widget con cũ hoặc mới khi số lượng các widget con thay đổi.
- Cần tạo một đối tượng List mới cho Column.children nếu có một widget con nào đó thay đổi, hoặc số lượng các widget con thay đổi.
** Worked! **
class SomeWidgetState extends State<SomeWidget> {
List<Widget> _children;
void initState() {
this._children = [];
}
// Add or remove some children..
void someHandler() {
setState(() {
// The key here allows Flutter to reuse the underlying render
// objects even if the children list is recreated.
this._children.add(newWidget(key: ...));
});
}
Widget build(BuildContext context) {
// Always create a new list of children as a Widget is immutable.
var newChildren = List.from(this._children);
this._children = newChildren;
return Column(children: this._children);
}
}
Ví dụ:
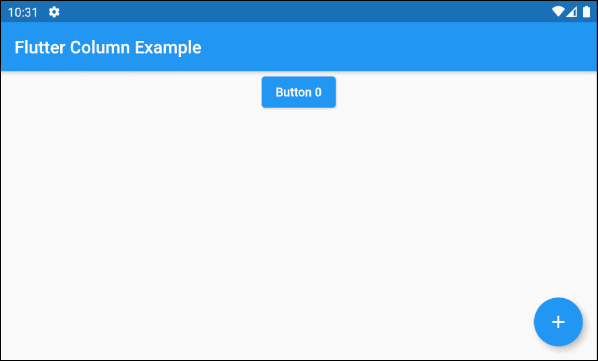
main.dart (children ex3)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'o7planning.org',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key}) : super(key: key);
@override
State<StatefulWidget> createState() {
return MyHomePageState();
}
}
class MyHomePageState extends State<MyHomePage> {
List<Widget> _children = [];
int idx = 0;
@override
void initState() {
super.initState();
this._children = [
ElevatedButton(
key: Key(this.idx.toString()),
child: Text("Button " + idx.toString()),
onPressed: (){}
)
];
}
void addChildHandler() {
this.idx++;
this.setState(() {
var newChild = ElevatedButton(
key: Key(this.idx.toString()),
child: Text("Button " + idx.toString()),
onPressed: (){}
);
this._children.add(newChild);
});
}
@override
Widget build(BuildContext context) {
// Create new List object:
this._children = this._children == null? [] : List.from(this._children);
return Scaffold(
appBar: AppBar(
title: Text("Flutter Column Example")
),
body: Center(
child: Column (
children: this._children
)
),
floatingActionButton: FloatingActionButton(
child: Icon(Icons.add),
onPressed: () {
this.addChildHandler();
}
),
);
}
}
4. mainAxisAlignment
Property mainAxisAlignment được sử dụng để chỉ định cách mà các widget con sẽ được bố trí trên trục chính (main axis). Đối với Column, trục chính (main axis) là chính là trục thẳng đứng.
MainAxisAlignment mainAxisAlignment: MainAxisAlignment.start
MainAxisAlignment.start
Với verticalDirection = VerticalDirection.down (Mặc định) và mainAxisAlignment = MainAxisAlignment.start thì các widget con của Column sẽ được đặt sát nhau từ trên xuống dưới.
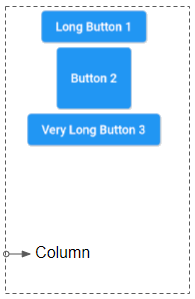
MainAxisAlignment.start
Column (
mainAxisAlignment: MainAxisAlignment.start,
children: [
ElevatedButton(child: Text("Long Button 1"), onPressed:(){}),
ElevatedButton(
child: Text("Button 2"),
onPressed:(){},
style: ButtonStyle(
minimumSize: MaterialStateProperty.all(Size.square(70))
)
),
ElevatedButton(child: Text("Very Long Button 3"), onPressed:(){})
]
)
MainAxisAlignment.center
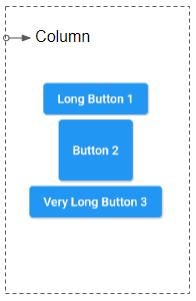
mainAxisAlignment: MainAxisAlignment.center
MainAxisAlignment.end
Với verticalDirection = VerticalDirection.down (Mặc định) và mainAxisAlignment = MainAxisAlignment.end thì các widget con của Column sẽ được đặt sát nhau từ dưới lên trên.
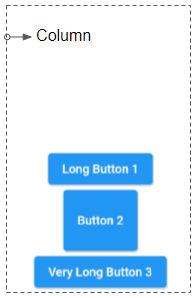
mainAxisAlignment: MainAxisAlignment.end
MainAxisAlignment.spaceBetween
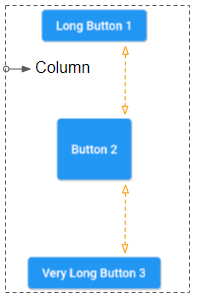
mainAxisAlignment: MainAxisAlignment.spaceBetween
MainAxisAlignment.spaceEvenly
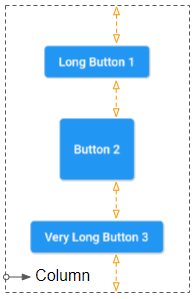
mainAxisAlignment: MainAxisAlignment.spaceEvenly
MainAxisAlignment.spaceAround
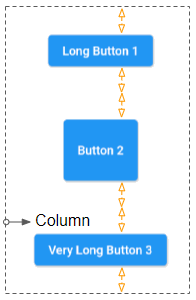
mainAxisAlignment: MainAxisAlignment.spaceAround
5. mainAxisSize
Property mainAxisSize chỉ định bao nhiêu không gian thẳng đứng nên được chiếm giữ bởi Column, giá trị mặc định của nó là MainAxisSize.max điều này có nghĩa là Column sẽ cố gắng chiếm giữ không gian thẳng đứng nhiều nhất có thể.
Nếu có một widget con với "flex > 0 && fit != FlexFit.loose" thì Column sẽ cố gắng chiếm giữ nhiều không gian nhiều nhất có thể mà không phụ thuộc vào giá trị của mainAxisSize.
Ngược lại, nếu mainAxisSize = MainAxisSize.min thì Column sẽ có một chiều cao vừa đủ cho tất cả các widget con của nó.
MainAxisSize mainAxisSize: MainAxisSize.max
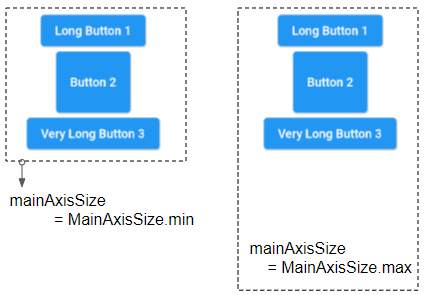
6. crossAxisAlignment
Property crossAxisAlignment được sử dụng để chỉ định cách mà các widget con sẽ được bố trí trên trục chéo (cross axis). Đối với Column, trục chéo (cross axis) chính là trục nằm ngang.
CrossAxisAlignment crossAxisAlignment: CrossAxisAlignment.center
CrossAxisAlignment.start
Với textDirection = TextDirection.ltr (Mặc định) và crossAxisAlignment = CrossAxisAlignment.start thì các widget con của Column sẽ được đặt sát cạnh trái của nó.
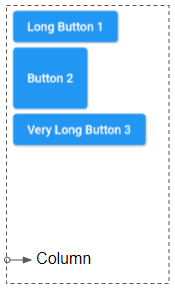
CrossAxisAlignment.center (Default).
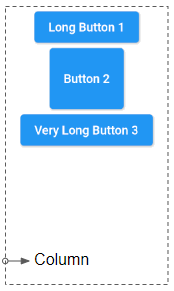
crossAxisAlignment: CrossAxisAlignment.center
CrossAxisAlignment.end
Với textDirection = TextDirection.ltr (Mặc định) và crossAxisAlignment = CrossAxisAlignment.end thì các widget con của Column sẽ được đặt sát cạnh bên phải của Column.
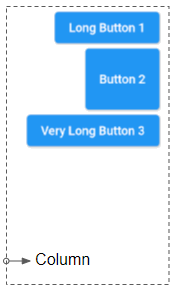
crossAxisAlignment: CrossAxisAlignment.end
CrossAxisAlignment.stretch
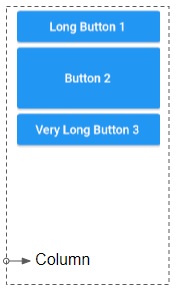
crossAxisAlignment: CrossAxisAlignment.stretch
CrossAxisAlignment.baseline
crossAxisAlignment: CrossAxisAlignment.baseline,
textBaseline: TextBaseline.alphabetic
7. textDirection
Property textDirection chỉ định cách mà các widget con của Column sẽ được bố trí trên trục nằm ngang và cách giải thích từ "start" và "end".
TextDirection textDirection
// TextDirection enum:
TextDirection.ltr (Left to Right) (Default)
TextDirection.rtl (Right to Left)
Nếu textDirection = TextDirection.ltr (Mặc định), từ "start" sẽ tương ứng với "left" và từ "end" sẽ tương ứng với "right".
Ngược lại, nếu textDirection = TextDirection.rtl, từ "start" sẽ tương ứng với "right" và từ "end" tương ứng với "left".
8. verticalDirection
Property verticalDirection chỉ định cách mà các widget con của Column sẽ được bố trí trên trục chính (trục thẳng đứng) và cách giải thích từ "start" và "end".
VerticalDirection verticalDirection: VerticalDirection.down
// VerticalDirection enum:
VerticalDirection.down (Default)
VerticalDirection.up
Nếu verticalDirection = VerticalDirection.down (Mặc định), từ "start" sẽ tương ứng với "top" và từ "end" sẽ tương ứng với "bottom".
Ngược lại, nếu verticalDirection = VerticalDirection.up, từ "start" sẽ tương ứng với "bottom" và từ "end" tương ứng với "top".
9. textBaseline
Nếu căn lề (align) các widget con dựa trên đường cơ sở (baseline), property textBaseline sẽ chỉ định loại đường cơ sở nào sẽ được sử dụng.
TextBaseline textBaseline: TextBaseline.alphabetic
// TextBaseline enum:
TextBaseline.alphabetic (Default)
TextBaseline.ideographic
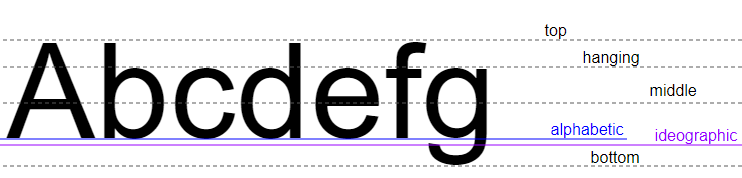
Ví dụ:
crossAxisAlignment: CrossAxisAlignment.baseline,
textBaseline: TextBaseline.alphabetic
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More