Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
Trong lập trình Flutter, khá thường xuyên bạn phải tạo các trang (màn hình) gần tương tự nhau. Chẳng hạn một ứng dụng với 3 trang ProfileScreen, SecurityScreen và UserSettingsScreen dưới đây:
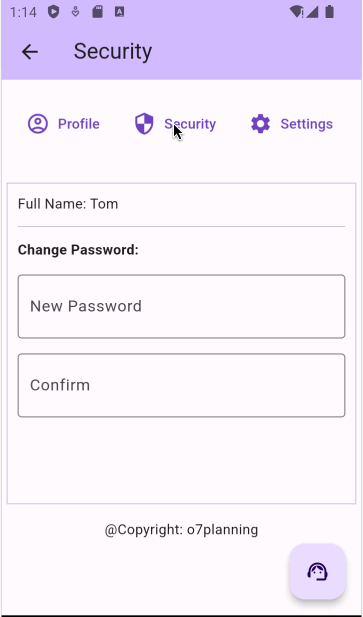
Các vùng được đánh dấu bởi màu đỏ là sự khác biệt giữa 3 trang (màn hình) nói trên.
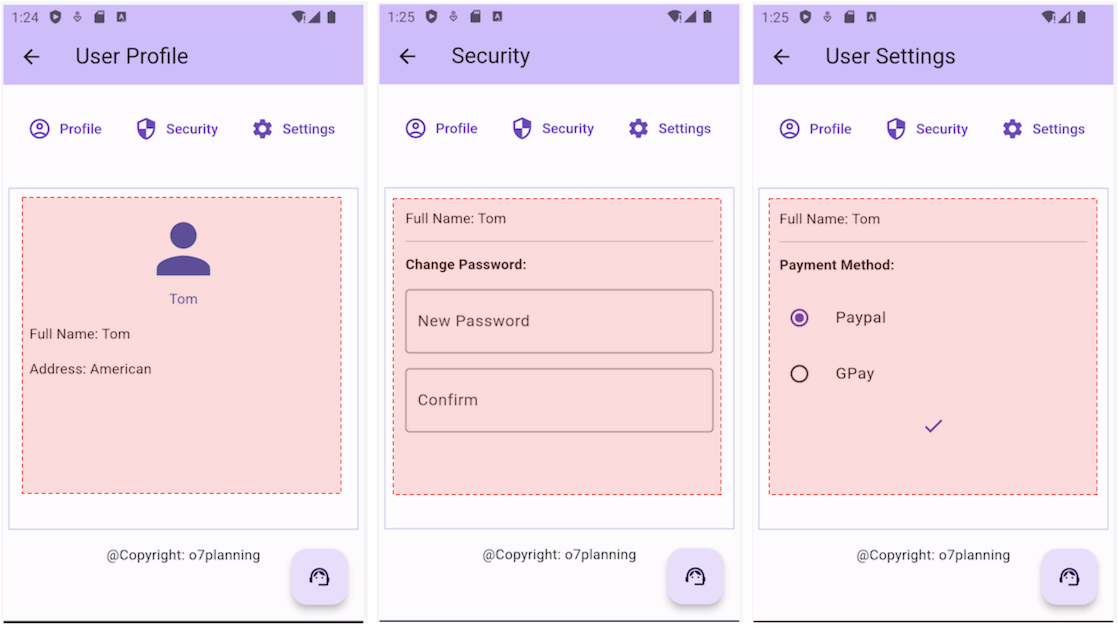
Trong bài viết này chúng ta sẽ thảo luận cách sử dụng lớp trừu tượng để thiết kế một giao diện người dùng cơ sở bao gồm các phần giống nhau của tất cả các trang nói trên. Phần khác biệt sẽ được thiết kế tại các lớp con.
1. BaseScreen
BaseScreen là một lớp trừu tượng tạo ra giao diện người dùng cơ sở. Phương thức trừu tượng buildMainContent() của nó sẽ tạo ra phần giao diện được đặt tại vùng màu đỏ.
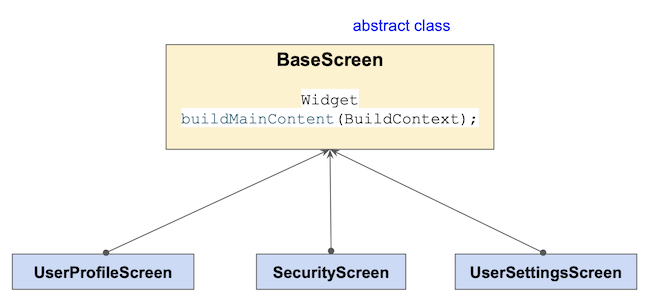
Phương thức trừu tượng buildMainContent() sẽ được triển khai tại các lớp con của BaseScreen.
Widget buildMainContent(BuildContext context);
Phương thức buildMainContent() sẽ tạo ra giao diện tại vùng màu đỏ.
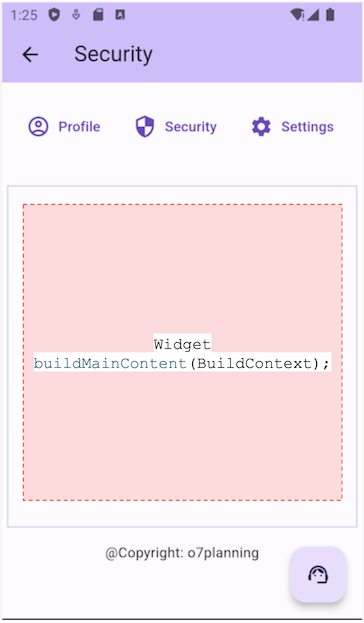
_base_screen.dart
import 'package:flutter/material.dart';
import '_top_menu_section.dart';
abstract class BaseScreen extends StatelessWidget {
const BaseScreen({super.key, required this.title});
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(title),
),
body: _buildBody(context),
floatingActionButton: FloatingActionButton(
onPressed: () {},
tooltip: 'Support',
child: const Icon(Icons.support_agent),
),
);
}
Widget _buildBody(BuildContext context) {
return SingleChildScrollView(
child: Column(
children: [
const TopMenuSection(),
const SizedBox(height: 10),
//
Container(
margin: const EdgeInsets.all(5),
padding: const EdgeInsets.all(20),
decoration: BoxDecoration(
border: Border.all(
color: Colors.indigo.withAlpha(80),
),
),
child: ConstrainedBox(
constraints: const BoxConstraints(minHeight: 300),
child: buildMainContent(context), // Main Content (***) <=====
),
),
//
const SizedBox(height: 10),
const Center(child: Text('@Copyright: o7planning')),
],
),
);
}
// Abstract method to create Main Content Widget. (***) <=====
Widget buildMainContent(BuildContext context);
}
Lớp TopMenuSection tạo ra một menu với 3 button.

_top_menu_section.dart
import 'package:flutter/material.dart';
class TopMenuSection extends StatelessWidget {
const TopMenuSection({super.key});
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.symmetric(vertical: 20, horizontal: 10),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
TextButton.icon(
onPressed: () {
Navigator.of(context).pushNamed('/profile');
},
icon: Icon(Icons.account_circle_outlined),
label: Text("Profile"),
),
TextButton.icon(
onPressed: () {
Navigator.of(context).pushNamed('/security');
},
icon: Icon(Icons.security),
label: Text("Security"),
),
TextButton.icon(
onPressed: () {
Navigator.of(context).pushNamed('/userSettings');
},
icon: Icon(Icons.settings),
label: Text("Settings"),
),
],
),
);
}
}
2. ProfileScreen
ProfileScreen là một lớp con của BaseScreen, nó sẽ triển khai phương thức buildMainContent().
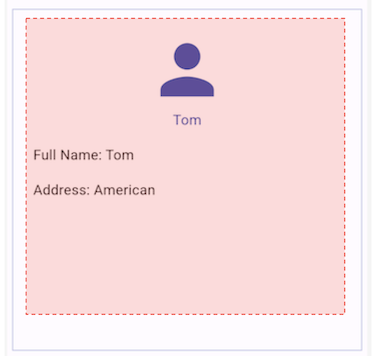
profile_screen.dart
import 'package:flutter/material.dart';
import '_base_screen.dart';
class ProfileScreen extends BaseScreen {
const ProfileScreen({super.key, required super.title});
@override
Widget buildMainContent(BuildContext context) {
return const Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Center(
child: Column(
children: [
Icon(Icons.person, size: 80, color: Colors.indigo),
Text(
'Tom',
style: TextStyle(color: Colors.indigo),
),
],
),
),
SizedBox(height: 15),
Text('Full Name: Tom'),
SizedBox(height: 15),
Text('Address: American'),
],
);
}
}
3. SecurityScreen
SecurityScreen là một lớp con của BaseScreen, nó sẽ triển khai phương thức buildMainContent().
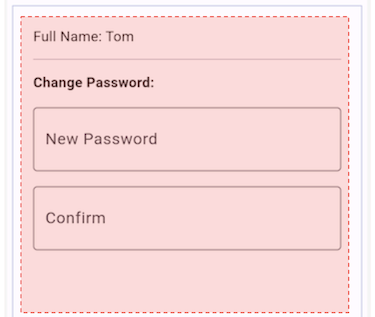
security_screen.dart
import 'package:flutter/material.dart';
import '_base_screen.dart';
class SecurityScreen extends BaseScreen {
const SecurityScreen({super.key, required super.title});
@override
Widget buildMainContent(BuildContext context) {
return const Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('Full Name: Tom'),
SizedBox(height: 5),
Divider(),
SizedBox(height: 5),
Text('Change Password:', style: TextStyle(fontWeight: FontWeight.bold)),
SizedBox(height: 15),
TextField(
obscureText: true,
decoration: InputDecoration(
label: Text('New Password'),
border: OutlineInputBorder(),
),
),
SizedBox(height: 15),
TextField(
obscureText: true,
decoration: InputDecoration(
label: Text('Confirm'),
border: OutlineInputBorder(),
),
),
],
);
}
}
4. UserSettingsScreen
UserSettingsScreen là một lớp con của BaseScreen, nó sẽ triển khai phương thức buildMainContent().
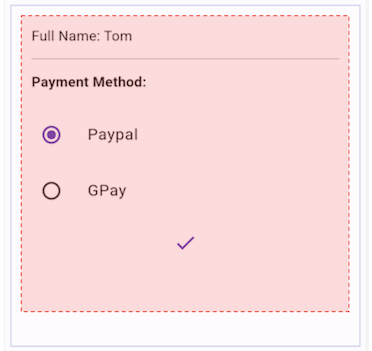
user_settings_screent.dart
import 'package:flutter/material.dart';
import '_base_screen.dart';
class UserSettingsScreen extends BaseScreen {
const UserSettingsScreen({super.key, required super.title});
@override
Widget buildMainContent(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('Full Name: Tom'),
SizedBox(height: 5),
Divider(),
SizedBox(height: 5),
Text('Payment Method:', style: TextStyle(fontWeight: FontWeight.bold)),
SizedBox(height: 15),
Column(
mainAxisSize: MainAxisSize.min,
children: [
RadioListTile<String>(
contentPadding: EdgeInsets.zero,
title: Text('Paypal'),
value: 'paypal',
groupValue: 'paypal',
onChanged: (String? value) {},
),
RadioListTile<String>(
contentPadding: EdgeInsets.zero,
title: Text('GPay'),
value: 'gpay',
groupValue: 'paypal',
onChanged: (String? value) {},
),
TextButton(onPressed: () {}, child: Icon(Icons.check)),
],
)
],
);
}
}
5. MainApp
main.dart
import 'package:flutter/material.dart';
import 'user_settings_screen.dart';
import 'security_screen.dart';
import 'profile_screen.dart';
void main() {
runApp(const MyApp());
}
var routeMap = {
"/profile": (BuildContext context) => ProfileScreen(title: 'User Profile'),
"/security": (BuildContext context) => SecurityScreen(title: 'Security'),
"/userSettings": (BuildContext context) =>
UserSettingsScreen(title: 'User Settings'),
};
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
routes: routeMap,
initialRoute: "/profile",
);
}
}
Các hướng dẫn lập trình Flutter
- Hướng dẫn và ví dụ Flutter Column
- Hướng dẫn và ví dụ Flutter Stack
- Hướng dẫn và ví dụ Flutter IndexedStack
- Hướng dẫn và ví dụ Flutter Spacer
- Hướng dẫn và ví dụ Flutter Expanded
- Hướng dẫn và ví dụ Flutter SizedBox
- Hướng dẫn và ví dụ Flutter Tween
- Cài đặt Flutter SDK trên Windows
- Cài đặt Flutter Plugin cho Android Studio
- Tạo ứng dụng Flutter đầu tiên của bạn - Hello Flutter
- Hướng dẫn và ví dụ Flutter Scaffold
- Hướng dẫn và ví dụ Flutter AppBar
- Hướng dẫn và ví dụ Flutter BottomAppBar
- Hướng dẫn và ví dụ Flutter SliverAppBar
- Hướng dẫn và ví dụ Flutter TextButton
- Hướng dẫn và ví dụ Flutter ElevatedButton
- Hướng dẫn và ví dụ Flutter ShapeBorder
- Hướng dẫn và ví dụ Flutter EdgeInsetsGeometry
- Hướng dẫn và ví dụ Flutter EdgeInsets
- Hướng dẫn và ví dụ Flutter CircularProgressIndicator
- Hướng dẫn và ví dụ Flutter LinearProgressIndicator
- Hướng dẫn và ví dụ Flutter Center
- Hướng dẫn và ví dụ Flutter Align
- Hướng dẫn và ví dụ Flutter Row
- Hướng dẫn và ví dụ Flutter SplashScreen
- Hướng dẫn và ví dụ Flutter Alignment
- Hướng dẫn và ví dụ Flutter Positioned
- Hướng dẫn và ví dụ Flutter ListTile
- Hướng dẫn và ví dụ Flutter SimpleDialog
- Hướng dẫn và ví dụ Flutter AlertDialog
- Navigation và Routing trong Flutter
- Hướng dẫn và ví dụ Flutter Navigator
- Hướng dẫn và ví dụ Flutter TabBar
- Hướng dẫn và ví dụ Flutter Banner
- Hướng dẫn và ví dụ Flutter BottomNavigationBar
- Hướng dẫn và ví dụ Flutter FancyBottomNavigation
- Hướng dẫn và ví dụ Flutter Card
- Hướng dẫn và ví dụ Flutter Border
- Hướng dẫn và ví dụ Flutter ContinuousRectangleBorder
- Hướng dẫn và ví dụ Flutter RoundedRectangleBorder
- Hướng dẫn và ví dụ Flutter CircleBorder
- Hướng dẫn và ví dụ Flutter StadiumBorder
- Hướng dẫn và ví dụ Flutter Container
- Hướng dẫn và ví dụ Flutter RotatedBox
- Hướng dẫn và ví dụ Flutter CircleAvatar
- Hướng dẫn và ví dụ Flutter TextField
- Hướng dẫn và ví dụ Flutter IconButton
- Hướng dẫn và ví dụ Flutter FlatButton
- Hướng dẫn và ví dụ Flutter SnackBar
- Hướng dẫn và ví dụ Flutter Drawer
- Ví dụ Flutter Navigator pushNamedAndRemoveUntil
- Hiển thị hình ảnh trên Internet trong Flutter
- Hiển thị ảnh Asset trong Flutter
- Flutter TextInputType các kiểu bàn phím
- Hướng dẫn và ví dụ Flutter NumberTextInputFormatter
- Hướng dẫn và ví dụ Flutter Builder
- Làm sao xác định chiều rộng của Widget cha trong Flutter
- Bài thực hành Flutter thiết kế giao diện màn hình đăng nhập
- Bài thực hành Flutter thiết kế giao diện trang (1)
- Khuôn mẫu thiết kế Flutter với các lớp trừu tượng
- Bài thực hành Flutter thiết kế trang Profile với Stack
- Bài thực hành Flutter thiết kế trang profile (2)
- Hướng dẫn và ví dụ Flutter ListView
- Hướng dẫn và ví dụ Flutter GridView
- Bài thực hành Flutter với gói http và dart:convert (2)
- Bài thực hành Flutter với gói http và dart:convert (1)
- Ứng dụng Flutter Responsive với Menu Drawer
- Flutter GridView với SliverGridDelegate tuỳ biến
- Hướng dẫn và ví dụ Flutter image_picker
- Flutter upload ảnh sử dụng http và ImagePicker
- Hướng dẫn và ví dụ Flutter SharedPreferences
- Chỉ định cổng cố định cho Flutter Web trên Android Studio
- Tạo Module trong Flutter
- Hướng dẫn và ví dụ Flutter SkeletonLoader
- Hướng dẫn và ví dụ Flutter Slider
- Hướng dẫn và ví dụ Flutter Radio
- Bài thực hành Flutter SharedPreferences
- Hướng dẫn và ví dụ Flutter InkWell
- Hướng dẫn và ví dụ Flutter GetX GetBuilder
- Hướng dẫn và ví dụ Flutter GetX obs Obx
- Hướng dẫn và ví dụ Flutter flutter_form_builder
- Xử lý lỗi 404 trong Flutter GetX
- Ví dụ đăng nhập và đăng xuất với Flutter Getx
- Hướng dẫn và ví dụ Flutter multi_dropdown
Show More