Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
1. Giới thiệu
Tài liệu được viết dựa trên:
Android Studio 3.6.1
Bạn đang xem loạt bài hướng dẫn lập trình Android, đây là bài hướng dẫn thứ 2, trong tài liệu này tôi sẽ hướng dẫn bạn từng bước xây dựng một ứng dụng Android, các kiến thức cơ bản sẽ được đề cập bao gồm:
- Gọi Activity từ một Activity khác.
- Xử lý sự kiện cơ bản.
- Xây dựng giao diện cơ bản, và làm việc với nguồn dữ liệu.
Trước khi bắt đầu với tài liệu này, hãy đảm bảo rằng bạn đã chạy thành công ví dụ "Hello Android" và hiểu về cấu trúc một dự án Android. Bạn có thể xem tại:
2. Tạo project Android
Nếu bạn đang làm việc với một Android project trên Android Studio, hãy đóng project này lại, chúng ta sẽ tạo mới một project khác.
Trên Android Studio chọn:
- File/Close Project
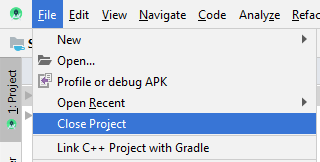
Tạo mới một project:
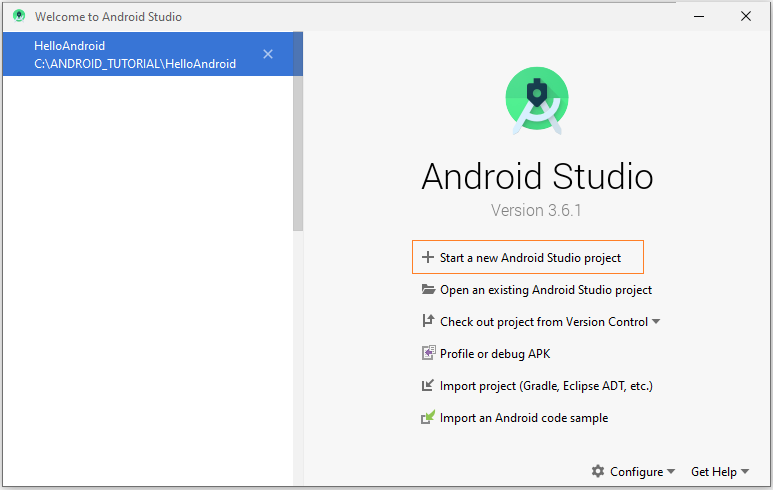
Tiếp theo Wizard sẽ hỏi bạn có muốn tạo một Activity nào không, chọn "Add No Activity", wizard sẽ sẽ chỉ tạo một project rỗng, không bao gồm một Activity nào cả.
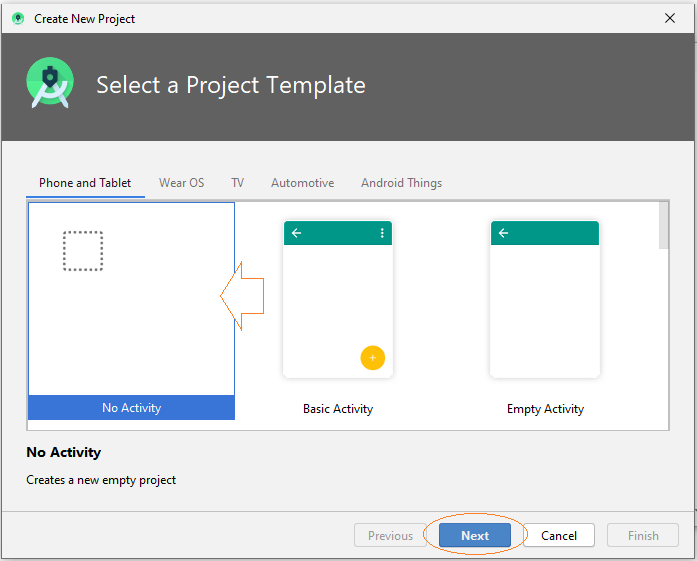
Nhập vào:
- Name: AndroidBasic2
- Package name: org.o7planning.androidbasic2
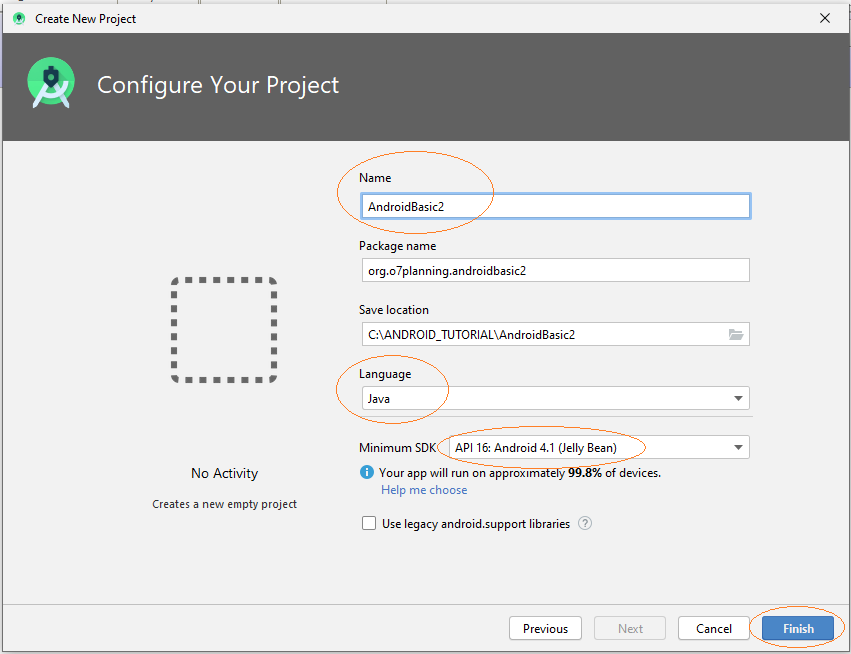
Ứng dụng đang tạo sẽ được sử dụng cho Phone và Tablet.
Chú ý: API 16, Android 4.1 hiện tại đang được sử dụng trên hầu hết các thiết bị Phone và Table (Khoảng 94%).
Project của bạn đã được tạo ra.
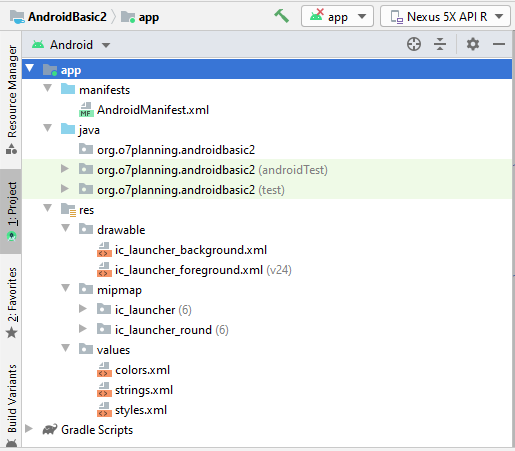
3. Tạo MainActivity và các Activity con
Chúng ta sẽ tạo một Activity chính (MainActivity), Activity này sẽ được gọi khi ứng dụng được chạy. Trên MainActivity sẽ có các button gọi tới các Activity khác.
Trên Android Studio chọn:
- File/New/Activity/Empty Activity
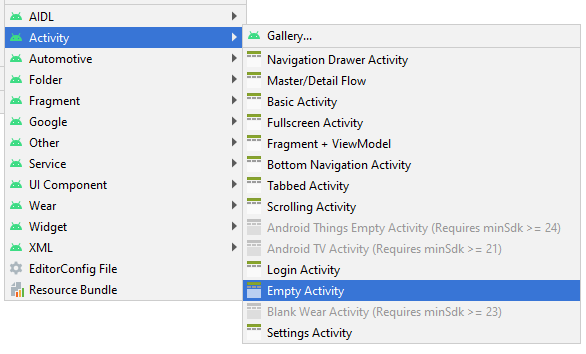
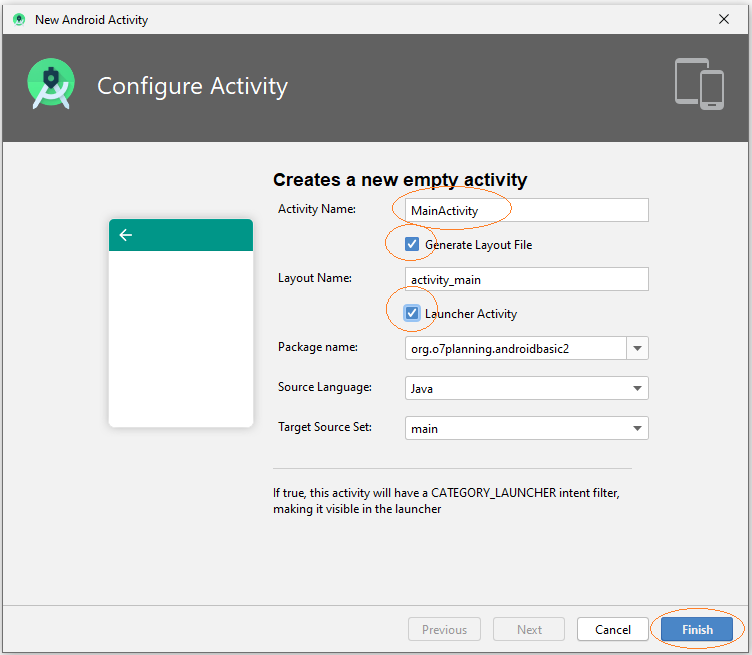
MainActivity đã được tạo ra, gồm 2 file MainActivity.java và main_activity.xml, thông tin của Activity này cũng đã được đăng ký với AndroidManifest.xml.
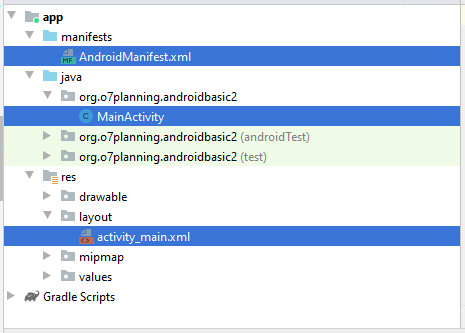
Tương tự như vậy chúng ta tạo thêm 5 Activity khác.
- Example1Activity
- Example2Activity
- Example3Activity
- Example4Activity
- Example5Activity
Trên Android Studio chọn:
- File/New/Activity/Empty Activity
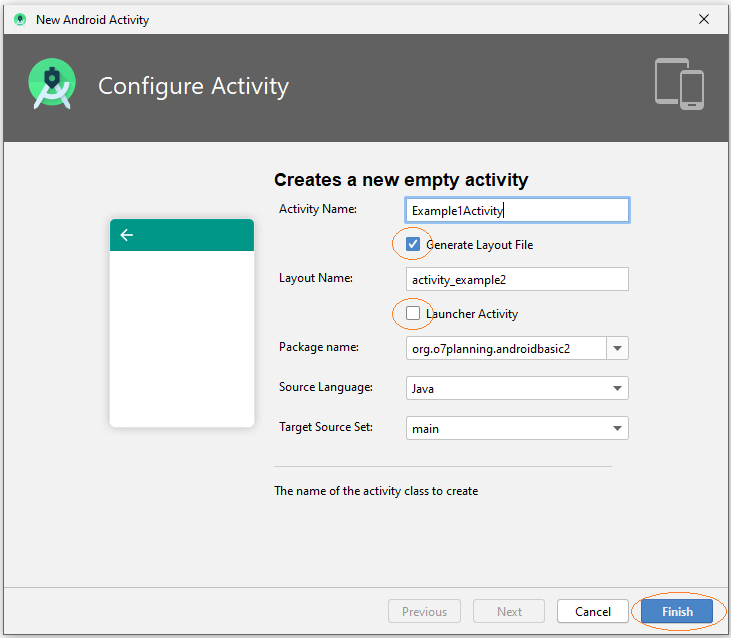
Chú ý: Tất cả các Activity vừa tạo mới không phải là một Activity chính, nó được gọi từ MainActivity, vì vậy bạn không nên check vào "Launcher Activity".
OK, 5 Activity mới đã được tạo ra, và chúng đã được đăng ký với AndroidManifest.xml.
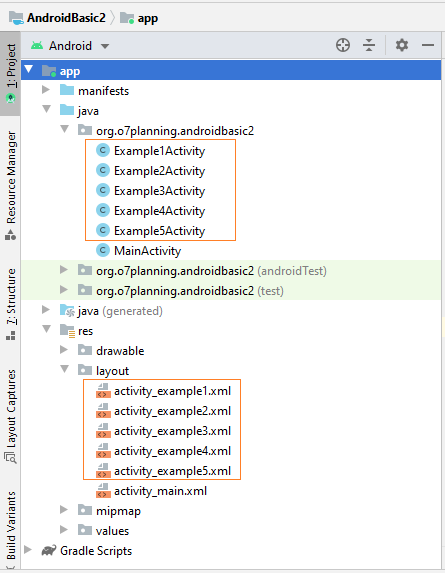
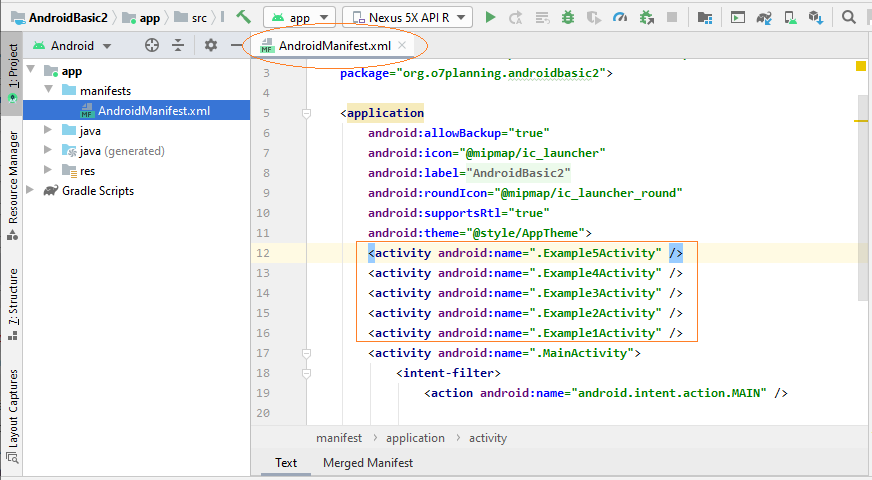
4. Thiết kế giao diện main_activity.xml
Trên Android Studio mở main_activity.xml để thiết kế giao diện cho nó.
Cửa sổ thiết kế có 3 chế độ (mode):
- Code
- Split
- Design
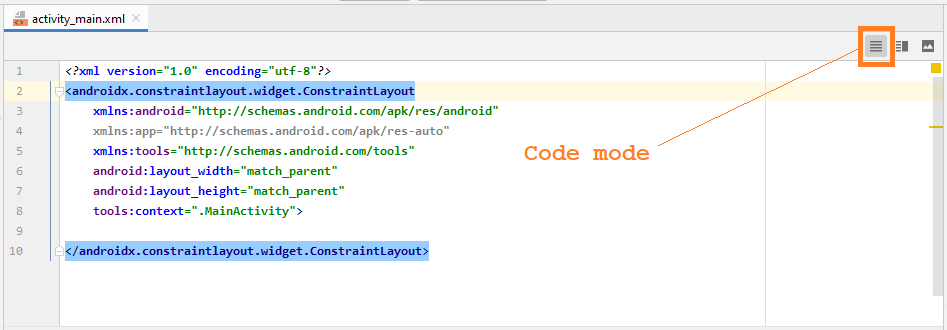
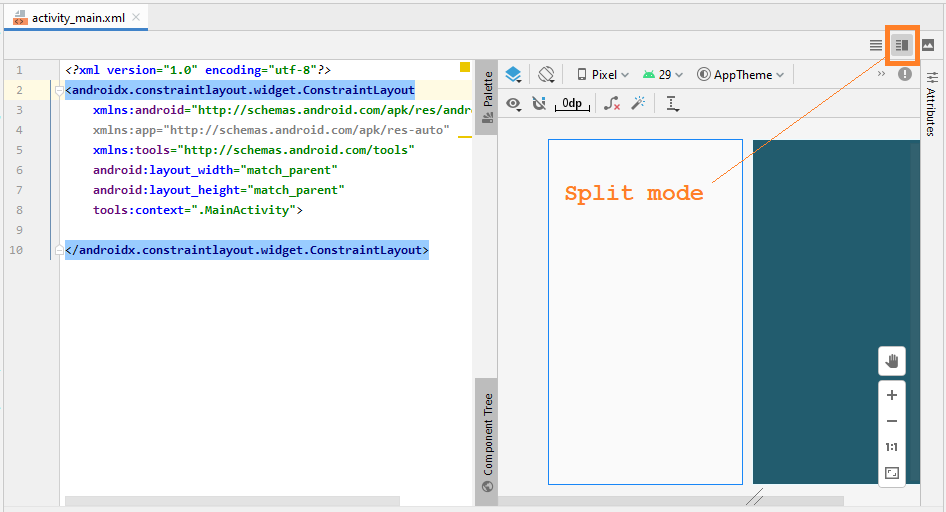
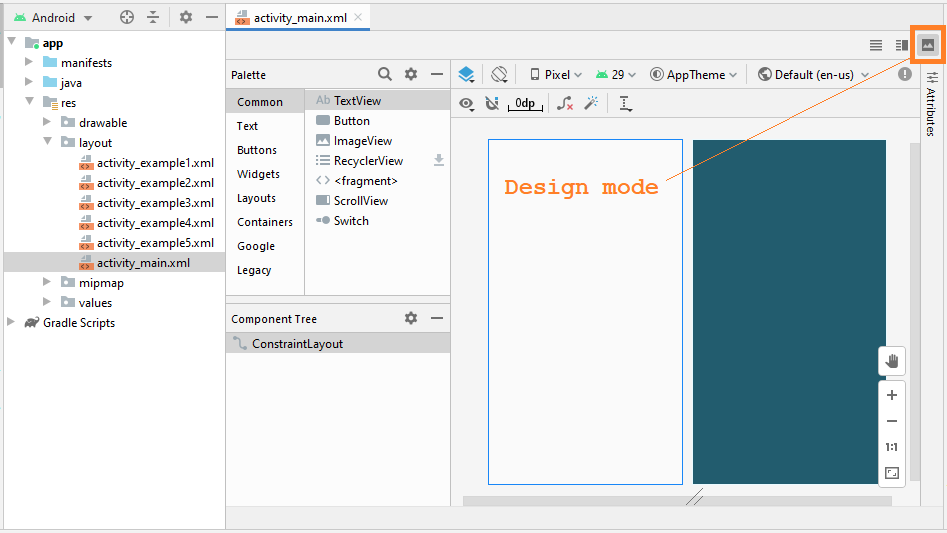
Chủ yếu bạn làm việc tại chế độ Design (Design mode), nó giúp bạn kéo thả các thành phần vào trong giao diện và tự động sinh ra (generate) mã XML:
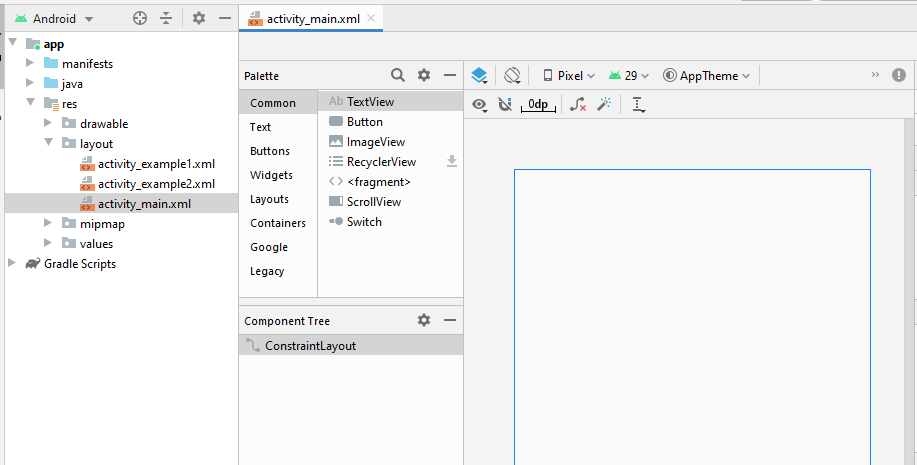
OK, chúng ta sẽ thiết kế một giao diện đơn giản, bao gồm 5 Button:
Kéo thả 5 Button vào giao diện:
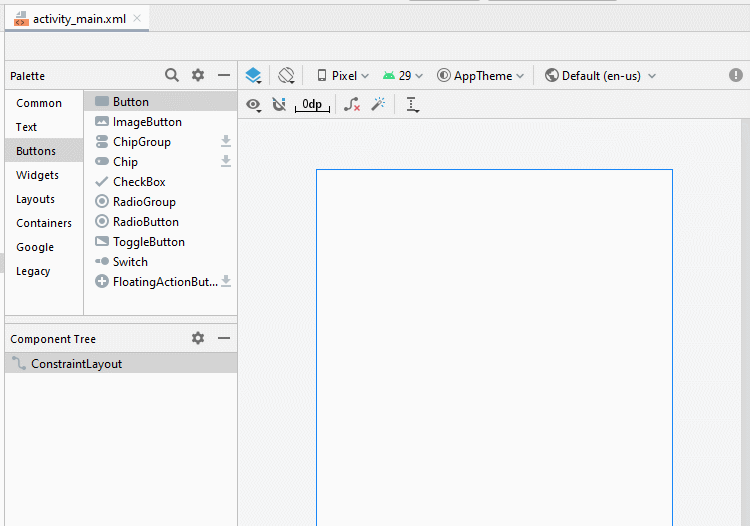
Sét đặt các giàng buộc (constraint) cho các Button.
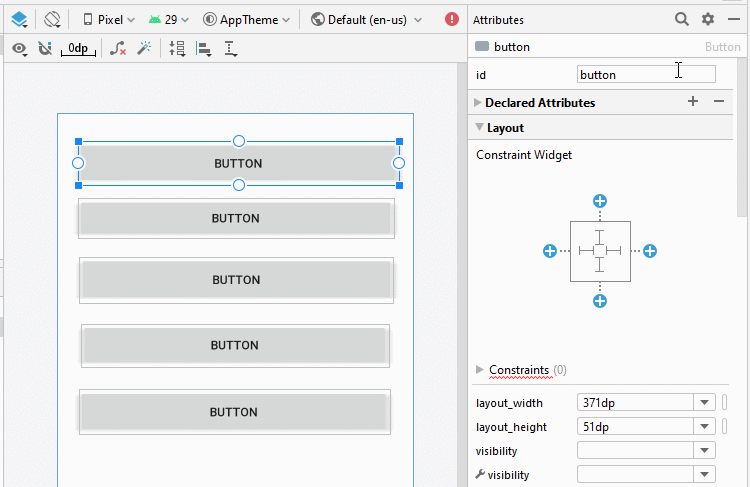
Sét đặt ID, Text cho các Button trên giao diện. ID rất quan trọng, trong mã Java bạn có thể truy cập vào một Button thông qua ID của nó.
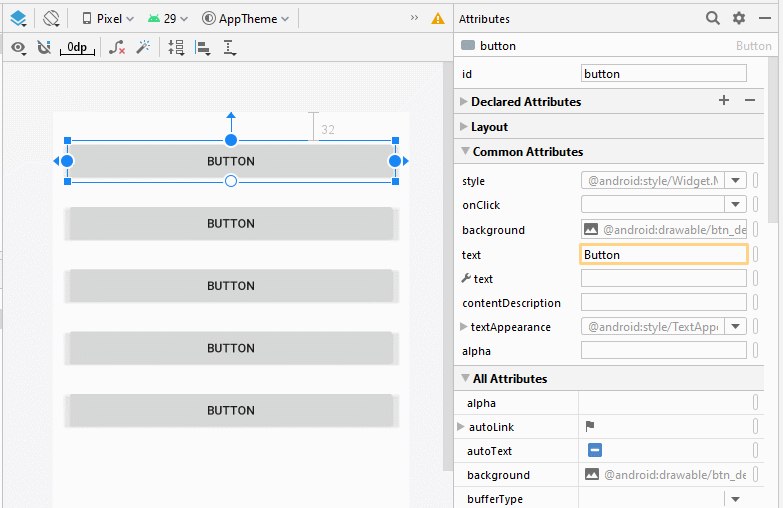
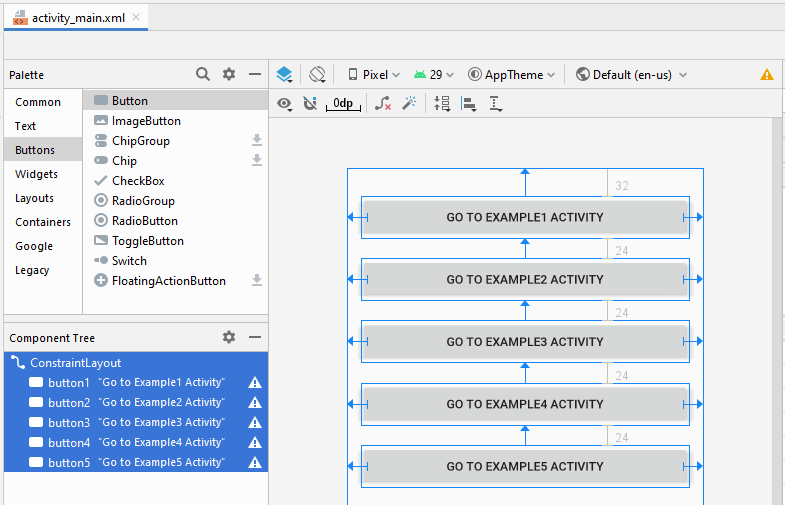
Trên cửa sổ thiết kế, chuyển sang chế độ Code (Code mode), bạn sẽ nhìn thấy mã XML được sinh ra (generate).
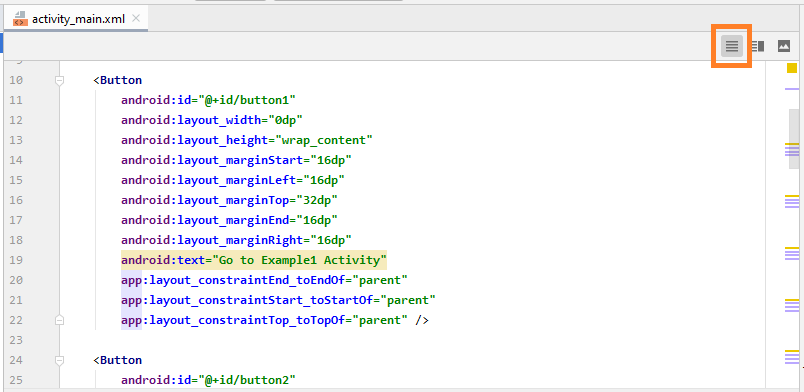
Dưới đây là nội dung tập tin activity_main.xml của tôi, bạn có thể copy và paste vào cửa sổ Code của bạn để có được một giao diện tương tự.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Go to Example1 Activity"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Go to Example2 Activity"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button1" />
<Button
android:id="@+id/button3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Go to Example3 Activity"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button2" />
<Button
android:id="@+id/button4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Go to Example4 Activity"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button3" />
<Button
android:id="@+id/button5"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Go to Example5 Activity"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button4" />
</androidx.constraintlayout.widget.ConstraintLayout>
5. Gọi một Activity từ một Activity
Ở đây chúng ta sẽ xử lý các sự kiện khi người dùng nhấn vào các Button, chúng sẽ gọi đến các Example1Activity, .. Example5Activity tương ứng.
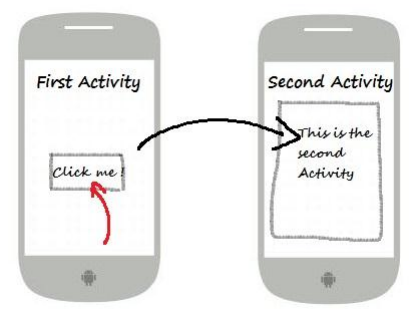
Các Activity nói chuyện với nhau thông qua một đối tượng Intent. Ví dụ Activity1 muốn gọi Activity2 chạy, nó đóng gói những gì cần nói, và lời yêu cầu vào một đối tượng Intent và gửi đối tượng Intent này tới Activity2. Bạn có thể xem hình minh họa dưới đây.
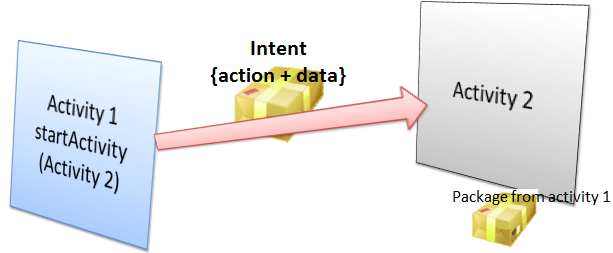
Mở lớp MainActivity, trên code Java bạn có thể truy cập tới các Button thông qua ID của chúng.
// Lấy ra button theo ID
Button button1 = (Button) this.findViewById(R.id.go_button1);
// Sét đặt sự kiện Click vào Button1.
button1.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Tạo một Intent:
// (Đối tượng chứa nội dung sẽ được gửi đến Example1Activity).
Intent myIntent = new Intent(MainActivity.this, Example1Activity.class);
// Các tham số gắn trên Intent (Không bắt buộc).
myIntent.putExtra("text1", "This is text1 sent from MainActivity at " + new Date());
myIntent.putExtra("text2", "This is text2 sent from MainActivity at " + new Date());
// Yêu cầu chạy Example1Activity.
MainActivity.this.startActivity(myIntent);
}
});
Code đầy đủ của MainActivity.java:
MainActivity.java
package org.o7planning.androidbasic2;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.Intent;
import android.view.View;
import android.widget.Button;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
private Button button1;
private Button button2;
private Button button3;
private Button button4;
private Button button5;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Find Button by its ID
this.button1 = (Button) this.findViewById(R.id.button1);
// Find button by its ID
this.button2 = (Button) this.findViewById(R.id.button2);
// Find button by its ID.
this.button3 = (Button) this.findViewById(R.id.button3);
// Find button by its ID.
this.button4 = (Button) this.findViewById(R.id.button4);
// Find button by its ID.
this.button5 = (Button) this.findViewById(R.id.button5);
// Called when the user clicks the button1.
button1.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Create a Intent:
// (This object contains content that will be sent to Example1Activity).
Intent myIntent = new Intent(MainActivity.this, Example1Activity.class);
// Put parameters
myIntent.putExtra("text1", "This is text1 sent from MainActivity at " + new Date());
myIntent.putExtra("text2", "This is text2 sent from MainActivity at " + new Date());
// Start Example1Activity.
MainActivity.this.startActivity(myIntent);
}
});
// Called when the user clicks the button2.
button2.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Create a Intent:
// (This object contains content that will be sent to Example2Activity).
Intent myIntent = new Intent(MainActivity.this, Example2Activity.class);
// Start Example2Activity.
MainActivity.this.startActivity(myIntent);
}
});
// Called when the user clicks the button3.
button3.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Create a Intent:
// (This object contains content that will be sent to Example3Activity).
Intent myIntent = new Intent(MainActivity.this, Example3Activity.class);
MainActivity.this.startActivity(myIntent);
}
});
button4.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Create a Intent:
// (This object contains content that will be sent to Example4Activity).
Intent myIntent = new Intent(MainActivity.this, Example4Activity.class);
// Start Example4Activity.
MainActivity.this.startActivity(myIntent);
}
});
button5.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Create a Intent:
// (This object contains content that will be sent to Example5Activity).
Intent myIntent = new Intent(MainActivity.this, Example5Activity.class);
// Start Example5Activity.
MainActivity.this.startActivity(myIntent);
}
});
}
}
6. Example1Activity - Gọi một Activity khác
Tiếp theo mở activity_example1.xml chúng ta sẽ thiết kế giao diện cho Example1Activity
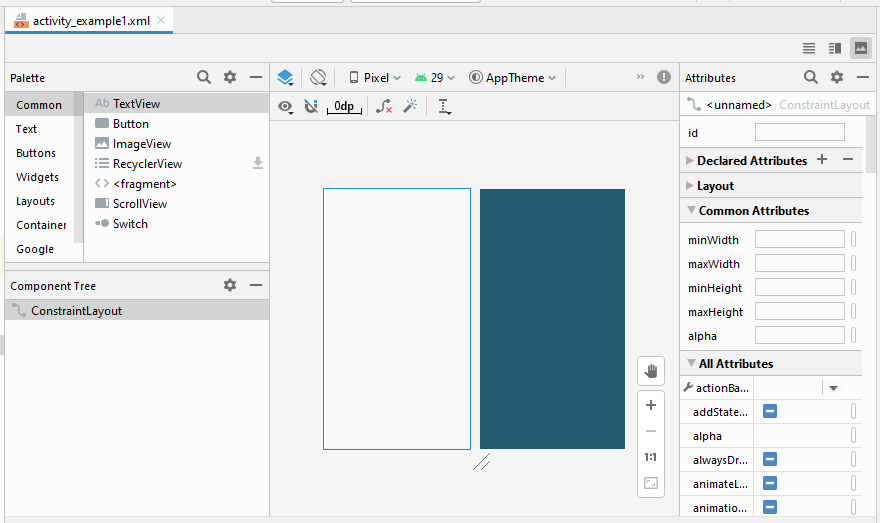
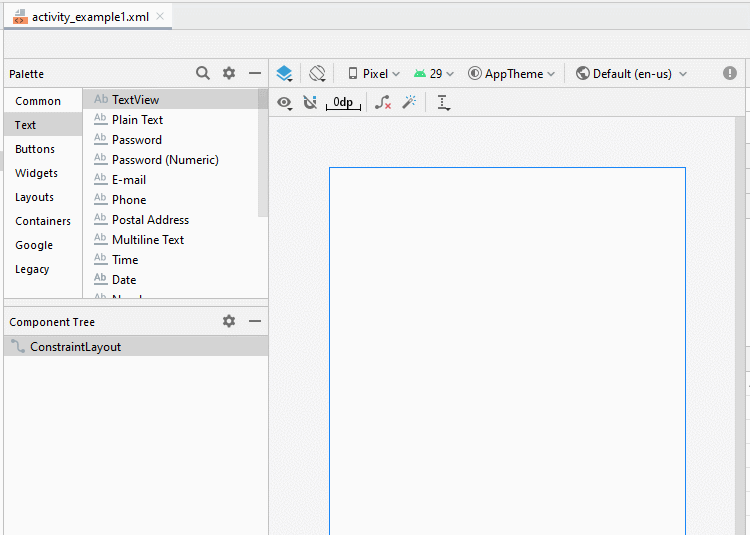
Sét đặt các giàng buộc (constraint) cho các thành phần trên giao diện.
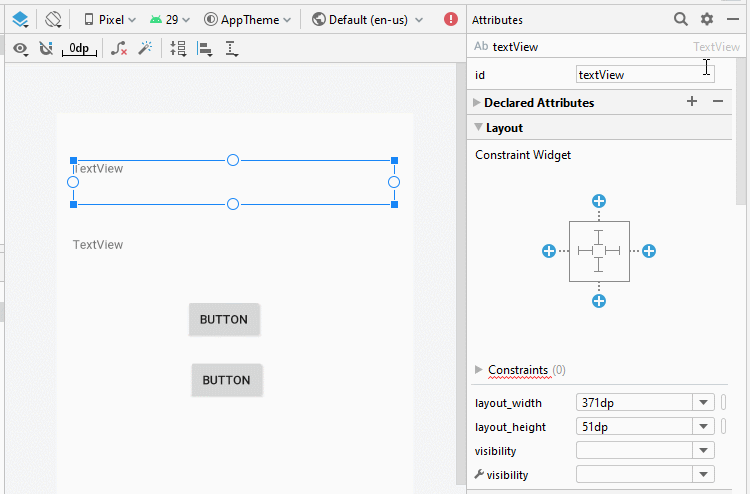
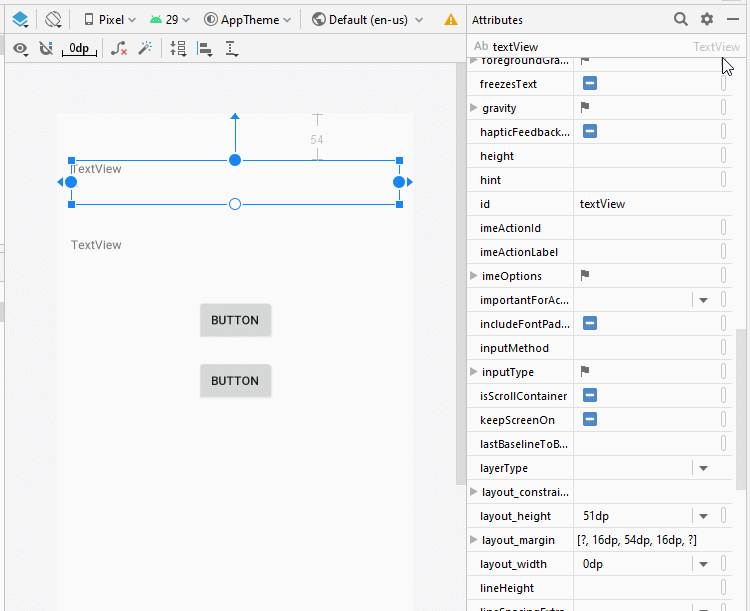
Sét đặt ID, Text cho các thành phần trên giao diện:
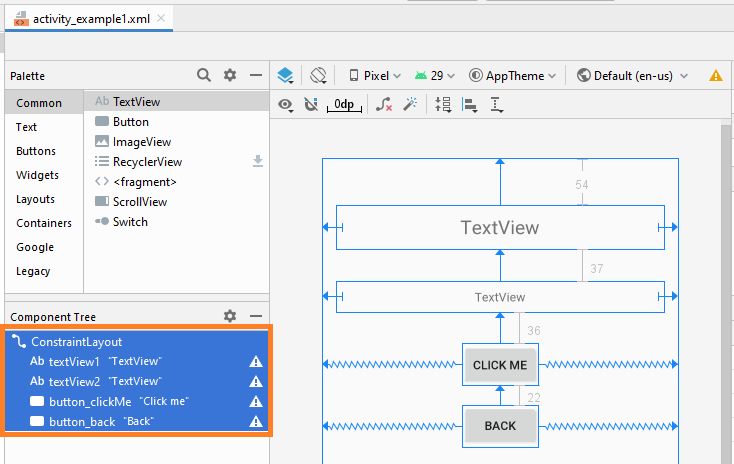
activity_example1.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Example1Activity">
<TextView
android:id="@+id/textView1"
android:layout_width="0dp"
android:layout_height="51dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="54dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:gravity="center"
android:text="TextView"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="36dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="37dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:gravity="center"
android:text="TextView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView1" />
<Button
android:id="@+id/button_clickMe"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="36dp"
android:text="Click me"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<Button
android:id="@+id/button_back"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="22dp"
android:text="Back"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_clickMe" />
</androidx.constraintlayout.widget.ConstraintLayout>
Example1Activity.java
package org.o7planning.androidbasic2;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.content.Intent;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class Example1Activity extends AppCompatActivity {
private Button buttonClickMe;
private Button buttonBack;
private TextView textView1;
private TextView textView2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_example1);
// Find TextView by its ID
this.textView1 = (TextView)this.findViewById(R.id.textView1);
// Find TextView by its ID
this.textView2 = (TextView)this.findViewById(R.id.textView2);
this.buttonClickMe = (Button)this.findViewById(R.id.button_clickMe);
this.buttonBack = (Button)this.findViewById(R.id.button_back);
// Get the intent sent from MainActivity.
Intent intent = getIntent();
// Parameter in Intent, sent from MainActivity
String value1 = intent.getStringExtra("text1");
// Parameter in Intent, sent from MainActivity
String value2 = intent.getStringExtra("text2");
this.textView1.setText(value1);
this.textView2.setText(value2);
// When user click "Click me" button.
this.buttonClickMe.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
textView2.setText("You click button");
}
});
// When user long click "Click me" button.
this.buttonClickMe.setOnLongClickListener(new Button.OnLongClickListener() {
// return true if the callback consumed the long click, false otherwise.
@Override
public boolean onLongClick(View v) {
textView2.setText("You long click button");
return true;
}
});
// When user click "Back" button.
this.buttonBack.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// Back to previous Activity.
Example1Activity.this.finish();
}
});
}
}
Chạy ví dụ:
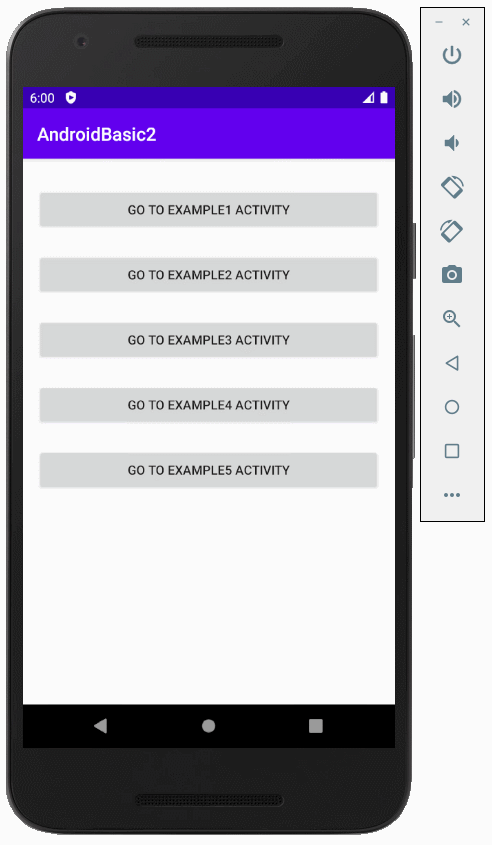
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More