Hướng dẫn và ví dụ Android ImageButton
1. Android ImageButton
Trong Android, ImageButton là một điều khiển giao diện người dùng (User interface control), nó hiển thị như một button với hình ảnh, và cho phép người dùng nhấp (click) vào để thực hiện một hành động.
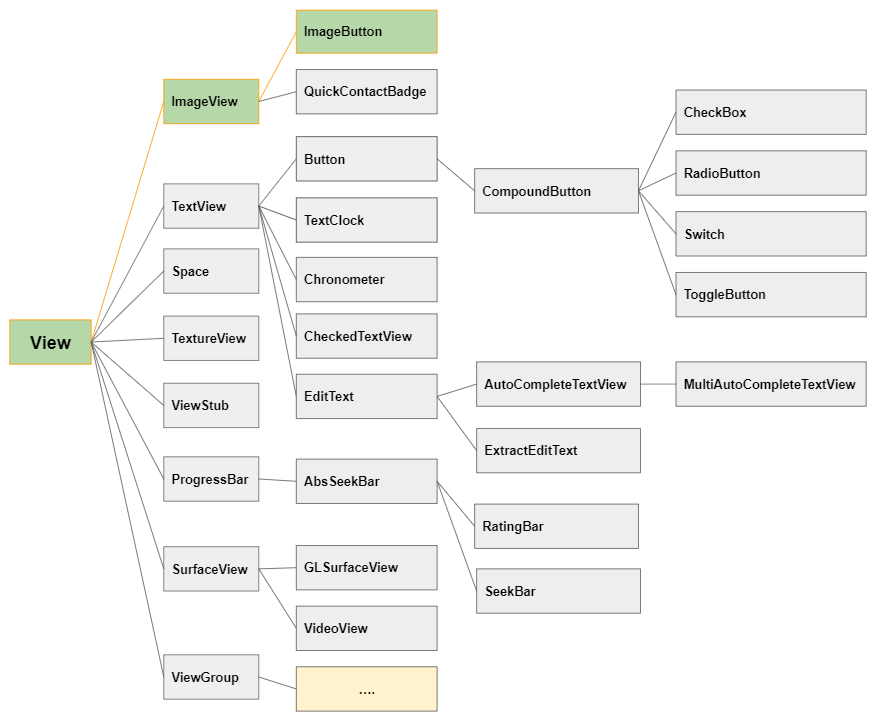
ImageButton là một lớp con của ImageView, vì vậy bạn có thể sét đặt Icon cho nó thông qua thuộc tính app:srcCompat. Chú ý, khác với Button, bạn không thể hiển thị một văn bản trên ImageButton.
<ImageButton
android:id="@+id/imageButton"
app:srcCompat="@drawable/icon_feel_good"
... />
Có một vài loại button khác mà bạn có thể tham khảo thêm trong các bài viết dưới đây:
Với mã Java, có một vài phương thức giúp bạn sét đặt Icon cho ImageButton, tất cả các phương thức này đều được thừa kế từ ImageView.
- setImageBitmap(Bitmap bitmap)
- setImageDrawable(Drawable drawable)
- setImageIcon(Icon icon)
- setImageResource(int resId)
- setImageURI(Uri uri)
2. ImageButton Styles
Thuộc tính style là một tùy chọn của ImageButton, nó cho phép bạn sét đặt kiểu dáng cho ImageButton. Có một vài kiểu dáng sẵn có trong thư viện của Android mà bạn có thể sẵn sàng sử dụng.
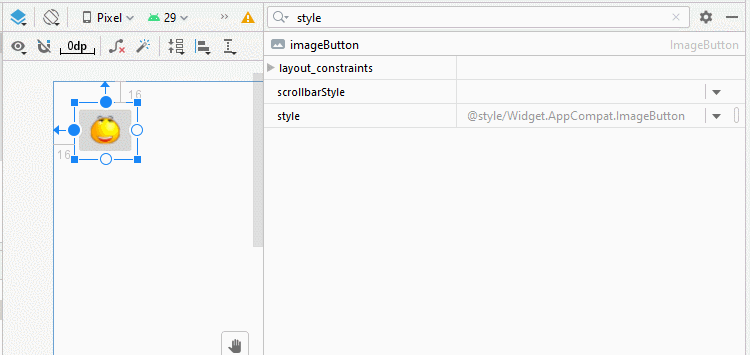
<ImageButton
android:id="@+id/imageButton"
style="@android:style/Widget.Holo.ImageButton"
app:srcCompat="@drawable/icon_feel_good"
... />
- style="@style/Widget.AppCompat.ImageButton"
- style="@android:style/Widget.Holo.ImageButton"
- style="@android:style/Widget.ImageButton"
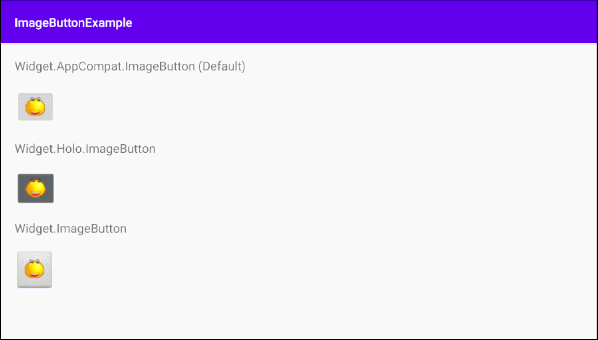
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView11"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.AppCompat.ImageButton (Default)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageButton
android:id="@+id/imageButton11"
style="@style/Widget.AppCompat.ImageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView11"
app:srcCompat="@drawable/icon_feel_good" />
<TextView
android:id="@+id/textView12"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.ImageButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageButton11" />
<ImageButton
android:id="@+id/imageButton12"
style="@android:style/Widget.Holo.ImageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView12"
app:srcCompat="@drawable/icon_feel_good" />
<TextView
android:id="@+id/textView13"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.ImageButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="1.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageButton12" />
<ImageButton
android:id="@+id/imageButton13"
style="@android:style/Widget.ImageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView13"
app:srcCompat="@drawable/icon_feel_good" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. ImageButton Event
Về cơ bản ImageButton và Button đều có các sự kiện giống nhau, bạn có thể tham khảo bài viết về Button để tìm hiểu về các sự kiện này.
Các sự kiện của ImageButton, Button:
- button.setOnTouchListener(View.OnTouchListener)
- button.setOnClickListener(View.OnClickListener)
- button.setOnLongClickListener(View.OnLongClickListener)
- button.setOnContextClickListener(View.OnContextClickListener);
- ...
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More