Hướng dẫn và ví dụ Android AlertDialog
1. Android AlertDialog
Android AlertDialog là một hộp thoại hiển thị một thông điệp, và hỗ trợ 1, 2 hoặc 3 button, nó giúp bạn dễ dàng tạo được một hộp thoại chỉ với một vài dòng code.
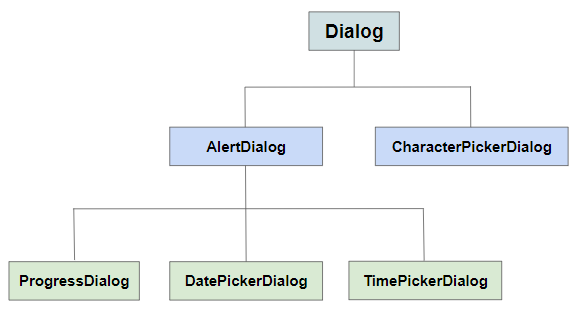
AlertDialog bao gồm 3 vùng:
- Vùng tiêu đề (Title area)
- Vùng nội dung (Content area)
- Vùng chứa các button (Buttons area)
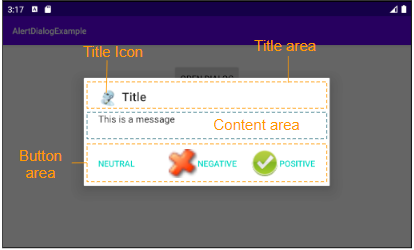
Landscape screen
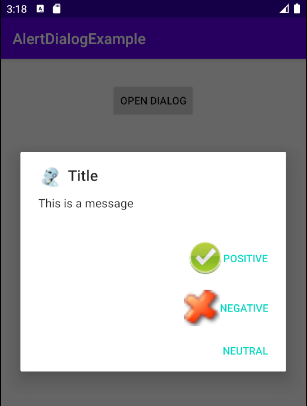
Portrait screen
Title area
Sử dụng phương thức setTitle(), setIcon() để sét đặt tiêu đề và icon cho hộp thoại, chúng sẽ được nhìn thấy trên Title area. Hoặc sử dụng phương thức setCustomTitle(View) nếu bạn muốn có một vùng tiêu đề (Title area) tùy biến.
Content area
Vùng nội dung (Content area) có thể hiển thị một thông điệp, một danh sách các lựa chọn,...
Buttons area
Vùng này có tối đa 3 button: Positive button, Negative button, Neutral button. Trong đó 2 button đầu tiên hỗ trợ Text & Icon, còn Neutral button chỉ hỗ trợ Text, tuy nhiên bạn có thể hiển thị Icon cho nó với một thủ thuật nhỏ (Xem thêm trong ví dụ).
2. Ví dụ AlertDialog (1)
Xem trước ví dụ:
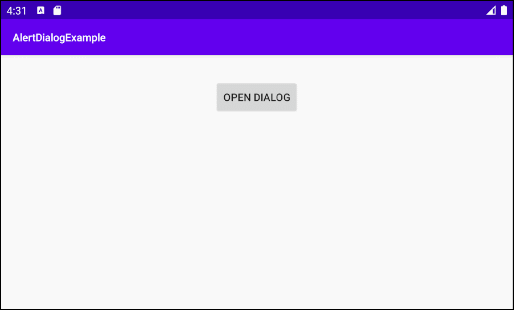
AlertButtonExample0.java
package org.o7planning.alertdialogexample;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogExample0 {
public static void showAlertDialog(final Context context) {
final Drawable positiveIcon = context.getResources().getDrawable(R.drawable.icon_positive);
final Drawable negativeIcon = context.getResources().getDrawable(R.drawable.icon_negative);
final Drawable neutralIcon = context.getResources().getDrawable(R.drawable.icon_neutral);
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Set Title and Message:
builder.setTitle("Title").setMessage("This is a message");
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Positive" button with OnClickListener.
builder.setPositiveButton("Positive", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose positive button",
Toast.LENGTH_SHORT).show();
}
});
builder.setPositiveButtonIcon(positiveIcon);
// Create "Negative" button with OnClickListener.
builder.setNegativeButton("Negative", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose positive button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
builder.setNegativeButtonIcon(negativeIcon);
// Create "Neutral" button with OnClickListener.
builder.setNeutralButton("Neutral", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Action for 'NO' Button
Toast.makeText(context,"You choose neutral button",
Toast.LENGTH_SHORT).show();
}
});
builder.setNeutralButtonIcon(neutralIcon); // Not working!!!
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
3. Ví dụ AlertDialog (2)
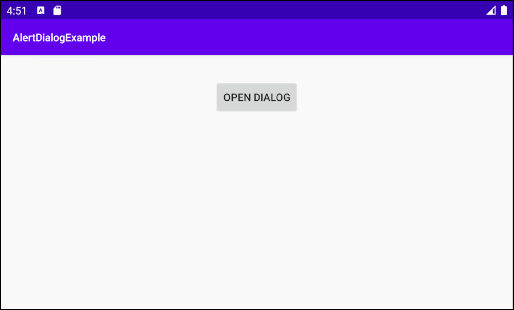
AlertDialogExample2.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogExample2 {
public static void showAlertDialog(final Context context) {
final Drawable positiveIcon = context.getResources().getDrawable(R.drawable.icon_positive);
final Drawable negativeIcon = context.getResources().getDrawable(R.drawable.icon_negative);
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Set Title and Message:
builder.setTitle("Confirmation").setMessage("Do you want to close this app?");
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Yes" button with OnClickListener.
builder.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose Yes button",
Toast.LENGTH_SHORT).show();
Activity activity = (Activity) context;
activity.finish();
}
});
builder.setPositiveButtonIcon(positiveIcon);
// Create "No" button with OnClickListener.
builder.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(context,"You choose No button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
builder.setNegativeButtonIcon(negativeIcon);
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
4. Ví dụ AlertDialog (List)
Ví dụ một AlertDialog với danh sách các lựa chọn cho người dùng.
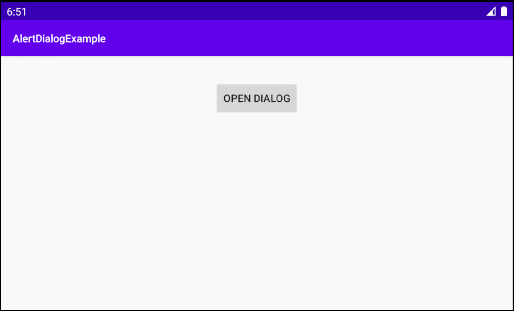
AlertDialogListExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogListExample {
public static void showAlertDialog(final Activity activity) {
final Drawable negativeIcon = activity.getResources().getDrawable(R.drawable.icon_negative);
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
// Set Title.
builder.setTitle("Select an Animal");
// Add a list
final String[] animals = {"Horse", "Cow", "Camel", "Sheep", "Goat"};
builder.setItems(animals, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String animal = animals[which];
dialog.dismiss(); // Close Dialog
// Do some thing....
// For example: Call method of MainActivity.
Toast.makeText(activity,"You select: " + animal,
Toast.LENGTH_SHORT).show();
// activity.someMethod(animal);
}
});
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Cancel" button with OnClickListener.
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(activity,"You choose No button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
builder.setNegativeButtonIcon(negativeIcon);
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
5. Ví dụ AlertDialog (Single Choice)
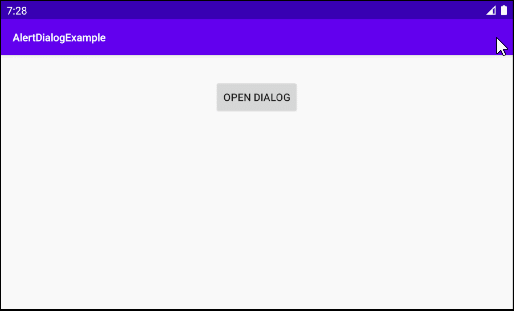
AlertDialogSingleChoiceExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.DialogInterface;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import java.util.HashSet;
import java.util.Set;
public class AlertDialogSingleChoiceExample {
public static void showAlertDialog(final Activity activity) {
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
// Set Title.
builder.setTitle("Select an Animal");
// Add a list
final String[] animals = {"Horse", "Cow", "Camel", "Sheep", "Goat"};
int checkedItem = 3; // Sheep
final Set<String> selectedItems = new HashSet<String>();
selectedItems.add(animals[checkedItem]);
builder.setSingleChoiceItems(animals, checkedItem, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// Do Something...
selectedItems.clear();
selectedItems.add(animals[which]);
}
});
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Yes" button with OnClickListener.
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
if(selectedItems.isEmpty()) {
return;
}
String animal = selectedItems.iterator().next();
// Close Dialog
dialog.dismiss();
// Do something, for example: Call a method of Activity...
Toast.makeText(activity,"You select " + animal,
Toast.LENGTH_SHORT).show();
}
});
// Create "Cancel" button with OnClickListener.
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(activity,"You choose Cancel button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
6. Ví dụ AlertDialog (Multi Choice)
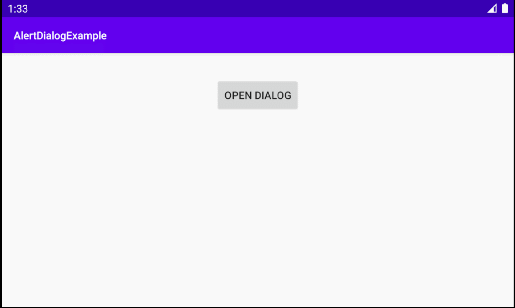
AlertDialogMultiChoiceExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.DialogInterface;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
import java.util.HashSet;
import java.util.Set;
public class AlertDialogMultiChoiceExample {
public static void showAlertDialog(final Activity activity) {
AlertDialog.Builder builder = new AlertDialog.Builder(activity);
// Set Title.
builder.setTitle("Select an Animal");
// Add a list
final String[] animals = {"Horse", "Cow", "Camel", "Sheep", "Goat"};
final boolean[] checkedInfos = new boolean[]{false, false, false, true, false}; // Sheep
builder.setMultiChoiceItems(animals, checkedInfos, new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface dialog, int which, boolean isChecked) {
checkedInfos[which] = isChecked;
}
});
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "Yes" button with OnClickListener.
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
// Close Dialog
dialog.dismiss();
String s= null;
for(int i=0; i< animals.length;i++) {
if(checkedInfos[i]) {
if(s == null) {
s = animals[i];
} else {
s+= ", " + animals[i];
}
}
}
s = s == null? "":s;
// Do something, for example: Call a method of Activity...
Toast.makeText(activity,"You select " + s,
Toast.LENGTH_SHORT).show();
}
});
// Create "Cancel" button with OnClickListener.
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(activity,"You choose Cancel button",
Toast.LENGTH_SHORT).show();
// Cancel
dialog.cancel();
}
});
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
7. Ví dụ tùy biến Title Area
Bằng cách sử dụng phương thức setCustomTitle(View) bạn có thể tùy biến vùng tiêu đề (Title area) của AlertDialog:
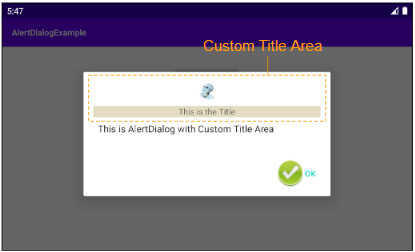
Và có thể sử dụng Android Resource File để tạo giao diện cho vùng tiêu đề (Title area).
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Custom Title Area.
LayoutInflater inflater = context.getLayoutInflater();
View view = inflater.inflate(R.layout.layout_custom_title, null);
builder.setCustomTitle(view);
...
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
OK, tạo một Layout Resource File:
- File > New > Android Resource File
- File Name: layout_custom_title.xml
- Resource type: Layout
- Directory name: layout
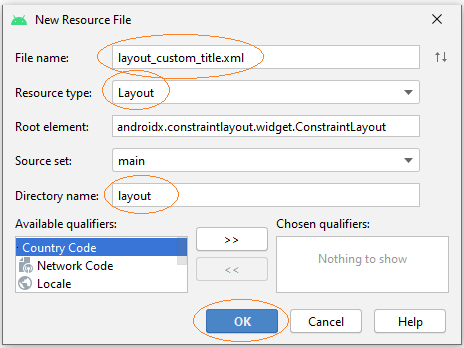
Thiết kế giao diện cho vùng tiêu đề (Title area):
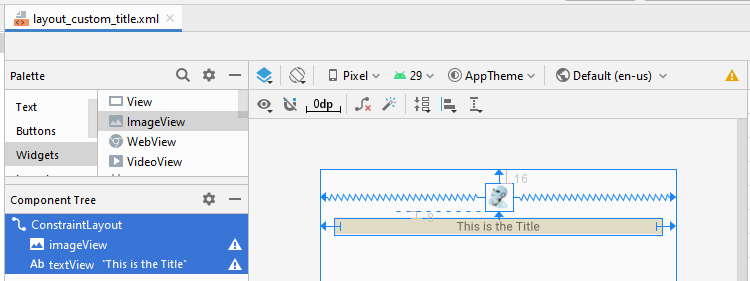
layout_custom_title.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/icon_title" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:background="#E3DCC4"
android:gravity="center"
android:text="This is the Title"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
AlertDialogCustomTitleExample.java
package org.o7planning.alertdialogexample;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Toast;
import androidx.appcompat.app.AlertDialog;
public class AlertDialogCustomTitleExample {
public static void showAlertDialog(final Activity context) {
final Drawable positiveIcon = context.getResources().getDrawable(R.drawable.icon_positive);
AlertDialog.Builder builder = new AlertDialog.Builder(context);
// Custom Title Area.
LayoutInflater inflater = context.getLayoutInflater();
View view = inflater.inflate(R.layout.layout_custom_title, null);
builder.setCustomTitle(view);
// Message.
builder.setMessage("This is AlertDialog with Custom Title Area");
//
builder.setCancelable(true);
builder.setIcon(R.drawable.icon_title);
// Create "OK" button with OnClickListener.
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
}
});
builder.setPositiveButtonIcon(positiveIcon);
// Create AlertDialog:
AlertDialog alert = builder.create();
alert.show();
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More