Hướng dẫn và ví dụ Android CheckBox
1. Android CheckBox
Trong Android, CheckBox là một nút có hai trạng thái checked (được chọn) và unchecked (không được chọn), nó là một thành phần cơ bản và được sử dụng rất thường xuyên trong các ứng dụng Android.
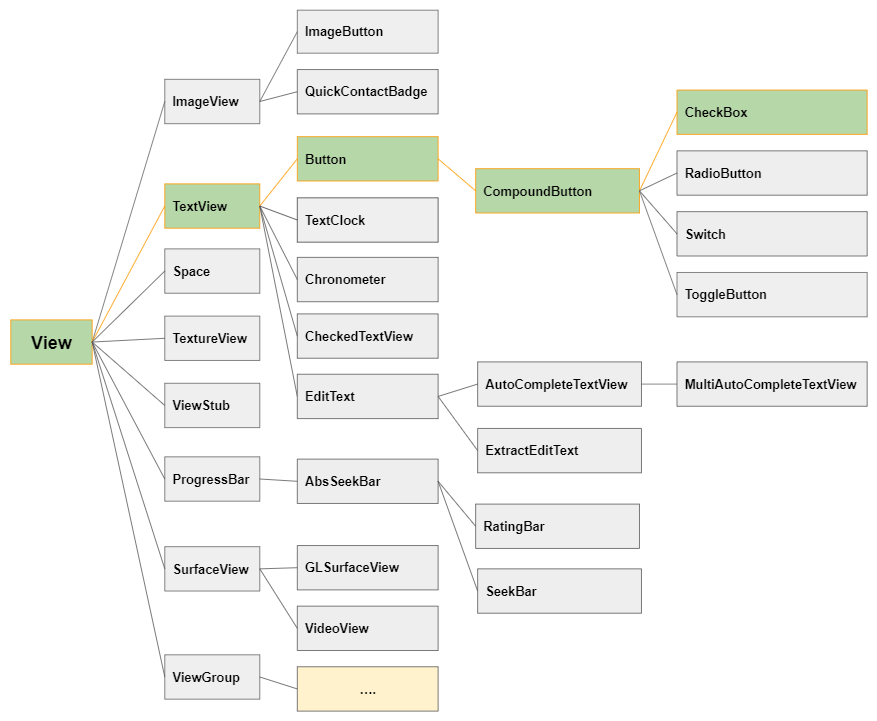
Về cơ bản, bạn có thể sử dụng nhiều CheckBox trong ứng dụng để cho phép người dùng lựa chọn một hoặc nhiều tùy chọn trong một tập hợp các giá trị.
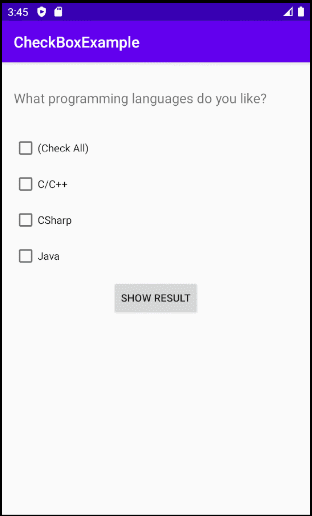
Theo mặc định các CheckBox có trạng thái unchecked, bạn có thể thay đổi trạng thái của nó thông qua thuộc tính android:checked.
<CheckBox
android:id="@+id/someId"
android:checked="true"
... />
Một vài thuộc tính quan trọng của CheckBox:
android:checked | Chỉ định trạng thái hiện thời cho CheckBox. |
android:gravity | Căn lề (align) cho văn bản của CheckBox. Các giá trị có thể là left, right, center, top, ... |
android:text | Sét đặt nội dung text (văn bản) cho CheckBox. |
android:textColor | Sét đặt mầu chữ của văn bản. |
android:textSize | Sét đặt kích thước phông chữ của văn bản. |
android:textStyle | Sét đặt kiểu dáng cho văn bản (bold, italic, bolditalic). |
android:background | Sét đặt mầu nền cho CheckBox. |
android:padding | Sét đặt padding cho CheckBox. |
android:onClick | Tên của phương thức sẽ được gọi khi người dùng click vào CheckBox. |
toggle()
Cả 4 lớp ToggleButton, CheckBox, RadioButton, Switch đều là lớp con của CompoundButton, vì vậy chúng được thừa kế phương thức toggle(), Đây là phương thức thường được sử dụng để chuyển đổi trạng thái của chúng từ Checked (ON) sang Unchecked (OFF), và ngược lại.
CompoundButton button = (CheckBox) findViewById(R.id.checkBox);
button.toggle();
2. CheckBox Events
Có khá nhiều các sự kiện liên quan tới một CheckBox, nhưng 2 sự kiện sau được sử dụng thường xuyên nhất:
- checkBox.setOnClickListener(View.OnClickListener)
- checkBox.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
On Click Event:
Sự kiện xẩy ra khi người dùng nhấn (click) vào CheckBox, cũng giống như hành động của người dùng khi nhấn vào một Button.
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean checked = ((CheckBox) v).isChecked();
// Check which checkbox was clicked
if (checked){
// Your code
}
else{
// Your code
}
}
});
On Checked Change Event:
Sự kiện xẩy ra khi CheckBox thay đổi trạng thái, do hành động của người dùng hoặc do tác dụng của việc gọi phương thức checkBox.setChecked(newState), ..
CheckBox chk = (CheckBox) findViewById(R.id.chk1);
chk.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if(isChecked) {
// Your code
} else {
// Your code
}
}
});
3. Ví dụ CheckBox
Xem trước ví dụ:
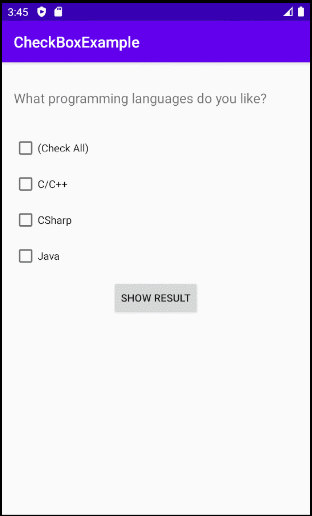
Giao diện của ứng dụng ví dụ:
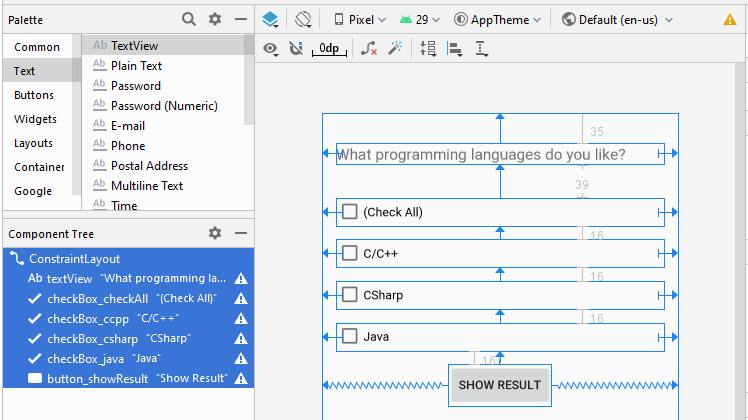
Chú ý: Nếu bạn quan tâm tới các bước để thiết kế giao diện của ứng dụng này xin hãy xem phần phụ lục phía cuối bài viết.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="35dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="What programming languages do you like?"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<CheckBox
android:id="@+id/checkBox_checkAll"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="39dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Check All)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<CheckBox
android:id="@+id/checkBox_ccpp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="C/C++"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_checkAll" />
<CheckBox
android:id="@+id/checkBox_csharp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="CSharp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_ccpp" />
<CheckBox
android:id="@+id/checkBox_java"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Java"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_csharp" />
<Button
android:id="@+id/button_showResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Show Result"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_java" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.checkboxexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private CheckBox checkBoxCheckAll;
private CheckBox checkBoxCcpp;
private CheckBox checkBoxCsharp;
private CheckBox checkBoxJava;
private Button buttonShowResult;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.checkBoxCheckAll = (CheckBox) this.findViewById(R.id.checkBox_checkAll);
this.checkBoxCcpp = (CheckBox) this.findViewById(R.id.checkBox_ccpp);
this.checkBoxCsharp = (CheckBox) this.findViewById(R.id.checkBox_csharp);
this.checkBoxJava = (CheckBox) this.findViewById(R.id.checkBox_java);
this.buttonShowResult = (Button) this.findViewById(R.id.button_showResult);
this.buttonShowResult.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showResult();
}
});
this.checkBoxCheckAll.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
checkAllCheckedChange(isChecked);
}
});
}
private void showResult() {
String message = null;
if(this.checkBoxCcpp.isChecked()) {
message = this.checkBoxCcpp.getText().toString();
}
if(this.checkBoxCsharp.isChecked()) {
if(message== null) {
message = this.checkBoxCsharp.getText().toString();
} else {
message += ", " + this.checkBoxCsharp.getText().toString();
}
}
if(this.checkBoxJava.isChecked()) {
if(message== null) {
message = this.checkBoxJava.getText().toString();
} else {
message += ", " + this.checkBoxJava.getText().toString();
}
}
message = message == null? "You select nothing": "You select: " + message;
Toast.makeText(this, message, Toast.LENGTH_LONG).show();
}
// When "Check All" change state.
private void checkAllCheckedChange(boolean isChecked) {
this.checkBoxCsharp.setChecked(isChecked);
this.checkBoxCcpp.setChecked(isChecked);
this.checkBoxJava.setChecked(isChecked);
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More