Hướng dẫn và ví dụ Android Camera
1. Android Camera
Camera là một thiết bị cho phép bạn chụp hình hoặc quay video. Trong Android có 2 cách để bạn làm việc với Camera.
Cách 1:
Trên hệ điều hành Android đã có sẵn ứng dụng để làm việc với Camera, ứng dụng của bạn có thể gọi ứng dụng đó thông qua một Intent không tường minh (Implicit Intent) để yêu cầu một hành động nào đó với Camera, chẳng hạn yêu cầu mở Camera và chụp ảnh, hoặc yêu cầu mở Camera để quay video, và sau đó nhận kết quả trả về.
Cách 2:
Android cung cấp cho bạn các API để làm việc trực tiếp với Camera.
Với Android Level < 21 bạn có thể làm việc trực tiếp với Camera thông qua class android.hardware.Camera, tuy nhiên class này đã lỗi thời (Deprected) và không còn được sử dụng trong Android Level >= 21, khuyến cáo bạn nên sử dụng Camera2 API.
Với Android Level < 21 bạn có thể làm việc trực tiếp với Camera thông qua class android.hardware.Camera, tuy nhiên class này đã lỗi thời (Deprected) và không còn được sử dụng trong Android Level >= 21, khuyến cáo bạn nên sử dụng Camera2 API.
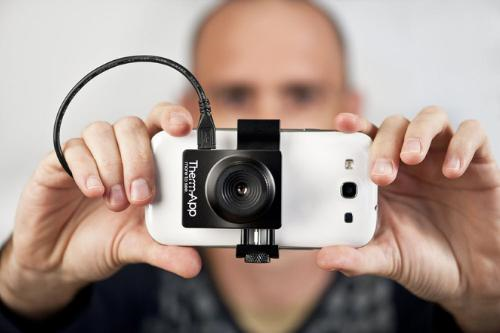
Trong tài liệu này tôi sẽ hướng dẫn bạn sử dụng Intent không tường minh để gọi vào ứng dụng Camera có sẵn trong hệ thống yêu cầu mở Camera để chụp hình hoặc quay video.
Bạn có thể xem hướng dẫn sử dụng Camera2 API tại:
- Android Camera API2
2. Tổng quan
Trên hệ điều hành Android đã có sẵn ứng dụng để làm việc với Camera, trên ứng dụng của bạn có thể tạo một Intent không tường minh (implicit intent) để gọi tới ứng dụng này, yêu cầu mở Camera để quay phim hoặc chụp hình.
// Tạo một Intent không tường minh,
// để yêu cầu hệ thống mở Camera chuẩn bị chụp hình.
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
int REQUEST_ID_IMAGE_CAPTURE = 100;
// Start Activity chụp hình, và chờ đợi kết quả trả về.
this.startActivityForResult(intent, REQUEST_ID_IMAGE_CAPTURE);
Các loại Intent cho Camera:
Loại Intent | Mô tả |
ACTION_IMAGE_CAPTURE_SECURE | Nó sẽ trả về một Image được chụp từ Camera, khi thiết bị được bảo mật |
ACTION_VIDEO_CAPTURE | Nó sẽ gọi một ứng dụng video trong Android để quay video từ Camera. |
EXTRA_SCREEN_ORIENTATION | Sử dụng để sét đặt chiều của màn hình là "vertical" hoặc "landscape" |
EXTRA_FULL_SCREEN | Nó được sử dụng để điều khiển giao diện người dùng của ViewImage |
INTENT_ACTION_VIDEO_CAMERA | Sử dụng để khởi động Camera ở chế độ quay video. |
EXTRA_SIZE_LIMIT | Sử dụng để chỉ định giá trị lớn nhất cho kích thước file ảnh và video. |
Trong trường hợp bạn muốn lưu trữ ảnh chụp hoặc video vừa quay được trên thiết bị bạn cần cấu hình cho phép đọc và ghi dữ liệu vào thiết bị. Cấu hình trên AndroidManifest.xml.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Với Android Level >= 23, bạn cần phải sử dụng code để hỏi người dùng cho phép đọc và ghi dữ liệu vào thiết bị.
// Với Android Level >= 23 bạn phải hỏi người dùng cho phép ghi dữ liệu vào thiết bị.
if (android.os.Build.VERSION.SDK_INT >= 23) {
// Kiểm tra quyền đọc/ghi dữ liệu vào thiết bị lưu trữ ngoài.
int readPermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.READ_EXTERNAL_STORAGE);
int writePermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.WRITE_EXTERNAL_STORAGE);
if (writePermission != PackageManager.PERMISSION_GRANTED ||
readPermission != PackageManager.PERMISSION_GRANTED) {
// Nếu không có quyền, cần nhắc người dùng cho phép.
this.requestPermissions(
new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
REQUEST_ID_READ_WRITE_PERMISSION
);
}
}
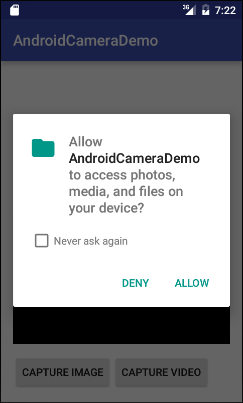
Xử lý khi người dùng trả lời yêu cầu.
// Khi yêu cầu hỏi người dùng được trả về (Chấp nhận hoặc không chấp nhận).
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case REQUEST_ID_READ_WRITE_PERMISSION: {
// Chú ý: Nếu yêu cầu bị hủy, mảng kết quả trả về là rỗng.
// Người dùng đã cấp quyền (đọc/ghi).
if (grantResults.length > 1
&& grantResults[0] == PackageManager.PERMISSION_GRANTED
&& grantResults[1] == PackageManager.PERMISSION_GRANTED) {
Toast.makeText(this, "Permission granted!", Toast.LENGTH_LONG).show();
this.captureVideo();
}
// Hủy bỏ hoặc bị từ chối.
else {
Toast.makeText(this, "Permission denied!", Toast.LENGTH_LONG).show();
}
break;
}
}
}
3. Camera Example
Tạo mới một project có tên AndroidCameraDemo:
- File > New > New Project > Empty Activity
- Name: AndroidCameraDemo
- Package name: org.o7planning.androidcamerademo
- Language: Java
Thêm cấu hình cho phép đọc và ghi dữ liệu trên thiết bị.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.androidcamerademo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Thiết kế giao diện ví dụ:
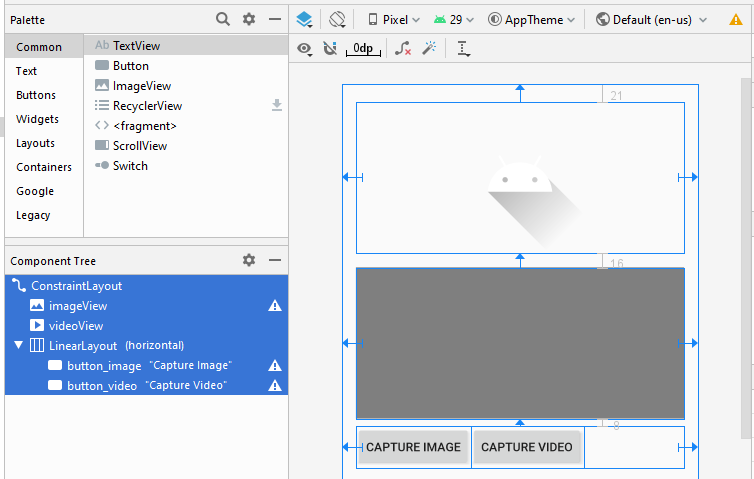
Nếu bạn quan tâm tới các bước để thiết kế giao diện ứng dụng này hãy xem phần phụ lục phía cuối bài viết.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="175dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="21dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/ic_launcher_foreground"
tools:ignore="VectorDrawableCompat" />
<VideoView
android:id="@+id/videoView"
android:layout_width="0dp"
android:layout_height="175dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/videoView">
<Button
android:id="@+id/button_image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Capture Image" />
<Button
android:id="@+id/button_video"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="0"
android:text="Capture Video" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidcamerademo;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.os.Bundle;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.net.Uri;
import android.os.Environment;
import android.os.StrictMode;
import android.provider.MediaStore;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.Toast;
import android.widget.VideoView;
import java.io.File;
public class MainActivity extends AppCompatActivity {
private Button buttonImage;
private Button buttonVideo;
private VideoView videoView;
private ImageView imageView;
private static final int REQUEST_ID_READ_WRITE_PERMISSION = 99;
private static final int REQUEST_ID_IMAGE_CAPTURE = 100;
private static final int REQUEST_ID_VIDEO_CAPTURE = 101;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.buttonImage = (Button) this.findViewById(R.id.button_image);
this.buttonVideo = (Button) this.findViewById(R.id.button_video);
this.videoView = (VideoView) this.findViewById(R.id.videoView);
this.imageView = (ImageView) this.findViewById(R.id.imageView);
this.buttonImage.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
captureImage();
}
});
this.buttonVideo.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
askPermissionAndCaptureVideo();
}
});
}
private void captureImage() {
// Create an implicit intent, for image capture.
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Start camera and wait for the results.
this.startActivityForResult(intent, REQUEST_ID_IMAGE_CAPTURE);
}
private void askPermissionAndCaptureVideo() {
// With Android Level >= 23, you have to ask the user
// for permission to read/write data on the device.
if (android.os.Build.VERSION.SDK_INT >= 23) {
// Check if we have read/write permission
int readPermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.READ_EXTERNAL_STORAGE);
int writePermission = ActivityCompat.checkSelfPermission(this,
Manifest.permission.WRITE_EXTERNAL_STORAGE);
if (writePermission != PackageManager.PERMISSION_GRANTED ||
readPermission != PackageManager.PERMISSION_GRANTED) {
// If don't have permission so prompt the user.
this.requestPermissions(
new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,
Manifest.permission.READ_EXTERNAL_STORAGE},
REQUEST_ID_READ_WRITE_PERMISSION
);
return;
}
}
this.captureVideo();
}
private void captureVideo() {
try {
// Create an implicit intent, for video capture.
Intent intent = new Intent(MediaStore.ACTION_VIDEO_CAPTURE);
// The external storage directory.
File dir = Environment.getExternalStorageDirectory();
if (!dir.exists()) {
dir.mkdirs();
}
// file:///storage/emulated/0/myvideo.mp4
String savePath = dir.getAbsolutePath() + "/myvideo.mp4";
File videoFile = new File(savePath);
Uri videoUri = Uri.fromFile(videoFile);
// Specify where to save video files.
intent.putExtra(MediaStore.EXTRA_OUTPUT, videoUri);
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
// ================================================================================================
// To Fix Error (**)
// ================================================================================================
StrictMode.VmPolicy.Builder builder = new StrictMode.VmPolicy.Builder();
StrictMode.setVmPolicy(builder.build());
// ================================================================================================
// You may get an Error (**) If your app targets API 24+
// "android.os.FileUriExposedException: file:///storage/emulated/0/xxx exposed beyond app through.."
// Explanation: https://stackoverflow.com/questions/38200282
// ================================================================================================
// Start camera and wait for the results.
this.startActivityForResult(intent, REQUEST_ID_VIDEO_CAPTURE); // (**)
} catch(Exception e) {
Toast.makeText(this, "Error capture video: " +e.getMessage(), Toast.LENGTH_LONG).show();
e.printStackTrace();
}
}
// When you have the request results
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case REQUEST_ID_READ_WRITE_PERMISSION: {
// Note: If request is cancelled, the result arrays are empty.
// Permissions granted (read/write).
if (grantResults.length > 1
&& grantResults[0] == PackageManager.PERMISSION_GRANTED
&& grantResults[1] == PackageManager.PERMISSION_GRANTED) {
Toast.makeText(this, "Permission granted!", Toast.LENGTH_LONG).show();
this.captureVideo();
}
// Cancelled or denied.
else {
Toast.makeText(this, "Permission denied!", Toast.LENGTH_LONG).show();
}
break;
}
}
}
// When results returned
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_ID_IMAGE_CAPTURE) {
if (resultCode == RESULT_OK) {
Bitmap bp = (Bitmap) data.getExtras().get("data");
this.imageView.setImageBitmap(bp);
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(this, "Action canceled", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Action Failed", Toast.LENGTH_LONG).show();
}
} else if (requestCode == REQUEST_ID_VIDEO_CAPTURE) {
if (resultCode == RESULT_OK) {
Uri videoUri = data.getData();
Log.i("MyLog", "Video saved to: " + videoUri);
Toast.makeText(this, "Video saved to:\n" +
videoUri, Toast.LENGTH_LONG).show();
this.videoView.setVideoURI(videoUri);
this.videoView.start();
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(this, "Action Cancelled.",
Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Action Failed",
Toast.LENGTH_LONG).show();
}
}
}
}
Ứng dụng sẽ lưu video xuống SD Card của thiết bị giả lập (Emulator), vì vậy hãy đảm bảo rằng bạn đã thiết lập SD Card.
OK, bây giờ bạn có thể chạy ứng dụng. Ở đây tôi chạy ứng dụng trên thiết bị mô phỏng với Camera cũng là camera mô phỏng.
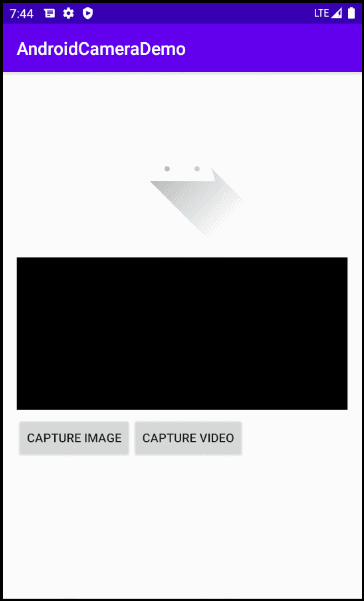
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More