Hướng dẫn và ví dụ Android TextInputLayout
1. Android TextInputLayout
TextInputLayout là một thành phần giao diện chứa một trường đầu vào văn bản (Text Input Field) và hỗ trợ trường đầu vào văn bản này về mặt trực quan. Nếu EditText đang được sử dụng, hãy đảm bảo rằng android:background của nó là @null để TextInputLayout có thể sét đặt nền thích hợp cho nó.
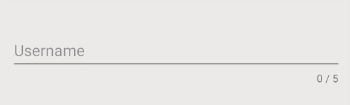
Văn bản gợi ý (hint text) tự động nổi lên khi người dùng focus vào EditText.
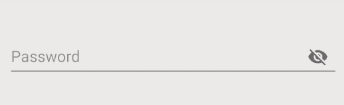
Một trường mật khẩu (Password field) với một ImageView bên phải cho phép hiển thị mật khẩu.
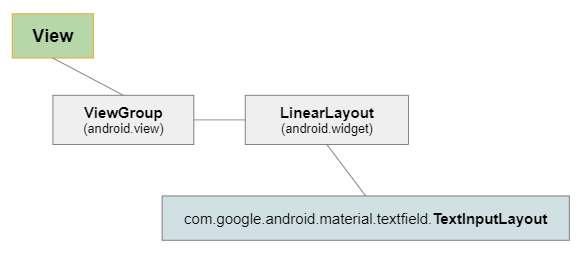
TextInputLayout là một lớp con của LinearLayout, vì vậy nó có thể sắp xếp các View con trên một hàng hoặc một cột. Một trong các View con của nó là một trường đầu vào văn bản (Text input field), chẳng hạn như EditText, các View con khác đóng vai trò hỗ trợ về mặt trực quan.
Chú ý: Bạn cũng có thể đặt các TextInputLayout lồng nhau để có được một thành phần giao diện phức tạp hơn.
Library:
TextInputLayout không sẵn có trong thư viện tiêu chuẩn của Android, vì vậy để sử dụng bạn cần cài đặt nó vào project của bạn từ Palette của cửa sổ thiết kế hoặc khai báo thư viện này một cách thủ công.
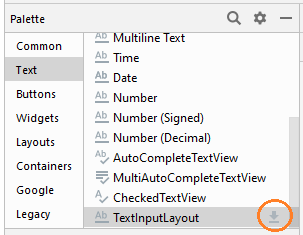
Sau khi cài đặt thư viện này từ Palette bạn sẽ thấy nó được khai báo trong tập tin build.gradle (Module: app):
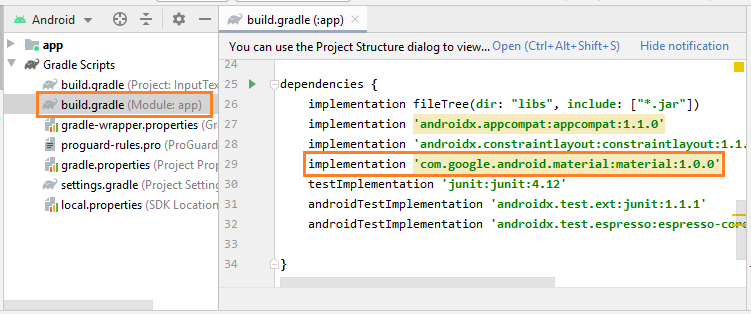
implementation 'com.google.android.material:material:1.0.0'
2. Floating Hint
Văn bản gợi ý (hint text) tự động nổi lên khi người dùng focus vào một EditText là tính năng hỗ trợ cơ bản của TextInputLayout, bạn có thể sử dụng nó mà không cần phải viết thêm bất kỳ một dòng mã Java bổ sung nào.
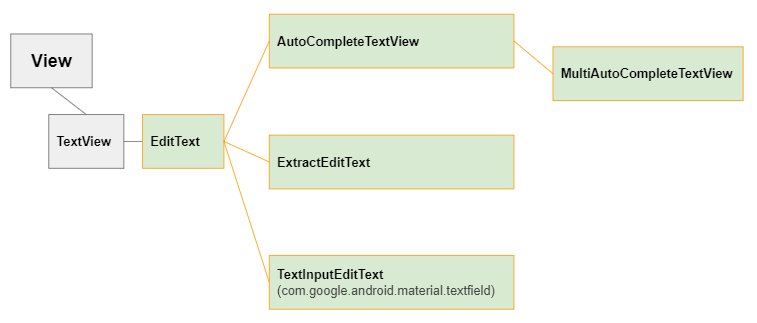
Kéo thả một TextInputLayout vào giao diện, theo mặc định nó sẽ chứa một View con là TextInputEditText, đây là một lớp hậu duệ của EditText.
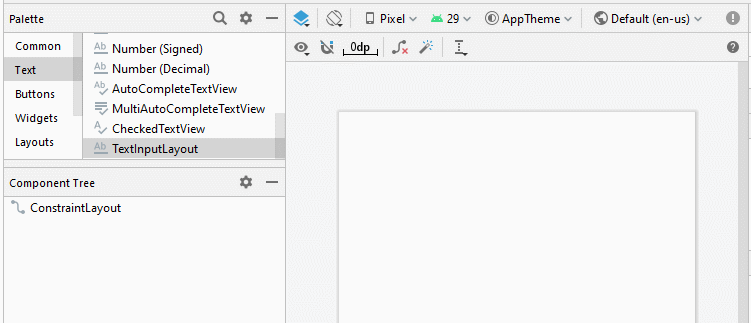
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputLayout"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="50dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Username" />
</com.google.android.material.textfield.TextInputLayout>
Và đây là kết quả nhận được:
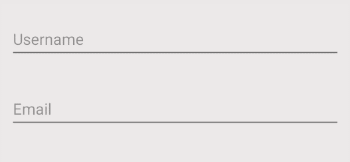
Các thuộc tính liên quan tới tính năng "Floating Hint" bao gồm:
- app:hintEnabled (Default true)
- app:hintAnimationEnabled (Default true)
- app:hintTextAppearance
app:hintEnabled
Thuộc tính app:hintEnabled được sử dụng để enable/disable (bật/tắt) tính năng "Floating Hint" của TextInputLayout. Giá trị mặc định của nó là true.
app:hintAnimationEnabled
Thuộc tính app:hintAnimationEnabled chỉ định có hay không hiệu ứng hoạt hình khi văn bản gợi ý (Hint text) nổi nên. Giá trị mặc định là true.
app:hintTextAppearance
Sét đặt mầu sắc, kích thước, kiểu dáng,... cho văn bản gợi ý (Hint text).
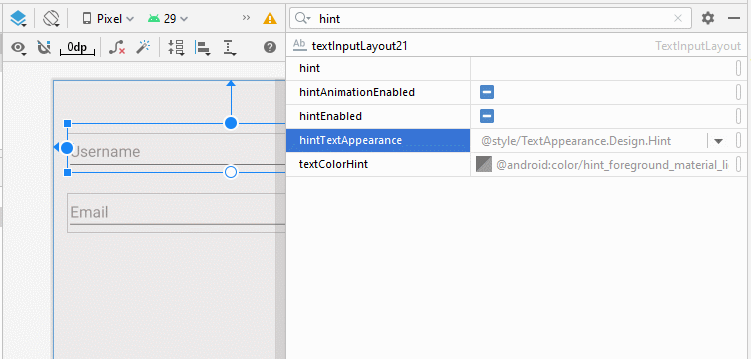
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Large"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Medium"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Small"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Body1"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Body2"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Display1"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Display2"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Display3"
- app:hintTextAppearance="@style/TextAppearance.AppCompat.Display4"
- ...
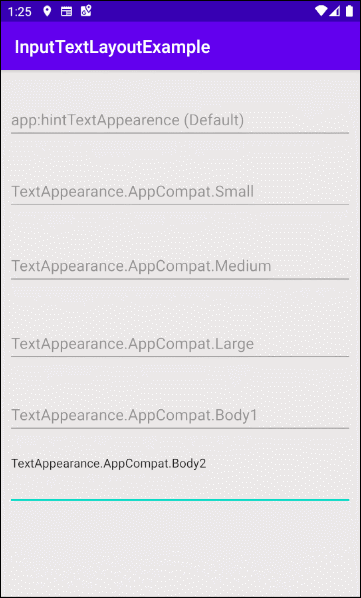
3. Character Counting
TextInputLayout cũng hỗ trợ tính năng đếm số ký tự, đây cũng là một trong các tính năng khá thường xuyên được sử dụng trong các ứng dụng.
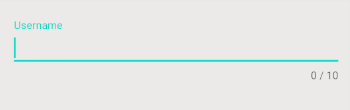
Kéo thả một TextInputLayout vào giao diện, theo mặc định nó sẽ chứa một TextInputEditText:
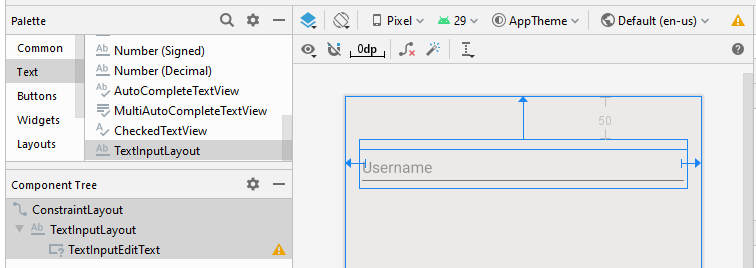
Tiếp theo: Thiết lập giá trị cho một vài thuộc tính của TextInputLayout.
- app:counterEnabled = "true"
- app:counterMaxLength: Đây là một thuộc tính tuỳ chọn để chỉ định số lượng ký tự tối đa của văn bản, giá trị này được dùng cho báo cáo. Người dùng vẫn có thể nhập vào một văn bản với số ký tự nhiều hơn.
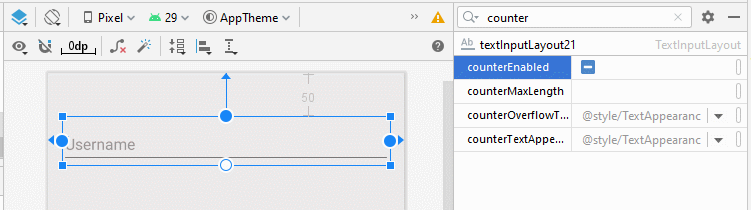
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ECE9E9"
tools:context=".MainActivity">
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputLayout21"
app:counterEnabled="true"
app:counterMaxLength="10"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="50dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Username" />
</com.google.android.material.textfield.TextInputLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
Và kết quả mà bạn nhận được:
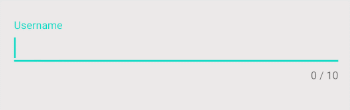
Chú ý: Sử dụng thuộc tính android:maxLength cho Input Field (EditText,..) nếu bạn muốn giới hạn số lượng ký tự của văn bản, và nó được bảo đảm.
4. Password Visibility Toggle
Kéo thả TextInputLayout vào giao diện, theo mặc định nó có sẵn TextInputEditText là một View con. Sau đó, sét đặt thuộc tính android:inputType = "textPassword" cho TextInputEditText.
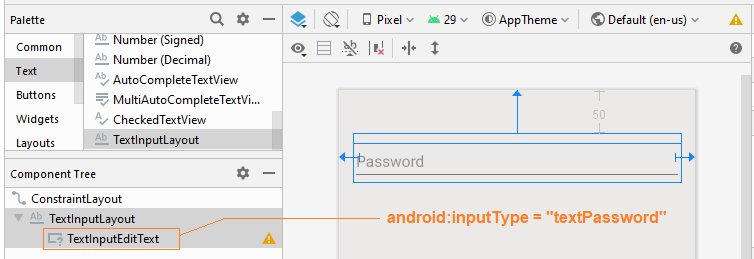
Sét đặt giá trị cho các thuộc tính sau:
- app:passwordToggleEnabled
- app:passwordToggleDrawable
- app:passwordToggleContentDescription
- app:passwordToggleTint
- app:passwordToggleTintMode
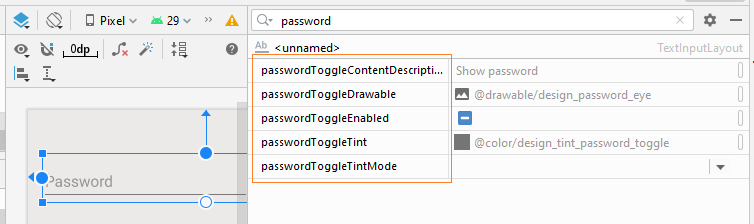
app:passwordEnabled
Thuộc tính app:passwordEnabled="true" là cần thiết để bạn bật tính năng ẩn/hiện mật khẩu cho TextInputLayout, các thuộc tính khác như đề cập ở trên chỉ là các tùy chọn.
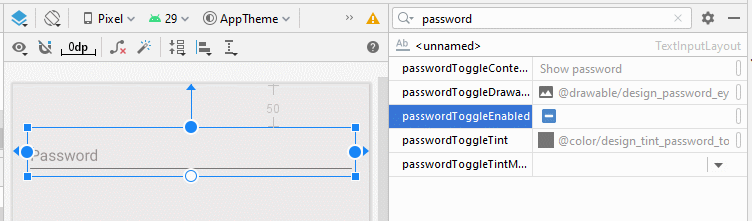
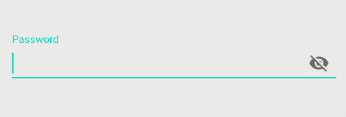
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ECE9E9"
tools:context=".MainActivity">
<com.google.android.material.textfield.TextInputLayout
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="50dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:passwordToggleEnabled="true">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Password"
android:inputType="textPassword" />
</com.google.android.material.textfield.TextInputLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
5. Floating Label Error
Một trong các tính năng khác của TextInputLayout là hiển thị một Label thông báo lỗi cho người dùng. Tuy nhiên để sử dụng tính năng này bạn cần viết mã Java bổ sung để điều khiển việc ẩn/hiện của Label.
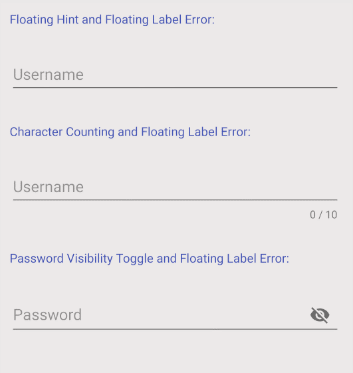
OK, dưới đây là một ví dụ đơn giản:
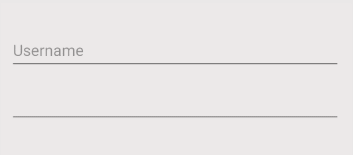
Giao diện của ví dụ:
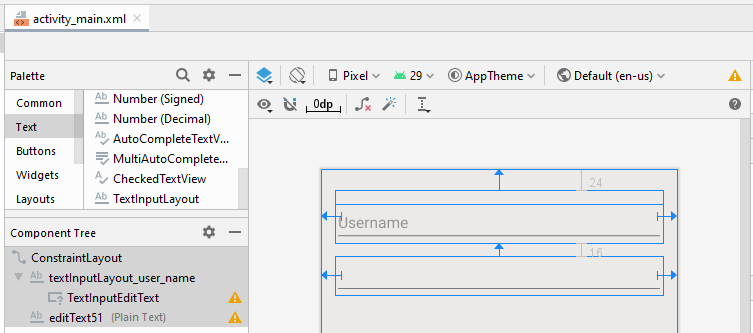
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ECE9E9"
tools:context=".MainActivity">
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputLayout_user_name"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:hintTextAppearance="@style/TextAppearance.AppCompat.Medium"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Username" />
</com.google.android.material.textfield.TextInputLayout>
<EditText
android:id="@+id/editText51"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textInputLayout_user_name" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.inputtextlayoutexample;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import androidx.appcompat.app.AppCompatActivity;
import com.google.android.material.textfield.TextInputLayout;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.setupFloatingLabelError();
}
private void setupFloatingLabelError() {
final TextInputLayout textInputLayoutUserName = (TextInputLayout) findViewById(R.id.textInputLayout_user_name);
textInputLayoutUserName.getEditText().addTextChangedListener(new TextWatcher() {
// ...
@Override
public void onTextChanged(CharSequence text, int start, int count, int after) {
if (text.length() == 0 ) {
textInputLayoutUserName.setError("Username is required");
textInputLayoutUserName.setErrorEnabled(true);
} else if (text.length() < 5 ) {
textInputLayoutUserName.setError("Username is required and length must be >= 5");
textInputLayoutUserName.setErrorEnabled(true);
} else {
textInputLayoutUserName.setErrorEnabled(false);
}
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count,
int after) {
}
@Override
public void afterTextChanged(Editable s) {
}
});
}
}
6. TextInputLayout Styles
Thuộc tính style là một tùy chọn của TextInputLayout, nó cho phép bạn sét đặt kiểu dáng cho TextInputLayout. Có một vài kiểu dáng sẵn có trong thư viện của Android mà bạn có thể sẵn sàng sử dụng.
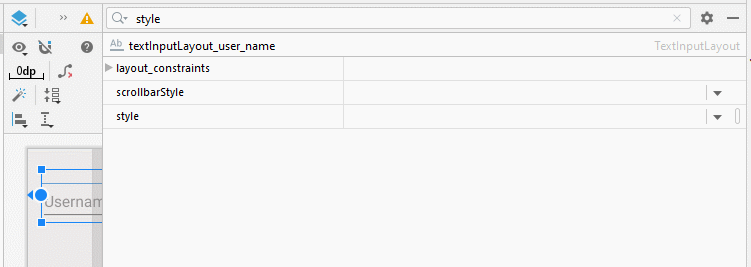
- style="@style/Widget.Design.TextInputLayout"
- style="@style/Widget.MaterialComponents.TextInputLayout.FilledBox"
- style="@style/Widget.MaterialComponents.TextInputLayout.FilledBox.Dense"
- style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox"
- style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
- ...
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox"
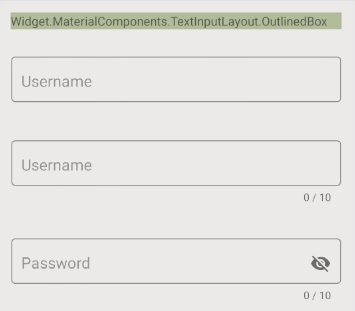
style="@style/Widget.MaterialComponents.TextInputLayout.FilledBox"
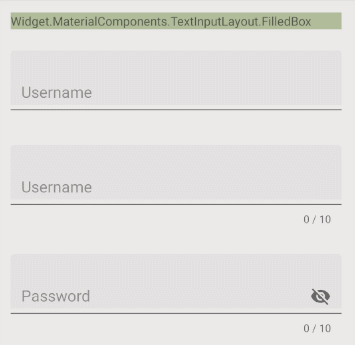
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More