Hướng dẫn và ví dụ Android Chip và ChipGroup
1. Chip
Chip là một thành phần mới được đưa vào Android từ phiên bản SDK 28, về cơ bản là một thành phần giao diện bao gồm biểu tượng (icon) và văn bản, được đặt trong một nền hình chữ nhật với các góc tròn.
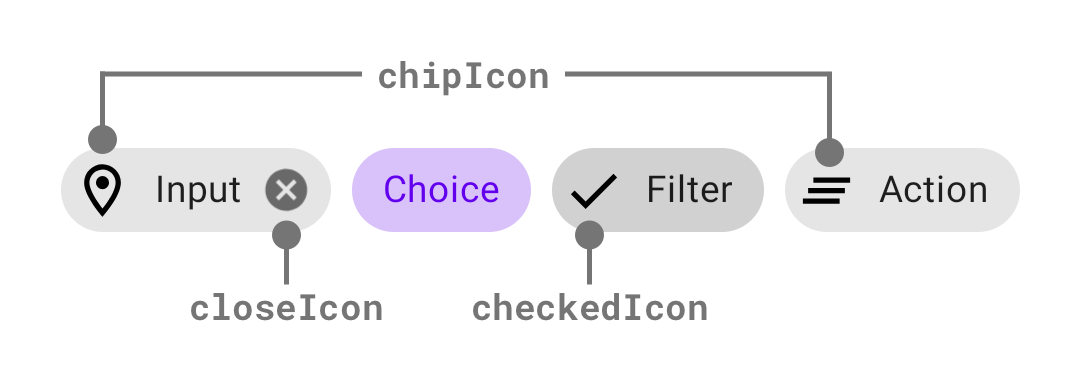
Để có thể sử dụng đối tượng Chip trong dự án bạn cần phải cài đặt nó.
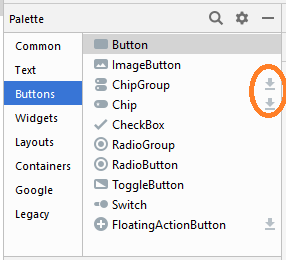
Sau khi cài đặt xong, một thư viện bao gồm Chip sẽ được khai báo trong tập tin build.gradle (Module app).
implementation 'com.google.android.material:material:1.1.0'
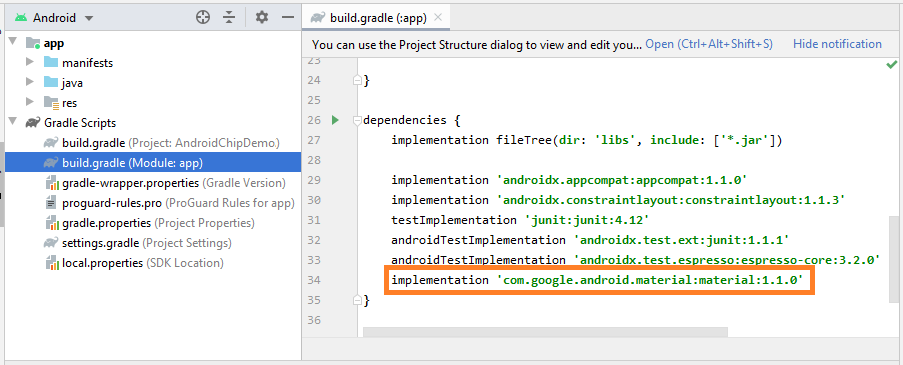
Mã XML của một Chip đơn giản:
Chip Sample
<!-- IMPORTANT: android:theme is required -->
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Action"
android:theme="@style/Theme.MaterialComponents.Light"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Chip Action" />
theme attribute
Thuộc tính (attribute) android:theme là bắt buộc đối với một Chip, nếu không có thuộc tính này toàn bộ ứng dụng của bạn sẽ không hoạt động mà không có một thông báo nào, tôi đã rất ngạc nhiên vì điều đó trong lần đầu tiên sử dụng Chip.
- android:theme="@style/Theme.MaterialComponents"
- android:theme="@style/Theme.MaterialComponents.Light"
- android:theme="@style/Theme.MaterialComponents.***"
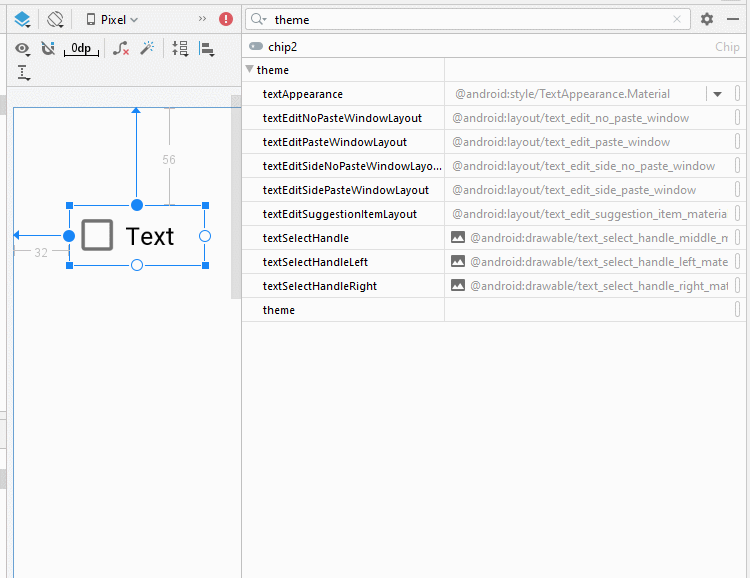
style attribute:
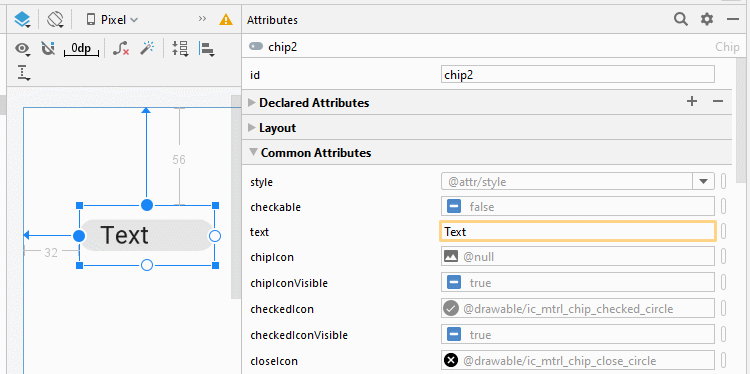
Thuộc tính (attribute) style có 4 giá trị, nó giúp bạn chỉ định kiểu của Chip:
- @style/Widget.MaterialComponents.Chip.Action (Default)
- @style/Widget.MaterialComponents.Chip.Entry
- @style/Widget.MaterialComponents.Chip.Choice
- @style/Widget.MaterialComponents.Chip.Filter
Style Default
Thuộc tính (attribute) style là không bắt buộc, giá trị mặc định của nó là "@style/Widget.MaterialComponents.Chip.Action".
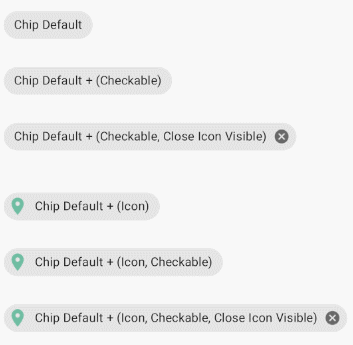
Chip Default Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Default"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Default + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Default + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Action"
Đây là giá trị mặc định của style.
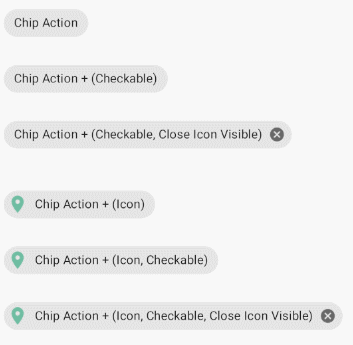
style="@style/Widget.MaterialComponents.Chip.Entry"
Chip Entry = Chip Default + Close Icon + Checkable
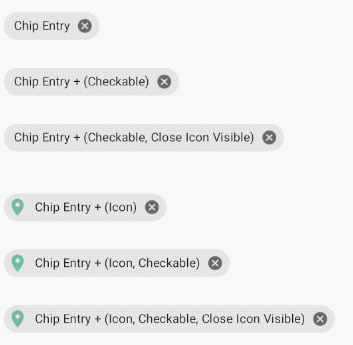
Chip Entry Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Entry"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Entry + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Entry + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Choice"
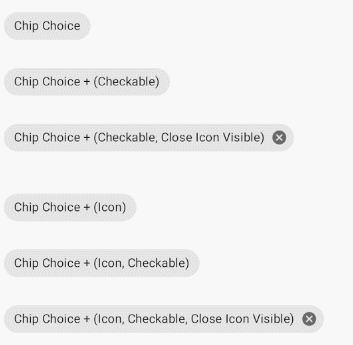
Các đặc điểm của Chip Choice:
- Khi Chip Choice được chọn (selected) mầu nền của nó sẽ đậm hơn.
- Không có biểu tượng check ở bên trái, kể cả khi bạn thiết lập checkable=true.
- Icon - Không hiển thị kể cả khi bạn thiết lập giá trị cho nó.
Chip Choice Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Choice"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Choice + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Choice + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
style="@style/Widget.MaterialComponents.Chip.Filter"
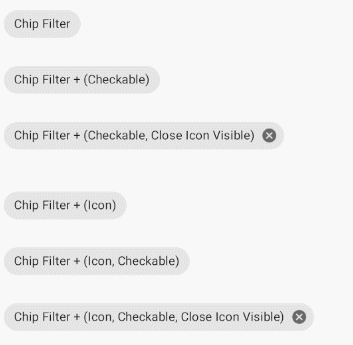
Đặc điểm của Chip Filter:
- Chip Filter = Chip Default + Checkable
- Icon - Sẽ không hiển thị kể cả khi bạn thiết lập giá trị cho nó.
Chip Filter Sample
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip1"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="50dp"
android:text="Chip Filter"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip2"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip1" />
<com.google.android.material.chip.Chip
android:id="@+id/chip3"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip2" />
<com.google.android.material.chip.Chip
android:id="@+id/chip4"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="32dp"
android:checkable="true"
android:text="Chip Filter + (Icon)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip3" />
<com.google.android.material.chip.Chip
android:id="@+id/chip5"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Icon, Checkable)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip4" />
<com.google.android.material.chip.Chip
android:id="@+id/chip6"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="16dp"
android:checkable="true"
android:text="Chip Filter + (Icon, Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:chipIcon="@drawable/my_icon"
app:closeIconVisible="true"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip5" />
</androidx.constraintlayout.widget.ConstraintLayout>
2. Chip Theme
Thuộc tính (attribute) android:theme là bắt buộc đối với một Chip. Ứng dụng của bạn có thể không hoạt động nếu Chip không có thuộc tính này, hoặc bạn sét đặt một giá trị không hợp lệ cho nó.
Các giá trị có thể là:
- android:theme="@style/Theme.MaterialComponents.Light"
- android:theme="@style/Theme.MaterialComponents.Bridge"
- android:theme="@style/Theme.MaterialComponents.DayNight"
- .....
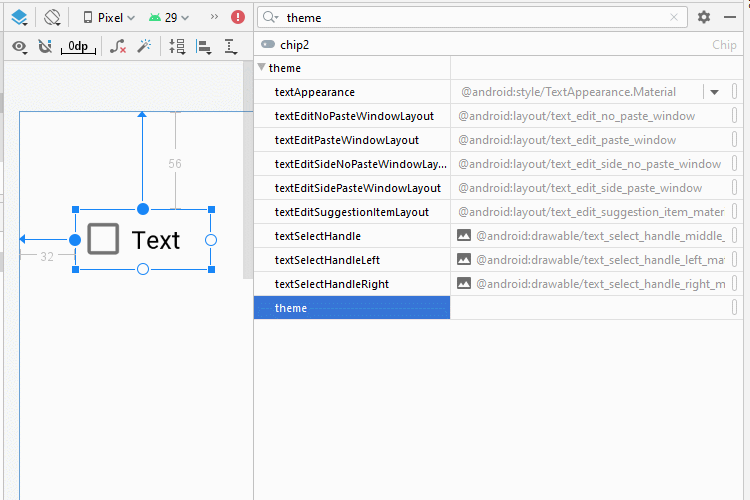
android:theme="@style/Theme.MaterialComponents.Light"
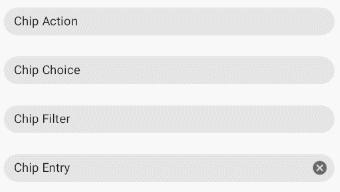
android:theme="@style/Theme.MaterialComponents.Bridge"
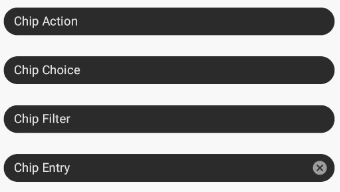
3. Chip Listener
Có rất nhiều các sự kiện liên quan tới Chip, nhưng về cơ bản có 3 sự kiện thông dụng sau:
- chip.setOnClickListener(View.OnClickListener)
- chip.setOnCloseIconClickListener(View.OnClickListener)
- chip.setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
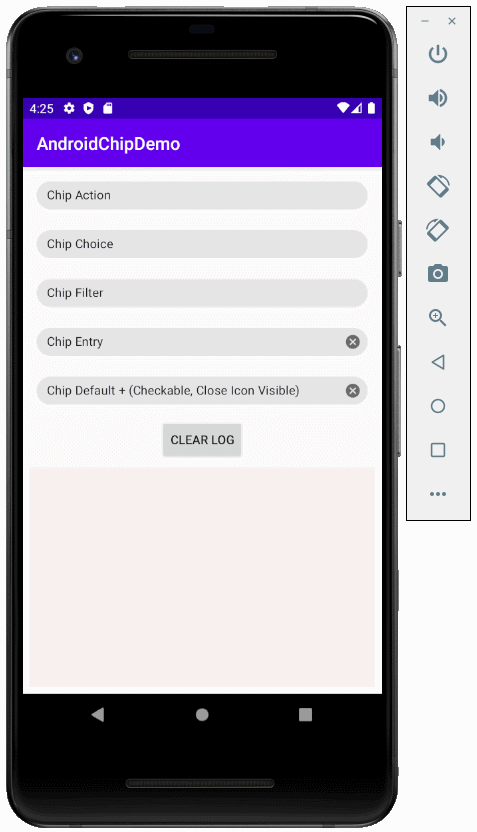
main_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.google.android.material.chip.Chip
android:id="@+id/chip_action"
style="@style/Widget.MaterialComponents.Chip.Action"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:clickable="true"
android:text="Chip Action"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_choice"
style="@style/Widget.MaterialComponents.Chip.Choice"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Choice"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_action" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_filter"
style="@style/Widget.MaterialComponents.Chip.Filter"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Filter"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_choice" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_entry"
style="@style/Widget.MaterialComponents.Chip.Entry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Chip Entry"
android:theme="@style/Theme.MaterialComponents.Light"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_filter" />
<com.google.android.material.chip.Chip
android:id="@+id/chip_custom"
android:layout_width="0dp"
android:layout_height="48dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:checkable="true"
android:text="Chip Default + (Checkable, Close Icon Visible)"
android:theme="@style/Theme.MaterialComponents.Light"
app:closeIconVisible="true"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_entry" />
<Button
android:id="@+id/button_clear"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Clear Log"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chip_custom" />
<EditText
android:id="@+id/editText_log"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginLeft="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginRight="8dp"
android:layout_marginBottom="8dp"
android:background="#F8EFEF"
android:ems="10"
android:gravity="top|left"
android:inputType="textMultiLine"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_clear" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidchipdemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.EditText;
import com.google.android.material.chip.Chip;
public class MainActivity extends AppCompatActivity {
private Chip chipAction;
private Chip chipChoice;
private Chip chipEntry;
private Chip chipFilter;
private Chip chipCustom;
private EditText editTextLog;
private Button buttonClear;
private View.OnClickListener clickListener;
private View.OnClickListener closeIconClickListener;
private CompoundButton.OnCheckedChangeListener checkedChangeListener;
private static final String LOG_TAG = "AndroidChipDemo";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.chipAction = (Chip) this.findViewById(R.id.chip_action);
this.chipChoice = (Chip) this.findViewById(R.id.chip_choice);
this.chipEntry = (Chip) this.findViewById(R.id.chip_entry);
this.chipFilter = (Chip) this.findViewById(R.id.chip_filter);
this.chipCustom = (Chip) this.findViewById(R.id.chip_custom);
this.editTextLog = (EditText) this.findViewById(R.id.editText_log);
this.buttonClear = (Button) this.findViewById(R.id.button_clear);
//
this.clickListener= new ClickListenerImpl();
this.closeIconClickListener = new CloseIconClickListenerImpl();
this.checkedChangeListener = new CheckedChangeListenerImpl();
//
this.chipAction.setOnClickListener( clickListener);
this.chipAction.setOnCloseIconClickListener(closeIconClickListener);
this.chipAction.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipChoice.setOnClickListener( clickListener);
this.chipChoice.setOnCloseIconClickListener(closeIconClickListener);
this.chipChoice.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipEntry.setOnClickListener( clickListener);
this.chipEntry.setOnCloseIconClickListener(closeIconClickListener);
this.chipEntry.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipFilter.setOnClickListener( clickListener);
this.chipFilter.setOnCloseIconClickListener(closeIconClickListener);
this.chipFilter.setOnCheckedChangeListener(checkedChangeListener);
//
this.chipCustom.setOnClickListener( clickListener);
this.chipCustom.setOnCloseIconClickListener(closeIconClickListener);
this.chipCustom.setOnCheckedChangeListener(checkedChangeListener);
//
this.buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editTextLog.setText("");
}
});
}
private void appendLog(String text) {
this.editTextLog.append(text) ;
this.editTextLog.append("\n");
Log.i(LOG_TAG, text);
}
class ClickListenerImpl implements View.OnClickListener {
@Override
public void onClick(View v) {
appendLog("Clicked");
}
}
class CloseIconClickListenerImpl implements View.OnClickListener {
@Override
public void onClick(View v) {
appendLog("Close Icon Clicked");
}
}
class CheckedChangeListenerImpl implements CompoundButton.OnCheckedChangeListener {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
appendLog("Checked Changed! isChecked? " + isChecked);
}
}
}
4. ChipGroup
ChipGroup được sử dụng để chứa nhiều Chip. Theo mặc định các Chip sẽ được sắp xếp trên nhiều dòng nếu một dòng không thể chứa được tất cả các Chip.
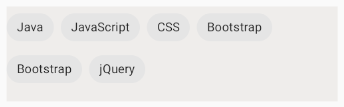
Tuy nhiên bạn có thể thiết lập để đảm bảo rằng các Chip sẽ được đặt trên 1 dòng duy nhất.
<com.google.android.material.chip.ChipGroup
app:singleLine="true"
...>
</com.google.android.material.chip.ChipGroup>
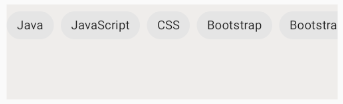
ChipGroup cũng có thể hoạt động giống như một RadioGroup, điều đó có nghĩa là nếu một Chip của nó được sét đặt trạng thái checked, tất cả các Chip khác của nó sẽ có trạng thái unchecked.
<com.google.android.material.chip.ChipGroup
app:singleSelection="true"
...>
</com.google.android.material.chip.ChipGroup>
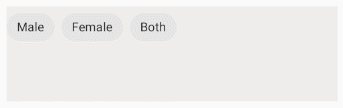
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More